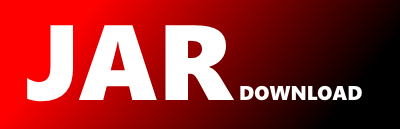
de.opitzconsulting.orcasDsl.ForeignKey Maven / Gradle / Ivy
Show all versions of orcas-domainextension-syex Show documentation
/**
*/
package de.opitzconsulting.orcasDsl;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Foreign Key'.
*
*
*
* The following features are supported:
*
*
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getConsName Cons Name}
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getSrcColumns Src Columns}
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getDestTable Dest Table}
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getDestColumns Dest Columns}
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getDelete_rule Delete rule}
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getDeferrtype Deferrtype}
* - {@link de.opitzconsulting.orcasDsl.ForeignKey#getStatus Status}
*
*
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey()
* @model
* @generated
*/
public interface ForeignKey extends EObject
{
/**
* Returns the value of the 'Cons Name' attribute.
*
*
* If the meaning of the 'Cons Name' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Cons Name' attribute.
* @see #setConsName(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_ConsName()
* @model
* @generated
*/
String getConsName();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.ForeignKey#getConsName Cons Name}' attribute.
*
*
* @param value the new value of the 'Cons Name' attribute.
* @see #getConsName()
* @generated
*/
void setConsName(String value);
/**
* Returns the value of the 'Src Columns' containment reference list.
* The list contents are of type {@link de.opitzconsulting.orcasDsl.ColumnRef}.
*
*
* If the meaning of the 'Src Columns' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Src Columns' containment reference list.
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_SrcColumns()
* @model containment="true"
* @generated
*/
EList getSrcColumns();
/**
* Returns the value of the 'Dest Table' attribute.
*
*
* If the meaning of the 'Dest Table' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Dest Table' attribute.
* @see #setDestTable(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_DestTable()
* @model
* @generated
*/
String getDestTable();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.ForeignKey#getDestTable Dest Table}' attribute.
*
*
* @param value the new value of the 'Dest Table' attribute.
* @see #getDestTable()
* @generated
*/
void setDestTable(String value);
/**
* Returns the value of the 'Dest Columns' containment reference list.
* The list contents are of type {@link de.opitzconsulting.orcasDsl.ColumnRef}.
*
*
* If the meaning of the 'Dest Columns' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Dest Columns' containment reference list.
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_DestColumns()
* @model containment="true"
* @generated
*/
EList getDestColumns();
/**
* Returns the value of the 'Delete rule' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.FkDeleteRuleType}.
*
*
* If the meaning of the 'Delete rule' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Delete rule' attribute.
* @see de.opitzconsulting.orcasDsl.FkDeleteRuleType
* @see #setDelete_rule(FkDeleteRuleType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_Delete_rule()
* @model
* @generated
*/
FkDeleteRuleType getDelete_rule();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.ForeignKey#getDelete_rule Delete rule}' attribute.
*
*
* @param value the new value of the 'Delete rule' attribute.
* @see de.opitzconsulting.orcasDsl.FkDeleteRuleType
* @see #getDelete_rule()
* @generated
*/
void setDelete_rule(FkDeleteRuleType value);
/**
* Returns the value of the 'Deferrtype' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.DeferrType}.
*
*
* If the meaning of the 'Deferrtype' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Deferrtype' attribute.
* @see de.opitzconsulting.orcasDsl.DeferrType
* @see #setDeferrtype(DeferrType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_Deferrtype()
* @model
* @generated
*/
DeferrType getDeferrtype();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.ForeignKey#getDeferrtype Deferrtype}' attribute.
*
*
* @param value the new value of the 'Deferrtype' attribute.
* @see de.opitzconsulting.orcasDsl.DeferrType
* @see #getDeferrtype()
* @generated
*/
void setDeferrtype(DeferrType value);
/**
* Returns the value of the 'Status' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.EnableType}.
*
*
* If the meaning of the 'Status' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Status' attribute.
* @see de.opitzconsulting.orcasDsl.EnableType
* @see #setStatus(EnableType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getForeignKey_Status()
* @model
* @generated
*/
EnableType getStatus();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.ForeignKey#getStatus Status}' attribute.
*
*
* @param value the new value of the 'Status' attribute.
* @see de.opitzconsulting.orcasDsl.EnableType
* @see #getStatus()
* @generated
*/
void setStatus(EnableType value);
} // ForeignKey