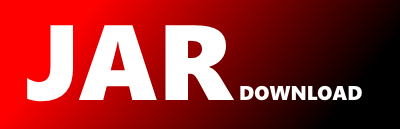
com.tangosol.dev.compiler.java.EmptyStatement Maven / Gradle / Ivy
/*
* Copyright (c) 2000, 2020, Oracle and/or its affiliates.
*
* Licensed under the Universal Permissive License v 1.0 as shown at
* http://oss.oracle.com/licenses/upl.
*/
package com.tangosol.dev.compiler.java;
import com.tangosol.dev.assembler.CodeAttribute;
import com.tangosol.dev.assembler.ClassConstant;
import com.tangosol.dev.compiler.CompilerException;
import com.tangosol.dev.compiler.Context;
import com.tangosol.dev.component.DataType;
import com.tangosol.util.ErrorList;
import java.util.Set;
import java.util.Map;
/**
* This class implements an empty Java statement.
*
* EmptyStatement:
* ;
*
* @version 1.00, 09/15/98
* @author Cameron Purdy
*/
public class EmptyStatement extends Statement
{
// ----- construction ---------------------------------------------------
/**
* Construct an empty statement.
*
* @param stmt the statement within which this element exists
* @param token the semi-colon (empty statement)
*/
public EmptyStatement(Statement stmt, Token token)
{
super(stmt, token);
}
// ----- code generation ------------------------------------------------
/**
* Perform semantic checks, parse tree re-organization, name binding,
* and optimizations.
*
* @param ctx the compiler context
* @param setUVars the set of potentially unassigned variables
* @param setFVars the set of potentially assigned final variables
* @param mapThrown the set of potentially thrown checked exceptions
* @param errlist the error list
*
* @exception CompilerException thrown if an error occurs that should
* stop the compilation process
*/
protected Element precompile(Context ctx, DualSet setUVars, DualSet setFVars, Map mapThrown, ErrorList errlist)
throws CompilerException
{
// JLS 16.2.1: V is definitely assigned after an empty statement
// iff it is definitely assigned before the empty statement.
return this;
}
/**
* Perform final optimizations and code generation.
*
* @param ctx the compiler context
* @param code the assembler code attribute to compile to
* @param fReached true if this language element is reached (JLS 14.19)
* @param errlist the error list to log errors to
*
* @return true if the element can complete normally (JLS 14.1)
*
* @exception CompilerException thrown if an error occurs that should
* stop the compilation process
*/
protected boolean compileImpl(Context ctx, CodeAttribute code, boolean fReached, ErrorList errlist)
throws CompilerException
{
// JLS 14.19: An empty statement can complete normally iff it is
// reachable.
return fReached;
}
// ----- data members ---------------------------------------------------
/**
* The class name.
*/
private static final String CLASS = "EmptyStatement";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy