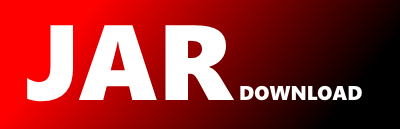
oracle.kv.impl.diagnostic.Checker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oracle-nosql-server Show documentation
Show all versions of oracle-nosql-server Show documentation
NoSQL Database Server - supplies build and runtime support for the server (store) side of the Oracle NoSQL Database.
The newest version!
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.impl.diagnostic;
import java.util.concurrent.Callable;
/**
* Encapsulates a definition and mechanism for checking a kind of parameters of
* boot configuration. Subclasses of Checker will define the different types of
* Checker that can be carried out.
*/
public abstract class Checker implements Callable {
private String NEW_LINE = "\n";
/* Return immediately when get error message */
private boolean returnImmediate;
private String allMessage;
/*
* check the parameters are valid or not, it is called in call(). When
* there is an error found, it should return a non-null value.
*/
public abstract String check() throws Exception;
public Checker(boolean returnImmediate) {
this.returnImmediate = returnImmediate;
}
public void setReturnImmediate(boolean returnImmediate) {
this.returnImmediate = returnImmediate;
}
/**
* Aggregate all error messages when executing
*
* @param retMessage
*/
protected void aggregateMessage(String retMessage) {
if (!returnImmediate) {
if (retMessage != null && !retMessage.isEmpty()) {
if (allMessage == null || allMessage.isEmpty()) {
allMessage = retMessage;
} else {
allMessage += (NEW_LINE + retMessage);
}
}
} else {
allMessage = retMessage;
}
}
/**
* Determines whether the checker should continue its verification or not.
* A non-null retMessage means that a check found a problem. Verification
* should continue if the -force flag was set, or if the retMessage is
* empty or null.
*
* @param retMessage
*/
protected boolean shouldStopCheck(String retMessage) {
if (!returnImmediate) {
return false;
}
if (retMessage != null && !retMessage.isEmpty()) {
return true;
}
return false;
}
/**
* Get message generated in thread
*
* @return message generated by the thread
*/
protected String getMessage() {
if (allMessage == null)
return allMessage;
return allMessage.trim();
}
@Override
public String call() throws Exception {
return check();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy