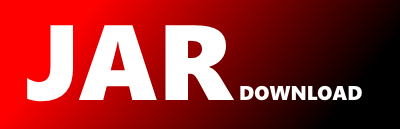
com.opentext.ia.sdk.sip.SipSegmentationStrategy Maven / Gradle / Ivy
/*
* Copyright (c) 2016-2017 by OpenText Corporation. All Rights Reserved.
*/
package com.opentext.ia.sdk.sip;
import java.util.Arrays;
import java.util.Iterator;
import com.opentext.ia.sdk.support.io.DomainObjectTooBigException;
/**
* Strategy for segmenting domain objects into different SIPs.
* @param The type of domain objects to segment into different SIPs
*/
@FunctionalInterface
public interface SipSegmentationStrategy {
/**
* Determine whether to start a new SIP.
* @param domainObject The domain object to be added to either the current SIP or a new one
* @param metrics Metrics about the assembly of the current SIP
* @return true
if a new SIP should be started for the given domain object, or false
if
* the domain object should be stored in the current SIP
*/
boolean shouldStartNewSip(D domainObject, SipMetrics metrics);
/**
* Return a {@linkplain SipSegmentationStrategy} that allows a maximum number of AIUs per SIP.
* @param The type of domain objects to segment into different SIPs
* @param maxAiusPerSip The maximum number of AIUs that may go into a SIP
* @return A {@linkplain SipSegmentationStrategy} that allows a maximum number of AIUs per SIP
*/
static SipSegmentationStrategy byMaxAius(long maxAiusPerSip) {
return (domainObject, metrics) -> metrics.numAius() % maxAiusPerSip == 0;
}
/**
* Return a {@linkplain SipSegmentationStrategy} that allows a maximum number of digital objects per SIP.
* @param The type of domain objects to segment into different SIPs
* @param maxDigitalObjects The maximum number of AIUs that may go into a SIP
* @return A {@linkplain SipSegmentationStrategy} that allows a maximum number of digital objects per SIP
*/
static SipSegmentationStrategy byMaxDigitalObjects(long maxDigitalObjects) {
return (domainObject, metrics) -> metrics.numDigitalObjects() % maxDigitalObjects == 0;
}
/**
* Return a {@linkplain SipSegmentationStrategy} that allows a maximum size (uncompressed) of the PDI per SIP.
* @param The type of domain objects to segment into different SIPs
* @param maxSize The maximum size of the PDI
* @return A {@linkplain SipSegmentationStrategy} that allows a maximum size of the PDI per SIP
*/
static SipSegmentationStrategy byMaxPdiSize(long maxSize) {
return (domainObject, metrics) -> metrics.pdiSize() >= maxSize;
}
/**
* Return a {@linkplain SipSegmentationStrategy} that allows a maximum total size (uncompressed) of digital objects
* per SIP.
* @param The type of domain objects to segment into different SIPs
* @param maxSize The maximum size of the digital objects
* @return A {@linkplain SipSegmentationStrategy} that allows a maximum total size of digital objects per SIP
*/
static SipSegmentationStrategy byMaxDigitalObjectsSize(long maxSize) {
return (domainObject, metrics) -> metrics.digitalObjectsSize() >= maxSize;
}
/**
* Return a {@linkplain SipSegmentationStrategy} that allows a maximum total size (uncompressed) of the SIP. Does not
* take into account the size of the object currently being added to the SIP
* @param The type of domain objects to segment into different SIPs
* @param maxSize The maximum size of the SIP
* @return A {@linkplain SipSegmentationStrategy} that allows a maximum total size of the SIP
*/
static SipSegmentationStrategy byMaxSipSize(long maxSize) {
return (domainObject, metrics) -> metrics.sipSize() >= maxSize;
}
/**
* Return a {@linkplain SipSegmentationStrategy} that allows a maximum total size (uncompressed) of the SIP including
* the object being added, but NOT including the PDI.
* @param The type of domain objects to segment into different SIPs
* @param maxSize The maximum size of the SIP
* @param digitalObjectsExtraction The extractor for the type of digital object being added.
* @return A {@linkplain SipSegmentationStrategy} that allows a maximum total size of the SIP including the object
* being added to the SIP
*/
static SipSegmentationStrategy byMaxProspectiveSipSize(long maxSize,
DigitalObjectsExtraction digitalObjectsExtraction) {
return (domainObject, metrics) -> maxSize < metrics.sipSize()
+ getDomainObjectSize(digitalObjectsExtraction.apply(domainObject), maxSize);
}
/**
* Return the size of a domain object in bytes.
* @param maxSize this is the maximum allowed size for the SIP
* @param iterator used to iterate over the digital objects contained in the domain object
* @return the size of the domain object in bytes
*/
static long getDomainObjectSize(Iterator extends DigitalObject> iterator, long maxSize) {
long result = 0;
while (iterator.hasNext()) {
result += iterator.next().getSize();
}
if (result > maxSize) {
throw new DomainObjectTooBigException(result, maxSize);
}
return result;
}
/**
* Return a {@linkplain SipSegmentationStrategy} that combines a number of partial strategies.
* @param The type of domain objects to segment into different SIPs
* @param partialStrategies The partial strategies to combine
* @return A {@linkplain SipSegmentationStrategy} that combines a number of partial strategies
*/
@SafeVarargs
@SuppressWarnings("varargs")
static SipSegmentationStrategy combining(SipSegmentationStrategy... partialStrategies) {
return (domainObject, metrics) -> Arrays.stream(partialStrategies)
.anyMatch(s -> s.shouldStartNewSip(domainObject, metrics));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy