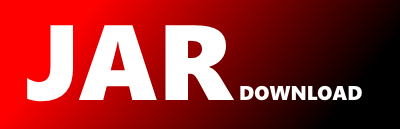
com.opsmatters.newrelic.batch.model.AlertConfiguration Maven / Gradle / Ivy
/*
* Copyright 2018 Gerald Curley
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.opsmatters.newrelic.batch.model;
import java.util.List;
import java.util.ArrayList;
import java.util.logging.Logger;
import com.opsmatters.newrelic.api.model.alerts.policies.AlertPolicy;
import com.opsmatters.newrelic.api.model.alerts.channels.AlertChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.EmailChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.SlackChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.HipChatChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.CampfireChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.OpsGenieChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.PagerDutyChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.UserChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.VictorOpsChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.WebhookChannel;
import com.opsmatters.newrelic.api.model.alerts.channels.xMattersChannel;
import com.opsmatters.newrelic.api.model.alerts.conditions.AlertCondition;
import com.opsmatters.newrelic.api.model.alerts.conditions.ExternalServiceAlertCondition;
import com.opsmatters.newrelic.api.model.alerts.conditions.NrqlAlertCondition;
import com.opsmatters.newrelic.api.model.alerts.conditions.InfraAlertCondition;
import com.opsmatters.newrelic.api.model.alerts.conditions.InfraMetricAlertCondition;
import com.opsmatters.newrelic.api.model.alerts.conditions.InfraProcessRunningAlertCondition;
import com.opsmatters.newrelic.api.model.alerts.conditions.InfraHostNotReportingAlertCondition;
/**
* Represents a set of alert policies, conditions and channels.
*
* @author Gerald Curley (opsmatters)
*/
public class AlertConfiguration
{
private static final Logger logger = Logger.getLogger(AlertConfiguration.class.getName());
private List policies = new ArrayList();
private List channels = new ArrayList();
private List alertConditions = new ArrayList();
private List externalServiceConditions = new ArrayList();
private List nrqlConditions = new ArrayList();
private List infraConditions = new ArrayList();
/**
* Default constructor.
*/
public AlertConfiguration()
{
}
/**
* Replaces the alert policies with the given set of policies.
* @param policies The set of policies
*/
public void setAlertPolicies(List policies)
{
this.policies.clear();
this.policies.addAll(policies);
}
/**
* Adds the given alert policies to the current set of policies.
* @param policies The policies to add
*/
public void addAlertPolicies(List policies)
{
this.policies.addAll(policies);
}
/**
* Returns the set of alert policies.
* @return The set of policies
*/
public List getAlertPolicies()
{
return policies;
}
/**
* Returns the number of alert policies.
* @return The number of alert policies
*/
public int numAlertPolicies()
{
return policies.size();
}
/**
* Replaces the alert channels with the given set of channels.
* @param channels The set of channels
*/
public void setAlertChannels(List extends AlertChannel> channels)
{
this.channels.clear();
this.channels.addAll(channels);
}
/**
* Adds the given alert channels to the current set of channels.
* @param channels The channels to add
*/
public void addAlertChannels(List extends AlertChannel> channels)
{
this.channels.addAll(channels);
}
/**
* Returns the set of alert channels.
* @return The set of channels
*/
public List getAlertChannels()
{
return channels;
}
/**
* Returns the set of email alert channels.
* @return The set of email channels
*/
public List getEmailChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof EmailChannel)
channels.add((EmailChannel)channel);
}
return channels;
}
/**
* Returns the set of Slack alert channels.
* @return The set of Slack channels
*/
public List getSlackChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof SlackChannel)
channels.add((SlackChannel)channel);
}
return channels;
}
/**
* Returns the set of HipChat alert channels.
* @return The set of HipChat channels
*/
public List getHipChatChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof HipChatChannel)
channels.add((HipChatChannel)channel);
}
return channels;
}
/**
* Returns the set of Campfire alert channels.
* @return The set of Campfire channels
*/
public List getCampfireChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof CampfireChannel)
channels.add((CampfireChannel)channel);
}
return channels;
}
/**
* Returns the set of OpsGenie alert channels.
* @return The set of OpsGenie channels
*/
public List getOpsGenieChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof OpsGenieChannel)
channels.add((OpsGenieChannel)channel);
}
return channels;
}
/**
* Returns the set of PagerDuty alert channels.
* @return The set of PagerDuty channels
*/
public List getPagerDutyChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof PagerDutyChannel)
channels.add((PagerDutyChannel)channel);
}
return channels;
}
/**
* Returns the set of user alert channels.
* @return The set of user channels
*/
public List getUserChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof UserChannel)
channels.add((UserChannel)channel);
}
return channels;
}
/**
* Returns the set of VictorOps alert channels.
* @return The set of VictorOps channels
*/
public List getVictorOpsChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof VictorOpsChannel)
channels.add((VictorOpsChannel)channel);
}
return channels;
}
/**
* Returns the set of webhook alert channels.
* @return The set of webhook channels
*/
public List getWebhookChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof WebhookChannel)
channels.add((WebhookChannel)channel);
}
return channels;
}
/**
* Returns the set of xMatters alert channels.
* @return The set of xMatters channels
*/
public List getxMattersChannels()
{
List channels = new ArrayList();
for(AlertChannel channel : this.channels)
{
if(channel instanceof xMattersChannel)
channels.add((xMattersChannel)channel);
}
return channels;
}
/**
* Returns the number of alert channels.
* @return The number of alert channels
*/
public int numAlertChannels()
{
return channels.size();
}
/**
* Replaces the alert conditions with the given set of alert conditions.
* @param alertConditions The set of alert conditions
*/
public void setAlertConditions(List alertConditions)
{
this.alertConditions.clear();
this.alertConditions.addAll(alertConditions);
}
/**
* Adds the given alert conditions to the current set of alert conditions.
* @param alertConditions The alert conditions to add
*/
public void addAlertConditions(List alertConditions)
{
this.alertConditions.addAll(alertConditions);
}
/**
* Returns the set of alert conditions.
* @return The set of alert conditions
*/
public List getAlertConditions()
{
return alertConditions;
}
/**
* Returns the number of alert conditions.
* @return The number of alert conditions
*/
public int numAlertConditions()
{
return alertConditions.size();
}
/**
* Replaces the external service alert conditions with the given set of alert conditions.
* @param externalServiceConditions The set of external service alert conditions
*/
public void setExternalServiceAlertConditions(List externalServiceConditions)
{
this.externalServiceConditions.clear();
this.externalServiceConditions.addAll(externalServiceConditions);
}
/**
* Adds the given external service alert conditions to the current set of conditions.
* @param externalServiceConditions The external service alert conditions to add
*/
public void addExternalServiceAlertConditions(List externalServiceConditions)
{
this.externalServiceConditions.addAll(externalServiceConditions);
}
/**
* Returns the set of external service alert conditions.
* @return The set of external service alert conditions
*/
public List getExternalServiceAlertConditions()
{
return externalServiceConditions;
}
/**
* Returns the number of external service alert conditions.
* @return The number of external service alert conditions
*/
public int numExternalServiceAlertConditions()
{
return externalServiceConditions.size();
}
/**
* Replaces the NRQL alert conditions with the given set of alert conditions.
* @param nrqlConditions The set of NRQL alert conditions
*/
public void setNrqlAlertConditions(List nrqlConditions)
{
this.nrqlConditions.clear();
this.nrqlConditions.addAll(nrqlConditions);
}
/**
* Adds the given NRQL alert conditions to the current set of conditions.
* @param nrqlConditions The NRQL alert conditions to add
*/
public void addNrqlAlertConditions(List nrqlConditions)
{
this.nrqlConditions.addAll(nrqlConditions);
}
/**
* Returns the set of NRQL alert conditions.
* @return The set of NRQL alert conditions
*/
public List getNrqlAlertConditions()
{
return nrqlConditions;
}
/**
* Returns the number of NRQL alert conditions.
* @return The number of NRQL alert conditions
*/
public int numNrqlAlertConditions()
{
return nrqlConditions.size();
}
/**
* Replaces the infrastructure alert conditions with the given set of alert conditions.
* @param infraConditions The set of infrastructure alert conditions
*/
public void setInfraAlertConditions(List extends InfraAlertCondition> infraConditions)
{
this.infraConditions.clear();
this.infraConditions.addAll(infraConditions);
}
/**
* Adds the given infrastructure alert conditions to the current set of conditions.
* @param infraConditions The infrastructure alert conditions to add
*/
public void addInfraAlertConditions(List extends InfraAlertCondition> infraConditions)
{
this.infraConditions.addAll(infraConditions);
}
/**
* Returns the set of infrastructure alert conditions.
* @return The set of infrastructure alert conditions
*/
public List getInfraAlertConditions()
{
return infraConditions;
}
/**
* Returns the set of infrastructure metric alert conditions.
* @return The set of infrastructure metric alert conditions
*/
public List getInfraMetricAlertConditions()
{
List conditions = new ArrayList();
for(InfraAlertCondition condition : this.infraConditions)
{
if(condition instanceof InfraMetricAlertCondition)
conditions.add((InfraMetricAlertCondition)condition);
}
return conditions;
}
/**
* Returns the set of infrastructure process alert conditions.
* @return The set of infrastructure process alert conditions
*/
public List getInfraProcessRunningAlertConditions()
{
List conditions = new ArrayList();
for(InfraAlertCondition condition : this.infraConditions)
{
if(condition instanceof InfraProcessRunningAlertCondition)
conditions.add((InfraProcessRunningAlertCondition)condition);
}
return conditions;
}
/**
* Returns the set of infrastructure host alert conditions.
* @return The set of infrastructure host alert conditions
*/
public List getInfraHostNotReportingAlertConditions()
{
List conditions = new ArrayList();
for(InfraAlertCondition condition : this.infraConditions)
{
if(condition instanceof InfraHostNotReportingAlertCondition)
conditions.add((InfraHostNotReportingAlertCondition)condition);
}
return conditions;
}
/**
* Returns the number of infrastructure alert conditions.
* @return The number of infrastructure alert conditions
*/
public int numInfraAlertConditions()
{
return infraConditions.size();
}
/**
* Returns a string representation of the object.
*/
@Override
public String toString()
{
return "AlertConfiguration [policies="+policies.size()
+", channels="+channels.size()
+", alertConditions="+alertConditions.size()
+", externalServiceConditions="+externalServiceConditions.size()
+", nrqlConditions="+nrqlConditions.size()
+", infraConditions="+infraConditions.size()
+"]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy