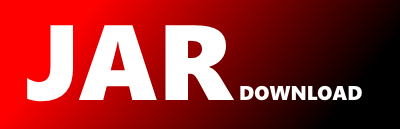
de.opitzconsulting.orcasDsl.impl.ColumnImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orcas-domainextension-syex Show documentation
Show all versions of orcas-domainextension-syex Show documentation
orcas model modified by syntax extensions (contains the original model). If custom-syntax-extensions are provided, there will be a custom artifact.
The newest version!
/**
*/
package de.opitzconsulting.orcasDsl.impl;
import de.opitzconsulting.orcasDsl.CharType;
import de.opitzconsulting.orcasDsl.Column;
import de.opitzconsulting.orcasDsl.ColumnIdentity;
import de.opitzconsulting.orcasDsl.DataType;
import de.opitzconsulting.orcasDsl.OrcasDslPackage;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
/**
*
* An implementation of the model object 'Column'.
*
*
* The following features are implemented:
*
*
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getName Name}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getDomain Domain}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getName_string Name string}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getData_type Data type}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getPrecision Precision}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getScale Scale}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getByteorchar Byteorchar}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#isUnsigned Unsigned}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getWith_time_zone With time zone}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getObject_type Object type}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getDefault_value Default value}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getVirtual Virtual}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getDefault_name Default name}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getIdentity Identity}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#getNot_null_constraint_name Not null constraint name}
* - {@link de.opitzconsulting.orcasDsl.impl.ColumnImpl#isNotnull Notnull}
*
*
* @generated
*/
public class ColumnImpl extends MinimalEObjectImpl.Container implements Column
{
/**
* The default value of the '{@link #getName() Name}' attribute.
*
*
* @see #getName()
* @generated
* @ordered
*/
public static final String NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getName() Name}' attribute.
*
*
* @see #getName()
* @generated
* @ordered
*/
public String name = NAME_EDEFAULT;
/**
* The default value of the '{@link #getDomain() Domain}' attribute.
*
*
* @see #getDomain()
* @generated
* @ordered
*/
public static final String DOMAIN_EDEFAULT = null;
/**
* The cached value of the '{@link #getDomain() Domain}' attribute.
*
*
* @see #getDomain()
* @generated
* @ordered
*/
public String domain = DOMAIN_EDEFAULT;
/**
* The default value of the '{@link #getName_string() Name string}' attribute.
*
*
* @see #getName_string()
* @generated
* @ordered
*/
public static final String NAME_STRING_EDEFAULT = null;
/**
* The cached value of the '{@link #getName_string() Name string}' attribute.
*
*
* @see #getName_string()
* @generated
* @ordered
*/
public String name_string = NAME_STRING_EDEFAULT;
/**
* The default value of the '{@link #getData_type() Data type}' attribute.
*
*
* @see #getData_type()
* @generated
* @ordered
*/
public static final DataType DATA_TYPE_EDEFAULT = null;
/**
* The cached value of the '{@link #getData_type() Data type}' attribute.
*
*
* @see #getData_type()
* @generated
* @ordered
*/
public DataType data_type = DATA_TYPE_EDEFAULT;
/**
* The default value of the '{@link #getPrecision() Precision}' attribute.
*
*
* @see #getPrecision()
* @generated
* @ordered
*/
public static final int PRECISION_EDEFAULT = 0;
/**
* The cached value of the '{@link #getPrecision() Precision}' attribute.
*
*
* @see #getPrecision()
* @generated
* @ordered
*/
public int precision = PRECISION_EDEFAULT;
/**
* The default value of the '{@link #getScale() Scale}' attribute.
*
*
* @see #getScale()
* @generated
* @ordered
*/
public static final int SCALE_EDEFAULT = 0;
/**
* The cached value of the '{@link #getScale() Scale}' attribute.
*
*
* @see #getScale()
* @generated
* @ordered
*/
public int scale = SCALE_EDEFAULT;
/**
* The default value of the '{@link #getByteorchar() Byteorchar}' attribute.
*
*
* @see #getByteorchar()
* @generated
* @ordered
*/
public static final CharType BYTEORCHAR_EDEFAULT = null;
/**
* The cached value of the '{@link #getByteorchar() Byteorchar}' attribute.
*
*
* @see #getByteorchar()
* @generated
* @ordered
*/
public CharType byteorchar = BYTEORCHAR_EDEFAULT;
/**
* The default value of the '{@link #isUnsigned() Unsigned}' attribute.
*
*
* @see #isUnsigned()
* @generated
* @ordered
*/
public static final boolean UNSIGNED_EDEFAULT = false;
/**
* The cached value of the '{@link #isUnsigned() Unsigned}' attribute.
*
*
* @see #isUnsigned()
* @generated
* @ordered
*/
public boolean unsigned = UNSIGNED_EDEFAULT;
/**
* The default value of the '{@link #getWith_time_zone() With time zone}' attribute.
*
*
* @see #getWith_time_zone()
* @generated
* @ordered
*/
public static final String WITH_TIME_ZONE_EDEFAULT = null;
/**
* The cached value of the '{@link #getWith_time_zone() With time zone}' attribute.
*
*
* @see #getWith_time_zone()
* @generated
* @ordered
*/
public String with_time_zone = WITH_TIME_ZONE_EDEFAULT;
/**
* The default value of the '{@link #getObject_type() Object type}' attribute.
*
*
* @see #getObject_type()
* @generated
* @ordered
*/
public static final String OBJECT_TYPE_EDEFAULT = null;
/**
* The cached value of the '{@link #getObject_type() Object type}' attribute.
*
*
* @see #getObject_type()
* @generated
* @ordered
*/
public String object_type = OBJECT_TYPE_EDEFAULT;
/**
* The default value of the '{@link #getDefault_value() Default value}' attribute.
*
*
* @see #getDefault_value()
* @generated
* @ordered
*/
public static final String DEFAULT_VALUE_EDEFAULT = null;
/**
* The cached value of the '{@link #getDefault_value() Default value}' attribute.
*
*
* @see #getDefault_value()
* @generated
* @ordered
*/
public String default_value = DEFAULT_VALUE_EDEFAULT;
/**
* The default value of the '{@link #getVirtual() Virtual}' attribute.
*
*
* @see #getVirtual()
* @generated
* @ordered
*/
public static final String VIRTUAL_EDEFAULT = null;
/**
* The cached value of the '{@link #getVirtual() Virtual}' attribute.
*
*
* @see #getVirtual()
* @generated
* @ordered
*/
public String virtual = VIRTUAL_EDEFAULT;
/**
* The default value of the '{@link #getDefault_name() Default name}' attribute.
*
*
* @see #getDefault_name()
* @generated
* @ordered
*/
public static final String DEFAULT_NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getDefault_name() Default name}' attribute.
*
*
* @see #getDefault_name()
* @generated
* @ordered
*/
public String default_name = DEFAULT_NAME_EDEFAULT;
/**
* The cached value of the '{@link #getIdentity() Identity}' containment reference.
*
*
* @see #getIdentity()
* @generated
* @ordered
*/
public ColumnIdentity identity;
/**
* The default value of the '{@link #getNot_null_constraint_name() Not null constraint name}' attribute.
*
*
* @see #getNot_null_constraint_name()
* @generated
* @ordered
*/
public static final String NOT_NULL_CONSTRAINT_NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getNot_null_constraint_name() Not null constraint name}' attribute.
*
*
* @see #getNot_null_constraint_name()
* @generated
* @ordered
*/
public String not_null_constraint_name = NOT_NULL_CONSTRAINT_NAME_EDEFAULT;
/**
* The default value of the '{@link #isNotnull() Notnull}' attribute.
*
*
* @see #isNotnull()
* @generated
* @ordered
*/
public static final boolean NOTNULL_EDEFAULT = false;
/**
* The cached value of the '{@link #isNotnull() Notnull}' attribute.
*
*
* @see #isNotnull()
* @generated
* @ordered
*/
public boolean notnull = NOTNULL_EDEFAULT;
/**
*
*
* @generated
*/
public ColumnImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
public EClass eStaticClass()
{
return OrcasDslPackage.Literals.COLUMN;
}
/**
*
*
* @generated
*/
public String getName()
{
return name;
}
/**
*
*
* @generated
*/
public void setName(String newName)
{
String oldName = name;
name = newName;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__NAME, oldName, name));
}
/**
*
*
* @generated
*/
public String getDomain()
{
return domain;
}
/**
*
*
* @generated
*/
public void setDomain(String newDomain)
{
String oldDomain = domain;
domain = newDomain;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__DOMAIN, oldDomain, domain));
}
/**
*
*
* @generated
*/
public String getName_string()
{
return name_string;
}
/**
*
*
* @generated
*/
public void setName_string(String newName_string)
{
String oldName_string = name_string;
name_string = newName_string;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__NAME_STRING, oldName_string, name_string));
}
/**
*
*
* @generated
*/
public DataType getData_type()
{
return data_type;
}
/**
*
*
* @generated
*/
public void setData_type(DataType newData_type)
{
DataType oldData_type = data_type;
data_type = newData_type == null ? DATA_TYPE_EDEFAULT : newData_type;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__DATA_TYPE, oldData_type, data_type));
}
/**
*
*
* @generated
*/
public int getPrecision()
{
return precision;
}
/**
*
*
* @generated
*/
public void setPrecision(int newPrecision)
{
int oldPrecision = precision;
precision = newPrecision;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__PRECISION, oldPrecision, precision));
}
/**
*
*
* @generated
*/
public int getScale()
{
return scale;
}
/**
*
*
* @generated
*/
public void setScale(int newScale)
{
int oldScale = scale;
scale = newScale;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__SCALE, oldScale, scale));
}
/**
*
*
* @generated
*/
public CharType getByteorchar()
{
return byteorchar;
}
/**
*
*
* @generated
*/
public void setByteorchar(CharType newByteorchar)
{
CharType oldByteorchar = byteorchar;
byteorchar = newByteorchar == null ? BYTEORCHAR_EDEFAULT : newByteorchar;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__BYTEORCHAR, oldByteorchar, byteorchar));
}
/**
*
*
* @generated
*/
public boolean isUnsigned()
{
return unsigned;
}
/**
*
*
* @generated
*/
public void setUnsigned(boolean newUnsigned)
{
boolean oldUnsigned = unsigned;
unsigned = newUnsigned;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__UNSIGNED, oldUnsigned, unsigned));
}
/**
*
*
* @generated
*/
public String getWith_time_zone()
{
return with_time_zone;
}
/**
*
*
* @generated
*/
public void setWith_time_zone(String newWith_time_zone)
{
String oldWith_time_zone = with_time_zone;
with_time_zone = newWith_time_zone;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__WITH_TIME_ZONE, oldWith_time_zone, with_time_zone));
}
/**
*
*
* @generated
*/
public String getObject_type()
{
return object_type;
}
/**
*
*
* @generated
*/
public void setObject_type(String newObject_type)
{
String oldObject_type = object_type;
object_type = newObject_type;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__OBJECT_TYPE, oldObject_type, object_type));
}
/**
*
*
* @generated
*/
public String getDefault_value()
{
return default_value;
}
/**
*
*
* @generated
*/
public void setDefault_value(String newDefault_value)
{
String oldDefault_value = default_value;
default_value = newDefault_value;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__DEFAULT_VALUE, oldDefault_value, default_value));
}
/**
*
*
* @generated
*/
public String getVirtual()
{
return virtual;
}
/**
*
*
* @generated
*/
public void setVirtual(String newVirtual)
{
String oldVirtual = virtual;
virtual = newVirtual;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__VIRTUAL, oldVirtual, virtual));
}
/**
*
*
* @generated
*/
public String getDefault_name()
{
return default_name;
}
/**
*
*
* @generated
*/
public void setDefault_name(String newDefault_name)
{
String oldDefault_name = default_name;
default_name = newDefault_name;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__DEFAULT_NAME, oldDefault_name, default_name));
}
/**
*
*
* @generated
*/
public ColumnIdentity getIdentity()
{
return identity;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetIdentity(ColumnIdentity newIdentity, NotificationChain msgs)
{
ColumnIdentity oldIdentity = identity;
identity = newIdentity;
if (eNotificationRequired())
{
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__IDENTITY, oldIdentity, newIdentity);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
public void setIdentity(ColumnIdentity newIdentity)
{
if (newIdentity != identity)
{
NotificationChain msgs = null;
if (identity != null)
msgs = ((InternalEObject)identity).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - OrcasDslPackage.COLUMN__IDENTITY, null, msgs);
if (newIdentity != null)
msgs = ((InternalEObject)newIdentity).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - OrcasDslPackage.COLUMN__IDENTITY, null, msgs);
msgs = basicSetIdentity(newIdentity, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__IDENTITY, newIdentity, newIdentity));
}
/**
*
*
* @generated
*/
public String getNot_null_constraint_name()
{
return not_null_constraint_name;
}
/**
*
*
* @generated
*/
public void setNot_null_constraint_name(String newNot_null_constraint_name)
{
String oldNot_null_constraint_name = not_null_constraint_name;
not_null_constraint_name = newNot_null_constraint_name;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__NOT_NULL_CONSTRAINT_NAME, oldNot_null_constraint_name, not_null_constraint_name));
}
/**
*
*
* @generated
*/
public boolean isNotnull()
{
return notnull;
}
/**
*
*
* @generated
*/
public void setNotnull(boolean newNotnull)
{
boolean oldNotnull = notnull;
notnull = newNotnull;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.COLUMN__NOTNULL, oldNotnull, notnull));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs)
{
switch (featureID)
{
case OrcasDslPackage.COLUMN__IDENTITY:
return basicSetIdentity(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case OrcasDslPackage.COLUMN__NAME:
return getName();
case OrcasDslPackage.COLUMN__DOMAIN:
return getDomain();
case OrcasDslPackage.COLUMN__NAME_STRING:
return getName_string();
case OrcasDslPackage.COLUMN__DATA_TYPE:
return getData_type();
case OrcasDslPackage.COLUMN__PRECISION:
return getPrecision();
case OrcasDslPackage.COLUMN__SCALE:
return getScale();
case OrcasDslPackage.COLUMN__BYTEORCHAR:
return getByteorchar();
case OrcasDslPackage.COLUMN__UNSIGNED:
return isUnsigned();
case OrcasDslPackage.COLUMN__WITH_TIME_ZONE:
return getWith_time_zone();
case OrcasDslPackage.COLUMN__OBJECT_TYPE:
return getObject_type();
case OrcasDslPackage.COLUMN__DEFAULT_VALUE:
return getDefault_value();
case OrcasDslPackage.COLUMN__VIRTUAL:
return getVirtual();
case OrcasDslPackage.COLUMN__DEFAULT_NAME:
return getDefault_name();
case OrcasDslPackage.COLUMN__IDENTITY:
return getIdentity();
case OrcasDslPackage.COLUMN__NOT_NULL_CONSTRAINT_NAME:
return getNot_null_constraint_name();
case OrcasDslPackage.COLUMN__NOTNULL:
return isNotnull();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case OrcasDslPackage.COLUMN__NAME:
setName((String)newValue);
return;
case OrcasDslPackage.COLUMN__DOMAIN:
setDomain((String)newValue);
return;
case OrcasDslPackage.COLUMN__NAME_STRING:
setName_string((String)newValue);
return;
case OrcasDslPackage.COLUMN__DATA_TYPE:
setData_type((DataType)newValue);
return;
case OrcasDslPackage.COLUMN__PRECISION:
setPrecision((Integer)newValue);
return;
case OrcasDslPackage.COLUMN__SCALE:
setScale((Integer)newValue);
return;
case OrcasDslPackage.COLUMN__BYTEORCHAR:
setByteorchar((CharType)newValue);
return;
case OrcasDslPackage.COLUMN__UNSIGNED:
setUnsigned((Boolean)newValue);
return;
case OrcasDslPackage.COLUMN__WITH_TIME_ZONE:
setWith_time_zone((String)newValue);
return;
case OrcasDslPackage.COLUMN__OBJECT_TYPE:
setObject_type((String)newValue);
return;
case OrcasDslPackage.COLUMN__DEFAULT_VALUE:
setDefault_value((String)newValue);
return;
case OrcasDslPackage.COLUMN__VIRTUAL:
setVirtual((String)newValue);
return;
case OrcasDslPackage.COLUMN__DEFAULT_NAME:
setDefault_name((String)newValue);
return;
case OrcasDslPackage.COLUMN__IDENTITY:
setIdentity((ColumnIdentity)newValue);
return;
case OrcasDslPackage.COLUMN__NOT_NULL_CONSTRAINT_NAME:
setNot_null_constraint_name((String)newValue);
return;
case OrcasDslPackage.COLUMN__NOTNULL:
setNotnull((Boolean)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.COLUMN__NAME:
setName(NAME_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__DOMAIN:
setDomain(DOMAIN_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__NAME_STRING:
setName_string(NAME_STRING_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__DATA_TYPE:
setData_type(DATA_TYPE_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__PRECISION:
setPrecision(PRECISION_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__SCALE:
setScale(SCALE_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__BYTEORCHAR:
setByteorchar(BYTEORCHAR_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__UNSIGNED:
setUnsigned(UNSIGNED_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__WITH_TIME_ZONE:
setWith_time_zone(WITH_TIME_ZONE_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__OBJECT_TYPE:
setObject_type(OBJECT_TYPE_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__DEFAULT_VALUE:
setDefault_value(DEFAULT_VALUE_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__VIRTUAL:
setVirtual(VIRTUAL_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__DEFAULT_NAME:
setDefault_name(DEFAULT_NAME_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__IDENTITY:
setIdentity((ColumnIdentity)null);
return;
case OrcasDslPackage.COLUMN__NOT_NULL_CONSTRAINT_NAME:
setNot_null_constraint_name(NOT_NULL_CONSTRAINT_NAME_EDEFAULT);
return;
case OrcasDslPackage.COLUMN__NOTNULL:
setNotnull(NOTNULL_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.COLUMN__NAME:
return NAME_EDEFAULT == null ? name != null : !NAME_EDEFAULT.equals(name);
case OrcasDslPackage.COLUMN__DOMAIN:
return DOMAIN_EDEFAULT == null ? domain != null : !DOMAIN_EDEFAULT.equals(domain);
case OrcasDslPackage.COLUMN__NAME_STRING:
return NAME_STRING_EDEFAULT == null ? name_string != null : !NAME_STRING_EDEFAULT.equals(name_string);
case OrcasDslPackage.COLUMN__DATA_TYPE:
return data_type != DATA_TYPE_EDEFAULT;
case OrcasDslPackage.COLUMN__PRECISION:
return precision != PRECISION_EDEFAULT;
case OrcasDslPackage.COLUMN__SCALE:
return scale != SCALE_EDEFAULT;
case OrcasDslPackage.COLUMN__BYTEORCHAR:
return byteorchar != BYTEORCHAR_EDEFAULT;
case OrcasDslPackage.COLUMN__UNSIGNED:
return unsigned != UNSIGNED_EDEFAULT;
case OrcasDslPackage.COLUMN__WITH_TIME_ZONE:
return WITH_TIME_ZONE_EDEFAULT == null ? with_time_zone != null : !WITH_TIME_ZONE_EDEFAULT.equals(with_time_zone);
case OrcasDslPackage.COLUMN__OBJECT_TYPE:
return OBJECT_TYPE_EDEFAULT == null ? object_type != null : !OBJECT_TYPE_EDEFAULT.equals(object_type);
case OrcasDslPackage.COLUMN__DEFAULT_VALUE:
return DEFAULT_VALUE_EDEFAULT == null ? default_value != null : !DEFAULT_VALUE_EDEFAULT.equals(default_value);
case OrcasDslPackage.COLUMN__VIRTUAL:
return VIRTUAL_EDEFAULT == null ? virtual != null : !VIRTUAL_EDEFAULT.equals(virtual);
case OrcasDslPackage.COLUMN__DEFAULT_NAME:
return DEFAULT_NAME_EDEFAULT == null ? default_name != null : !DEFAULT_NAME_EDEFAULT.equals(default_name);
case OrcasDslPackage.COLUMN__IDENTITY:
return identity != null;
case OrcasDslPackage.COLUMN__NOT_NULL_CONSTRAINT_NAME:
return NOT_NULL_CONSTRAINT_NAME_EDEFAULT == null ? not_null_constraint_name != null : !NOT_NULL_CONSTRAINT_NAME_EDEFAULT.equals(not_null_constraint_name);
case OrcasDslPackage.COLUMN__NOTNULL:
return notnull != NOTNULL_EDEFAULT;
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (name: ");
result.append(name);
result.append(", domain: ");
result.append(domain);
result.append(", name_string: ");
result.append(name_string);
result.append(", data_type: ");
result.append(data_type);
result.append(", precision: ");
result.append(precision);
result.append(", scale: ");
result.append(scale);
result.append(", byteorchar: ");
result.append(byteorchar);
result.append(", unsigned: ");
result.append(unsigned);
result.append(", with_time_zone: ");
result.append(with_time_zone);
result.append(", object_type: ");
result.append(object_type);
result.append(", default_value: ");
result.append(default_value);
result.append(", virtual: ");
result.append(virtual);
result.append(", default_name: ");
result.append(default_name);
result.append(", not_null_constraint_name: ");
result.append(not_null_constraint_name);
result.append(", notnull: ");
result.append(notnull);
result.append(')');
return result.toString();
}
} //ColumnImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy