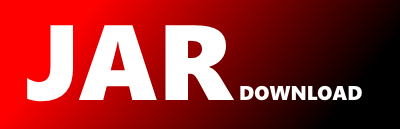
de.opitzconsulting.orcasDsl.impl.NestedTableStorageImpl Maven / Gradle / Ivy
/**
*/
package de.opitzconsulting.orcasDsl.impl;
import de.opitzconsulting.orcasDsl.NestedTableStorage;
import de.opitzconsulting.orcasDsl.OrcasDslPackage;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
/**
*
* An implementation of the model object 'Nested Table Storage'.
*
*
* The following features are implemented:
*
*
* - {@link de.opitzconsulting.orcasDsl.impl.NestedTableStorageImpl#getColumn_name Column name}
* - {@link de.opitzconsulting.orcasDsl.impl.NestedTableStorageImpl#getStorage_clause Storage clause}
* - {@link de.opitzconsulting.orcasDsl.impl.NestedTableStorageImpl#getStorage_clause_string Storage clause string}
*
*
* @generated
*/
public class NestedTableStorageImpl extends MinimalEObjectImpl.Container implements NestedTableStorage
{
/**
* The default value of the '{@link #getColumn_name() Column name}' attribute.
*
*
* @see #getColumn_name()
* @generated
* @ordered
*/
public static final String COLUMN_NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getColumn_name() Column name}' attribute.
*
*
* @see #getColumn_name()
* @generated
* @ordered
*/
public String column_name = COLUMN_NAME_EDEFAULT;
/**
* The default value of the '{@link #getStorage_clause() Storage clause}' attribute.
*
*
* @see #getStorage_clause()
* @generated
* @ordered
*/
public static final String STORAGE_CLAUSE_EDEFAULT = null;
/**
* The cached value of the '{@link #getStorage_clause() Storage clause}' attribute.
*
*
* @see #getStorage_clause()
* @generated
* @ordered
*/
public String storage_clause = STORAGE_CLAUSE_EDEFAULT;
/**
* The default value of the '{@link #getStorage_clause_string() Storage clause string}' attribute.
*
*
* @see #getStorage_clause_string()
* @generated
* @ordered
*/
public static final String STORAGE_CLAUSE_STRING_EDEFAULT = null;
/**
* The cached value of the '{@link #getStorage_clause_string() Storage clause string}' attribute.
*
*
* @see #getStorage_clause_string()
* @generated
* @ordered
*/
public String storage_clause_string = STORAGE_CLAUSE_STRING_EDEFAULT;
/**
*
*
* @generated
*/
public NestedTableStorageImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
public EClass eStaticClass()
{
return OrcasDslPackage.Literals.NESTED_TABLE_STORAGE;
}
/**
*
*
* @generated
*/
public String getColumn_name()
{
return column_name;
}
/**
*
*
* @generated
*/
public void setColumn_name(String newColumn_name)
{
String oldColumn_name = column_name;
column_name = newColumn_name;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.NESTED_TABLE_STORAGE__COLUMN_NAME, oldColumn_name, column_name));
}
/**
*
*
* @generated
*/
public String getStorage_clause()
{
return storage_clause;
}
/**
*
*
* @generated
*/
public void setStorage_clause(String newStorage_clause)
{
String oldStorage_clause = storage_clause;
storage_clause = newStorage_clause;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE, oldStorage_clause, storage_clause));
}
/**
*
*
* @generated
*/
public String getStorage_clause_string()
{
return storage_clause_string;
}
/**
*
*
* @generated
*/
public void setStorage_clause_string(String newStorage_clause_string)
{
String oldStorage_clause_string = storage_clause_string;
storage_clause_string = newStorage_clause_string;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE_STRING, oldStorage_clause_string, storage_clause_string));
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case OrcasDslPackage.NESTED_TABLE_STORAGE__COLUMN_NAME:
return getColumn_name();
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE:
return getStorage_clause();
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE_STRING:
return getStorage_clause_string();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case OrcasDslPackage.NESTED_TABLE_STORAGE__COLUMN_NAME:
setColumn_name((String)newValue);
return;
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE:
setStorage_clause((String)newValue);
return;
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE_STRING:
setStorage_clause_string((String)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.NESTED_TABLE_STORAGE__COLUMN_NAME:
setColumn_name(COLUMN_NAME_EDEFAULT);
return;
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE:
setStorage_clause(STORAGE_CLAUSE_EDEFAULT);
return;
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE_STRING:
setStorage_clause_string(STORAGE_CLAUSE_STRING_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.NESTED_TABLE_STORAGE__COLUMN_NAME:
return COLUMN_NAME_EDEFAULT == null ? column_name != null : !COLUMN_NAME_EDEFAULT.equals(column_name);
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE:
return STORAGE_CLAUSE_EDEFAULT == null ? storage_clause != null : !STORAGE_CLAUSE_EDEFAULT.equals(storage_clause);
case OrcasDslPackage.NESTED_TABLE_STORAGE__STORAGE_CLAUSE_STRING:
return STORAGE_CLAUSE_STRING_EDEFAULT == null ? storage_clause_string != null : !STORAGE_CLAUSE_STRING_EDEFAULT.equals(storage_clause_string);
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (column_name: ");
result.append(column_name);
result.append(", storage_clause: ");
result.append(storage_clause);
result.append(", storage_clause_string: ");
result.append(storage_clause_string);
result.append(')');
return result.toString();
}
} //NestedTableStorageImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy