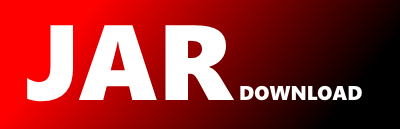
oracle.kv.impl.util.sklogger.CustomGauge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oracle-nosql-server Show documentation
Show all versions of oracle-nosql-server Show documentation
NoSQL Database Server - supplies build and runtime support for the server (store) side of the Oracle NoSQL Database.
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.impl.util.sklogger;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
/**
* A CustomGauge as one kind of {@link Metric} is useful when Gauge value is
* not possible to directly instrument code, as it is not in your control, such
* as gauge System memory value.
*
* Usage example:
*
* private static class TestCalculator implements GaugeCalculator {
* @Override
* public List getValuesList() {
* {@literal Map metrics = new HashMap<>(2);}
* metrics.put("free", Runtime.getRuntime().freeMemory());
* metrics.put("total", Runtime.getRuntime().maxMemory());
* return Arrays.asList(new GaugeResult(metrics));
* }
* }
* CustomGauge memory = new CustomGauge("memory", new TestCalculator());
* memory.getValuesList();
*
*
*/
public class CustomGauge extends Metric {
private final static List EMPTY_LABEL_VALUES = Arrays.asList();
private final GaugeCalculator gaugeCalculator;
/**
* @param name is metric name that will be used when metric is registered
* and/or processed.
* @param gaugeCalculator is used to get the current gauge value map.
*/
public CustomGauge(final String name,
GaugeCalculator gaugeCalculator) {
super(name);
this.gaugeCalculator = gaugeCalculator;
}
/**
* Implement the interface to get current GaugeResult list.
*/
public interface GaugeCalculator extends Metric.Element {
/**
* Get current GaugeResult list.
*/
List getValuesList();
}
/**
* {@inheritDoc}
*/
@Override
protected GaugeCalculator newElement() {
return gaugeCalculator;
}
// Convenience methods.
/**
* {@link GaugeCalculator#getValuesList}.
*/
public List getValuesList() {
try {
return gaugeCalculator.getValuesList();
} catch (Exception e) {
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public MetricFamilySamples collect() {
List gaugeResultList = null;
try {
gaugeResultList = gaugeCalculator.getValuesList();
} catch (Exception e) {
}
if (gaugeResultList == null) {
return null;
}
final List> samples =
new ArrayList>(
gaugeResultList.size());
for (GaugeResult e : gaugeResultList) {
samples.add(
new MetricFamilySamples.Sample(
EMPTY_LABEL_VALUES, e));
}
return new MetricFamilySamples(statsName,
Type.CUSTOM_GAUGE,
labelNames, samples);
}
/**
* Saving collected {@link CustomGauge} result.
*/
public static class GaugeResult extends Metric.Result {
private static final long serialVersionUID = 1L;
private Map map;
public GaugeResult(Map map) {
this.map = map;
}
/**
* {@inheritDoc}
*/
@Override
public Map toMap() {
return map;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy