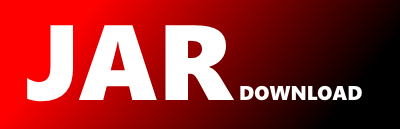
oracle.kv.impl.api.ops.GetIdentityAttrsAndValues Maven / Gradle / Ivy
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.impl.api.ops;
import static oracle.kv.impl.util.SerializationUtil.readString;
import static oracle.kv.impl.util.SerializationUtil.writeString;
import java.io.DataInput;
import java.io.DataOutput;
import java.io.IOException;
import oracle.kv.impl.util.SerialVersion;
import oracle.kv.impl.util.SerializationUtil;
/**
* A GetIdentityAttrsAndValues operation gets the attributes and new values of
* an identity column if needed.
*
* @see #writeFastExternal FastExternalizable format
*/
public class GetIdentityAttrsAndValues extends SingleKeyOperation {
final private long curVersion;
final private int clientIdentityCacheSize;
final private boolean needAttributes;
final private boolean needNextSequence;
final private String sgName;
/**
* Constructs a GetIdentityAttrsAndValues operation with a table id.
*/
public GetIdentityAttrsAndValues(byte[] keyBytes,
long curVersion,
int clientIdentityCacheSize,
boolean needAttributes,
boolean needNextSequence,
String sgName
) {
super(OpCode.GET_IDENTITY, keyBytes);
this.curVersion = curVersion;
this.clientIdentityCacheSize = clientIdentityCacheSize;
this.needAttributes = needAttributes;
this.needNextSequence = needNextSequence;
this.sgName = sgName;
}
GetIdentityAttrsAndValues(DataInput in, short serialVersion)
throws IOException {
super(OpCode.GET_IDENTITY, in, serialVersion);
this.curVersion = in.readLong();
this.clientIdentityCacheSize = in.readInt();
this.needAttributes = in.readBoolean();
this.needNextSequence = in.readBoolean();
this.sgName = readString(in, serialVersion);
}
/**
* Writes this object to the output stream. Format for {@code
* serialVersion} {@link SerialVersion#STD_UTF8_VERSION} or greater:
*
* - ({@link SingleKeyOperation}) {@code super}
*
- ({@link DataOutput#writeLong long}) {@link #getCurVersion
* curVersion}
*
- ({@link DataOutput#writeInt int}) {@link #getClientCacheSize
* clientIdentityCache}
*
- ({@link DataOutput#writeBoolean boolean}) {@link #getNeedAttributes
* needAttributes}
*
- ({@link DataOutput#writeBoolean boolean})
* {@link #getNeedNextSequence needNextSequence}
*
- ({@link DataOutput#writeLong long}) {@link #getTableId
* sysTableId}
*
- ({@link SerializationUtil#writeString String}) {@link #getSgName
* sgName}
*
*/
@Override
public void writeFastExternal(DataOutput out, short serialVersion)
throws IOException {
super.writeFastExternal(out, serialVersion);
out.writeLong(curVersion);
out.writeInt(clientIdentityCacheSize);
out.writeBoolean(needAttributes);
out.writeBoolean(needNextSequence);
writeString(out, serialVersion, sgName);
}
public long getCurVersion() {
return curVersion;
}
public int getClientCacheSize() {
return clientIdentityCacheSize;
}
public boolean getNeedAttributes() {
return needAttributes;
}
public boolean getNeedNextSequence() {
return needNextSequence;
}
public String getSgName() {
return sgName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy