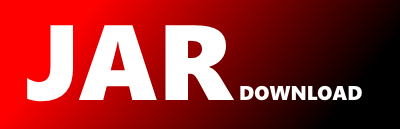
de.opitzconsulting.orcasDsl.impl.GenNameRuleImpl Maven / Gradle / Ivy
/**
*/
package de.opitzconsulting.orcasDsl.impl;
import de.opitzconsulting.orcasDsl.GenNameRule;
import de.opitzconsulting.orcasDsl.GenNameRulePart;
import de.opitzconsulting.orcasDsl.OrcasDslPackage;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
/**
*
* An implementation of the model object 'Gen Name Rule'.
*
*
* The following features are implemented:
*
*
* - {@link de.opitzconsulting.orcasDsl.impl.GenNameRuleImpl#getConstant_name Constant name}
* - {@link de.opitzconsulting.orcasDsl.impl.GenNameRuleImpl#getConstant_part Constant part}
* - {@link de.opitzconsulting.orcasDsl.impl.GenNameRuleImpl#getRegexp Regexp}
* - {@link de.opitzconsulting.orcasDsl.impl.GenNameRuleImpl#getReplace Replace}
*
*
* @generated
*/
public class GenNameRuleImpl extends MinimalEObjectImpl.Container implements GenNameRule
{
/**
* The default value of the '{@link #getConstant_name() Constant name}' attribute.
*
*
* @see #getConstant_name()
* @generated
* @ordered
*/
public static final String CONSTANT_NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getConstant_name() Constant name}' attribute.
*
*
* @see #getConstant_name()
* @generated
* @ordered
*/
public String constant_name = CONSTANT_NAME_EDEFAULT;
/**
* The default value of the '{@link #getConstant_part() Constant part}' attribute.
*
*
* @see #getConstant_part()
* @generated
* @ordered
*/
public static final GenNameRulePart CONSTANT_PART_EDEFAULT = GenNameRulePart.NULL;
/**
* The cached value of the '{@link #getConstant_part() Constant part}' attribute.
*
*
* @see #getConstant_part()
* @generated
* @ordered
*/
public GenNameRulePart constant_part = CONSTANT_PART_EDEFAULT;
/**
* The default value of the '{@link #getRegexp() Regexp}' attribute.
*
*
* @see #getRegexp()
* @generated
* @ordered
*/
public static final String REGEXP_EDEFAULT = null;
/**
* The cached value of the '{@link #getRegexp() Regexp}' attribute.
*
*
* @see #getRegexp()
* @generated
* @ordered
*/
public String regexp = REGEXP_EDEFAULT;
/**
* The default value of the '{@link #getReplace() Replace}' attribute.
*
*
* @see #getReplace()
* @generated
* @ordered
*/
public static final String REPLACE_EDEFAULT = null;
/**
* The cached value of the '{@link #getReplace() Replace}' attribute.
*
*
* @see #getReplace()
* @generated
* @ordered
*/
public String replace = REPLACE_EDEFAULT;
/**
*
*
* @generated
*/
public GenNameRuleImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
public EClass eStaticClass()
{
return OrcasDslPackage.Literals.GEN_NAME_RULE;
}
/**
*
*
* @generated
*/
public String getConstant_name()
{
return constant_name;
}
/**
*
*
* @generated
*/
public void setConstant_name(String newConstant_name)
{
String oldConstant_name = constant_name;
constant_name = newConstant_name;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.GEN_NAME_RULE__CONSTANT_NAME, oldConstant_name, constant_name));
}
/**
*
*
* @generated
*/
public GenNameRulePart getConstant_part()
{
return constant_part;
}
/**
*
*
* @generated
*/
public void setConstant_part(GenNameRulePart newConstant_part)
{
GenNameRulePart oldConstant_part = constant_part;
constant_part = newConstant_part == null ? CONSTANT_PART_EDEFAULT : newConstant_part;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.GEN_NAME_RULE__CONSTANT_PART, oldConstant_part, constant_part));
}
/**
*
*
* @generated
*/
public String getRegexp()
{
return regexp;
}
/**
*
*
* @generated
*/
public void setRegexp(String newRegexp)
{
String oldRegexp = regexp;
regexp = newRegexp;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.GEN_NAME_RULE__REGEXP, oldRegexp, regexp));
}
/**
*
*
* @generated
*/
public String getReplace()
{
return replace;
}
/**
*
*
* @generated
*/
public void setReplace(String newReplace)
{
String oldReplace = replace;
replace = newReplace;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.GEN_NAME_RULE__REPLACE, oldReplace, replace));
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_NAME:
return getConstant_name();
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_PART:
return getConstant_part();
case OrcasDslPackage.GEN_NAME_RULE__REGEXP:
return getRegexp();
case OrcasDslPackage.GEN_NAME_RULE__REPLACE:
return getReplace();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_NAME:
setConstant_name((String)newValue);
return;
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_PART:
setConstant_part((GenNameRulePart)newValue);
return;
case OrcasDslPackage.GEN_NAME_RULE__REGEXP:
setRegexp((String)newValue);
return;
case OrcasDslPackage.GEN_NAME_RULE__REPLACE:
setReplace((String)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_NAME:
setConstant_name(CONSTANT_NAME_EDEFAULT);
return;
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_PART:
setConstant_part(CONSTANT_PART_EDEFAULT);
return;
case OrcasDslPackage.GEN_NAME_RULE__REGEXP:
setRegexp(REGEXP_EDEFAULT);
return;
case OrcasDslPackage.GEN_NAME_RULE__REPLACE:
setReplace(REPLACE_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_NAME:
return CONSTANT_NAME_EDEFAULT == null ? constant_name != null : !CONSTANT_NAME_EDEFAULT.equals(constant_name);
case OrcasDslPackage.GEN_NAME_RULE__CONSTANT_PART:
return constant_part != CONSTANT_PART_EDEFAULT;
case OrcasDslPackage.GEN_NAME_RULE__REGEXP:
return REGEXP_EDEFAULT == null ? regexp != null : !REGEXP_EDEFAULT.equals(regexp);
case OrcasDslPackage.GEN_NAME_RULE__REPLACE:
return REPLACE_EDEFAULT == null ? replace != null : !REPLACE_EDEFAULT.equals(replace);
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (constant_name: ");
result.append(constant_name);
result.append(", constant_part: ");
result.append(constant_part);
result.append(", regexp: ");
result.append(regexp);
result.append(", replace: ");
result.append(replace);
result.append(')');
return result.toString();
}
} //GenNameRuleImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy