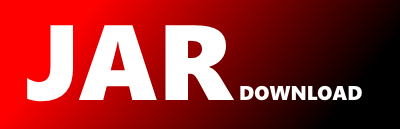
de.opitzconsulting.orcasDsl.impl.OrcasDslFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orcas-domainextension-syex Show documentation
Show all versions of orcas-domainextension-syex Show documentation
orcas model modified by syntax extensions (contains the original model). If custom-syntax-extensions are provided, there will be a custom artifact.
The newest version!
/**
*/
package de.opitzconsulting.orcasDsl.impl;
import de.opitzconsulting.orcasDsl.*;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EDataType;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.impl.EFactoryImpl;
import org.eclipse.emf.ecore.plugin.EcorePlugin;
/**
*
* An implementation of the model Factory.
*
* @generated
*/
public class OrcasDslFactoryImpl extends EFactoryImpl implements OrcasDslFactory
{
/**
* Creates the default factory implementation.
*
*
* @generated
*/
public static OrcasDslFactory init()
{
try
{
OrcasDslFactory theOrcasDslFactory = (OrcasDslFactory)EPackage.Registry.INSTANCE.getEFactory(OrcasDslPackage.eNS_URI);
if (theOrcasDslFactory != null)
{
return theOrcasDslFactory;
}
}
catch (Exception exception)
{
EcorePlugin.INSTANCE.log(exception);
}
return new OrcasDslFactoryImpl();
}
/**
* Creates an instance of the factory.
*
*
* @generated
*/
public OrcasDslFactoryImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
public EObject create(EClass eClass)
{
switch (eClass.getClassifierID())
{
case OrcasDslPackage.MODEL: return createModel();
case OrcasDslPackage.MODEL_ELEMENT: return createModelElement();
case OrcasDslPackage.TABLE: return createTable();
case OrcasDslPackage.TABLE_PARTITIONING: return createTablePartitioning();
case OrcasDslPackage.RANGE_PARTITIONS: return createRangePartitions();
case OrcasDslPackage.TABLE_SUB_PART: return createTableSubPart();
case OrcasDslPackage.RANGE_SUB_PARTS: return createRangeSubParts();
case OrcasDslPackage.LIST_SUB_PARTS: return createListSubParts();
case OrcasDslPackage.HASH_SUB_PARTS: return createHashSubParts();
case OrcasDslPackage.RANGE_SUB_PART: return createRangeSubPart();
case OrcasDslPackage.LIST_SUB_PART: return createListSubPart();
case OrcasDslPackage.SUB_SUB_PART: return createSubSubPart();
case OrcasDslPackage.RANGE_SUB_SUB_PART: return createRangeSubSubPart();
case OrcasDslPackage.LIST_SUB_SUB_PART: return createListSubSubPart();
case OrcasDslPackage.HASH_SUB_SUB_PART: return createHashSubSubPart();
case OrcasDslPackage.RANGE_PARTITION: return createRangePartition();
case OrcasDslPackage.RANGE_PARTITION_VALUE: return createRangePartitionValue();
case OrcasDslPackage.HASH_PARTITIONS: return createHashPartitions();
case OrcasDslPackage.HASH_PARTITION: return createHashPartition();
case OrcasDslPackage.REF_PARTITIONS: return createRefPartitions();
case OrcasDslPackage.REF_PARTITION: return createRefPartition();
case OrcasDslPackage.LIST_PARTITIONS: return createListPartitions();
case OrcasDslPackage.LIST_PARTITION: return createListPartition();
case OrcasDslPackage.LIST_PARTITION_VALUE: return createListPartitionValue();
case OrcasDslPackage.LOB_STORAGE: return createLobStorage();
case OrcasDslPackage.VARRAY_STORAGE: return createVarrayStorage();
case OrcasDslPackage.NESTED_TABLE_STORAGE: return createNestedTableStorage();
case OrcasDslPackage.LOB_STORAGE_PARAMETERS: return createLobStorageParameters();
case OrcasDslPackage.COLUMN: return createColumn();
case OrcasDslPackage.CONSTRAINT: return createConstraint();
case OrcasDslPackage.INDEX_OR_UNIQUE_KEY: return createIndexOrUniqueKey();
case OrcasDslPackage.FOREIGN_KEY: return createForeignKey();
case OrcasDslPackage.PRIMARY_KEY: return createPrimaryKey();
case OrcasDslPackage.UNIQUE_KEY: return createUniqueKey();
case OrcasDslPackage.INDEX: return createIndex();
case OrcasDslPackage.INDEX_EX_TABLE: return createIndexExTable();
case OrcasDslPackage.COLUMN_REF: return createColumnRef();
case OrcasDslPackage.SEQUENCE: return createSequence();
case OrcasDslPackage.COLUMN_IDENTITY: return createColumnIdentity();
case OrcasDslPackage.MVIEW: return createMview();
case OrcasDslPackage.MVIEW_LOG: return createMviewLog();
case OrcasDslPackage.COMMENT: return createComment();
case OrcasDslPackage.INLINE_COMMENT: return createInlineComment();
case OrcasDslPackage.DOMAIN_COLUMN: return createDomainColumn();
case OrcasDslPackage.HISTORY_TABLE: return createHistoryTable();
case OrcasDslPackage.DOMAIN: return createDomain();
case OrcasDslPackage.GEN_NAME_RULE: return createGenNameRule();
case OrcasDslPackage.GENERATE_PK: return createGeneratePk();
case OrcasDslPackage.GENERATE_UK: return createGenerateUk();
case OrcasDslPackage.GENERATE_INDEX: return createGenerateIndex();
case OrcasDslPackage.GENERATE_CC: return createGenerateCc();
case OrcasDslPackage.GENERATE_FK: return createGenerateFk();
case OrcasDslPackage.COLUMN_DOMAIN: return createColumnDomain();
default:
throw new IllegalArgumentException("The class '" + eClass.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
@Override
public Object createFromString(EDataType eDataType, String initialValue)
{
switch (eDataType.getClassifierID())
{
case OrcasDslPackage.LOB_STORAGE_TYPE:
return createLobStorageTypeFromString(eDataType, initialValue);
case OrcasDslPackage.LOB_DEDUPLICATE_TYPE:
return createLobDeduplicateTypeFromString(eDataType, initialValue);
case OrcasDslPackage.DATA_TYPE:
return createDataTypeFromString(eDataType, initialValue);
case OrcasDslPackage.FK_DELETE_RULE_TYPE:
return createFkDeleteRuleTypeFromString(eDataType, initialValue);
case OrcasDslPackage.DEFERR_TYPE:
return createDeferrTypeFromString(eDataType, initialValue);
case OrcasDslPackage.CHAR_TYPE:
return createCharTypeFromString(eDataType, initialValue);
case OrcasDslPackage.LOGGING_TYPE:
return createLoggingTypeFromString(eDataType, initialValue);
case OrcasDslPackage.ENABLE_TYPE:
return createEnableTypeFromString(eDataType, initialValue);
case OrcasDslPackage.PARALLEL_TYPE:
return createParallelTypeFromString(eDataType, initialValue);
case OrcasDslPackage.INDEX_GLOBAL_TYPE:
return createIndexGlobalTypeFromString(eDataType, initialValue);
case OrcasDslPackage.COMMENT_OBJECT_TYPE:
return createCommentObjectTypeFromString(eDataType, initialValue);
case OrcasDslPackage.PERMANENTNESS_TYPE:
return createPermanentnessTypeFromString(eDataType, initialValue);
case OrcasDslPackage.PERMANENTNESS_TRANSACTION_TYPE:
return createPermanentnessTransactionTypeFromString(eDataType, initialValue);
case OrcasDslPackage.CYCLE_TYPE:
return createCycleTypeFromString(eDataType, initialValue);
case OrcasDslPackage.ORDER_TYPE:
return createOrderTypeFromString(eDataType, initialValue);
case OrcasDslPackage.COMPRESS_FOR_TYPE:
return createCompressForTypeFromString(eDataType, initialValue);
case OrcasDslPackage.LOB_COMPRESS_FOR_TYPE:
return createLobCompressForTypeFromString(eDataType, initialValue);
case OrcasDslPackage.COMPRESS_TYPE:
return createCompressTypeFromString(eDataType, initialValue);
case OrcasDslPackage.BUILD_MODE_TYPE:
return createBuildModeTypeFromString(eDataType, initialValue);
case OrcasDslPackage.REFRESH_MODE_TYPE:
return createRefreshModeTypeFromString(eDataType, initialValue);
case OrcasDslPackage.REFRESH_METHOD_TYPE:
return createRefreshMethodTypeFromString(eDataType, initialValue);
case OrcasDslPackage.NEW_VALUES_TYPE:
return createNewValuesTypeFromString(eDataType, initialValue);
case OrcasDslPackage.SYNCHRONOUS_TYPE:
return createSynchronousTypeFromString(eDataType, initialValue);
case OrcasDslPackage.GEN_NAME_RULE_PART:
return createGenNameRulePartFromString(eDataType, initialValue);
default:
throw new IllegalArgumentException("The datatype '" + eDataType.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
@Override
public String convertToString(EDataType eDataType, Object instanceValue)
{
switch (eDataType.getClassifierID())
{
case OrcasDslPackage.LOB_STORAGE_TYPE:
return convertLobStorageTypeToString(eDataType, instanceValue);
case OrcasDslPackage.LOB_DEDUPLICATE_TYPE:
return convertLobDeduplicateTypeToString(eDataType, instanceValue);
case OrcasDslPackage.DATA_TYPE:
return convertDataTypeToString(eDataType, instanceValue);
case OrcasDslPackage.FK_DELETE_RULE_TYPE:
return convertFkDeleteRuleTypeToString(eDataType, instanceValue);
case OrcasDslPackage.DEFERR_TYPE:
return convertDeferrTypeToString(eDataType, instanceValue);
case OrcasDslPackage.CHAR_TYPE:
return convertCharTypeToString(eDataType, instanceValue);
case OrcasDslPackage.LOGGING_TYPE:
return convertLoggingTypeToString(eDataType, instanceValue);
case OrcasDslPackage.ENABLE_TYPE:
return convertEnableTypeToString(eDataType, instanceValue);
case OrcasDslPackage.PARALLEL_TYPE:
return convertParallelTypeToString(eDataType, instanceValue);
case OrcasDslPackage.INDEX_GLOBAL_TYPE:
return convertIndexGlobalTypeToString(eDataType, instanceValue);
case OrcasDslPackage.COMMENT_OBJECT_TYPE:
return convertCommentObjectTypeToString(eDataType, instanceValue);
case OrcasDslPackage.PERMANENTNESS_TYPE:
return convertPermanentnessTypeToString(eDataType, instanceValue);
case OrcasDslPackage.PERMANENTNESS_TRANSACTION_TYPE:
return convertPermanentnessTransactionTypeToString(eDataType, instanceValue);
case OrcasDslPackage.CYCLE_TYPE:
return convertCycleTypeToString(eDataType, instanceValue);
case OrcasDslPackage.ORDER_TYPE:
return convertOrderTypeToString(eDataType, instanceValue);
case OrcasDslPackage.COMPRESS_FOR_TYPE:
return convertCompressForTypeToString(eDataType, instanceValue);
case OrcasDslPackage.LOB_COMPRESS_FOR_TYPE:
return convertLobCompressForTypeToString(eDataType, instanceValue);
case OrcasDslPackage.COMPRESS_TYPE:
return convertCompressTypeToString(eDataType, instanceValue);
case OrcasDslPackage.BUILD_MODE_TYPE:
return convertBuildModeTypeToString(eDataType, instanceValue);
case OrcasDslPackage.REFRESH_MODE_TYPE:
return convertRefreshModeTypeToString(eDataType, instanceValue);
case OrcasDslPackage.REFRESH_METHOD_TYPE:
return convertRefreshMethodTypeToString(eDataType, instanceValue);
case OrcasDslPackage.NEW_VALUES_TYPE:
return convertNewValuesTypeToString(eDataType, instanceValue);
case OrcasDslPackage.SYNCHRONOUS_TYPE:
return convertSynchronousTypeToString(eDataType, instanceValue);
case OrcasDslPackage.GEN_NAME_RULE_PART:
return convertGenNameRulePartToString(eDataType, instanceValue);
default:
throw new IllegalArgumentException("The datatype '" + eDataType.getName() + "' is not a valid classifier");
}
}
/**
*
*
* @generated
*/
public Model createModel()
{
ModelImpl model = new ModelImpl();
return model;
}
/**
*
*
* @generated
*/
public ModelElement createModelElement()
{
ModelElementImpl modelElement = new ModelElementImpl();
return modelElement;
}
/**
*
*
* @generated
*/
public Table createTable()
{
TableImpl table = new TableImpl();
return table;
}
/**
*
*
* @generated
*/
public TablePartitioning createTablePartitioning()
{
TablePartitioningImpl tablePartitioning = new TablePartitioningImpl();
return tablePartitioning;
}
/**
*
*
* @generated
*/
public RangePartitions createRangePartitions()
{
RangePartitionsImpl rangePartitions = new RangePartitionsImpl();
return rangePartitions;
}
/**
*
*
* @generated
*/
public TableSubPart createTableSubPart()
{
TableSubPartImpl tableSubPart = new TableSubPartImpl();
return tableSubPart;
}
/**
*
*
* @generated
*/
public RangeSubParts createRangeSubParts()
{
RangeSubPartsImpl rangeSubParts = new RangeSubPartsImpl();
return rangeSubParts;
}
/**
*
*
* @generated
*/
public ListSubParts createListSubParts()
{
ListSubPartsImpl listSubParts = new ListSubPartsImpl();
return listSubParts;
}
/**
*
*
* @generated
*/
public HashSubParts createHashSubParts()
{
HashSubPartsImpl hashSubParts = new HashSubPartsImpl();
return hashSubParts;
}
/**
*
*
* @generated
*/
public RangeSubPart createRangeSubPart()
{
RangeSubPartImpl rangeSubPart = new RangeSubPartImpl();
return rangeSubPart;
}
/**
*
*
* @generated
*/
public ListSubPart createListSubPart()
{
ListSubPartImpl listSubPart = new ListSubPartImpl();
return listSubPart;
}
/**
*
*
* @generated
*/
public SubSubPart createSubSubPart()
{
SubSubPartImpl subSubPart = new SubSubPartImpl();
return subSubPart;
}
/**
*
*
* @generated
*/
public RangeSubSubPart createRangeSubSubPart()
{
RangeSubSubPartImpl rangeSubSubPart = new RangeSubSubPartImpl();
return rangeSubSubPart;
}
/**
*
*
* @generated
*/
public ListSubSubPart createListSubSubPart()
{
ListSubSubPartImpl listSubSubPart = new ListSubSubPartImpl();
return listSubSubPart;
}
/**
*
*
* @generated
*/
public HashSubSubPart createHashSubSubPart()
{
HashSubSubPartImpl hashSubSubPart = new HashSubSubPartImpl();
return hashSubSubPart;
}
/**
*
*
* @generated
*/
public RangePartition createRangePartition()
{
RangePartitionImpl rangePartition = new RangePartitionImpl();
return rangePartition;
}
/**
*
*
* @generated
*/
public RangePartitionValue createRangePartitionValue()
{
RangePartitionValueImpl rangePartitionValue = new RangePartitionValueImpl();
return rangePartitionValue;
}
/**
*
*
* @generated
*/
public HashPartitions createHashPartitions()
{
HashPartitionsImpl hashPartitions = new HashPartitionsImpl();
return hashPartitions;
}
/**
*
*
* @generated
*/
public HashPartition createHashPartition()
{
HashPartitionImpl hashPartition = new HashPartitionImpl();
return hashPartition;
}
/**
*
*
* @generated
*/
public RefPartitions createRefPartitions()
{
RefPartitionsImpl refPartitions = new RefPartitionsImpl();
return refPartitions;
}
/**
*
*
* @generated
*/
public RefPartition createRefPartition()
{
RefPartitionImpl refPartition = new RefPartitionImpl();
return refPartition;
}
/**
*
*
* @generated
*/
public ListPartitions createListPartitions()
{
ListPartitionsImpl listPartitions = new ListPartitionsImpl();
return listPartitions;
}
/**
*
*
* @generated
*/
public ListPartition createListPartition()
{
ListPartitionImpl listPartition = new ListPartitionImpl();
return listPartition;
}
/**
*
*
* @generated
*/
public ListPartitionValue createListPartitionValue()
{
ListPartitionValueImpl listPartitionValue = new ListPartitionValueImpl();
return listPartitionValue;
}
/**
*
*
* @generated
*/
public LobStorage createLobStorage()
{
LobStorageImpl lobStorage = new LobStorageImpl();
return lobStorage;
}
/**
*
*
* @generated
*/
public VarrayStorage createVarrayStorage()
{
VarrayStorageImpl varrayStorage = new VarrayStorageImpl();
return varrayStorage;
}
/**
*
*
* @generated
*/
public NestedTableStorage createNestedTableStorage()
{
NestedTableStorageImpl nestedTableStorage = new NestedTableStorageImpl();
return nestedTableStorage;
}
/**
*
*
* @generated
*/
public LobStorageParameters createLobStorageParameters()
{
LobStorageParametersImpl lobStorageParameters = new LobStorageParametersImpl();
return lobStorageParameters;
}
/**
*
*
* @generated
*/
public Column createColumn()
{
ColumnImpl column = new ColumnImpl();
return column;
}
/**
*
*
* @generated
*/
public Constraint createConstraint()
{
ConstraintImpl constraint = new ConstraintImpl();
return constraint;
}
/**
*
*
* @generated
*/
public IndexOrUniqueKey createIndexOrUniqueKey()
{
IndexOrUniqueKeyImpl indexOrUniqueKey = new IndexOrUniqueKeyImpl();
return indexOrUniqueKey;
}
/**
*
*
* @generated
*/
public ForeignKey createForeignKey()
{
ForeignKeyImpl foreignKey = new ForeignKeyImpl();
return foreignKey;
}
/**
*
*
* @generated
*/
public PrimaryKey createPrimaryKey()
{
PrimaryKeyImpl primaryKey = new PrimaryKeyImpl();
return primaryKey;
}
/**
*
*
* @generated
*/
public UniqueKey createUniqueKey()
{
UniqueKeyImpl uniqueKey = new UniqueKeyImpl();
return uniqueKey;
}
/**
*
*
* @generated
*/
public Index createIndex()
{
IndexImpl index = new IndexImpl();
return index;
}
/**
*
*
* @generated
*/
public IndexExTable createIndexExTable()
{
IndexExTableImpl indexExTable = new IndexExTableImpl();
return indexExTable;
}
/**
*
*
* @generated
*/
public ColumnRef createColumnRef()
{
ColumnRefImpl columnRef = new ColumnRefImpl();
return columnRef;
}
/**
*
*
* @generated
*/
public Sequence createSequence()
{
SequenceImpl sequence = new SequenceImpl();
return sequence;
}
/**
*
*
* @generated
*/
public ColumnIdentity createColumnIdentity()
{
ColumnIdentityImpl columnIdentity = new ColumnIdentityImpl();
return columnIdentity;
}
/**
*
*
* @generated
*/
public Mview createMview()
{
MviewImpl mview = new MviewImpl();
return mview;
}
/**
*
*
* @generated
*/
public MviewLog createMviewLog()
{
MviewLogImpl mviewLog = new MviewLogImpl();
return mviewLog;
}
/**
*
*
* @generated
*/
public Comment createComment()
{
CommentImpl comment = new CommentImpl();
return comment;
}
/**
*
*
* @generated
*/
public InlineComment createInlineComment()
{
InlineCommentImpl inlineComment = new InlineCommentImpl();
return inlineComment;
}
/**
*
*
* @generated
*/
public DomainColumn createDomainColumn()
{
DomainColumnImpl domainColumn = new DomainColumnImpl();
return domainColumn;
}
/**
*
*
* @generated
*/
public HistoryTable createHistoryTable()
{
HistoryTableImpl historyTable = new HistoryTableImpl();
return historyTable;
}
/**
*
*
* @generated
*/
public Domain createDomain()
{
DomainImpl domain = new DomainImpl();
return domain;
}
/**
*
*
* @generated
*/
public GenNameRule createGenNameRule()
{
GenNameRuleImpl genNameRule = new GenNameRuleImpl();
return genNameRule;
}
/**
*
*
* @generated
*/
public GeneratePk createGeneratePk()
{
GeneratePkImpl generatePk = new GeneratePkImpl();
return generatePk;
}
/**
*
*
* @generated
*/
public GenerateUk createGenerateUk()
{
GenerateUkImpl generateUk = new GenerateUkImpl();
return generateUk;
}
/**
*
*
* @generated
*/
public GenerateIndex createGenerateIndex()
{
GenerateIndexImpl generateIndex = new GenerateIndexImpl();
return generateIndex;
}
/**
*
*
* @generated
*/
public GenerateCc createGenerateCc()
{
GenerateCcImpl generateCc = new GenerateCcImpl();
return generateCc;
}
/**
*
*
* @generated
*/
public GenerateFk createGenerateFk()
{
GenerateFkImpl generateFk = new GenerateFkImpl();
return generateFk;
}
/**
*
*
* @generated
*/
public ColumnDomain createColumnDomain()
{
ColumnDomainImpl columnDomain = new ColumnDomainImpl();
return columnDomain;
}
/**
*
*
* @generated
*/
public LobStorageType createLobStorageTypeFromString(EDataType eDataType, String initialValue)
{
LobStorageType result = LobStorageType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertLobStorageTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public LobDeduplicateType createLobDeduplicateTypeFromString(EDataType eDataType, String initialValue)
{
LobDeduplicateType result = LobDeduplicateType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertLobDeduplicateTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public DataType createDataTypeFromString(EDataType eDataType, String initialValue)
{
DataType result = DataType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertDataTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public FkDeleteRuleType createFkDeleteRuleTypeFromString(EDataType eDataType, String initialValue)
{
FkDeleteRuleType result = FkDeleteRuleType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertFkDeleteRuleTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public DeferrType createDeferrTypeFromString(EDataType eDataType, String initialValue)
{
DeferrType result = DeferrType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertDeferrTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public CharType createCharTypeFromString(EDataType eDataType, String initialValue)
{
CharType result = CharType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertCharTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public LoggingType createLoggingTypeFromString(EDataType eDataType, String initialValue)
{
LoggingType result = LoggingType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertLoggingTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public EnableType createEnableTypeFromString(EDataType eDataType, String initialValue)
{
EnableType result = EnableType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertEnableTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public ParallelType createParallelTypeFromString(EDataType eDataType, String initialValue)
{
ParallelType result = ParallelType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertParallelTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public IndexGlobalType createIndexGlobalTypeFromString(EDataType eDataType, String initialValue)
{
IndexGlobalType result = IndexGlobalType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertIndexGlobalTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public CommentObjectType createCommentObjectTypeFromString(EDataType eDataType, String initialValue)
{
CommentObjectType result = CommentObjectType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertCommentObjectTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public PermanentnessType createPermanentnessTypeFromString(EDataType eDataType, String initialValue)
{
PermanentnessType result = PermanentnessType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertPermanentnessTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public PermanentnessTransactionType createPermanentnessTransactionTypeFromString(EDataType eDataType, String initialValue)
{
PermanentnessTransactionType result = PermanentnessTransactionType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertPermanentnessTransactionTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public CycleType createCycleTypeFromString(EDataType eDataType, String initialValue)
{
CycleType result = CycleType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertCycleTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public OrderType createOrderTypeFromString(EDataType eDataType, String initialValue)
{
OrderType result = OrderType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertOrderTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public CompressForType createCompressForTypeFromString(EDataType eDataType, String initialValue)
{
CompressForType result = CompressForType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertCompressForTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public LobCompressForType createLobCompressForTypeFromString(EDataType eDataType, String initialValue)
{
LobCompressForType result = LobCompressForType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertLobCompressForTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public CompressType createCompressTypeFromString(EDataType eDataType, String initialValue)
{
CompressType result = CompressType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertCompressTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public BuildModeType createBuildModeTypeFromString(EDataType eDataType, String initialValue)
{
BuildModeType result = BuildModeType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertBuildModeTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public RefreshModeType createRefreshModeTypeFromString(EDataType eDataType, String initialValue)
{
RefreshModeType result = RefreshModeType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertRefreshModeTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public RefreshMethodType createRefreshMethodTypeFromString(EDataType eDataType, String initialValue)
{
RefreshMethodType result = RefreshMethodType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertRefreshMethodTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public NewValuesType createNewValuesTypeFromString(EDataType eDataType, String initialValue)
{
NewValuesType result = NewValuesType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertNewValuesTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public SynchronousType createSynchronousTypeFromString(EDataType eDataType, String initialValue)
{
SynchronousType result = SynchronousType.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertSynchronousTypeToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public GenNameRulePart createGenNameRulePartFromString(EDataType eDataType, String initialValue)
{
GenNameRulePart result = GenNameRulePart.get(initialValue);
if (result == null) throw new IllegalArgumentException("The value '" + initialValue + "' is not a valid enumerator of '" + eDataType.getName() + "'");
return result;
}
/**
*
*
* @generated
*/
public String convertGenNameRulePartToString(EDataType eDataType, Object instanceValue)
{
return instanceValue == null ? null : instanceValue.toString();
}
/**
*
*
* @generated
*/
public OrcasDslPackage getOrcasDslPackage()
{
return (OrcasDslPackage)getEPackage();
}
/**
*
*
* @deprecated
* @generated
*/
@Deprecated
public static OrcasDslPackage getPackage()
{
return OrcasDslPackage.eINSTANCE;
}
} //OrcasDslFactoryImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy