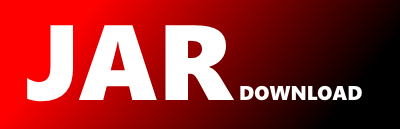
com.opentext.ia.sip.assembly.velocity.VelocityTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infoarchive-sdk-velocity Show documentation
Show all versions of infoarchive-sdk-velocity Show documentation
A library for building SIP PDI using the Velocity template engine
/*
* Copyright (c) 2016-2017 by OpenText Corporation. All Rights Reserved.
*/
package com.opentext.ia.sip.assembly.velocity;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.Map;
import org.apache.velocity.Template;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.Velocity;
import org.apache.velocity.app.VelocityEngine;
import org.apache.velocity.runtime.resource.loader.StringResourceLoader;
import org.apache.velocity.runtime.resource.util.StringResourceRepository;
import com.opentext.ia.sdk.sip.ContentInfo;
import com.opentext.ia.sdk.sip.DigitalObject;
import com.opentext.ia.sdk.sip.FixedHeaderAndFooterTemplate;
/**
* {@linkplain Template} using the Apache Velocity template engine.
* Templates have access to the following objects:
* model
* - The domain object (of type D)
* hashes
* - The encoded hashes of the {@linkplain DigitalObject}s associated with the domain object, if any
* isodate
* - A utility class to format a date in ISO 8601 format using the
format()
function
*
* @param The type of domain object to replace with text
*/
public class VelocityTemplate extends FixedHeaderAndFooterTemplate {
private static final String TEMPLATE_NAME = VelocityTemplate.class.getName();
private final Template template;
/**
* Create an instance.
* @param header The fixed header
* @param footer The fixed footer
* @param row The template for the rows
*/
public VelocityTemplate(InputStream header, InputStream footer, InputStream row) {
this(toString(header), toString(footer), toString(row));
}
/**
* Create an instance.
* @param header The fixed header
* @param footer The fixed footer
* @param row The template for the rows
*/
public VelocityTemplate(String header, String footer, String row) {
super(header, footer);
VelocityEngine engine = new VelocityEngine();
engine.setProperty(Velocity.RESOURCE_LOADER, "string");
engine.addProperty("string.resource.loader.class", StringResourceLoader.class.getName());
engine.addProperty("string.resource.loader.repository.static", "false");
engine.init();
StringResourceRepository repository = (StringResourceRepository)engine.getApplicationAttribute(
StringResourceLoader.REPOSITORY_NAME_DEFAULT);
repository.putStringResource(TEMPLATE_NAME, row);
template = engine.getTemplate(TEMPLATE_NAME);
}
@Override
public void writeRow(D domainObject, Map contentInfo, PrintWriter writer)
throws IOException {
VelocityContext context = new VelocityContext();
context.put("isodate", new DatesTool());
context.put("model", domainObject);
context.put("content", contentInfo);
template.merge(context, writer);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy