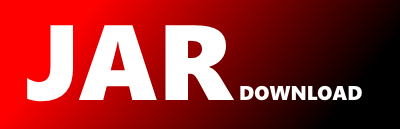
de.opitzconsulting.orcasDsl.MviewLog Maven / Gradle / Ivy
Show all versions of orcas-domainextension-syex Show documentation
/**
*/
package de.opitzconsulting.orcasDsl;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Mview Log'.
*
*
*
* The following features are supported:
*
*
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getTablespace Tablespace}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getParallel Parallel}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getParallel_degree Parallel degree}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getNewValues New Values}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getPrimaryKey Primary Key}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getRowid Rowid}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getWithSequence With Sequence}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getColumns Columns}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getCommitScn Commit Scn}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getPurge Purge}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getSynchronous Synchronous}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getStartWith Start With}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getNext Next}
* - {@link de.opitzconsulting.orcasDsl.MviewLog#getRepeatInterval Repeat Interval}
*
*
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog()
* @model
* @generated
*/
public interface MviewLog extends EObject
{
/**
* Returns the value of the 'Tablespace' attribute.
*
*
* If the meaning of the 'Tablespace' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Tablespace' attribute.
* @see #setTablespace(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Tablespace()
* @model
* @generated
*/
String getTablespace();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getTablespace Tablespace}' attribute.
*
*
* @param value the new value of the 'Tablespace' attribute.
* @see #getTablespace()
* @generated
*/
void setTablespace(String value);
/**
* Returns the value of the 'Parallel' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.ParallelType}.
*
*
* If the meaning of the 'Parallel' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Parallel' attribute.
* @see de.opitzconsulting.orcasDsl.ParallelType
* @see #setParallel(ParallelType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Parallel()
* @model
* @generated
*/
ParallelType getParallel();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getParallel Parallel}' attribute.
*
*
* @param value the new value of the 'Parallel' attribute.
* @see de.opitzconsulting.orcasDsl.ParallelType
* @see #getParallel()
* @generated
*/
void setParallel(ParallelType value);
/**
* Returns the value of the 'Parallel degree' attribute.
*
*
* If the meaning of the 'Parallel degree' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Parallel degree' attribute.
* @see #setParallel_degree(int)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Parallel_degree()
* @model
* @generated
*/
int getParallel_degree();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getParallel_degree Parallel degree}' attribute.
*
*
* @param value the new value of the 'Parallel degree' attribute.
* @see #getParallel_degree()
* @generated
*/
void setParallel_degree(int value);
/**
* Returns the value of the 'New Values' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.NewValuesType}.
*
*
* If the meaning of the 'New Values' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'New Values' attribute.
* @see de.opitzconsulting.orcasDsl.NewValuesType
* @see #setNewValues(NewValuesType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_NewValues()
* @model
* @generated
*/
NewValuesType getNewValues();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getNewValues New Values}' attribute.
*
*
* @param value the new value of the 'New Values' attribute.
* @see de.opitzconsulting.orcasDsl.NewValuesType
* @see #getNewValues()
* @generated
*/
void setNewValues(NewValuesType value);
/**
* Returns the value of the 'Primary Key' attribute.
*
*
* If the meaning of the 'Primary Key' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Primary Key' attribute.
* @see #setPrimaryKey(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_PrimaryKey()
* @model
* @generated
*/
String getPrimaryKey();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getPrimaryKey Primary Key}' attribute.
*
*
* @param value the new value of the 'Primary Key' attribute.
* @see #getPrimaryKey()
* @generated
*/
void setPrimaryKey(String value);
/**
* Returns the value of the 'Rowid' attribute.
*
*
* If the meaning of the 'Rowid' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Rowid' attribute.
* @see #setRowid(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Rowid()
* @model
* @generated
*/
String getRowid();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getRowid Rowid}' attribute.
*
*
* @param value the new value of the 'Rowid' attribute.
* @see #getRowid()
* @generated
*/
void setRowid(String value);
/**
* Returns the value of the 'With Sequence' attribute.
*
*
* If the meaning of the 'With Sequence' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'With Sequence' attribute.
* @see #setWithSequence(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_WithSequence()
* @model
* @generated
*/
String getWithSequence();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getWithSequence With Sequence}' attribute.
*
*
* @param value the new value of the 'With Sequence' attribute.
* @see #getWithSequence()
* @generated
*/
void setWithSequence(String value);
/**
* Returns the value of the 'Columns' containment reference list.
* The list contents are of type {@link de.opitzconsulting.orcasDsl.ColumnRef}.
*
*
* If the meaning of the 'Columns' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Columns' containment reference list.
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Columns()
* @model containment="true"
* @generated
*/
EList getColumns();
/**
* Returns the value of the 'Commit Scn' attribute.
*
*
* If the meaning of the 'Commit Scn' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Commit Scn' attribute.
* @see #setCommitScn(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_CommitScn()
* @model
* @generated
*/
String getCommitScn();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getCommitScn Commit Scn}' attribute.
*
*
* @param value the new value of the 'Commit Scn' attribute.
* @see #getCommitScn()
* @generated
*/
void setCommitScn(String value);
/**
* Returns the value of the 'Purge' attribute.
*
*
* If the meaning of the 'Purge' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Purge' attribute.
* @see #setPurge(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Purge()
* @model
* @generated
*/
String getPurge();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getPurge Purge}' attribute.
*
*
* @param value the new value of the 'Purge' attribute.
* @see #getPurge()
* @generated
*/
void setPurge(String value);
/**
* Returns the value of the 'Synchronous' attribute.
* The literals are from the enumeration {@link de.opitzconsulting.orcasDsl.SynchronousType}.
*
*
* If the meaning of the 'Synchronous' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Synchronous' attribute.
* @see de.opitzconsulting.orcasDsl.SynchronousType
* @see #setSynchronous(SynchronousType)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Synchronous()
* @model
* @generated
*/
SynchronousType getSynchronous();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getSynchronous Synchronous}' attribute.
*
*
* @param value the new value of the 'Synchronous' attribute.
* @see de.opitzconsulting.orcasDsl.SynchronousType
* @see #getSynchronous()
* @generated
*/
void setSynchronous(SynchronousType value);
/**
* Returns the value of the 'Start With' attribute.
*
*
* If the meaning of the 'Start With' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Start With' attribute.
* @see #setStartWith(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_StartWith()
* @model
* @generated
*/
String getStartWith();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getStartWith Start With}' attribute.
*
*
* @param value the new value of the 'Start With' attribute.
* @see #getStartWith()
* @generated
*/
void setStartWith(String value);
/**
* Returns the value of the 'Next' attribute.
*
*
* If the meaning of the 'Next' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Next' attribute.
* @see #setNext(String)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_Next()
* @model
* @generated
*/
String getNext();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getNext Next}' attribute.
*
*
* @param value the new value of the 'Next' attribute.
* @see #getNext()
* @generated
*/
void setNext(String value);
/**
* Returns the value of the 'Repeat Interval' attribute.
*
*
* If the meaning of the 'Repeat Interval' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Repeat Interval' attribute.
* @see #setRepeatInterval(int)
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage#getMviewLog_RepeatInterval()
* @model
* @generated
*/
int getRepeatInterval();
/**
* Sets the value of the '{@link de.opitzconsulting.orcasDsl.MviewLog#getRepeatInterval Repeat Interval}' attribute.
*
*
* @param value the new value of the 'Repeat Interval' attribute.
* @see #getRepeatInterval()
* @generated
*/
void setRepeatInterval(int value);
} // MviewLog