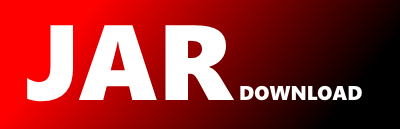
oracle.kv.impl.api.ops.PutBatch Maven / Gradle / Ivy
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.impl.api.ops;
import static oracle.kv.impl.util.ObjectUtil.checkNull;
import static oracle.kv.impl.util.SerialVersion.PUT_BATCH_OVERWRITE;
import static oracle.kv.impl.util.SerialVersion.STD_UTF8_VERSION;
import static oracle.kv.impl.util.SerializationUtil.readNonNullSequenceLength;
import static oracle.kv.impl.util.SerializationUtil.readSequenceLength;
import static oracle.kv.impl.util.SerializationUtil.writeArrayLength;
import static oracle.kv.impl.util.SerializationUtil.writeNonNullCollection;
import java.io.DataInput;
import java.io.DataOutput;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import oracle.kv.impl.api.bulk.BulkPut.KVPair;
import oracle.kv.impl.util.SerialVersion;
import oracle.kv.impl.util.SerializationUtil;
/**
* A put-batch operation.
*
* @see #writeFastExternal FastExternalizable format
*/
public class PutBatch extends MultiKeyOperation {
private final List kvPairs;
private final long[] tableIds;
private final boolean overwrite;
public PutBatch(List le, long[] tableIds, boolean overwrite) {
super(OpCode.PUT_BATCH, null, null, null);
checkNull("le", le);
for (final KVPair element : le) {
checkNull("le element", element);
}
this.kvPairs = le;
this.tableIds = tableIds;
this.overwrite = overwrite;
}
PutBatch(DataInput in, short serialVersion) throws IOException {
super(OpCode.PUT_BATCH, in, serialVersion);
final int kvPairCount = (serialVersion >= STD_UTF8_VERSION) ?
readNonNullSequenceLength(in) :
in.readInt();
kvPairs = new ArrayList(kvPairCount);
for (int i = 0; i < kvPairCount; i++) {
kvPairs.add(new KVPair(in, serialVersion));
}
final int tableIdCount = (serialVersion >= STD_UTF8_VERSION) ?
readSequenceLength(in) :
in.readInt();
if (tableIdCount == -1) {
tableIds = null;
} else {
tableIds = new long[tableIdCount];
for (int i = 0; i < tableIdCount; i++) {
tableIds[i] = in.readLong();
}
}
if (serialVersion >= PUT_BATCH_OVERWRITE) {
overwrite = in.readBoolean();
} else {
overwrite = false;
}
}
/**
* Writes this object to the output stream. Format for {@code
* serialVersion} {@link SerialVersion#STD_UTF8_VERSION} and greater:
*
* - ({@link MultiKeyOperation} {@code super}
*
- ({@link SerializationUtil#writeNonNullCollection non-null
* collection}) {@link #getKvPairs kvPairs}
*
- ({@link SerializationUtil#writeArrayLength sequence length})
* number of table IDs
*
- [Optional] ({@link DataOutput#writeLong long}{@code []})
* table IDs
*
*/
@Override
public void writeFastExternal(DataOutput out, short serialVersion)
throws IOException {
super.writeFastExternal(out, serialVersion);
if (serialVersion >= STD_UTF8_VERSION) {
writeNonNullCollection(out, serialVersion, kvPairs);
} else {
out.writeInt(kvPairs.size());
for (KVPair e : kvPairs) {
e.writeFastExternal(out, serialVersion);
}
}
if (serialVersion >= STD_UTF8_VERSION) {
writeArrayLength(out, tableIds);
} else {
out.writeInt((tableIds != null) ? tableIds.length : -1);
}
if (tableIds != null) {
for (final long tableId : tableIds) {
out.writeLong(tableId);
}
}
if (serialVersion >= PUT_BATCH_OVERWRITE) {
out.writeBoolean(overwrite);
}
}
List getKvPairs() {
return kvPairs;
}
@Override
public long[] getTableIds() {
return tableIds;
}
@Override
public boolean performsWrite() {
return true;
}
boolean getOverwrite() {
return overwrite;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy