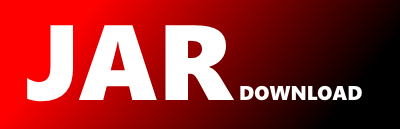
oracle.kv.util.http.LogControl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oracle-nosql-server Show documentation
Show all versions of oracle-nosql-server Show documentation
NoSQL Database Server - supplies build and runtime support for the server (store) side of the Oracle NoSQL Database.
The newest version!
/*-
* Copyright (C) 2011, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle NoSQL
* Database made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/nosqldb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle NoSQL Database for a copy of the license and
* additional information.
*/
package oracle.kv.util.http;
import java.io.IOException;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.logging.Level;
import oracle.kv.impl.util.JsonUtils;
import oracle.kv.impl.util.contextlogger.CorrelationId;
import oracle.kv.impl.util.contextlogger.LogContext;
public class LogControl extends JsonUtils {
/*
* For the time being, stuff both entrypoints and tenants into the same
* map. Later, perhaps this will be refined.
*/
private final Map ctlMap = new ConcurrentHashMap<>();
Level getTenantLogLevel(String tenantId) {
if (tenantId != null) {
final String level = ctlMap.get(tenantId);
if (level != null) {
return Level.parse(level);
}
}
return Level.OFF;
}
Level getEntrypointLogLevel(String entrypoint) {
if (entrypoint != null) {
final String level = ctlMap.get(entrypoint);
if (level != null) {
return Level.parse(level);
}
}
return Level.OFF;
}
public void setTenantLogLevel(String tenantId, Level level) {
ctlMap.put(tenantId, level.toString());
}
public void setEntrypointLogLevel(String entrypoint, Level level) {
ctlMap.put(entrypoint, level.toString());
}
public void removeTenantLogLevel(String tenantId) {
ctlMap.remove(tenantId);
}
public void removeEntrypointLogLevel(String entrypoint) {
ctlMap.remove(entrypoint);
}
public String toJsonString() {
try {
return mapper.writeValueAsString(ctlMap);
} catch (IOException e) {
return null;
}
}
/**
* Produce a new LogContext with a tentative log level based on path.
* Later, if a tenantId is discovered, the LogContext's tenantId and level
* might be modified by updateLogLevel, below.
*/
public LogContext generateLogContext(String path) {
Level level = getEntrypointLogLevel(path);
return new LogContext(CorrelationId.getNext(),
path,
null,
level);
}
/**
* An extant LogContext object can be updated with new information when the
* tenantId is discovered.
*/
public void updateLogContext(LogContext ctx, String tenantId) {
ctx.putOrigin(tenantId);
final Level lt = getTenantLogLevel(tenantId);
final Level le = getEntrypointLogLevel(ctx.getEntry());
Level newLevel = le.intValue() < lt.intValue() ? le : lt;
ctx.putLogLevel(newLevel);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy