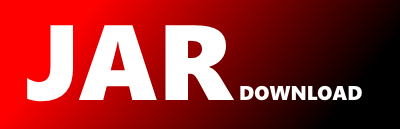
com.tangosol.dev.assembler.Constants Maven / Gradle / Ivy
/*
* Copyright (c) 2000, 2024, Oracle and/or its affiliates.
*
* Licensed under the Universal Permissive License v 1.0 as shown at
* https://oss.oracle.com/licenses/upl.
*/
package com.tangosol.dev.assembler;
/**
* Constants for the assembler package.
*/
public interface Constants
{
/**
* The default super class name.
*/
public static final String DEFAULT_SUPER = "java/lang/Object";
/**
* Value used internally by the assembler to denote an unknown value.
*/
public static final int UNKNOWN = Integer.MIN_VALUE;
// ----- access flags -----------------------------------------------
/**
* Interface (vs. Class).
*/
public static final int ACC_INTERFACE = 0x0200;
/**
* Accessibility: Public.
*/
public static final int ACC_PUBLIC = 0x0001;
/**
* Accessibility: Protected.
*/
public static final int ACC_PROTECTED = 0x0004;
/**
* Accessibility: Package Private.
* (The absence of ACC_PUBLIC, ACC_PROTECTED, and ACC_PRIVATE.)
*/
public static final int ACC_PACKAGE = 0x0000;
/**
* Accessibility: Private.
*/
public static final int ACC_PRIVATE = 0x0002;
/**
* Abstract (vs. concrete).
*/
public static final int ACC_ABSTRACT = 0x0400;
/**
* Declared synthetic; not present in source code.
*/
public static final int ACC_SYNTHETIC = 0x1000;
/**
* Declared as an annotation type.
*/
public static final int ACC_ANNOTATION = 0x2000;
/**
* Declared as an enum type.
*/
public static final int ACC_ENUM = 0x4000;
/**
* Static (vs. instance).
*/
public static final int ACC_STATIC = 0x0008;
/**
* Final (vs. derivable).
*/
public static final int ACC_FINAL = 0x0010;
/**
* Synchronized (also "ACC_SUPER" in later JVM specifications).
*/
public static final int ACC_SYNCHRONIZED = 0x0020;
/**
* Native (vs. pure).
*/
public static final int ACC_NATIVE = 0x0100;
/**
* Volatile.
*/
public static final int ACC_VOLATILE = 0x0040;
/**
* Transient (vs. persistent)
*/
public static final int ACC_TRANSIENT = 0x0080;
/**
* A bridge method, generated by the compiler.
*/
public static final int ACC_BRIDGE = 0x0040;
/**
* Declared with a variable number of arguments
*/
public static final int ACC_VARARGS = 0x0080;
/**
* Declared strictfp; floating-point mode is strict.
*/
public static final int ACC_STRICT = 0x0800;
/**
* The accessibility bits.
*/
public static final int ACC_ACCESS_MASK = ACC_PUBLIC |
ACC_PRIVATE |
ACC_PROTECTED;
/**
* All applicable bits.
*/
public static final int ACC_ALL = ACC_PUBLIC |
ACC_PRIVATE |
ACC_PROTECTED |
ACC_STATIC |
ACC_FINAL |
ACC_SYNCHRONIZED |
ACC_VOLATILE |
ACC_TRANSIENT |
ACC_NATIVE |
ACC_INTERFACE |
ACC_ABSTRACT;
// ----- java .class header ---------------------------------------------
/**
* The special sequence of bytes that identifies a .class structure.
*/
public static final int CLASS_COOKIE = 0xCAFEBABE;
/**
* The expected major version of the .class structure.
*/
public static final int VERSION_MAJOR = 45;
/**
* The expected minor version of the .class structure.
*/
public static final int VERSION_MINOR = 3;
/**
* The minimum supported major version of the class structure.
*/
public static final int VERSION_MAJOR_MIN = 45;
/**
* The minimum supported minor version of the class structure.
*/
public static final int VERSION_MINOR_MIN = 0;
/**
* The maximum supported major version of the class structure.
*/
public static final int VERSION_MAJOR_MAX = 52;
/**
* The maximum supported minor version of the class structure.
*/
public static final int VERSION_MINOR_MAX = 0;
// ----- java .class constant pool --------------------------------------
/**
* Constant pool: Class name.
*/
public static final int CONSTANT_CLASS = 7;
/**
* Constant pool: Specification for a field of an object/class/interface.
*/
public static final int CONSTANT_FIELDREF = 9;
/**
* Constant pool: Specification for a method invoked against a class
* type.
*/
public static final int CONSTANT_METHODREF = 10;
/**
* Constant pool: Specification for a method invoked against an interface
* type.
*/
public static final int CONSTANT_INTERFACEMETHODREF = 11;
/**
* Constant pool: A constant string referenced from Java byte-code
* (instructions LDC and LDC_W).
*/
public static final int CONSTANT_STRING = 8;
/**
* Constant pool: 4-byte integer.
*/
public static final int CONSTANT_INTEGER = 3;
/**
* Constant pool: 4-byte single-precision floating point number.
*/
public static final int CONSTANT_FLOAT = 4;
/**
* Constant pool: 8-byte integer.
*/
public static final int CONSTANT_LONG = 5;
/**
* Constant pool: 8-byte double-precision floating point number.
*/
public static final int CONSTANT_DOUBLE = 6;
/**
* Constant pool: Specify the name and type/signature of a field/method
* (used by *ref constant types).
*/
public static final int CONSTANT_NAMEANDTYPE = 12;
/**
* Constant pool: UTF-8 encoded string value.
*/
public static final int CONSTANT_UTF8 = 1;
/**
* Constant pool: A MethodHandle used to reference a field/method,
* commonly used by invokedynamic op.
*/
public static final int CONSTANT_METHODHANDLE = 15;
/**
* Constant pool: Provides a descriptor for a method used by
* BootstrapMethods.
*/
public static final int CONSTANT_METHODTYPE = 16;
/**
* Constant pool: Used by dynamic instruction.
*/
public static final int CONSTANT_DYNAMIC = 17;
/**
* Constant pool: Used by the invokedynamic instruction.
*/
public static final int CONSTANT_INVOKEDYNAMIC = 18;
/**
* Constant pool: Not legal in this version of the JVM.
*/
public static final int CONSTANT_UNICODE = 2;
/**
* The number of constant pool elements used by the constant, indexed by
* JVM constant classification tag.
*/
public static final int[] CONSTANT_SIZE = {0,1,0,1,1,2,2,1,1,1,1,1,1,0,0,1,1,1,1};
/**
* The desired order (in an assembled constant pool) of the constant
* types.
*/
public static final int[] CONSTANT_ORDER =
{
CONSTANT_INTEGER,
CONSTANT_LONG,
CONSTANT_FLOAT,
CONSTANT_DOUBLE,
CONSTANT_UTF8,
CONSTANT_STRING,
CONSTANT_CLASS,
CONSTANT_NAMEANDTYPE,
CONSTANT_FIELDREF,
CONSTANT_METHODREF,
CONSTANT_INTERFACEMETHODREF,
CONSTANT_METHODHANDLE,
CONSTANT_METHODTYPE,
CONSTANT_DYNAMIC,
CONSTANT_INVOKEDYNAMIC
};
// ----- java .class constants implied by byte codes --------------------
/**
* ICONST_M1 implies an IntConstant of -1.
*/
public static final IntConstant CONSTANT_ICONST_M1 = new IntConstant(-1);
/**
* ICONST_0 implies an IntConstant of 0.
*/
public static final IntConstant CONSTANT_ICONST_0 = new IntConstant(0);
/**
* ICONST_1 implies an IntConstant of 1.
*/
public static final IntConstant CONSTANT_ICONST_1 = new IntConstant(1);
/**
* ICONST_2 implies an IntConstant of 2.
*/
public static final IntConstant CONSTANT_ICONST_2 = new IntConstant(2);
/**
* ICONST_3 implies an IntConstant of 3.
*/
public static final IntConstant CONSTANT_ICONST_3 = new IntConstant(3);
/**
* ICONST_4 implies an IntConstant of 4.
*/
public static final IntConstant CONSTANT_ICONST_4 = new IntConstant(4);
/**
* ICONST_5 implies an IntConstant of 5.
*/
public static final IntConstant CONSTANT_ICONST_5 = new IntConstant(5);
/**
* LCONST_0 implies an LongConstant of 0.
*/
public static final LongConstant CONSTANT_LCONST_0 = new LongConstant(0L);
/**
* LCONST_1 implies an LongConstant of 1.
*/
public static final LongConstant CONSTANT_LCONST_1 = new LongConstant(1L);
/**
* FCONST_0 implies an FloatConstant of 0.
*/
public static final FloatConstant CONSTANT_FCONST_0 = new FloatConstant(0.0F);
/**
* FCONST_1 implies an FloatConstant of 1.
*/
public static final FloatConstant CONSTANT_FCONST_1 = new FloatConstant(1.0F);
/**
* FCONST_2 implies an FloatConstant of 2.
*/
public static final FloatConstant CONSTANT_FCONST_2 = new FloatConstant(2.0F);
/**
* DCONST_0 implies an DoubleConstant of 0.
*/
public static final DoubleConstant CONSTANT_DCONST_0 = new DoubleConstant(0.0);
/**
* DCONST_1 implies an DoubleConstant of 1.
*/
public static final DoubleConstant CONSTANT_DCONST_1 = new DoubleConstant(1.0);
// ----- java .class methods --------------------------------------------
/**
* JVM method name for a constructor.
*/
public static final String CONSTRUCTOR_NAME = "";
/**
* JVM method name for the static initializer.
*/
public static final String INITIALIZER_NAME = "";
/**
* JVM method signature for the static initializer.
*/
public static final String INITIALIZER_SIG = "()V";
// ----- java .class attributes -----------------------------------------
/**
* Attribute: Source file name.
*/
public static final String ATTR_FILENAME = "SourceFile";
/**
* Attribute: Deprecated Class/Field/Method.
*/
public static final String ATTR_DEPRECATED = "Deprecated";
/**
* Attribute: Synthetic Class/Field/Method.
*/
public static final String ATTR_SYNTHETIC = "Synthetic";
/**
* Attribute: Inner class information.
*/
public static final String ATTR_INNERCLASSES = "InnerClasses";
/**
* Attribute: Constant Field.
*/
public static final String ATTR_CONSTANT = "ConstantValue";
/**
* Attribute: Method declared Exceptions.
*/
public static final String ATTR_EXCEPTIONS = "Exceptions";
/**
* Attribute: Method byte code.
*/
public static final String ATTR_CODE = "Code";
/**
* Attribute: Byte-code pc to source-code line number table.
*/
public static final String ATTR_LINENUMBERS = "LineNumberTable";
/**
* Attribute: Byte-code variable index to source-code variable name
* table.
*/
public static final String ATTR_VARIABLES = "LocalVariableTable";
/**
* Attribute: Byte-code variable index to source-code variable type
*/
public static final String ATTR_VARIABLETYPES = "LocalVariableTypeTable";
/**
* Attribute: Additional type-signature information.
*/
public static final String ATTR_SIGNATURE = "Signature";
/**
* Attribute: Default value for a method annotation.
*/
public static final String ATTR_ANNOTDEFAULT = "AnnotationDefault";
/**
* Attribute: Enclosing method of a local or anonymous inner class.
*/
public static final String ATTR_ENCMETHOD = "EnclosingMethod";
/**
* Attribute: Runtime-visible class/field/method annotations.
*/
public static final String ATTR_RTVISANNOT = "RuntimeVisibleAnnotations";
/**
* Attribute: Runtime-invisible class/field/method annotations.
*/
public static final String ATTR_RTINVISANNOT = "RuntimeInvisibleAnnotations";
/**
* Attribute: Runtime-visible method parameter annotations.
*/
public static final String ATTR_RTVISPARAMANNOT = "RuntimeVisibleParameterAnnotations";
/**
* Attribute: Runtime-invisible method parameter annotations.
*/
public static final String ATTR_RTINVISPARAMANNOT = "RuntimeInvisibleParameterAnnotations";
/**
* Attribute: Type verification Code attribute: {@value}
*/
public static final String ATTR_STACKMAPTABLE = "StackMapTable";
/**
* Attribute: Bootstrap Method definitions used by invokedynamic instructions
* applicable to class attributes only.
*/
public static final String ATTR_BOOTSTRAPMETHODS = "BootstrapMethods";
/**
* Attribute: Runtime-visible-type class/field/method/code annotations.
*/
public static final String ATTR_RTVISTANNOT = "RuntimeVisibleTypeAnnotations";
/**
* Attribute: Runtime-invisible-type class/field/method/code annotations.
*/
public static final String ATTR_RTINVISTANNOT = "RuntimeInvisibleTypeAnnotations";
/**
* Attribute: Method Parameters applicable to method attributes.
*/
public static final String ATTR_METHODPARAMS = "MethodParameters";
// ----- .java byte codes -----------------------------------------------
/**
* Byte Codes (also JASM ops except when specified): Enumerated by value.
*/
public static final int NOP = 0x00;
public static final int ACONST_NULL = 0x01; // not JASM op
public static final int ICONST_M1 = 0x02; // not JASM op
public static final int ICONST_0 = 0x03; // not JASM op
public static final int ICONST_1 = 0x04; // not JASM op
public static final int ICONST_2 = 0x05; // not JASM op
public static final int ICONST_3 = 0x06; // not JASM op
public static final int ICONST_4 = 0x07; // not JASM op
public static final int ICONST_5 = 0x08; // not JASM op
public static final int LCONST_0 = 0x09; // not JASM op
public static final int LCONST_1 = 0x0a; // not JASM op
public static final int FCONST_0 = 0x0b; // not JASM op
public static final int FCONST_1 = 0x0c; // not JASM op
public static final int FCONST_2 = 0x0d; // not JASM op
public static final int DCONST_0 = 0x0e; // not JASM op
public static final int DCONST_1 = 0x0f; // not JASM op
public static final int BIPUSH = 0x10; // not JASM op
public static final int SIPUSH = 0x11; // not JASM op
public static final int LDC = 0x12;
public static final int LDC_W = 0x13; // not JASM op
public static final int LDC2_W = 0x14; // not JASM op
public static final int ILOAD = 0x15;
public static final int LLOAD = 0x16;
public static final int FLOAD = 0x17;
public static final int DLOAD = 0x18;
public static final int ALOAD = 0x19;
public static final int ILOAD_0 = 0x1a; // not JASM op
public static final int ILOAD_1 = 0x1b; // not JASM op
public static final int ILOAD_2 = 0x1c; // not JASM op
public static final int ILOAD_3 = 0x1d; // not JASM op
public static final int LLOAD_0 = 0x1e; // not JASM op
public static final int LLOAD_1 = 0x1f; // not JASM op
public static final int LLOAD_2 = 0x20; // not JASM op
public static final int LLOAD_3 = 0x21; // not JASM op
public static final int FLOAD_0 = 0x22; // not JASM op
public static final int FLOAD_1 = 0x23; // not JASM op
public static final int FLOAD_2 = 0x24; // not JASM op
public static final int FLOAD_3 = 0x25; // not JASM op
public static final int DLOAD_0 = 0x26; // not JASM op
public static final int DLOAD_1 = 0x27; // not JASM op
public static final int DLOAD_2 = 0x28; // not JASM op
public static final int DLOAD_3 = 0x29; // not JASM op
public static final int ALOAD_0 = 0x2a; // not JASM op
public static final int ALOAD_1 = 0x2b; // not JASM op
public static final int ALOAD_2 = 0x2c; // not JASM op
public static final int ALOAD_3 = 0x2d; // not JASM op
public static final int IALOAD = 0x2e;
public static final int LALOAD = 0x2f;
public static final int FALOAD = 0x30;
public static final int DALOAD = 0x31;
public static final int AALOAD = 0x32;
public static final int BALOAD = 0x33;
public static final int CALOAD = 0x34;
public static final int SALOAD = 0x35;
public static final int ISTORE = 0x36;
public static final int LSTORE = 0x37;
public static final int FSTORE = 0x38;
public static final int DSTORE = 0x39;
public static final int ASTORE = 0x3a;
public static final int ISTORE_0 = 0x3b; // not JASM op
public static final int ISTORE_1 = 0x3c; // not JASM op
public static final int ISTORE_2 = 0x3d; // not JASM op
public static final int ISTORE_3 = 0x3e; // not JASM op
public static final int LSTORE_0 = 0x3f; // not JASM op
public static final int LSTORE_1 = 0x40; // not JASM op
public static final int LSTORE_2 = 0x41; // not JASM op
public static final int LSTORE_3 = 0x42; // not JASM op
public static final int FSTORE_0 = 0x43; // not JASM op
public static final int FSTORE_1 = 0x44; // not JASM op
public static final int FSTORE_2 = 0x45; // not JASM op
public static final int FSTORE_3 = 0x46; // not JASM op
public static final int DSTORE_0 = 0x47; // not JASM op
public static final int DSTORE_1 = 0x48; // not JASM op
public static final int DSTORE_2 = 0x49; // not JASM op
public static final int DSTORE_3 = 0x4a; // not JASM op
public static final int ASTORE_0 = 0x4b; // not JASM op
public static final int ASTORE_1 = 0x4c; // not JASM op
public static final int ASTORE_2 = 0x4d; // not JASM op
public static final int ASTORE_3 = 0x4e; // not JASM op
public static final int IASTORE = 0x4f;
public static final int LASTORE = 0x50;
public static final int FASTORE = 0x51;
public static final int DASTORE = 0x52;
public static final int AASTORE = 0x53;
public static final int BASTORE = 0x54;
public static final int CASTORE = 0x55;
public static final int SASTORE = 0x56;
public static final int POP = 0x57;
public static final int POP2 = 0x58;
public static final int DUP = 0x59;
public static final int DUP_X1 = 0x5a;
public static final int DUP_X2 = 0x5b;
public static final int DUP2 = 0x5c;
public static final int DUP2_X1 = 0x5d;
public static final int DUP2_X2 = 0x5e;
public static final int SWAP = 0x5f;
public static final int IADD = 0x60;
public static final int LADD = 0x61;
public static final int FADD = 0x62;
public static final int DADD = 0x63;
public static final int ISUB = 0x64;
public static final int LSUB = 0x65;
public static final int FSUB = 0x66;
public static final int DSUB = 0x67;
public static final int IMUL = 0x68;
public static final int LMUL = 0x69;
public static final int FMUL = 0x6a;
public static final int DMUL = 0x6b;
public static final int IDIV = 0x6c;
public static final int LDIV = 0x6d;
public static final int FDIV = 0x6e;
public static final int DDIV = 0x6f;
public static final int IREM = 0x70;
public static final int LREM = 0x71;
public static final int FREM = 0x72;
public static final int DREM = 0x73;
public static final int INEG = 0x74;
public static final int LNEG = 0x75;
public static final int FNEG = 0x76;
public static final int DNEG = 0x77;
public static final int ISHL = 0x78;
public static final int LSHL = 0x79;
public static final int ISHR = 0x7a;
public static final int LSHR = 0x7b;
public static final int IUSHR = 0x7c;
public static final int LUSHR = 0x7d;
public static final int IAND = 0x7e;
public static final int LAND = 0x7f;
public static final int IOR = 0x80;
public static final int LOR = 0x81;
public static final int IXOR = 0x82;
public static final int LXOR = 0x83;
public static final int IINC = 0x84;
public static final int I2L = 0x85;
public static final int I2F = 0x86;
public static final int I2D = 0x87;
public static final int L2I = 0x88;
public static final int L2F = 0x89;
public static final int L2D = 0x8a;
public static final int F2I = 0x8b;
public static final int F2L = 0x8c;
public static final int F2D = 0x8d;
public static final int D2I = 0x8e;
public static final int D2L = 0x8f;
public static final int D2F = 0x90;
public static final int I2B = 0x91;
public static final int I2C = 0x92;
public static final int I2S = 0x93;
public static final int LCMP = 0x94;
public static final int FCMPL = 0x95;
public static final int FCMPG = 0x96;
public static final int DCMPL = 0x97;
public static final int DCMPG = 0x98;
public static final int IFEQ = 0x99;
public static final int IFNE = 0x9a;
public static final int IFLT = 0x9b;
public static final int IFGE = 0x9c;
public static final int IFGT = 0x9d;
public static final int IFLE = 0x9e;
public static final int IF_ICMPEQ = 0x9f;
public static final int IF_ICMPNE = 0xa0;
public static final int IF_ICMPLT = 0xa1;
public static final int IF_ICMPGE = 0xa2;
public static final int IF_ICMPGT = 0xa3;
public static final int IF_ICMPLE = 0xa4;
public static final int IF_ACMPEQ = 0xa5;
public static final int IF_ACMPNE = 0xa6;
public static final int GOTO = 0xa7;
public static final int JSR = 0xa8;
public static final int RET = 0xa9;
public static final int TABLESWITCH = 0xaa;
public static final int LOOKUPSWITCH = 0xab;
public static final int IRETURN = 0xac;
public static final int LRETURN = 0xad;
public static final int FRETURN = 0xae;
public static final int DRETURN = 0xaf;
public static final int ARETURN = 0xb0;
public static final int RETURN = 0xb1;
public static final int GETSTATIC = 0xb2;
public static final int PUTSTATIC = 0xb3;
public static final int GETFIELD = 0xb4;
public static final int PUTFIELD = 0xb5;
public static final int INVOKEVIRTUAL = 0xb6;
public static final int INVOKESPECIAL = 0xb7;
public static final int INVOKESTATIC = 0xb8;
public static final int INVOKEINTERFACE = 0xb9;
public static final int INVOKEDYNAMIC = 0xba;
public static final int NEW = 0xbb;
public static final int NEWARRAY = 0xbc; // not JASM op
public static final int ANEWARRAY = 0xbd;
public static final int ARRAYLENGTH = 0xbe;
public static final int ATHROW = 0xbf;
public static final int CHECKCAST = 0xc0;
public static final int INSTANCEOF = 0xc1;
public static final int MONITORENTER = 0xc2;
public static final int MONITOREXIT = 0xc3;
public static final int WIDE = 0xc4; // not JASM op
public static final int MULTIANEWARRAY = 0xc5;
public static final int IFNULL = 0xc6;
public static final int IFNONNULL = 0xc7;
public static final int GOTO_W = 0xc8; // not JASM op
public static final int JSR_W = 0xc9; // not JASM op
/**
* JASM Instruction: Push an integer (single word) constant.
*/
public static final int ICONST = 0xe5;
/**
* JASM Instruction: Push a long integer (double word) constant.
*/
public static final int LCONST = 0xe6;
/**
* JASM Instruction: Push a single-precision floating point (single
* word) constant.
*/
public static final int FCONST = 0xe7;
/**
* JASM Instruction: Push a double-precision floating point (double
* word) constant.
*/
public static final int DCONST = 0xe8;
/**
* JASM Instruction: Push a reference (single word) constant.
*/
public static final int ACONST = 0xe9;
/**
* JASM Instruction: Begin scope.
*/
public static final int BEGIN = 0xea;
/**
* JASM Instruction: Close scope.
*/
public static final int END = 0xeb;
/**
* JASM Instruction: Declare integer (single word) variable.
*/
public static final int IVAR = 0xec;
/**
* JASM Instruction: Declare long integer (double word) variable.
*/
public static final int LVAR = 0xed;
/**
* JASM Instruction: Declare single-precision floating point (single
* word) variable.
*/
public static final int FVAR = 0xee;
/**
* JASM Instruction: Declare double-precision floating point (double
* word) variable.
*/
public static final int DVAR = 0xef;
/**
* JASM Instruction: Declare reference (single word) variable.
*/
public static final int AVAR = 0xf0;
/**
* JASM Instruction: Return address (single word) variable.
*/
public static final int RVAR = 0xf1;
/**
* JASM Instruction: Store return address variable.
*/
public static final int RSTORE = 0xf2;
/**
* JASM Instruction: Allocate boolean array.
*/
public static final int ZNEWARRAY = 0xf3;
/**
* JASM Instruction: Allocate byte array.
*/
public static final int BNEWARRAY = 0xf4;
/**
* JASM Instruction: Allocate short array.
*/
public static final int CNEWARRAY = 0xf5;
/**
* JASM Instruction: Allocate char array.
*/
public static final int SNEWARRAY = 0xf6;
/**
* JASM Instruction: Allocate integer array.
*/
public static final int INEWARRAY = 0xf7;
/**
* JASM Instruction: Allocate long integer array.
*/
public static final int LNEWARRAY = 0xf8;
/**
* JASM Instruction: Allocate single-precision float array.
*/
public static final int FNEWARRAY = 0xf9;
/**
* JASM Instruction: Allocate double-precision float array.
*/
public static final int DNEWARRAY = 0xfa;
/**
* JASM Instruction: JumpTarget.
*/
public static final int LABEL = 0xfb;
/**
* JASM Instruction: Switch (assembles to either TABLESWITCH or
* LOOKUPSWITCH).
*/
public static final int SWITCH = 0xfc;
/**
* JASM Instruction: Switch case (for SWITCH, TABLESWITCH, and
* LOOKUPSWITCH).
*/
public static final int CASE = 0xfd;
/**
* JASM Instruction: Try.
*/
public static final int TRY = 0xfe;
/**
* JASM Instruction: Catch.
*/
public static final int CATCH = 0xff;
/**
* Byte Codes and JASM ops: Name by value. Null for non-existent.
*/
public static final String[] OPNAME =
{
/* [0x00] = */ "nop",
/* [0x01] = */ "aconst_null",
/* [0x02] = */ "iconst_m1",
/* [0x03] = */ "iconst_0",
/* [0x04] = */ "iconst_1",
/* [0x05] = */ "iconst_2",
/* [0x06] = */ "iconst_3",
/* [0x07] = */ "iconst_4",
/* [0x08] = */ "iconst_5",
/* [0x09] = */ "lconst_0",
/* [0x0a] = */ "lconst_1",
/* [0x0b] = */ "fconst_0",
/* [0x0c] = */ "fconst_1",
/* [0x0d] = */ "fconst_2",
/* [0x0e] = */ "dconst_0",
/* [0x0f] = */ "dconst_1",
/* [0x10] = */ "bipush",
/* [0x11] = */ "sipush",
/* [0x12] = */ "ldc",
/* [0x13] = */ "ldc_w",
/* [0x14] = */ "ldc2_w",
/* [0x15] = */ "iload",
/* [0x16] = */ "lload",
/* [0x17] = */ "fload",
/* [0x18] = */ "dload",
/* [0x19] = */ "aload",
/* [0x1a] = */ "iload_0",
/* [0x1b] = */ "iload_1",
/* [0x1c] = */ "iload_2",
/* [0x1d] = */ "iload_3",
/* [0x1e] = */ "lload_0",
/* [0x1f] = */ "lload_1",
/* [0x20] = */ "lload_2",
/* [0x21] = */ "lload_3",
/* [0x22] = */ "fload_0",
/* [0x23] = */ "fload_1",
/* [0x24] = */ "fload_2",
/* [0x25] = */ "fload_3",
/* [0x26] = */ "dload_0",
/* [0x27] = */ "dload_1",
/* [0x28] = */ "dload_2",
/* [0x29] = */ "dload_3",
/* [0x2a] = */ "aload_0",
/* [0x2b] = */ "aload_1",
/* [0x2c] = */ "aload_2",
/* [0x2d] = */ "aload_3",
/* [0x2e] = */ "iaload",
/* [0x2f] = */ "laload",
/* [0x30] = */ "faload",
/* [0x31] = */ "daload",
/* [0x32] = */ "aaload",
/* [0x33] = */ "baload",
/* [0x34] = */ "caload",
/* [0x35] = */ "saload",
/* [0x36] = */ "istore",
/* [0x37] = */ "lstore",
/* [0x38] = */ "fstore",
/* [0x39] = */ "dstore",
/* [0x3a] = */ "astore",
/* [0x3b] = */ "istore_0",
/* [0x3c] = */ "istore_1",
/* [0x3d] = */ "istore_2",
/* [0x3e] = */ "istore_3",
/* [0x3f] = */ "lstore_0",
/* [0x40] = */ "lstore_1",
/* [0x41] = */ "lstore_2",
/* [0x42] = */ "lstore_3",
/* [0x43] = */ "fstore_0",
/* [0x44] = */ "fstore_1",
/* [0x45] = */ "fstore_2",
/* [0x46] = */ "fstore_3",
/* [0x47] = */ "dstore_0",
/* [0x48] = */ "dstore_1",
/* [0x49] = */ "dstore_2",
/* [0x4a] = */ "dstore_3",
/* [0x4b] = */ "astore_0",
/* [0x4c] = */ "astore_1",
/* [0x4d] = */ "astore_2",
/* [0x4e] = */ "astore_3",
/* [0x4f] = */ "iastore",
/* [0x50] = */ "lastore",
/* [0x51] = */ "fastore",
/* [0x52] = */ "dastore",
/* [0x53] = */ "aastore",
/* [0x54] = */ "bastore",
/* [0x55] = */ "castore",
/* [0x56] = */ "sastore",
/* [0x57] = */ "pop",
/* [0x58] = */ "pop2",
/* [0x59] = */ "dup",
/* [0x5a] = */ "dup_x1",
/* [0x5b] = */ "dup_x2",
/* [0x5c] = */ "dup2",
/* [0x5d] = */ "dup2_x1",
/* [0x5e] = */ "dup2_x2",
/* [0x5f] = */ "swap",
/* [0x60] = */ "iadd",
/* [0x61] = */ "ladd",
/* [0x62] = */ "fadd",
/* [0x63] = */ "dadd",
/* [0x64] = */ "isub",
/* [0x65] = */ "lsub",
/* [0x66] = */ "fsub",
/* [0x67] = */ "dsub",
/* [0x68] = */ "imul",
/* [0x69] = */ "lmul",
/* [0x6a] = */ "fmul",
/* [0x6b] = */ "dmul",
/* [0x6c] = */ "idiv",
/* [0x6d] = */ "ldiv",
/* [0x6e] = */ "fdiv",
/* [0x6f] = */ "ddiv",
/* [0x70] = */ "irem",
/* [0x71] = */ "lrem",
/* [0x72] = */ "frem",
/* [0x73] = */ "drem",
/* [0x74] = */ "ineg",
/* [0x75] = */ "lneg",
/* [0x76] = */ "fneg",
/* [0x77] = */ "dneg",
/* [0x78] = */ "ishl",
/* [0x79] = */ "lshl",
/* [0x7a] = */ "ishr",
/* [0x7b] = */ "lshr",
/* [0x7c] = */ "iushr",
/* [0x7d] = */ "lushr",
/* [0x7e] = */ "iand",
/* [0x7f] = */ "land",
/* [0x80] = */ "ior",
/* [0x81] = */ "lor",
/* [0x82] = */ "ixor",
/* [0x83] = */ "lxor",
/* [0x84] = */ "iinc",
/* [0x85] = */ "i2l",
/* [0x86] = */ "i2f",
/* [0x87] = */ "i2d",
/* [0x88] = */ "l2i",
/* [0x89] = */ "l2f",
/* [0x8a] = */ "l2d",
/* [0x8b] = */ "f2i",
/* [0x8c] = */ "f2l",
/* [0x8d] = */ "f2d",
/* [0x8e] = */ "d2i",
/* [0x8f] = */ "d2l",
/* [0x90] = */ "d2f",
/* [0x91] = */ "i2b",
/* [0x92] = */ "i2c",
/* [0x93] = */ "i2s",
/* [0x94] = */ "lcmp",
/* [0x95] = */ "fcmpl",
/* [0x96] = */ "fcmpg",
/* [0x97] = */ "dcmpl",
/* [0x98] = */ "dcmpg",
/* [0x99] = */ "ifeq",
/* [0x9a] = */ "ifne",
/* [0x9b] = */ "iflt",
/* [0x9c] = */ "ifge",
/* [0x9d] = */ "ifgt",
/* [0x9e] = */ "ifle",
/* [0x9f] = */ "if_icmpeq",
/* [0xa0] = */ "if_icmpne",
/* [0xa1] = */ "if_icmplt",
/* [0xa2] = */ "if_icmpge",
/* [0xa3] = */ "if_icmpgt",
/* [0xa4] = */ "if_icmple",
/* [0xa5] = */ "if_acmpeq",
/* [0xa6] = */ "if_acmpne",
/* [0xa7] = */ "goto",
/* [0xa8] = */ "jsr",
/* [0xa9] = */ "ret",
/* [0xaa] = */ "tableswitch",
/* [0xab] = */ "lookupswitch",
/* [0xac] = */ "ireturn",
/* [0xad] = */ "lreturn",
/* [0xae] = */ "freturn",
/* [0xaf] = */ "dreturn",
/* [0xb0] = */ "areturn",
/* [0xb1] = */ "return",
/* [0xb2] = */ "getstatic",
/* [0xb3] = */ "putstatic",
/* [0xb4] = */ "getfield",
/* [0xb5] = */ "putfield",
/* [0xb6] = */ "invokevirtual",
/* [0xb7] = */ "invokespecial",
/* [0xb8] = */ "invokestatic",
/* [0xb9] = */ "invokeinterface",
/* [0xba] = */ "invokedynamic",
/* [0xbb] = */ "new",
/* [0xbc] = */ "newarray",
/* [0xbd] = */ "anewarray",
/* [0xbe] = */ "arraylength",
/* [0xbf] = */ "athrow",
/* [0xc0] = */ "checkcast",
/* [0xc1] = */ "instanceof",
/* [0xc2] = */ "monitorenter",
/* [0xc3] = */ "monitorexit",
/* [0xc4] = */ "wide",
/* [0xc5] = */ "multianewarray",
/* [0xc6] = */ "ifnull",
/* [0xc7] = */ "ifnonnull",
/* [0xc8] = */ "goto_w",
/* [0xc9] = */ "jsr_w",
/* [0xca] = */ null,
/* [0xcb] = */ null,
/* [0xcc] = */ null,
/* [0xcd] = */ null,
/* [0xce] = */ null,
/* [0xcf] = */ null,
/* [0xd0] = */ null,
/* [0xd1] = */ null,
/* [0xd2] = */ null,
/* [0xd3] = */ null,
/* [0xd4] = */ null,
/* [0xd5] = */ null,
/* [0xd6] = */ null,
/* [0xd7] = */ null,
/* [0xd8] = */ null,
/* [0xd9] = */ null,
/* [0xda] = */ null,
/* [0xdb] = */ null,
/* [0xdc] = */ null,
/* [0xdd] = */ null,
/* [0xde] = */ null,
/* [0xdf] = */ null,
/* [0xe0] = */ null,
/* [0xe1] = */ null,
/* [0xe2] = */ null,
/* [0xe3] = */ null,
/* [0xe4] = */ null,
/* [0xe5] = */ "iconst",
/* [0xe6] = */ "lconst",
/* [0xe7] = */ "fconst",
/* [0xe8] = */ "dconst",
/* [0xe9] = */ "aconst",
/* [0xea] = */ "begin",
/* [0xeb] = */ "end",
/* [0xec] = */ "ivar",
/* [0xed] = */ "lvar",
/* [0xee] = */ "fvar",
/* [0xef] = */ "dvar",
/* [0xf0] = */ "avar",
/* [0xf1] = */ "rvar",
/* [0xf2] = */ "rstore",
/* [0xf3] = */ "znewarray",
/* [0xf4] = */ "bnewarray",
/* [0xf5] = */ "cnewarray",
/* [0xf6] = */ "snewarray",
/* [0xf7] = */ "inewarray",
/* [0xf8] = */ "lnewarray",
/* [0xf9] = */ "fnewarray",
/* [0xfa] = */ "dnewarray",
/* [0xfb] = */ "label",
/* [0xfc] = */ "switch",
/* [0xfd] = */ "case",
/* [0xfe] = */ "try",
/* [0xff] = */ "catch"
};
/**
* Byte Codes and JASM ops: Effect on stack height by value.
*/
public static final int[] OPEFFECT =
{
/* [0x00] = */ 0, // nop
/* [0x01] = */ 1, // aconst_null
/* [0x02] = */ 1, // iconst_m1
/* [0x03] = */ 1, // iconst_0
/* [0x04] = */ 1, // iconst_1
/* [0x05] = */ 1, // iconst_2
/* [0x06] = */ 1, // iconst_3
/* [0x07] = */ 1, // iconst_4
/* [0x08] = */ 1, // iconst_5
/* [0x09] = */ 2, // lconst_0
/* [0x0a] = */ 2, // lconst_1
/* [0x0b] = */ 1, // fconst_0
/* [0x0c] = */ 1, // fconst_1
/* [0x0d] = */ 1, // fconst_2
/* [0x0e] = */ 2, // dconst_0
/* [0x0f] = */ 2, // dconst_1
/* [0x10] = */ 1, // bipush
/* [0x11] = */ 1, // sipush
/* [0x12] = */ 1, // ldc
/* [0x13] = */ 1, // ldc_w
/* [0x14] = */ 2, // ldc2_w
/* [0x15] = */ 1, // iload
/* [0x16] = */ 2, // lload
/* [0x17] = */ 1, // fload
/* [0x18] = */ 2, // dload
/* [0x19] = */ 1, // aload
/* [0x1a] = */ 1, // iload_0
/* [0x1b] = */ 1, // iload_1
/* [0x1c] = */ 1, // iload_2
/* [0x1d] = */ 1, // iload_3
/* [0x1e] = */ 2, // lload_0
/* [0x1f] = */ 2, // lload_1
/* [0x20] = */ 2, // lload_2
/* [0x21] = */ 2, // lload_3
/* [0x22] = */ 1, // fload_0
/* [0x23] = */ 1, // fload_1
/* [0x24] = */ 1, // fload_2
/* [0x25] = */ 1, // fload_3
/* [0x26] = */ 2, // dload_0
/* [0x27] = */ 2, // dload_1
/* [0x28] = */ 2, // dload_2
/* [0x29] = */ 2, // dload_3
/* [0x2a] = */ 1, // aload_0
/* [0x2b] = */ 1, // aload_1
/* [0x2c] = */ 1, // aload_2
/* [0x2d] = */ 1, // aload_3
/* [0x2e] = */ -1, // iaload
/* [0x2f] = */ 0, // laload
/* [0x30] = */ -1, // faload
/* [0x31] = */ 0, // daload
/* [0x32] = */ -1, // aaload
/* [0x33] = */ -1, // baload
/* [0x34] = */ -1, // caload
/* [0x35] = */ -1, // saload
/* [0x36] = */ -1, // istore
/* [0x37] = */ -2, // lstore
/* [0x38] = */ -1, // fstore
/* [0x39] = */ -2, // dstore
/* [0x3a] = */ -1, // astore
/* [0x3b] = */ -1, // istore_0
/* [0x3c] = */ -1, // istore_1
/* [0x3d] = */ -1, // istore_2
/* [0x3e] = */ -1, // istore_3
/* [0x3f] = */ -2, // lstore_0
/* [0x40] = */ -2, // lstore_1
/* [0x41] = */ -2, // lstore_2
/* [0x42] = */ -2, // lstore_3
/* [0x43] = */ -1, // fstore_0
/* [0x44] = */ -1, // fstore_1
/* [0x45] = */ -1, // fstore_2
/* [0x46] = */ -1, // fstore_3
/* [0x47] = */ -2, // dstore_0
/* [0x48] = */ -2, // dstore_1
/* [0x49] = */ -2, // dstore_2
/* [0x4a] = */ -2, // dstore_3
/* [0x4b] = */ -1, // astore_0
/* [0x4c] = */ -1, // astore_1
/* [0x4d] = */ -1, // astore_2
/* [0x4e] = */ -1, // astore_3
/* [0x4f] = */ -3, // iastore
/* [0x50] = */ -4, // lastore
/* [0x51] = */ -3, // fastore
/* [0x52] = */ -4, // dastore
/* [0x53] = */ -3, // aastore
/* [0x54] = */ -3, // bastore
/* [0x55] = */ -3, // castore
/* [0x56] = */ -3, // sastore
/* [0x57] = */ -1, // pop
/* [0x58] = */ -2, // pop2
/* [0x59] = */ 1, // dup
/* [0x5a] = */ 1, // dup_x1
/* [0x5b] = */ 1, // dup_x2
/* [0x5c] = */ 2, // dup2
/* [0x5d] = */ 2, // dup2_x1
/* [0x5e] = */ 2, // dup2_x2
/* [0x5f] = */ 0, // swap
/* [0x60] = */ -1, // iadd
/* [0x61] = */ -2, // ladd
/* [0x62] = */ -1, // fadd
/* [0x63] = */ -2, // dadd
/* [0x64] = */ -1, // isub
/* [0x65] = */ -2, // lsub
/* [0x66] = */ -1, // fsub
/* [0x67] = */ -2, // dsub
/* [0x68] = */ -1, // imul
/* [0x69] = */ -2, // lmul
/* [0x6a] = */ -1, // fmul
/* [0x6b] = */ -2, // dmul
/* [0x6c] = */ -1, // idiv
/* [0x6d] = */ -2, // ldiv
/* [0x6e] = */ -1, // fdiv
/* [0x6f] = */ -2, // ddiv
/* [0x70] = */ -1, // irem
/* [0x71] = */ -2, // lrem
/* [0x72] = */ -1, // frem
/* [0x73] = */ -2, // drem
/* [0x74] = */ 0, // ineg
/* [0x75] = */ 0, // lneg
/* [0x76] = */ 0, // fneg
/* [0x77] = */ 0, // dneg
/* [0x78] = */ -1, // ishl
/* [0x79] = */ -1, // lshl
/* [0x7a] = */ -1, // ishr
/* [0x7b] = */ -1, // lshr
/* [0x7c] = */ -1, // iushr
/* [0x7d] = */ -1, // lushr
/* [0x7e] = */ -1, // iand
/* [0x7f] = */ -2, // land
/* [0x80] = */ -1, // ior
/* [0x81] = */ -2, // lor
/* [0x82] = */ -1, // ixor
/* [0x83] = */ -2, // lxor
/* [0x84] = */ 0, // iinc
/* [0x85] = */ 1, // i2l
/* [0x86] = */ 0, // i2f
/* [0x87] = */ 1, // i2d
/* [0x88] = */ -1, // l2i
/* [0x89] = */ -1, // l2f
/* [0x8a] = */ 0, // l2d
/* [0x8b] = */ 0, // f2i
/* [0x8c] = */ 1, // f2l
/* [0x8d] = */ 1, // f2d
/* [0x8e] = */ -1, // d2i
/* [0x8f] = */ 0, // d2l
/* [0x90] = */ -1, // d2f
/* [0x91] = */ 0, // i2b
/* [0x92] = */ 0, // i2c
/* [0x93] = */ 0, // i2s
/* [0x94] = */ -3, // lcmp
/* [0x95] = */ -1, // fcmpl
/* [0x96] = */ -1, // fcmpg
/* [0x97] = */ -3, // dcmpl
/* [0x98] = */ -3, // dcmpg
/* [0x99] = */ -1, // ifeq
/* [0x9a] = */ -1, // ifne
/* [0x9b] = */ -1, // iflt
/* [0x9c] = */ -1, // ifge
/* [0x9d] = */ -1, // ifgt
/* [0x9e] = */ -1, // ifle
/* [0x9f] = */ -2, // if_icmpeq
/* [0xa0] = */ -2, // if_icmpne
/* [0xa1] = */ -2, // if_icmplt
/* [0xa2] = */ -2, // if_icmpge
/* [0xa3] = */ -2, // if_icmpgt
/* [0xa4] = */ -2, // if_icmple
/* [0xa5] = */ -2, // if_acmpeq
/* [0xa6] = */ -2, // if_acmpne
/* [0xa7] = */ 0, // goto
/* [0xa8] = */ 0, // jsr
/* [0xa9] = */ 0, // ret
/* [0xaa] = */ -1, // tableswitch
/* [0xab] = */ -1, // lookupswitch
/* [0xac] = */ -1, // ireturn
/* [0xad] = */ -2, // lreturn
/* [0xae] = */ -1, // freturn
/* [0xaf] = */ -2, // dreturn
/* [0xb0] = */ -1, // areturn
/* [0xb1] = */ 0, // return
/* [0xb2] = */ 0, // getstatic (calculated)
/* [0xb3] = */ 0, // putstatic (calculated)
/* [0xb4] = */ 0, // getfield (calculated)
/* [0xb5] = */ 0, // putfield (calculated)
/* [0xb6] = */ 0, // invokevirtual (calculated)
/* [0xb7] = */ 0, // invokespecial (calculated)
/* [0xb8] = */ 0, // invokestatic (calculated)
/* [0xb9] = */ 0, // invokeinterface (calculated)
/* [0xba] = */ 0, // invokedynamic (calculated)
/* [0xbb] = */ 1, // new
/* [0xbc] = */ 0, // newarray
/* [0xbd] = */ 0, // anewarray
/* [0xbe] = */ 0, // arraylength
/* [0xbf] = */ -1, // athrow
/* [0xc0] = */ 0, // checkcast
/* [0xc1] = */ 0, // instanceof
/* [0xc2] = */ -1, // monitorenter
/* [0xc3] = */ -1, // monitorexit
/* [0xc4] = */ 0, // wide (not supported)
/* [0xc5] = */ 0, // multianewarray (calculated)
/* [0xc6] = */ -1, // ifnull
/* [0xc7] = */ -1, // ifnonnull
/* [0xc8] = */ 0, // goto_w
/* [0xc9] = */ 0, // jsr_w
/* [0xca] = */ 0,
/* [0xcb] = */ 0,
/* [0xcc] = */ 0,
/* [0xcd] = */ 0,
/* [0xce] = */ 0,
/* [0xcf] = */ 0,
/* [0xd0] = */ 0,
/* [0xd1] = */ 0,
/* [0xd2] = */ 0,
/* [0xd3] = */ 0,
/* [0xd4] = */ 0,
/* [0xd5] = */ 0,
/* [0xd6] = */ 0,
/* [0xd7] = */ 0,
/* [0xd8] = */ 0,
/* [0xd9] = */ 0,
/* [0xda] = */ 0,
/* [0xdb] = */ 0,
/* [0xdc] = */ 0,
/* [0xdd] = */ 0,
/* [0xde] = */ 0,
/* [0xdf] = */ 0,
/* [0xe0] = */ 0,
/* [0xe1] = */ 0,
/* [0xe2] = */ 0,
/* [0xe3] = */ 0,
/* [0xe4] = */ 0,
/* [0xe5] = */ 1, // iconst
/* [0xe6] = */ 2, // lconst
/* [0xe7] = */ 1, // fconst
/* [0xe8] = */ 2, // dconst
/* [0xe9] = */ 1, // aconst
/* [0xea] = */ 0, // begin
/* [0xeb] = */ 0, // end
/* [0xec] = */ 0, // ivar
/* [0xed] = */ 0, // lvar
/* [0xee] = */ 0, // fvar
/* [0xef] = */ 0, // dvar
/* [0xf0] = */ 0, // avar
/* [0xf1] = */ 0, // rvar
/* [0xf2] = */ -1, // rstore
/* [0xf3] = */ 0, // znewarray
/* [0xf4] = */ 0, // bnewarray
/* [0xf5] = */ 0, // cnewarray
/* [0xf6] = */ 0, // snewarray
/* [0xf7] = */ 0, // inewarray
/* [0xf8] = */ 0, // lnewarray
/* [0xf9] = */ 0, // fnewarray
/* [0xfa] = */ 0, // dnewarray
/* [0xfb] = */ 0, // label
/* [0xfc] = */ -1, // switch
/* [0xfd] = */ 0, // case
/* [0xfe] = */ 0, // try
/* [0xff] = */ 0, // catch (calculated)
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy