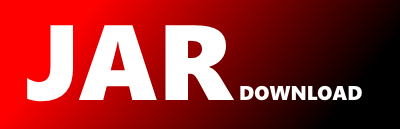
com.opentext.ia.sdk.sip.HashedContents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infoarchive-sdk-core Show documentation
Show all versions of infoarchive-sdk-core Show documentation
A library that makes it quick and easy to create SIPs in InfoArchive
/*
* Copyright (c) 2016-2017 by OpenText Corporation. All Rights Reserved.
*/
package com.opentext.ia.sdk.sip;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
/**
* Hashes of zero or more contents extracted from some source.
* @param The type of source
*/
public class HashedContents {
private final S source;
private final Map contentInfo;
/**
* Store the hashes of a given source's contents.
* @param source The source from which the contents was extracted
* @param contentInfo The reference information and the hashes of the source's contents
*/
public HashedContents(S source, Map contentInfo) {
this.source = Objects.requireNonNull(source);
this.contentInfo = Objects.requireNonNull(contentInfo);
}
/**
* Return the source from which the contents was extracted.
* @return The source from which the contents was extracted
*/
public S getSource() {
return source;
}
public Map getContentInfo() {
return contentInfo;
}
/**
* Return a hash code value for this object.
* @return A hash code value for this object
*/
@Override
public int hashCode() {
return Objects.hash(source, contentInfo);
}
/**
* Indicates whether some other object is "equal to" this one.
* @param obj The reference object with which to compare
* @return true
if this object is the same as the reference object; false
otherwise
*/
@Override
public boolean equals(Object obj) {
if (obj == null || getClass() != obj.getClass()) {
return false;
}
@SuppressWarnings("unchecked")
HashedContents other = (HashedContents)obj;
return source.equals(other.source) && contentInfo.equals(other.contentInfo);
}
/**
* Return a human-readable version of this object.
* @return A human-readable version of this object
*/
@Override
public String toString() {
return source + " with contents " + contentInfo.entrySet()
.stream()
.map(e -> "- " + e.getKey() + "=" + e.getValue())
.collect(Collectors.joining(System.lineSeparator()));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy