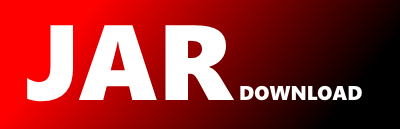
de.opitzconsulting.orcasDsl.Index Maven / Gradle / Ivy
/**
*/
package de.opitzconsulting.orcasDsl;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Index'.
*
*
*
* The following features are supported:
*
*
* - {@link de.opitzconsulting.orcasDsl.Index#getIndex_columns Index columns}
* - {@link de.opitzconsulting.orcasDsl.Index#getDomain_index_expression Domain index expression}
* - {@link de.opitzconsulting.orcasDsl.Index#getFunction_based_expression Function based expression}
* - {@link de.opitzconsulting.orcasDsl.Index#getBitmap Bitmap}
* - {@link de.opitzconsulting.orcasDsl.Index#getUnique Unique}
* - {@link de.opitzconsulting.orcasDsl.Index#getLogging Logging}
* - {@link de.opitzconsulting.orcasDsl.Index#getCompression Compression}
* - {@link de.opitzconsulting.orcasDsl.Index#getParallel Parallel}
* - {@link de.opitzconsulting.orcasDsl.Index#getParallel_degree Parallel degree}
* - {@link de.opitzconsulting.orcasDsl.Index#getGlobal Global}
*
*
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex()
* @model
* @generated
*/
public interface Index extends IndexOrUniqueKey
{
/**
* Returns the value of the 'Index columns' containment reference list.
* The list contents are of type {@link de.opitzconsulting.orcasDsl.ColumnRef}.
*
*
* If the meaning of the 'Index columns' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Index columns' containment reference list.
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Index_columns()
* @model containment="true"
* @generated
*/
EList getIndex_columns();
/**
* Returns the value of the 'Domain index expression' attribute.
*
*
* If the meaning of the 'Domain index expression' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Domain index expression' attribute.
* @see #setDomain_index_expression(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Domain_index_expression()
* @model
* @generated
*/
String getDomain_index_expression();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getDomain_index_expression Domain index expression}' attribute.
*
*
* @param value the new value of the 'Domain index expression' attribute.
* @see #getDomain_index_expression()
* @generated
*/
void setDomain_index_expression(String value);
/**
* Returns the value of the 'Function based expression' attribute.
*
*
* If the meaning of the 'Function based expression' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Function based expression' attribute.
* @see #setFunction_based_expression(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Function_based_expression()
* @model
* @generated
*/
String getFunction_based_expression();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getFunction_based_expression Function based expression}' attribute.
*
*
* @param value the new value of the 'Function based expression' attribute.
* @see #getFunction_based_expression()
* @generated
*/
void setFunction_based_expression(String value);
/**
* Returns the value of the 'Bitmap' attribute.
*
*
* If the meaning of the 'Bitmap' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Bitmap' attribute.
* @see #setBitmap(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Bitmap()
* @model
* @generated
*/
String getBitmap();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getBitmap Bitmap}' attribute.
*
*
* @param value the new value of the 'Bitmap' attribute.
* @see #getBitmap()
* @generated
*/
void setBitmap(String value);
/**
* Returns the value of the 'Unique' attribute.
*
*
* If the meaning of the 'Unique' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Unique' attribute.
* @see #setUnique(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Unique()
* @model
* @generated
*/
String getUnique();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getUnique Unique}' attribute.
*
*
* @param value the new value of the 'Unique' attribute.
* @see #getUnique()
* @generated
*/
void setUnique(String value);
/**
* Returns the value of the 'Logging' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.LoggingType}.
*
*
* If the meaning of the 'Logging' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Logging' attribute.
* @see de.opitzconsulting.orcasDsl.LoggingType
* @see #setLogging(LoggingType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Logging()
* @model
* @generated
*/
LoggingType getLogging();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getLogging Logging}' attribute.
*
*
* @param value the new value of the 'Logging' attribute.
* @see de.opitzconsulting.orcasDsl.LoggingType
* @see #getLogging()
* @generated
*/
void setLogging(LoggingType value);
/**
* Returns the value of the 'Compression' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.CompressType}.
*
*
* If the meaning of the 'Compression' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Compression' attribute.
* @see de.opitzconsulting.orcasDsl.CompressType
* @see #setCompression(CompressType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Compression()
* @model
* @generated
*/
CompressType getCompression();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getCompression Compression}' attribute.
*
*
* @param value the new value of the 'Compression' attribute.
* @see de.opitzconsulting.orcasDsl.CompressType
* @see #getCompression()
* @generated
*/
void setCompression(CompressType value);
/**
* Returns the value of the 'Parallel' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.ParallelType}.
*
*
* If the meaning of the 'Parallel' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Parallel' attribute.
* @see de.opitzconsulting.orcasDsl.ParallelType
* @see #setParallel(ParallelType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Parallel()
* @model
* @generated
*/
ParallelType getParallel();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getParallel Parallel}' attribute.
*
*
* @param value the new value of the 'Parallel' attribute.
* @see de.opitzconsulting.orcasDsl.ParallelType
* @see #getParallel()
* @generated
*/
void setParallel(ParallelType value);
/**
* Returns the value of the 'Parallel degree' attribute.
*
*
* If the meaning of the 'Parallel degree' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Parallel degree' attribute.
* @see #setParallel_degree(int)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Parallel_degree()
* @model
* @generated
*/
int getParallel_degree();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getParallel_degree Parallel degree}' attribute.
*
*
* @param value the new value of the 'Parallel degree' attribute.
* @see #getParallel_degree()
* @generated
*/
void setParallel_degree(int value);
/**
* Returns the value of the 'Global' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.IndexGlobalType}.
*
*
* If the meaning of the 'Global' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Global' attribute.
* @see de.opitzconsulting.orcasDsl.IndexGlobalType
* @see #setGlobal(IndexGlobalType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getIndex_Global()
* @model
* @generated
*/
IndexGlobalType getGlobal();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.Index#getGlobal Global}' attribute.
*
*
* @param value the new value of the 'Global' attribute.
* @see de.opitzconsulting.orcasDsl.IndexGlobalType
* @see #getGlobal()
* @generated
*/
void setGlobal(IndexGlobalType value);
} // Index