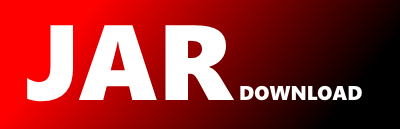
de.opitzconsulting.orcasDsl.impl.SequenceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orcas-domainextension-syex Show documentation
Show all versions of orcas-domainextension-syex Show documentation
orcas model modified by syntax extensions (contains the original model). If custom-syntax-extensions are provided, there will be a custom artifact.
The newest version!
/**
*/
package de.opitzconsulting.orcasDsl.impl;
import de.opitzconsulting.orcasDsl.CycleType;
import de.opitzconsulting.orcasDsl.OrcasDslPackage;
import de.opitzconsulting.orcasDsl.OrderType;
import de.opitzconsulting.orcasDsl.Sequence;
import java.math.BigInteger;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
/**
*
* An implementation of the model object 'Sequence'.
*
*
* The following features are implemented:
*
*
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getSequence_name Sequence name}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getStartwith Startwith}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getIncrement_by Increment by}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getMaxvalue Maxvalue}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getMinvalue Minvalue}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getCycle Cycle}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getCache Cache}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getOrder Order}
* - {@link de.opitzconsulting.orcasDsl.impl.SequenceImpl#getMax_value_select Max value select}
*
*
* @generated
*/
public class SequenceImpl extends ModelElementImpl implements Sequence
{
/**
* The default value of the '{@link #getSequence_name() Sequence name}' attribute.
*
*
* @see #getSequence_name()
* @generated
* @ordered
*/
public static final String SEQUENCE_NAME_EDEFAULT = null;
/**
* The cached value of the '{@link #getSequence_name() Sequence name}' attribute.
*
*
* @see #getSequence_name()
* @generated
* @ordered
*/
public String sequence_name = SEQUENCE_NAME_EDEFAULT;
/**
* The default value of the '{@link #getStartwith() Startwith}' attribute.
*
*
* @see #getStartwith()
* @generated
* @ordered
*/
public static final BigInteger STARTWITH_EDEFAULT = null;
/**
* The cached value of the '{@link #getStartwith() Startwith}' attribute.
*
*
* @see #getStartwith()
* @generated
* @ordered
*/
public BigInteger startwith = STARTWITH_EDEFAULT;
/**
* The default value of the '{@link #getIncrement_by() Increment by}' attribute.
*
*
* @see #getIncrement_by()
* @generated
* @ordered
*/
public static final BigInteger INCREMENT_BY_EDEFAULT = null;
/**
* The cached value of the '{@link #getIncrement_by() Increment by}' attribute.
*
*
* @see #getIncrement_by()
* @generated
* @ordered
*/
public BigInteger increment_by = INCREMENT_BY_EDEFAULT;
/**
* The default value of the '{@link #getMaxvalue() Maxvalue}' attribute.
*
*
* @see #getMaxvalue()
* @generated
* @ordered
*/
public static final BigInteger MAXVALUE_EDEFAULT = null;
/**
* The cached value of the '{@link #getMaxvalue() Maxvalue}' attribute.
*
*
* @see #getMaxvalue()
* @generated
* @ordered
*/
public BigInteger maxvalue = MAXVALUE_EDEFAULT;
/**
* The default value of the '{@link #getMinvalue() Minvalue}' attribute.
*
*
* @see #getMinvalue()
* @generated
* @ordered
*/
public static final BigInteger MINVALUE_EDEFAULT = null;
/**
* The cached value of the '{@link #getMinvalue() Minvalue}' attribute.
*
*
* @see #getMinvalue()
* @generated
* @ordered
*/
public BigInteger minvalue = MINVALUE_EDEFAULT;
/**
* The default value of the '{@link #getCycle() Cycle}' attribute.
*
*
* @see #getCycle()
* @generated
* @ordered
*/
public static final CycleType CYCLE_EDEFAULT = null;
/**
* The cached value of the '{@link #getCycle() Cycle}' attribute.
*
*
* @see #getCycle()
* @generated
* @ordered
*/
public CycleType cycle = CYCLE_EDEFAULT;
/**
* The default value of the '{@link #getCache() Cache}' attribute.
*
*
* @see #getCache()
* @generated
* @ordered
*/
public static final BigInteger CACHE_EDEFAULT = null;
/**
* The cached value of the '{@link #getCache() Cache}' attribute.
*
*
* @see #getCache()
* @generated
* @ordered
*/
public BigInteger cache = CACHE_EDEFAULT;
/**
* The default value of the '{@link #getOrder() Order}' attribute.
*
*
* @see #getOrder()
* @generated
* @ordered
*/
public static final OrderType ORDER_EDEFAULT = null;
/**
* The cached value of the '{@link #getOrder() Order}' attribute.
*
*
* @see #getOrder()
* @generated
* @ordered
*/
public OrderType order = ORDER_EDEFAULT;
/**
* The default value of the '{@link #getMax_value_select() Max value select}' attribute.
*
*
* @see #getMax_value_select()
* @generated
* @ordered
*/
public static final String MAX_VALUE_SELECT_EDEFAULT = null;
/**
* The cached value of the '{@link #getMax_value_select() Max value select}' attribute.
*
*
* @see #getMax_value_select()
* @generated
* @ordered
*/
public String max_value_select = MAX_VALUE_SELECT_EDEFAULT;
/**
*
*
* @generated
*/
public SequenceImpl()
{
super();
}
/**
*
*
* @generated
*/
@Override
public EClass eStaticClass()
{
return OrcasDslPackage.Literals.SEQUENCE;
}
/**
*
*
* @generated
*/
public String getSequence_name()
{
return sequence_name;
}
/**
*
*
* @generated
*/
public void setSequence_name(String newSequence_name)
{
String oldSequence_name = sequence_name;
sequence_name = newSequence_name;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__SEQUENCE_NAME, oldSequence_name, sequence_name));
}
/**
*
*
* @generated
*/
public BigInteger getStartwith()
{
return startwith;
}
/**
*
*
* @generated
*/
public void setStartwith(BigInteger newStartwith)
{
BigInteger oldStartwith = startwith;
startwith = newStartwith;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__STARTWITH, oldStartwith, startwith));
}
/**
*
*
* @generated
*/
public BigInteger getIncrement_by()
{
return increment_by;
}
/**
*
*
* @generated
*/
public void setIncrement_by(BigInteger newIncrement_by)
{
BigInteger oldIncrement_by = increment_by;
increment_by = newIncrement_by;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__INCREMENT_BY, oldIncrement_by, increment_by));
}
/**
*
*
* @generated
*/
public BigInteger getMaxvalue()
{
return maxvalue;
}
/**
*
*
* @generated
*/
public void setMaxvalue(BigInteger newMaxvalue)
{
BigInteger oldMaxvalue = maxvalue;
maxvalue = newMaxvalue;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__MAXVALUE, oldMaxvalue, maxvalue));
}
/**
*
*
* @generated
*/
public BigInteger getMinvalue()
{
return minvalue;
}
/**
*
*
* @generated
*/
public void setMinvalue(BigInteger newMinvalue)
{
BigInteger oldMinvalue = minvalue;
minvalue = newMinvalue;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__MINVALUE, oldMinvalue, minvalue));
}
/**
*
*
* @generated
*/
public CycleType getCycle()
{
return cycle;
}
/**
*
*
* @generated
*/
public void setCycle(CycleType newCycle)
{
CycleType oldCycle = cycle;
cycle = newCycle == null ? CYCLE_EDEFAULT : newCycle;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__CYCLE, oldCycle, cycle));
}
/**
*
*
* @generated
*/
public BigInteger getCache()
{
return cache;
}
/**
*
*
* @generated
*/
public void setCache(BigInteger newCache)
{
BigInteger oldCache = cache;
cache = newCache;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__CACHE, oldCache, cache));
}
/**
*
*
* @generated
*/
public OrderType getOrder()
{
return order;
}
/**
*
*
* @generated
*/
public void setOrder(OrderType newOrder)
{
OrderType oldOrder = order;
order = newOrder == null ? ORDER_EDEFAULT : newOrder;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__ORDER, oldOrder, order));
}
/**
*
*
* @generated
*/
public String getMax_value_select()
{
return max_value_select;
}
/**
*
*
* @generated
*/
public void setMax_value_select(String newMax_value_select)
{
String oldMax_value_select = max_value_select;
max_value_select = newMax_value_select;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, OrcasDslPackage.SEQUENCE__MAX_VALUE_SELECT, oldMax_value_select, max_value_select));
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType)
{
switch (featureID)
{
case OrcasDslPackage.SEQUENCE__SEQUENCE_NAME:
return getSequence_name();
case OrcasDslPackage.SEQUENCE__STARTWITH:
return getStartwith();
case OrcasDslPackage.SEQUENCE__INCREMENT_BY:
return getIncrement_by();
case OrcasDslPackage.SEQUENCE__MAXVALUE:
return getMaxvalue();
case OrcasDslPackage.SEQUENCE__MINVALUE:
return getMinvalue();
case OrcasDslPackage.SEQUENCE__CYCLE:
return getCycle();
case OrcasDslPackage.SEQUENCE__CACHE:
return getCache();
case OrcasDslPackage.SEQUENCE__ORDER:
return getOrder();
case OrcasDslPackage.SEQUENCE__MAX_VALUE_SELECT:
return getMax_value_select();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue)
{
switch (featureID)
{
case OrcasDslPackage.SEQUENCE__SEQUENCE_NAME:
setSequence_name((String)newValue);
return;
case OrcasDslPackage.SEQUENCE__STARTWITH:
setStartwith((BigInteger)newValue);
return;
case OrcasDslPackage.SEQUENCE__INCREMENT_BY:
setIncrement_by((BigInteger)newValue);
return;
case OrcasDslPackage.SEQUENCE__MAXVALUE:
setMaxvalue((BigInteger)newValue);
return;
case OrcasDslPackage.SEQUENCE__MINVALUE:
setMinvalue((BigInteger)newValue);
return;
case OrcasDslPackage.SEQUENCE__CYCLE:
setCycle((CycleType)newValue);
return;
case OrcasDslPackage.SEQUENCE__CACHE:
setCache((BigInteger)newValue);
return;
case OrcasDslPackage.SEQUENCE__ORDER:
setOrder((OrderType)newValue);
return;
case OrcasDslPackage.SEQUENCE__MAX_VALUE_SELECT:
setMax_value_select((String)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.SEQUENCE__SEQUENCE_NAME:
setSequence_name(SEQUENCE_NAME_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__STARTWITH:
setStartwith(STARTWITH_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__INCREMENT_BY:
setIncrement_by(INCREMENT_BY_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__MAXVALUE:
setMaxvalue(MAXVALUE_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__MINVALUE:
setMinvalue(MINVALUE_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__CYCLE:
setCycle(CYCLE_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__CACHE:
setCache(CACHE_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__ORDER:
setOrder(ORDER_EDEFAULT);
return;
case OrcasDslPackage.SEQUENCE__MAX_VALUE_SELECT:
setMax_value_select(MAX_VALUE_SELECT_EDEFAULT);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID)
{
switch (featureID)
{
case OrcasDslPackage.SEQUENCE__SEQUENCE_NAME:
return SEQUENCE_NAME_EDEFAULT == null ? sequence_name != null : !SEQUENCE_NAME_EDEFAULT.equals(sequence_name);
case OrcasDslPackage.SEQUENCE__STARTWITH:
return STARTWITH_EDEFAULT == null ? startwith != null : !STARTWITH_EDEFAULT.equals(startwith);
case OrcasDslPackage.SEQUENCE__INCREMENT_BY:
return INCREMENT_BY_EDEFAULT == null ? increment_by != null : !INCREMENT_BY_EDEFAULT.equals(increment_by);
case OrcasDslPackage.SEQUENCE__MAXVALUE:
return MAXVALUE_EDEFAULT == null ? maxvalue != null : !MAXVALUE_EDEFAULT.equals(maxvalue);
case OrcasDslPackage.SEQUENCE__MINVALUE:
return MINVALUE_EDEFAULT == null ? minvalue != null : !MINVALUE_EDEFAULT.equals(minvalue);
case OrcasDslPackage.SEQUENCE__CYCLE:
return cycle != CYCLE_EDEFAULT;
case OrcasDslPackage.SEQUENCE__CACHE:
return CACHE_EDEFAULT == null ? cache != null : !CACHE_EDEFAULT.equals(cache);
case OrcasDslPackage.SEQUENCE__ORDER:
return order != ORDER_EDEFAULT;
case OrcasDslPackage.SEQUENCE__MAX_VALUE_SELECT:
return MAX_VALUE_SELECT_EDEFAULT == null ? max_value_select != null : !MAX_VALUE_SELECT_EDEFAULT.equals(max_value_select);
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString()
{
if (eIsProxy()) return super.toString();
StringBuffer result = new StringBuffer(super.toString());
result.append(" (sequence_name: ");
result.append(sequence_name);
result.append(", startwith: ");
result.append(startwith);
result.append(", increment_by: ");
result.append(increment_by);
result.append(", maxvalue: ");
result.append(maxvalue);
result.append(", minvalue: ");
result.append(minvalue);
result.append(", cycle: ");
result.append(cycle);
result.append(", cache: ");
result.append(cache);
result.append(", order: ");
result.append(order);
result.append(", max_value_select: ");
result.append(max_value_select);
result.append(')');
return result.toString();
}
} //SequenceImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy