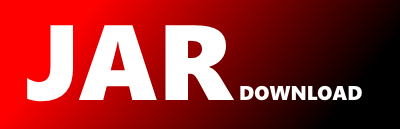
oracle.kv.stats.KVStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oracle-nosql-server Show documentation
Show all versions of oracle-nosql-server Show documentation
NoSQL Database Server - supplies build and runtime support for the server (store) side of the Oracle NoSQL Database.
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.stats;
import java.io.Serializable;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import oracle.kv.KVStore;
import oracle.kv.KVStoreConfig;
import oracle.kv.impl.api.RequestDispatcher;
import oracle.kv.impl.api.ops.InternalOperation.OpCode;
import oracle.kv.impl.api.rgstate.RepNodeState;
import oracle.kv.impl.topo.RepNodeId;
import oracle.kv.impl.topo.Topology;
import oracle.kv.table.TableAPI;
import com.sleepycat.utilint.Latency;
/**
* Statistics associated with accessing the KVStore from a client via the
* KVStore handle. These statistics are from the client's perspective and can
* therefore vary from client to client depending on the configuration and load
* on a specific client as well as the network path between the client and the
* nodes in the KVStore.
*
* @see KVStore#getStats(boolean)
*/
public class KVStats implements Serializable {
private static final long serialVersionUID = 1L;
private final List opMetrics;
private final List nodeMetrics;
private final long requestRetryCount;
/**
* @hidden
* Internal use only.
*/
public KVStats(boolean clear,
RequestDispatcher requestDispatcher) {
opMetrics = new LinkedList();
for (Map.Entry entry :
requestDispatcher.getLatencyStats(clear).entrySet()) {
opMetrics.add(new OperationMetricsImpl(entry.getKey(),
entry.getValue()));
}
nodeMetrics = new LinkedList();
final Topology topology = requestDispatcher.getTopology();
if (topology == null) {
requestRetryCount = 0;
return;
}
for (RepNodeState rns :
requestDispatcher.getRepGroupStateTable().getRepNodeStates()) {
/* Filter out the deleted RNs */
if (topology.get(rns.getRepNodeId()) == null) {
continue;
}
nodeMetrics.add(new NodeMetricsImpl(topology, rns));
if (clear) {
rns.resetStatsCounts();
}
}
requestRetryCount = requestDispatcher.getTotalRetryCount(clear);
}
/**
* Returns a list of metrics associated with each operation supported by
* KVStore. The following table lists the method names and the name
* associated with it by the {@link OperationMetrics#getOperationName()}.
*
* It's worth noting that the metrics related to the Iterator methods are
* special, since each use of an iterator call may result in multiple
* underlying operations depending upon the batchSize
used for
* the iteration.
*
*
*
* {@link KVStore#delete}, {@link TableAPI#delete}
* delete
*
*
* {@link KVStore#deleteIfVersion}, {@link TableAPI#deleteIfVersion}
* deleteIfVersion
*
*
* {@link KVStore#execute}, {@link TableAPI#execute}
* execute
*
*
* {@link KVStore#get}, {@link TableAPI#get}
* get
*
*
* {@link KVStore#multiDelete}, {@link TableAPI#multiDelete}
* multiDelete
*
*
* {@link KVStore#multiGet}, {@link TableAPI#multiGet}
* multiGet
*
*
* {@link KVStore#multiGetIterator}
* multiGetIterator
*
*
* {@link KVStore#multiGetKeys}, {@link TableAPI#multiGetKeys}
* multiGetKeys
*
*
* {@link KVStore#multiGetKeysIterator}
* multiGetKeysIterator
*
*
* {@link KVStore#put}, {@link TableAPI#put}
* put
*
*
* {@link KVStore#putIfAbsent}, {@link TableAPI#putIfAbsent}
* putIfAbsent
*
*
* {@link KVStore#putIfPresent}, {@link TableAPI#putIfPresent}
* putIfPresent
*
*
* {@link KVStore#putIfVersion}, {@link TableAPI#putIfVersion}
* putIfVersion
*
*
* {@link KVStore#storeIterator}, {@link TableAPI#tableIterator}
* storeIterator
*
*
* {@link KVStore#storeKeysIterator}, {@link TableAPI#tableKeysIterator}
* storeKeysIterator
*
*
* {@link TableAPI#tableIterator(oracle.kv.table.IndexKey, oracle.kv.table.MultiRowOptions, oracle.kv.table.TableIteratorOptions)}
* indexIterator
*
*
* {@link TableAPI#tableKeysIterator(oracle.kv.table.IndexKey, oracle.kv.table.MultiRowOptions, oracle.kv.table.TableIteratorOptions)}
* indexKeysIterator
*
*
*
* @return the list of metrics. One for each of the operations listed
* above.
*/
public List getOpMetrics() {
return opMetrics;
}
/**
* Returns a descriptive string containing metrics for each operation that
* was actually performed over during the statistics gathering interval,
* one per line.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
if (requestRetryCount > 0) {
sb.append(String.format("request retry count= %,d\n",
requestRetryCount));
}
for (OperationMetrics metrics : getOpMetrics()) {
if (metrics.getTotalOps() > 0) {
sb.append(metrics.toString()).append("\n");
}
}
for (NodeMetrics metrics : getNodeMetrics()) {
sb.append(metrics.toString()).append("\n");
}
return sb.toString();
}
/**
* Returns the metrics associated with each node in the KVStore.
*
* @return a list containing one entry for each node in the KVStore.
*/
public List getNodeMetrics() {
return nodeMetrics;
}
/**
* @deprecated since 3.4, no longer supported. All of the methods of the
* returned StoreIteratorMetrics object will return 0.
*/
@Deprecated
public StoreIteratorMetrics getStoreIteratorMetrics() {
return new StoreIteratorMetricsStub();
}
/**
* Returns the total number of times requests were retried. A single
* user-level request may be retried transparently at one or more nodes
* until the request succeeds or it times out. This count reflects those
* retry operations.
*
* @see KVStoreConfig#getRequestTimeout(TimeUnit)
*/
public long getRequestRetryCount() {
return requestRetryCount;
}
private static class OperationMetricsImpl
implements OperationMetrics, Serializable {
private static final long serialVersionUID = 1L;
private final String operationName;
private final Latency latency;
OperationMetricsImpl(OpCode opCode, Latency latency) {
this.operationName = opCodeToNameMap.get(opCode);
this.latency = latency;
}
@Override
public String getOperationName() {
return operationName;
}
@Override
public float getAverageLatencyMs() {
return latency.getAvg();
}
@Override
public int getMaxLatencyMs() {
return latency.getMax();
}
@Override
public int getMinLatencyMs() {
return latency.getMin();
}
@Override
public int getTotalOps() {
return latency.getTotalOps();
}
@Override
public int getTotalRequests() {
return latency.getTotalRequests();
}
@Override
public int get95thLatencyMs() {
return latency.get95thPercent();
}
@Override
public int get99thLatencyMs() {
return latency.get99thPercent();
}
/**
* Returns a descriptive string containing the values associated with
* each of the metrics associated with the operation.
*/
@Override
public String toString() {
return String.format("%s: total ops= %,d req= %,d " +
"min/avg/max= %,d/%,.2f/%,d ms",
operationName,
getTotalOps(),
getTotalRequests(),
getMinLatencyMs(),
getAverageLatencyMs(),
getMaxLatencyMs());
}
}
/**
* Converts an upper case enum name into camel case.
*/
private static String camelCase(String enumName) {
StringBuffer sb = new StringBuffer(enumName.length());
for (int i=0; i < enumName.length(); i++) {
char nchar = enumName.charAt(i);
if (nchar == '_') {
if (i++ >= enumName.length()) {
break;
}
nchar = Character.toUpperCase(enumName.charAt(i));
} else {
nchar = Character.toLowerCase(nchar);
}
sb.append(nchar);
}
return sb.toString();
}
private static Map opCodeToNameMap =
new HashMap();
static {
for (OpCode opCode : OpCode.values()) {
opCodeToNameMap.put(opCode, camelCase(opCode.name()));
}
}
private static class NodeMetricsImpl implements NodeMetrics {
private static final long serialVersionUID = 1L;
private final RepNodeId repNodeId;
private final String datacenterName;
private final boolean isActive;
private final boolean isMaster;
private final int maxActiveRequestCount;
private final long accumRespTimeMs;
private final long requestCount;
private final long failedRequestCount;
private NodeMetricsImpl(Topology topology,
RepNodeState rns) {
repNodeId = rns.getRepNodeId();
datacenterName = topology.getDatacenter(repNodeId).getName();
isActive = !rns.reqHandlerNeedsResolution();
isMaster = rns.getRepState().isMaster();
maxActiveRequestCount = rns.getMaxActiveRequestCount();
accumRespTimeMs = rns.getAccumRespTimeMs();
requestCount = rns.getTotalRequestCount();
failedRequestCount = rns.getErrorCount();
}
@Override
public String getNodeName() {
return repNodeId.toString();
}
@Override
public String getDataCenterName() {
return datacenterName;
}
@Override
public String getZoneName() {
return datacenterName;
}
@Override
public boolean isActive() {
return isActive;
}
@Override
public boolean isMaster() {
return isMaster;
}
@Override
public int getMaxActiveRequestCount() {
return maxActiveRequestCount;
}
@Override
public long getRequestCount() {
return requestCount;
}
@Override
public long getFailedRequestCount() {
return failedRequestCount;
}
@Override
public int getAvLatencyMs() {
return requestCount > 0 ? (int)(accumRespTimeMs/requestCount) : 0;
}
/**
* Returns a descriptive string containing the values associated with
* each of the metrics associated with the node.
*/
@Override
public String toString() {
return String.format("%s (Zone %s): isActive= %b, " +
"isMaster= %b, " +
"maxActiveRequests= %,d, " +
"request count= %,d" +
/* Print only if failed. */
((failedRequestCount == 0) ?
", " :
String.format("(failed %,d), ",
failedRequestCount)) +
"avRespTime= %,d ms ",
repNodeId.toString(),
datacenterName,
isActive,
isMaster,
maxActiveRequestCount,
requestCount,
getAvLatencyMs());
}
}
/*
* Stub class to support deprecated API.
*/
@SuppressWarnings("deprecation")
private static class StoreIteratorMetricsStub
implements StoreIteratorMetrics {
@Override
public long getBlockedResultsQueuePuts() { return 0L; }
@Override
public long getAverageBlockedResultsQueuePutTime() { return 0L; }
@Override
public long getMinBlockedResultsQueuePutTime() { return 0L; }
@Override
public long getMaxBlockedResultsQueuePutTime() { return 0L; }
@Override
public long getBlockedResultsQueueGets() { return 0L; }
@Override
public long getAverageBlockedResultsQueueGetTime() { return 0L; }
@Override
public long getMinBlockedResultsQueueGetTime() { return 0L; }
@Override
public long getMaxBlockedResultsQueueGetTime() { return 0L; }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy