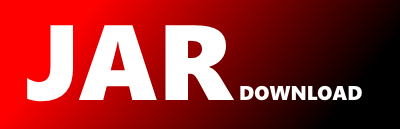
oracle.kv.impl.api.ops.PutHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oracle-nosql-server Show documentation
Show all versions of oracle-nosql-server Show documentation
NoSQL Database Server - supplies build and runtime support for the server (store) side of the Oracle NoSQL Database.
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.impl.api.ops;
import static com.sleepycat.je.Put.CURRENT;
import static com.sleepycat.je.Put.NO_OVERWRITE;
import static oracle.kv.impl.api.ops.OperationHandler.CURSOR_DEFAULT;
import com.sleepycat.je.Cursor;
import com.sleepycat.je.Database;
import com.sleepycat.je.DatabaseEntry;
import com.sleepycat.je.Get;
import com.sleepycat.je.LockMode;
import com.sleepycat.je.OperationResult;
import com.sleepycat.je.Transaction;
import com.sleepycat.je.WriteOptions;
import oracle.kv.ReturnValueVersion.Choice;
import oracle.kv.Version;
import oracle.kv.impl.api.ops.InternalOperation.OpCode;
import oracle.kv.impl.rep.migration.MigrationStreamHandle;
import oracle.kv.impl.topo.PartitionId;
import oracle.kv.impl.util.TxnUtil;
/**
* Server handler for {@link Put}.
*
* Throughput calculation
* +---------------------------------------------------------------------------+
* | Op | Choice | # | Read | Write |
* |---------------+--------+---+----------------------+-----------------------|
* | | | P | 0 | new record size + |
* | | | | | old record size |
* | | NONE |---+----------------------+-----------------------|
* | | | A | 0 | new record size |
* | +--------+---+----------------------+-----------------------|
* | | | P | MIN_READ | new record size + |
* | | | | | old record size |
* | Put | VERSION|---+----------------------+-----------------------|
* | | | A | 0 | new record size |
* | +--------+---+----------------------+-----------------------|
* | | | P | old record size | new record size + |
* | | | | | old record size |
* | | VALUE |---+----------------------+-----------------------|
* | | | A | 0 | new record size |
* +---------------------------------------------------------------------------+
* # = Target record is present (P) or absent (A)
*/
public class PutHandler extends BasicPutHandler {
public PutHandler(OperationHandler handler) {
super(handler, OpCode.PUT, Put.class);
}
@Override
public Result execute(Put op, Transaction txn, PartitionId partitionId) {
verifyDataAccess(op);
ResultValueVersion prevVal = null;
long expTime = 0L;
Version version = null;
boolean wasUpdate;
byte[] keyBytes = op.getKeyBytes();
byte[] valueBytes = op.getValueBytes();
assert (keyBytes != null) && (valueBytes != null);
final DatabaseEntry keyEntry = new DatabaseEntry(keyBytes);
final DatabaseEntry dataEntry = valueDatabaseEntry(valueBytes);
OperationResult opres;
WriteOptions jeOptions = makeOption(op.getTTL(), op.getUpdateTTL());
final Database db = getRepNode().getPartitionDB(partitionId);
/*
* We first try to do a put(NO_OVERWITE). If this succeeds (i.e. the
* row did not exist already), we are done. Otherwise, we must search
* for the record again and then do a put(CURRENT). This is because
* (a) the put(NO_OVERWITE) will not keep the cursor positioned on the
* existing row and (b) we cannot just do put(OVERWRITE) because we
* need before-update info (at the minumum the size of the record
* before the update).
*/
final Cursor cursor = db.openCursor(txn, CURSOR_DEFAULT);
try {
while (true) {
opres = putEntry(cursor, keyEntry, dataEntry,
NO_OVERWRITE, jeOptions);
if (opres != null) {
op.addWriteBytes(getStorageSize(cursor),
getNIndexWrites(cursor));
version = getVersion(cursor);
expTime = opres.getExpirationTime();
wasUpdate = false;
} else {
final Choice choice = op.getReturnValueVersionChoice();
final DatabaseEntry prevData =
(choice.needValue() ? new DatabaseEntry() : NO_DATA);
opres = cursor.get(keyEntry, prevData, Get.SEARCH,
LockMode.RMW.toReadOptions());
if (opres == null) {
/* Another thread deleted the record. Continue. */
continue;
}
prevVal =
getBeforeUpdateInfo(choice, cursor, prevData, opres);
final int oldRecordSize = getStorageSize(cursor);
/* Charge for the above search */
if (choice.needValue()) {
op.addReadBytes(oldRecordSize);
} else if (choice.needVersion()) {
op.addReadBytes(MIN_READ);
}
/* Charge for the deletion of the old record */
op.addWriteBytes(oldRecordSize, 0);
/* Do the update */
opres = putEntry(cursor, null, dataEntry, CURRENT, jeOptions);
/* Charge for the above update */
op.addWriteBytes(getStorageSize(cursor),
getNIndexWrites(cursor));
expTime = opres.getExpirationTime();
version = getVersion(cursor);
wasUpdate = true;
reserializeResultValue(op, prevVal);
}
MigrationStreamHandle.get().addPut(keyEntry,
dataEntry,
version.getVLSN(),
expTime);
return new Result.PutResult(getOpCode(),
op.getReadKB(),
op.getWriteKB(),
prevVal,
version,
expTime,
wasUpdate);
}
} finally {
TxnUtil.close(cursor);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy