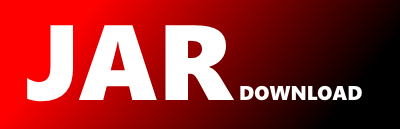
com.tangosol.coherence.http.AbstractHttpServer Maven / Gradle / Ivy
Show all versions of coherence Show documentation
/*
* Copyright (c) 2000, 2022, Oracle and/or its affiliates.
*
* Licensed under the Universal Permissive License v 1.0 as shown at
* https://oss.oracle.com/licenses/upl.
*/
package com.tangosol.coherence.http;
import com.tangosol.net.Service;
import com.tangosol.net.Session;
import java.io.IOException;
import java.security.Principal;
import java.security.PrivilegedActionException;
import java.security.PrivilegedExceptionAction;
import javax.security.auth.Subject;
import jakarta.ws.rs.core.SecurityContext;
import org.glassfish.hk2.api.DynamicConfiguration;
import org.glassfish.hk2.api.DynamicConfigurationService;
import org.glassfish.hk2.api.ServiceLocator;
import org.glassfish.hk2.api.ServiceLocatorFactory;
import org.glassfish.hk2.utilities.BuilderHelper;
import org.glassfish.jersey.server.ApplicationHandler;
import org.glassfish.jersey.server.ContainerRequest;
import org.glassfish.jersey.server.ResourceConfig;
/**
* Abstract base class for {@link HttpServer} implementations.
*
* This class and its sub-classes use Jersey ResourceConfig instances to implement
* the actual http endpoints to be served.
*
* @author as 2011.06.16
*/
public abstract class AbstractHttpServer
extends AbstractGenericHttpServer
implements HttpServer
{
// ----- AbstractHttpServer methods -------------------------------------
/**
* Factory method for Jersey container instances.
*
* @param config the resource configuration
* @param locator the parent service locator
*
* @return container instance
*/
protected abstract Object instantiateContainer(ResourceConfig config, ServiceLocator locator);
// ----- helpers --------------------------------------------------------
@Override
protected Object createContainer(ResourceConfig resourceConfig)
{
ServiceLocatorFactory factory = ServiceLocatorFactory.getInstance();
ServiceLocator locator = factory.create(getClass().getName());
DynamicConfiguration config = locator
.getService(DynamicConfigurationService.class)
.createDynamicConfiguration();
if (getParentService() != null)
{
config.bind(BuilderHelper.createConstantDescriptor(getParentService(), null, Service.class));
}
config.bind(BuilderHelper.createConstantDescriptor(getSession(), null, Session.class));
config.commit();
return instantiateContainer(resourceConfig, locator);
}
/**
* Handle HTTP(S) request.
*
* @param app web application that should handle request
* @param request the request
* @param subject the subject, can be null
*
* @throws IOException if an error occurs
*/
protected void handleRequest(final ApplicationHandler app,
final ContainerRequest request,
final Subject subject)
throws IOException
{
if (subject == null)
{
app.handle(request);
}
else
{
try
{
Subject.doAs(subject, new PrivilegedExceptionAction