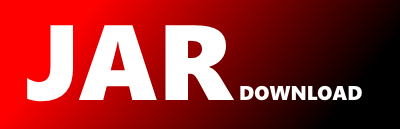
oracle.kv.util.http.HttpServerInitializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oracle-nosql-server Show documentation
Show all versions of oracle-nosql-server Show documentation
NoSQL Database Server - supplies build and runtime support for the server (store) side of the Oracle NoSQL Database.
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.util.http;
import oracle.kv.impl.util.sklogger.SkLogger;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.socket.SocketChannel;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.ssl.SslContext;
import io.netty.handler.timeout.ReadTimeoutHandler;
final class HttpServerInitializer extends ChannelInitializer {
private static final String CODEC_HANDLER_NAME = "http-codec";
private static final String AGG_HANDLER_NAME = "http-aggregator";
private static final String HTTP_HANDLER_NAME = "http-server-handler";
private static final String READ_TIMEOUT_HANDLER_NAME =
"http-read-timeout-handler";
private final HttpServerHandler handler;
private final int maxChunkSize;
private final int maxRequestSize;
private final int idleReadTimeout;
private final SkLogger logger;
private final SslContext sslCtx;
public HttpServerInitializer(HttpServerHandler handler,
HttpServer server,
SslContext sslCtx,
SkLogger logger) {
this.handler = handler;
this.logger = logger;
this.maxRequestSize = server.getMaxRequestSize();
this.maxChunkSize = server.getMaxChunkSize();
this.idleReadTimeout = server.getIdleReadTimeout();
this.sslCtx = sslCtx;
}
/**
* Initialize a channel with handlers that:
* 1 -- handle and HTTP
* 2 -- handle chunked HTTP requests implicitly, only calling channelRead
* with FullHttpRequest.
* 3 -- the request handler itself
*
* TODO: HttpContentCompressor, other options?
*/
@Override
public void initChannel(SocketChannel ch) {
logger.fine("HttpServiceInitializer, initializing new channel");
ChannelPipeline p = ch.pipeline();
if (sslCtx != null) {
p.addLast(sslCtx.newHandler(ch.alloc()));
}
p.addLast(READ_TIMEOUT_HANDLER_NAME,
new ReadTimeoutHandler(idleReadTimeout));
p.addLast(CODEC_HANDLER_NAME,
new HttpServerCodec(4096, // initial line
8192, // header size
maxChunkSize)); // chunksize
p.addLast(AGG_HANDLER_NAME,
new HttpObjectAggregator(maxRequestSize));
p.addLast(HTTP_HANDLER_NAME, handler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy