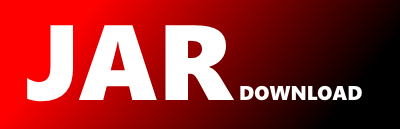
de.opitzconsulting.orcasDsl.util.OrcasDslSwitch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orcas-domainextension-syex Show documentation
Show all versions of orcas-domainextension-syex Show documentation
orcas model modified by syntax extensions (contains the original model). If custom-syntax-extensions are provided, there will be a custom artifact.
/**
*/
package de.opitzconsulting.orcasDsl.util;
import de.opitzconsulting.orcasDsl.*;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.util.Switch;
/**
*
* The Switch for the model's inheritance hierarchy.
* It supports the call {@link #doSwitch(EObject) doSwitch(object)}
* to invoke the caseXXX
method for each class of the model,
* starting with the actual class of the object
* and proceeding up the inheritance hierarchy
* until a non-null result is returned,
* which is the result of the switch.
*
* @see de.opitzconsulting.orcasDsl.OrcasDslPackage
* @generated
*/
public class OrcasDslSwitch extends Switch
{
/**
* The cached model package
*
*
* @generated
*/
protected static OrcasDslPackage modelPackage;
/**
* Creates an instance of the switch.
*
*
* @generated
*/
public OrcasDslSwitch()
{
if (modelPackage == null)
{
modelPackage = OrcasDslPackage.eINSTANCE;
}
}
/**
* Checks whether this is a switch for the given package.
*
*
* @param ePackage the package in question.
* @return whether this is a switch for the given package.
* @generated
*/
@Override
protected boolean isSwitchFor(EPackage ePackage)
{
return ePackage == modelPackage;
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
@Override
protected T doSwitch(int classifierID, EObject theEObject)
{
switch (classifierID)
{
case OrcasDslPackage.MODEL:
{
Model model = (Model)theEObject;
T result = caseModel(model);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.MODEL_ELEMENT:
{
ModelElement modelElement = (ModelElement)theEObject;
T result = caseModelElement(modelElement);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.TABLE:
{
Table table = (Table)theEObject;
T result = caseTable(table);
if (result == null) result = caseModelElement(table);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.TABLE_PARTITIONING:
{
TablePartitioning tablePartitioning = (TablePartitioning)theEObject;
T result = caseTablePartitioning(tablePartitioning);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.RANGE_PARTITIONS:
{
RangePartitions rangePartitions = (RangePartitions)theEObject;
T result = caseRangePartitions(rangePartitions);
if (result == null) result = caseTablePartitioning(rangePartitions);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.TABLE_SUB_PART:
{
TableSubPart tableSubPart = (TableSubPart)theEObject;
T result = caseTableSubPart(tableSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.RANGE_SUB_PARTS:
{
RangeSubParts rangeSubParts = (RangeSubParts)theEObject;
T result = caseRangeSubParts(rangeSubParts);
if (result == null) result = caseTableSubPart(rangeSubParts);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LIST_SUB_PARTS:
{
ListSubParts listSubParts = (ListSubParts)theEObject;
T result = caseListSubParts(listSubParts);
if (result == null) result = caseTableSubPart(listSubParts);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.HASH_SUB_PARTS:
{
HashSubParts hashSubParts = (HashSubParts)theEObject;
T result = caseHashSubParts(hashSubParts);
if (result == null) result = caseTableSubPart(hashSubParts);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.RANGE_SUB_PART:
{
RangeSubPart rangeSubPart = (RangeSubPart)theEObject;
T result = caseRangeSubPart(rangeSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LIST_SUB_PART:
{
ListSubPart listSubPart = (ListSubPart)theEObject;
T result = caseListSubPart(listSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.SUB_SUB_PART:
{
SubSubPart subSubPart = (SubSubPart)theEObject;
T result = caseSubSubPart(subSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.RANGE_SUB_SUB_PART:
{
RangeSubSubPart rangeSubSubPart = (RangeSubSubPart)theEObject;
T result = caseRangeSubSubPart(rangeSubSubPart);
if (result == null) result = caseSubSubPart(rangeSubSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LIST_SUB_SUB_PART:
{
ListSubSubPart listSubSubPart = (ListSubSubPart)theEObject;
T result = caseListSubSubPart(listSubSubPart);
if (result == null) result = caseSubSubPart(listSubSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.HASH_SUB_SUB_PART:
{
HashSubSubPart hashSubSubPart = (HashSubSubPart)theEObject;
T result = caseHashSubSubPart(hashSubSubPart);
if (result == null) result = caseSubSubPart(hashSubSubPart);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.RANGE_PARTITION:
{
RangePartition rangePartition = (RangePartition)theEObject;
T result = caseRangePartition(rangePartition);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.RANGE_PARTITION_VALUE:
{
RangePartitionValue rangePartitionValue = (RangePartitionValue)theEObject;
T result = caseRangePartitionValue(rangePartitionValue);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.HASH_PARTITIONS:
{
HashPartitions hashPartitions = (HashPartitions)theEObject;
T result = caseHashPartitions(hashPartitions);
if (result == null) result = caseTablePartitioning(hashPartitions);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.HASH_PARTITION:
{
HashPartition hashPartition = (HashPartition)theEObject;
T result = caseHashPartition(hashPartition);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.REF_PARTITIONS:
{
RefPartitions refPartitions = (RefPartitions)theEObject;
T result = caseRefPartitions(refPartitions);
if (result == null) result = caseTablePartitioning(refPartitions);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.REF_PARTITION:
{
RefPartition refPartition = (RefPartition)theEObject;
T result = caseRefPartition(refPartition);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LIST_PARTITIONS:
{
ListPartitions listPartitions = (ListPartitions)theEObject;
T result = caseListPartitions(listPartitions);
if (result == null) result = caseTablePartitioning(listPartitions);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LIST_PARTITION:
{
ListPartition listPartition = (ListPartition)theEObject;
T result = caseListPartition(listPartition);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LIST_PARTITION_VALUE:
{
ListPartitionValue listPartitionValue = (ListPartitionValue)theEObject;
T result = caseListPartitionValue(listPartitionValue);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LOB_STORAGE:
{
LobStorage lobStorage = (LobStorage)theEObject;
T result = caseLobStorage(lobStorage);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.VARRAY_STORAGE:
{
VarrayStorage varrayStorage = (VarrayStorage)theEObject;
T result = caseVarrayStorage(varrayStorage);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.NESTED_TABLE_STORAGE:
{
NestedTableStorage nestedTableStorage = (NestedTableStorage)theEObject;
T result = caseNestedTableStorage(nestedTableStorage);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.LOB_STORAGE_PARAMETERS:
{
LobStorageParameters lobStorageParameters = (LobStorageParameters)theEObject;
T result = caseLobStorageParameters(lobStorageParameters);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.COLUMN:
{
Column column = (Column)theEObject;
T result = caseColumn(column);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.CONSTRAINT:
{
Constraint constraint = (Constraint)theEObject;
T result = caseConstraint(constraint);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.INDEX_OR_UNIQUE_KEY:
{
IndexOrUniqueKey indexOrUniqueKey = (IndexOrUniqueKey)theEObject;
T result = caseIndexOrUniqueKey(indexOrUniqueKey);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.FOREIGN_KEY:
{
ForeignKey foreignKey = (ForeignKey)theEObject;
T result = caseForeignKey(foreignKey);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.PRIMARY_KEY:
{
PrimaryKey primaryKey = (PrimaryKey)theEObject;
T result = casePrimaryKey(primaryKey);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.UNIQUE_KEY:
{
UniqueKey uniqueKey = (UniqueKey)theEObject;
T result = caseUniqueKey(uniqueKey);
if (result == null) result = caseIndexOrUniqueKey(uniqueKey);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.INDEX:
{
Index index = (Index)theEObject;
T result = caseIndex(index);
if (result == null) result = caseIndexOrUniqueKey(index);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.INDEX_EX_TABLE:
{
IndexExTable indexExTable = (IndexExTable)theEObject;
T result = caseIndexExTable(indexExTable);
if (result == null) result = caseModelElement(indexExTable);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.COLUMN_REF:
{
ColumnRef columnRef = (ColumnRef)theEObject;
T result = caseColumnRef(columnRef);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.SEQUENCE:
{
Sequence sequence = (Sequence)theEObject;
T result = caseSequence(sequence);
if (result == null) result = caseModelElement(sequence);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.COLUMN_IDENTITY:
{
ColumnIdentity columnIdentity = (ColumnIdentity)theEObject;
T result = caseColumnIdentity(columnIdentity);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.MVIEW:
{
Mview mview = (Mview)theEObject;
T result = caseMview(mview);
if (result == null) result = caseModelElement(mview);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.MVIEW_LOG:
{
MviewLog mviewLog = (MviewLog)theEObject;
T result = caseMviewLog(mviewLog);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.COMMENT:
{
Comment comment = (Comment)theEObject;
T result = caseComment(comment);
if (result == null) result = caseModelElement(comment);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.INLINE_COMMENT:
{
InlineComment inlineComment = (InlineComment)theEObject;
T result = caseInlineComment(inlineComment);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.DOMAIN_COLUMN:
{
DomainColumn domainColumn = (DomainColumn)theEObject;
T result = caseDomainColumn(domainColumn);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.HISTORY_TABLE:
{
HistoryTable historyTable = (HistoryTable)theEObject;
T result = caseHistoryTable(historyTable);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.DOMAIN:
{
Domain domain = (Domain)theEObject;
T result = caseDomain(domain);
if (result == null) result = caseModelElement(domain);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.GEN_NAME_RULE:
{
GenNameRule genNameRule = (GenNameRule)theEObject;
T result = caseGenNameRule(genNameRule);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.GENERATE_PK:
{
GeneratePk generatePk = (GeneratePk)theEObject;
T result = caseGeneratePk(generatePk);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.GENERATE_UK:
{
GenerateUk generateUk = (GenerateUk)theEObject;
T result = caseGenerateUk(generateUk);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.GENERATE_INDEX:
{
GenerateIndex generateIndex = (GenerateIndex)theEObject;
T result = caseGenerateIndex(generateIndex);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.GENERATE_CC:
{
GenerateCc generateCc = (GenerateCc)theEObject;
T result = caseGenerateCc(generateCc);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.GENERATE_FK:
{
GenerateFk generateFk = (GenerateFk)theEObject;
T result = caseGenerateFk(generateFk);
if (result == null) result = defaultCase(theEObject);
return result;
}
case OrcasDslPackage.COLUMN_DOMAIN:
{
ColumnDomain columnDomain = (ColumnDomain)theEObject;
T result = caseColumnDomain(columnDomain);
if (result == null) result = caseModelElement(columnDomain);
if (result == null) result = defaultCase(theEObject);
return result;
}
default: return defaultCase(theEObject);
}
}
/**
* Returns the result of interpreting the object as an instance of 'Model'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Model'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseModel(Model object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Model Element'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Model Element'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseModelElement(ModelElement object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Table'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Table'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseTable(Table object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Table Partitioning'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Table Partitioning'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseTablePartitioning(TablePartitioning object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Range Partitions'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Range Partitions'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRangePartitions(RangePartitions object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Table Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Table Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseTableSubPart(TableSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Range Sub Parts'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Range Sub Parts'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRangeSubParts(RangeSubParts object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'List Sub Parts'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'List Sub Parts'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseListSubParts(ListSubParts object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Hash Sub Parts'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Hash Sub Parts'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseHashSubParts(HashSubParts object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Range Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Range Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRangeSubPart(RangeSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'List Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'List Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseListSubPart(ListSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Sub Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Sub Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseSubSubPart(SubSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Range Sub Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Range Sub Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRangeSubSubPart(RangeSubSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'List Sub Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'List Sub Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseListSubSubPart(ListSubSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Hash Sub Sub Part'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Hash Sub Sub Part'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseHashSubSubPart(HashSubSubPart object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Range Partition'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Range Partition'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRangePartition(RangePartition object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Range Partition Value'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Range Partition Value'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRangePartitionValue(RangePartitionValue object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Hash Partitions'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Hash Partitions'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseHashPartitions(HashPartitions object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Hash Partition'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Hash Partition'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseHashPartition(HashPartition object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Ref Partitions'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Ref Partitions'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRefPartitions(RefPartitions object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Ref Partition'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Ref Partition'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseRefPartition(RefPartition object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'List Partitions'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'List Partitions'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseListPartitions(ListPartitions object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'List Partition'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'List Partition'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseListPartition(ListPartition object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'List Partition Value'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'List Partition Value'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseListPartitionValue(ListPartitionValue object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Lob Storage'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Lob Storage'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseLobStorage(LobStorage object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Varray Storage'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Varray Storage'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseVarrayStorage(VarrayStorage object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Nested Table Storage'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Nested Table Storage'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseNestedTableStorage(NestedTableStorage object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Lob Storage Parameters'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Lob Storage Parameters'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseLobStorageParameters(LobStorageParameters object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Column'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Column'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseColumn(Column object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Constraint'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Constraint'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseConstraint(Constraint object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Index Or Unique Key'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Index Or Unique Key'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseIndexOrUniqueKey(IndexOrUniqueKey object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Foreign Key'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Foreign Key'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseForeignKey(ForeignKey object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Primary Key'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Primary Key'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T casePrimaryKey(PrimaryKey object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Unique Key'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Unique Key'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseUniqueKey(UniqueKey object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Index'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Index'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseIndex(Index object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Index Ex Table'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Index Ex Table'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseIndexExTable(IndexExTable object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Column Ref'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Column Ref'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseColumnRef(ColumnRef object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Sequence'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Sequence'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseSequence(Sequence object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Column Identity'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Column Identity'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseColumnIdentity(ColumnIdentity object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Mview'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Mview'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseMview(Mview object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Mview Log'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Mview Log'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseMviewLog(MviewLog object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Comment'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Comment'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseComment(Comment object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Inline Comment'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Inline Comment'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseInlineComment(InlineComment object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Domain Column'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Domain Column'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseDomainColumn(DomainColumn object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'History Table'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'History Table'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseHistoryTable(HistoryTable object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Domain'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Domain'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseDomain(Domain object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Gen Name Rule'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Gen Name Rule'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGenNameRule(GenNameRule object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Generate Pk'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Generate Pk'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGeneratePk(GeneratePk object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Generate Uk'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Generate Uk'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGenerateUk(GenerateUk object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Generate Index'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Generate Index'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGenerateIndex(GenerateIndex object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Generate Cc'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Generate Cc'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGenerateCc(GenerateCc object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Generate Fk'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Generate Fk'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGenerateFk(GenerateFk object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Column Domain'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Column Domain'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseColumnDomain(ColumnDomain object)
{
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EObject'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch, but this is the last case anyway.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'EObject'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject)
* @generated
*/
@Override
public T defaultCase(EObject object)
{
return null;
}
} //OrcasDslSwitch
© 2015 - 2025 Weber Informatics LLC | Privacy Policy