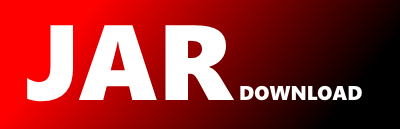
de.opitzconsulting.orcasDsl.Column Maven / Gradle / Ivy
Show all versions of orcas-domainextension-syex Show documentation
/**
*/
package de.opitzconsulting.orcasDsl;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Column'.
*
*
*
* The following features are supported:
*
*
* - {@link de.opitzconsulting.orcasDsl.Column#getName Name}
* - {@link de.opitzconsulting.orcasDsl.Column#getDomain Domain}
* - {@link de.opitzconsulting.orcasDsl.Column#getName_string Name string}
* - {@link de.opitzconsulting.orcasDsl.Column#getData_type Data type}
* - {@link de.opitzconsulting.orcasDsl.Column#getPrecision Precision}
* - {@link de.opitzconsulting.orcasDsl.Column#getScale Scale}
* - {@link de.opitzconsulting.orcasDsl.Column#getByteorchar Byteorchar}
* - {@link de.opitzconsulting.orcasDsl.Column#isUnsigned Unsigned}
* - {@link de.opitzconsulting.orcasDsl.Column#getWith_time_zone With time zone}
* - {@link de.opitzconsulting.orcasDsl.Column#getObject_type Object type}
* - {@link de.opitzconsulting.orcasDsl.Column#getDefault_value Default value}
* - {@link de.opitzconsulting.orcasDsl.Column#getVirtual Virtual}
* - {@link de.opitzconsulting.orcasDsl.Column#getDefault_name Default name}
* - {@link de.opitzconsulting.orcasDsl.Column#getIdentity Identity}
* - {@link de.opitzconsulting.orcasDsl.Column#getNot_null_constraint_name Not null constraint name}
* - {@link de.opitzconsulting.orcasDsl.Column#isNotnull Notnull}
*
*
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn()
* @model
* @generated
*/
public interface Column extends EObject
{
/**
* Returns the value of the 'Name' attribute.
*
*
* If the meaning of the 'Name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Name' attribute.
* @see #setName(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Name()
* @model
* @generated
*/
String getName();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getName Name}' attribute.
*
*
* @param value the new value of the 'Name' attribute.
* @see #getName()
* @generated
*/
void setName(String value);
/**
* Returns the value of the 'Domain' attribute.
*
*
* If the meaning of the 'Domain' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Domain' attribute.
* @see #setDomain(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Domain()
* @model
* @generated
*/
String getDomain();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getDomain Domain}' attribute.
*
*
* @param value the new value of the 'Domain' attribute.
* @see #getDomain()
* @generated
*/
void setDomain(String value);
/**
* Returns the value of the 'Name string' attribute.
*
*
* If the meaning of the 'Name string' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Name string' attribute.
* @see #setName_string(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Name_string()
* @model
* @generated
*/
String getName_string();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getName_string Name string}' attribute.
*
*
* @param value the new value of the 'Name string' attribute.
* @see #getName_string()
* @generated
*/
void setName_string(String value);
/**
* Returns the value of the 'Data type' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.DataType}.
*
*
* If the meaning of the 'Data type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Data type' attribute.
* @see de.opitzconsulting.orcasDsl.DataType
* @see #setData_type(DataType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Data_type()
* @model
* @generated
*/
DataType getData_type();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getData_type Data type}' attribute.
*
*
* @param value the new value of the 'Data type' attribute.
* @see de.opitzconsulting.orcasDsl.DataType
* @see #getData_type()
* @generated
*/
void setData_type(DataType value);
/**
* Returns the value of the 'Precision' attribute.
*
*
* If the meaning of the 'Precision' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Precision' attribute.
* @see #setPrecision(int)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Precision()
* @model
* @generated
*/
int getPrecision();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getPrecision Precision}' attribute.
*
*
* @param value the new value of the 'Precision' attribute.
* @see #getPrecision()
* @generated
*/
void setPrecision(int value);
/**
* Returns the value of the 'Scale' attribute.
*
*
* If the meaning of the 'Scale' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Scale' attribute.
* @see #setScale(int)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Scale()
* @model
* @generated
*/
int getScale();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getScale Scale}' attribute.
*
*
* @param value the new value of the 'Scale' attribute.
* @see #getScale()
* @generated
*/
void setScale(int value);
/**
* Returns the value of the 'Byteorchar' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.CharType}.
*
*
* If the meaning of the 'Byteorchar' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Byteorchar' attribute.
* @see de.opitzconsulting.orcasDsl.CharType
* @see #setByteorchar(CharType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Byteorchar()
* @model
* @generated
*/
CharType getByteorchar();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getByteorchar Byteorchar}' attribute.
*
*
* @param value the new value of the 'Byteorchar' attribute.
* @see de.opitzconsulting.orcasDsl.CharType
* @see #getByteorchar()
* @generated
*/
void setByteorchar(CharType value);
/**
* Returns the value of the 'Unsigned' attribute.
*
*
* If the meaning of the 'Unsigned' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Unsigned' attribute.
* @see #setUnsigned(boolean)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Unsigned()
* @model
* @generated
*/
boolean isUnsigned();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#isUnsigned Unsigned}' attribute.
*
*
* @param value the new value of the 'Unsigned' attribute.
* @see #isUnsigned()
* @generated
*/
void setUnsigned(boolean value);
/**
* Returns the value of the 'With time zone' attribute.
*
*
* If the meaning of the 'With time zone' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'With time zone' attribute.
* @see #setWith_time_zone(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_With_time_zone()
* @model
* @generated
*/
String getWith_time_zone();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getWith_time_zone With time zone}' attribute.
*
*
* @param value the new value of the 'With time zone' attribute.
* @see #getWith_time_zone()
* @generated
*/
void setWith_time_zone(String value);
/**
* Returns the value of the 'Object type' attribute.
*
*
* If the meaning of the 'Object type' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Object type' attribute.
* @see #setObject_type(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Object_type()
* @model
* @generated
*/
String getObject_type();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getObject_type Object type}' attribute.
*
*
* @param value the new value of the 'Object type' attribute.
* @see #getObject_type()
* @generated
*/
void setObject_type(String value);
/**
* Returns the value of the 'Default value' attribute.
*
*
* If the meaning of the 'Default value' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Default value' attribute.
* @see #setDefault_value(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Default_value()
* @model
* @generated
*/
String getDefault_value();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getDefault_value Default value}' attribute.
*
*
* @param value the new value of the 'Default value' attribute.
* @see #getDefault_value()
* @generated
*/
void setDefault_value(String value);
/**
* Returns the value of the 'Virtual' attribute.
*
*
* If the meaning of the 'Virtual' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Virtual' attribute.
* @see #setVirtual(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Virtual()
* @model
* @generated
*/
String getVirtual();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getVirtual Virtual}' attribute.
*
*
* @param value the new value of the 'Virtual' attribute.
* @see #getVirtual()
* @generated
*/
void setVirtual(String value);
/**
* Returns the value of the 'Default name' attribute.
*
*
* If the meaning of the 'Default name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Default name' attribute.
* @see #setDefault_name(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Default_name()
* @model
* @generated
*/
String getDefault_name();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getDefault_name Default name}' attribute.
*
*
* @param value the new value of the 'Default name' attribute.
* @see #getDefault_name()
* @generated
*/
void setDefault_name(String value);
/**
* Returns the value of the 'Identity' containment reference.
*
*
* If the meaning of the 'Identity' containment reference isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Identity' containment reference.
* @see #setIdentity(ColumnIdentity)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Identity()
* @model containment="true"
* @generated
*/
ColumnIdentity getIdentity();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getIdentity Identity}' containment reference.
*
*
* @param value the new value of the 'Identity' containment reference.
* @see #getIdentity()
* @generated
*/
void setIdentity(ColumnIdentity value);
/**
* Returns the value of the 'Not null constraint name' attribute.
*
*
* If the meaning of the 'Not null constraint name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Not null constraint name' attribute.
* @see #setNot_null_constraint_name(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Not_null_constraint_name()
* @model
* @generated
*/
String getNot_null_constraint_name();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#getNot_null_constraint_name Not null constraint name}' attribute.
*
*
* @param value the new value of the 'Not null constraint name' attribute.
* @see #getNot_null_constraint_name()
* @generated
*/
void setNot_null_constraint_name(String value);
/**
* Returns the value of the 'Notnull' attribute.
*
*
* If the meaning of the 'Notnull' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Notnull' attribute.
* @see #setNotnull(boolean)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getColumn_Notnull()
* @model
* @generated
*/
boolean isNotnull();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Column#isNotnull Notnull}' attribute.
*
*
* @param value the new value of the 'Notnull' attribute.
* @see #isNotnull()
* @generated
*/
void setNotnull(boolean value);
} // Column