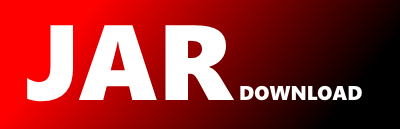
com.tangosol.coherence.jcache.passthroughcache.PassThroughCacheEntryEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of coherence-jcache Show documentation
Show all versions of coherence-jcache Show documentation
Oracle Coherence Community Edition
/*
* Copyright (c) 2000, 2020, Oracle and/or its affiliates.
*
* Licensed under the Universal Permissive License v 1.0 as shown at
* http://oss.oracle.com/licenses/upl.
*/
package com.tangosol.coherence.jcache.passthroughcache;
import com.tangosol.util.MapEvent;
import javax.cache.Cache;
import javax.cache.event.CacheEntryEvent;
import javax.cache.event.EventType;
/**
* An implementation of a {@link CacheEntryEvent} based on an underlying
* Coherence {@link MapEvent}.
*
* @param the type of the {@link Cache} keys
* @param the type of the {@link Cache} values
*
* @author bo 2013.11.01
* @since Coherence 12.1.3
*/
public class PassThroughCacheEntryEvent
extends CacheEntryEvent
{
// ----- constructors ---------------------------------------------------
/**
* Constructs a {@link PassThroughCacheEntryEvent}.
*
* @param cache the {@link Cache} on which the {@link CacheEntryEvent} occurred
* @param type the {@link EventType}
* @param event the underlying {@link MapEvent}
*/
public PassThroughCacheEntryEvent(Cache cache, EventType type, MapEvent event)
{
super(cache, type);
m_event = event;
}
// ----- CacheEntry methods ---------------------------------------------
@Override
public K getKey()
{
return (K) m_event.getKey();
}
@Override
public V getValue()
{
return (V) m_event.getNewValue();
}
@Override
public T unwrap(Class clz)
{
if (clz != null && clz.isInstance(m_event))
{
return (T) m_event;
}
else
{
throw new IllegalArgumentException("Unsupported unwrap(" + clz + ")");
}
}
// ----- CacheEntryEvent methods ----------------------------------------
@Override
public boolean isOldValueAvailable()
{
return m_event.getOldValue() != null;
}
@Override
public V getOldValue()
{
return (V) m_event.getOldValue();
}
// ----- PassThroughCacheEntryEvent methods -----------------------------
/**
* Creates a {@link PassThroughCacheEntryEvent} based on a {@link MapEvent}
*
* @param event the {@link MapEvent}
*
* @param the key type
* @param the value type
*
* @return a new {@link PassThroughCacheEntryEvent}
*/
public static PassThroughCacheEntryEvent from(MapEvent event)
{
// determine the type of the event
EventType type;
switch (event.getId())
{
case MapEvent.ENTRY_INSERTED:
type = EventType.CREATED;
break;
case MapEvent.ENTRY_UPDATED:
type = EventType.UPDATED;
break;
case MapEvent.ENTRY_DELETED:
type = EventType.REMOVED;
break;
default:
type = null;
}
// TODO: create a null implementation of the Cache based on the MapEvent
return new PassThroughCacheEntryEvent(null, type, event);
}
// ------ data members --------------------------------------------------
/**
* The underlying {@link MapEvent} to be adapted into a {@link CacheEntryEvent}.
*/
private MapEvent m_event;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy