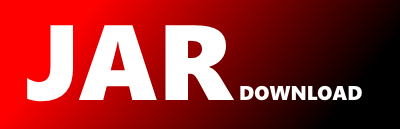
com.opsbears.webcomponents.servicepool.StartupService Maven / Gradle / Ivy
package com.opsbears.webcomponents.servicepool;
import javax.annotation.ParametersAreNonnullByDefault;
import java.util.Collection;
import java.util.Collections;
import java.util.concurrent.Future;
/**
* The startup service launches a task at startup and returns the healthy status once the task is completed.
*/
@ParametersAreNonnullByDefault
public class StartupService extends AbstractService {
public StartupService(
String name,
Runnable runnable,
StatusChangeListener statusChangeListener
) {
super(name, Collections.emptyList(), Collections.emptyList(), statusChangeListener, runnable);
}
public StartupService(
String name,
Runnable runnable,
StatusChangeListener statusChangeListener,
Collection needs,
Collection provides
) {
super(name, needs, provides, statusChangeListener, runnable);
}
@Override
public Future start() throws ServiceAlreadyRunningException {
synchronized (statusLock) {
if (status == Status.STARTING || status == Status.HEALTHY || status == Status.UNHEALTHY || status == Status.STOPPING) {
throw new ServiceAlreadyRunningException();
}
status = Status.STARTING;
statusChangeListener.onStatusChange(this, status);
Future> future = executor.submit(new RunnableProxy());
return new ProxyFuture(future, service, service::updateTerminatedStatus);
}
}
private class RunnableProxy implements Runnable {
@Override
public void run() {
try {
runnable.run();
synchronized (statusLock) {
if (status == Status.STARTING) {
status = Status.HEALTHY;
statusChangeListener.onStatusChange(service, status);
}
}
} catch (RuntimeException e) {
synchronized (statusLock) {
if (status == Status.STARTING) {
status = Status.CRASHED;
crashException = e;
statusChangeListener.onStatusChange(service, status);
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy