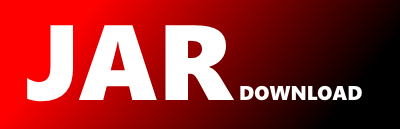
oracle.nosql.driver.NoSQLHandleConfig Maven / Gradle / Ivy
Show all versions of nosqldriver Show documentation
/*-
* Copyright (c) 2011, 2020 Oracle and/or its affiliates. All rights reserved.
*
* Licensed under the Universal Permissive License v 1.0 as shown at
* https://oss.oracle.com/licenses/upl/
*/
package oracle.nosql.driver;
import static oracle.nosql.driver.util.CheckNull.requireNonNull;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.logging.Logger;
import io.netty.handler.ssl.SslContext;
import oracle.nosql.driver.Region.RegionProvider;
import oracle.nosql.driver.iam.SignatureProvider;
/**
* NoSQLHandleConfig groups parameters used to configure a {@link
* NoSQLHandle}. It also provides a way to default common parameters for use by
* {@link NoSQLHandle} methods. When creating a {@link NoSQLHandle} the
* NoSQLHandleConfig instance is copied so modification operations on the
* instance have no effect on existing handles which are immutable. Handle
* state with default values can be overridden in individual operations.
*
* Most of the configuration parameters are optional and have default
* values if not specified. The only required configuration is the
* service endpoint required by the constructor.
*/
public class NoSQLHandleConfig implements Cloneable {
/**
* The default value for request, and table request timeouts in
* milliseconds, if not configured.
*/
private static final int DEFAULT_TIMEOUT = 5000;
private static final int DEFAULT_TABLE_REQ_TIMEOUT = 10000;
/**
* The default value for Consistency if not configured.
*/
private static final Consistency DEFAULT_CONSISTENCY =
Consistency.EVENTUAL;
private static final Set VALID_SSL_PROTOCOLS = new HashSet<>();
static {
VALID_SSL_PROTOCOLS.add("SSLv2");
VALID_SSL_PROTOCOLS.add("SSLv3");
VALID_SSL_PROTOCOLS.add("TLSv1");
VALID_SSL_PROTOCOLS.add("TLSv1.1");
VALID_SSL_PROTOCOLS.add("TLSv1.2");
VALID_SSL_PROTOCOLS.add("TLSv1.3");
}
/*
* The url used to contact an HTTP proxy
*/
private final URL serviceURL;
/*
* Cloud Service Only. The region will be accessed by the NoSQLHandle, which
* will be used to build the service URL.
*/
private Region region;
/**
* The default request timeout, used when a specific operation doesn't
* set its own.
*/
private int timeout;
/**
* The default table request timeout, used when a table operation doesn't
* set its own.
*/
private int tableRequestTimeout;
/**
* The default read consistency, used when a specific operation doesn't
* set its own.
*/
private Consistency consistency;
/**
* The size of the connection pool, which is a fixed-size pool.
*/
private int connectionPoolSize = 2;
/**
* The maximum number of pending acquires for the pool
*/
private int poolMaxPending = 6;
/**
* The number of threads to configure for handling asynchronous netty
* traffic.
*/
private int numThreads = 2;
/**
* The maximum content associated with a request. Payloads that exceed
* this value will result in an IllegalArgumentException.
*
* Default: 0 (use HttpClient default value, currently 32MB)
*/
private int maxContentLength = 0;
/**
* The maximum size of http chunks.
*
* Default: 0 (use HttpClient default value, currently 64KB)
*/
private int maxChunkSize = 0;
/**
* A RetryHandler, or null if not configured by the user.
*/
private RetryHandler retryHandler;
/**
* An AuthorizationProvider, or null if not configured by the user.
*/
private AuthorizationProvider authProvider;
/**
* SslContext. In production this may be set internally so not used here.
* This is used for testing, at least.
*/
private SslContext sslCtx;
/**
* The Logger used by the driver, or null if not configured by the user.
*/
private Logger logger;
/**
* The cipher suites used by the driver, or null if not configured
* by the user.
*/
private List ciphers;
/**
* The protocols used by the driver, or null if not configured
* by the user.
*/
private List protocols;
/**
* The size of cache used for storing SSLSession objects, or 0 if not
* configured by the user.
*/
private int sslSessionCacheSize = 0;
/**
* The timeout limit of SSLSession objects, or 0 if not configured by
* the user.
*/
private int sslSessionTimeout = 0;
/**
* Cloud service only.
*
* The default compartment name or ocid, if set. This may be null
* in which case the compartment used is either the root compartment for
* the tenancy or the compartment specified in a request. Any
* compartment specified in a request or table name overrides this
* default.
*/
private String compartment;
/**
* Enable rate limiting.
* Cloud service only.
*/
private boolean rateLimitingEnabled;
/**
* Default percentage of table limits to use in rate limiting.
* Cloud service only.
*/
private double defaultRateLimiterPercentage;
/*
* HTTP Proxy configuration, optional
*/
private String proxyHost;
private int proxyPort;
private String proxyUsername;
private String proxyPassword;
/**
* Specifies an endpoint or region id to use to connect to the Oracle
* NoSQL Database Cloud Service or, if on-premise, the Oracle NoSQL
* Database proxy server.
*
* There is flexibility in how endpoints are specified. A fully specified
* endpoint is of the format:
*
http[s]://host:port
* This interface accepts portions of a fully specified endpoint, including
* a region id (see {@link Region}) string if using the Cloud service.
*
* A valid endpoint is one of these:
*
* - region id string (cloud service only)
* - a string with the syntax [http[s]://]host[:port]
*
*
* For example, these are valid endpoint arguments:
*
* - us-ashburn-1
* - nosql.us-ashburn-1.oci.oraclecloud.com
* - https://nosql.us-ashburn-1.oci.oraclecloud.com:443
* - localhost:8080
* - https://machine-hosting-proxy:443
*
*
* If using the endpoint (vs region id) syntax, if the port is omitted,
* the endpoint defaults to 443. If the protocol is omitted, the
* endpoint uses https if the port is 443, and http in all other cases.
*
* If using the Cloud Service see {@link Region} for information on
* available regions. If using the Cloud Service the constructor,
* {@link NoSQLHandleConfig#NoSQLHandleConfig(Region,AuthorizationProvider)},
* is available rather than using a Region's id string.
*
* @param endpoint identifies a region id or server for use by the
* NoSQLHandle. This is a required parameter.
*
* @throws IllegalArgumentException if the endpoint is null or malformed.
*/
public NoSQLHandleConfig(String endpoint) {
super();
endpoint = checkRegionId(endpoint, null);
this.serviceURL = createURL(endpoint, "/");
}
/**
* Specifies an endpoint or region id to use to connect to the Oracle
* NoSQL Database Cloud Service or, if on-premise, the Oracle NoSQL
* Database proxy server. In addition an {@link AuthorizationProvider}
* is specified.
*
* There is flexibility in how endpoints are specified. A fully specified
* endpoint is of the format:
*
http[s]://host:port
* This interface accepts portions of a fully specified endpoint, including
* a region id (see {@link Region}) string if using the Cloud service.
*
* A valid endpoint is one of these:
*
* - region id string (cloud service only)
* - a string with the syntax [http[s]://]host[:port]
*
*
* For example, these are valid endpoint arguments:
*
* - us-ashburn-1
* - nosql.us-ashburn-1.oci.oraclecloud.com
* - https://nosql.us-ashburn-1.oci.oraclecloud.com:443
* - localhost:8080
* - https://machine-hosting-proxy:443
*
*
* If using the endpoint (vs region id) syntax, if the port is omitted,
* the endpoint defaults to 443. If the protocol is omitted, the
* endpoint uses https if the port is 443, and http in all other cases.
*
* If using the Cloud Service see {@link Region} for information on
* available regions. If using the Cloud Service the constructor,
* {@link NoSQLHandleConfig#NoSQLHandleConfig(Region,AuthorizationProvider)},
* is available rather than using a Region's id string.
*
* @param endpoint identifies a region id or server for use by the
* NoSQLHandle. This is a required parameter.
*
* @param provider the {@link AuthorizationProvider} to use for the handle
*
* @throws IllegalArgumentException if the endpoint is null or malformed.
*/
public NoSQLHandleConfig(String endpoint, AuthorizationProvider provider) {
super();
endpoint = checkRegionId(endpoint, provider);
this.serviceURL = createURL(endpoint, "/");
this.authProvider = provider;
}
/**
* Cloud service only.
*
* Specify a region to use to connect to the Oracle NoSQL Database Cloud
* Service. The service endpoint will be inferred from the given region.
* This is the recommended constructor for applications using the Oracle
* NoSQL Database Cloud Service.
*
* @param region identifies the region will be accessed by the NoSQLHandle.
*
* @param provider the {@link AuthorizationProvider} to use for the handle
*
* @throws IllegalArgumentException if the region is null or malformed.
*/
public NoSQLHandleConfig(Region region, AuthorizationProvider provider) {
super();
requireNonNull(region, "Region must be non-null");
checkRegion(region, provider);
this.serviceURL = createURL(region.endpoint(), "/");
this.region = region;
this.authProvider = provider;
}
/**
* Cloud service only.
*
* Specify a {@link AuthorizationProvider} to use to connect to the
* Oracle NoSQL Database Cloud Service. The service endpoint will be
* inferred from the given provider. This is the recommended constructor
* for applications using the Oracle NoSQL Database Cloud Service.
*
* @param provider the {@link AuthorizationProvider} to use for the handle
*
* @throws IllegalArgumentException if the region is null or malformed.
*/
public NoSQLHandleConfig(AuthorizationProvider provider) {
super();
requireNonNull(provider, "AuthorizationProvider must be non-null");
if (provider instanceof RegionProvider) {
this.region = ((RegionProvider) provider).getRegion();
}
if (region == null) {
throw new IllegalArgumentException(
"Unable to find region from given AuthorizationProvider");
}
this.serviceURL = createURL(region.endpoint(), "/");
this.authProvider = provider;
}
/**
* @hidden
*
* @param endpoint the endpoint to use
* @param path the path to use
* @return the constructed URL
* Return a URL from an endpoint string
*/
public static URL createURL(String endpoint, String path) {
requireNonNull(endpoint,
"Endpoint must be non-null");
/* The defaults for protocol and port */
String protocol = "https";
int port = 443;
String host = null;
/* Possible formats are:
* - host
* - protocol://host
* - host:port
* - protocol://host:port
*/
String[] parts = endpoint.split(":");
switch (parts.length) {
case 1:
/* endpoint is just */
host = parts[0];
break;
case 2:
/* Could be ://, or could be : */
if (parts[0].toLowerCase().startsWith("http")) {
protocol = parts[0].toLowerCase();
host = parts[1]; /* may have slashes to strip out */
if (protocol.equals("http")) {
/* Override the default of 443 */
port = 8080;
}
} else {
host = parts[0];
port = validatePort(parts[1], endpoint);
if (port != 443) {
/* Override the default of https */
protocol = "http";
}
}
break;
case 3:
/* the full ://: */
protocol = parts[0].toLowerCase();
host = parts[1];
port = validatePort(parts[2], endpoint);
break;
default:
throw new IllegalArgumentException("Invalid endpoint: " +
endpoint);
}
/* Strip out any slashes if the format was protocol://host */
if (host.startsWith("//")) {
host = host.substring(2);
}
try {
return new URL(protocol, host, port, path);
} catch (MalformedURLException e) {
throw new IllegalArgumentException(e);
}
}
/*
* Check that a port is a valid, non negative integer.
*/
private static int validatePort(String portString, String endpoint) {
try {
int port = Integer.parseInt(portString);
if (port < 0) {
throw new IllegalArgumentException
("invalid port value of " + port + " for endpoint:" +
endpoint);
}
return port;
} catch (Exception e) {
throw new IllegalArgumentException("invalid port value for " +
"endpoint:" + e);
}
}
/**
* Sets the URL to use to connect to the service, as alternative to
* setting the endpont.
*
* @param serviceURL a URL to locate a server for use by the NoSQLHandle.
* It is used and validated in {@link NoSQLHandleFactory#createNoSQLHandle}.
* This is a required parameter.
*
* @throws IllegalArgumentException if the URL is null or malformed.
*/
public NoSQLHandleConfig(URL serviceURL) {
super();
requireNonNull(serviceURL,
"NoSQLHandleConfig: serviceURL must be non-null");
try {
this.serviceURL = new URL(serviceURL.getProtocol(),
serviceURL.getHost(),
serviceURL.getPort(), "/");
} catch (MalformedURLException e) {
throw new IllegalArgumentException(e);
}
}
/**
* Returns the version of the driver implemented by this library.
* @return the version as a string
*/
static public String getLibraryVersion() {
return DriverMain.getLibraryVersion();
}
/**
* Returns the URL to use for the {@link NoSQLHandle} connection
*
* @return the URL.
*/
public URL getServiceURL() {
return serviceURL;
}
/**
* Cloud service only.
*
* Returns the region will be accessed by the NoSQLHandle.
*
* @return the region.
*/
public Region getRegion() {
return region;
}
/**
* Returns the configured request timeout value, in milliseconds.
*
* @return the timeout, in milliseconds, or 0 if it has not been set
*/
public int getRequestTimeout() {
return timeout;
}
/**
* Returns the default value for request timeout. If there is no
* configured timeout or it is configured as 0, a "default" default
* value of 5000 milliseconds is used.
*
* @return the default timeout, in milliseconds
*/
public int getDefaultRequestTimeout() {
return timeout == 0 ? DEFAULT_TIMEOUT : timeout;
}
/**
* Returns the configured table request timeout value, in milliseconds.
* The table request timeout default can be specified independently to allow
* it to be larger than a typical data request. If it is not specified the
* default table request timeout of 10000 is used.
*
* @return the timeout, in milliseconds, or 0 if it has not been set
*/
public int getTableRequestTimeout() {
return tableRequestTimeout;
}
/**
* Returns the default value for a table request timeout. If there is no
* configured timeout or it is configured as 0, a "default" default
* value of 10000 milliseconds is used.
*
* @return the default timeout, in milliseconds
*/
public int getDefaultTableRequestTimeout() {
return tableRequestTimeout == 0 ? DEFAULT_TABLE_REQ_TIMEOUT :
tableRequestTimeout;
}
/**
* Returns the configured default consistency
*
* @return the Consistency, or null if it has not been configured
*/
public Consistency getConsistency() {
return consistency;
}
/**
* Returns the default Consistency value that will be used by the
* system. If Consistency has been set using {@link #setConsistency}
* that will be returned. If not a default value of
* {@link Consistency#EVENTUAL} is returned
*
* @return the default Consistency
*/
public Consistency getDefaultConsistency() {
return consistency == null ? DEFAULT_CONSISTENCY : consistency;
}
/**
* Returns the {@link RetryHandler} configured for the handle, or null
* if none is set.
*
* @return the handler
*/
public RetryHandler getRetryHandler() {
return retryHandler;
}
/**
* Returns the {@link AuthorizationProvider} configured for the handle, or
* null if none is set.
*
* @return the AuthorizationProvider
*/
public AuthorizationProvider getAuthorizationProvider() {
return authProvider;
}
/**
* Sets the default request timeout. The default timeout is
* 5 seconds.
*
* @param timeout the timeout value, in milliseconds
*
* @return this
*/
public NoSQLHandleConfig setRequestTimeout(int timeout) {
this.timeout = timeout;
return this;
}
/**
* Sets the default table request timeout. The default timeout is
* 5 seconds. The table request timeout can be specified independently
* of that specified by {@link #setRequestTimeout} because table requests can
* take longer and justify longer timeouts. The default timeout is 10
* seconds (10000 milliseconds).
*
* @param tableRequestTimeout the timeout value, in milliseconds
*
* @return this
*/
public NoSQLHandleConfig setTableRequestTimeout(int tableRequestTimeout) {
this.tableRequestTimeout = tableRequestTimeout;
return this;
}
/**
* Sets the default request {@link Consistency}. If not set in this
* object or by a specific request the default consistency used
* is {@link Consistency#EVENTUAL}
*
* @param consistency the Consistency
*
* @return this
*/
public NoSQLHandleConfig setConsistency(Consistency consistency) {
requireNonNull(
consistency,
"NoSQLHandleConfig.setConsistency: consistency must be non-null");
this.consistency = consistency;
return this;
}
/**
* Sets the number of threads to use for handling
* network traffic. This number affects the performance of concurrent
* requests in a multithreaded application.
*
* @param numThreads the number
*
* @return this
*/
public NoSQLHandleConfig setNumThreads(int numThreads) {
if (numThreads <= 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setNumThreads: numThreads must " +
"be a positive value");
}
this.numThreads = numThreads;
return this;
}
/**
* Sets the maximum number of individual connections to use to connect
* to the service. Each request/response pair uses a connection. The
* pool exists to allow concurrent requests and will bound the number of
* concurrent requests. Additional requests will wait for a connection to
* become available.
*
* @param poolSize the pool size
*
* @return this
*/
public NoSQLHandleConfig setConnectionPoolSize(int poolSize) {
if (poolSize <= 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setConnectionPoolSize: poolSize must " +
"be a positive value");
}
this.connectionPoolSize = poolSize;
return this;
}
/**
* Sets the maximum number of pending acquire operations allowed on the
* connection pool. This number is used if the degree of concurrency
* desired exceeds the size of the connection pool temporarily.
*
* @param poolMaxPending the maximum number allowed
*
* @return this
*/
public NoSQLHandleConfig setPoolMaxPending(int poolMaxPending) {
if (poolMaxPending <= 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setPoolMaxPending: poolMaxPending must " +
"be a positive value");
}
this.poolMaxPending = poolMaxPending;
return this;
}
/**
* Sets the maximum size in bytes of request/response payloads.
* On-premise only. This setting is ignored for cloud operations.
* If not set, or set to zero, the default value of 32MB is used.
*
* @param maxContentLength the maximum bytes allowed in
* requests/responses. Pass zero to use the default.
*
* @return this
*/
public NoSQLHandleConfig setMaxContentLength(int maxContentLength) {
if (maxContentLength < 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setMaxContentLength: maxContentLength must " +
"not be negative");
}
this.maxContentLength = maxContentLength;
return this;
}
/**
* Returns the maximum size, in bytes, of a request operation payload.
* On-premise only. This value is ignored for cloud operations.
*
* @return the size
*/
public int getMaxContentLength() {
return maxContentLength;
}
/**
* @hidden
* Sets the maximum size in bytes of http chunks.
* If not set, or set to zero, the default value of 64KB is used.
*
* @param maxChunkSize the maximum bytes allowed in
* http chunks. Pass zero to use the default.
*
* @return this
*/
public NoSQLHandleConfig setMaxChunkSize(int maxChunkSize) {
if (maxChunkSize < 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setMaxChunkSize: maxChunkSize must " +
"not be negative");
}
this.maxChunkSize = maxChunkSize;
return this;
}
/**
* @hidden
* Returns the maximum size, in bytes, of any http chunk
*
* @return the size
*/
public int getMaxChunkSize() {
return maxChunkSize;
}
/**
* Returns the maximum number of individual connections to use to connect
* to the service. Each request/response pair uses a connection. The
* pool exists to allow concurrent requests and will bound the number of
* concurrent requests. Additional requests will wait for a connection to
* become available.
*
* @return the pool size
*/
public int getConnectionPoolSize() {
return connectionPoolSize;
}
/**
* Returns the maximum number of pending acquire operations allowed on
* the connection pool.
*
* @return the maximum pending acquire size
*/
public int getPoolMaxPending() {
return poolMaxPending;
}
/**
* Returns the number of threads to use for handling
* network traffic.
*
* @return the pool size
*/
public int getNumThreads() {
return numThreads;
}
/**
* Sets the {@link RetryHandler} to use for the handle. If no handler is
* configured a default is used. The handler must be safely usable by
* multiple threads.
*
* @param retryHandler the handler
*
* @return this
*/
public NoSQLHandleConfig setRetryHandler(RetryHandler retryHandler) {
requireNonNull(
retryHandler,
"NoSQLHandleConfig.setRetryHandler: retryHandler must be non-null");
this.retryHandler = retryHandler;
return this;
}
/**
* Sets the {@link AuthorizationProvider} to use for the handle. The
* provider must be safely usable by multiple threads.
*
* @param provider the AuthorizationProvider
*
* @return this
*/
public NoSQLHandleConfig
setAuthorizationProvider(AuthorizationProvider provider) {
requireNonNull(provider, "NoSQLHandleConfig.setAuthorizationProvider" +
": provider must be non-null");
this.authProvider = provider;
return this;
}
/**
* Enables internal rate limiting.
* Cloud service only.
* @param enable If true, enable internal rate limiting.
* If false, disable internal rate limiting.
* @return this
*/
public NoSQLHandleConfig setRateLimitingEnabled(boolean enable) {
this.rateLimitingEnabled = enable;
return this;
}
/**
* @hidden
* Internal use only
* @return true if rate limiting is enabled
*/
public boolean getRateLimitingEnabled() {
return rateLimitingEnabled;
}
/**
* Sets a default rate limiter use percentage.
* Cloud service only.
*
* Sets a default percentage of table limits to use. This may be
* useful for cases where a client should only use a portion of
* full table limits. This only applies if rate limiting is enabled using
* {@link #setRateLimitingEnabled}.
*
* The default for this value is 100.0 (full table limits).
*
* @param percent the percentage of table limits to use. This value
* must be positive.
*/
public void setDefaultRateLimitingPercentage(double percent) {
if (percent <= 0.0) {
throw new IllegalArgumentException(
"rate limiter percentage must be positive");
}
defaultRateLimiterPercentage = percent;
}
/**
* @hidden
* Internal use only
* @return the default percentage
*/
public double getDefaultRateLimitingPercentage() {
if (defaultRateLimiterPercentage == 0.0) {
return 100.0;
}
return defaultRateLimiterPercentage;
}
/**
* Sets the {@link RetryHandler} using a default retry handler configured
* with the specified number of retries and a static delay.
* A delay of 0 means "use the default delay algorithm" which is an
* incremental backoff algorithm. A non-zero delay will work but is not
* recommended for production systems as it is not flexible.
*
* The default retry handler will not retry exceptions of type
* {@link OperationThrottlingException}. The reason is that these
* operations are long-running, and while technically they can be
* retried, an immediate retry is unlikely to succeed because of the
* low rates allowed for these operations.
*
* @param numRetries the number of retries to perform automatically.
* This parameter may be 0 for no retries.
* @param delayMS the delay, in milliseconds. Pass 0 to use the default
* delay algorithm.
*
* @return this
*/
public NoSQLHandleConfig configureDefaultRetryHandler(int numRetries,
int delayMS) {
retryHandler = new DefaultRetryHandler(numRetries, delayMS);
return this;
}
/**
* Sets the Logger used for the driver.
*
* @param logger the Logger.
*
* @return this
*/
public NoSQLHandleConfig setLogger(Logger logger) {
requireNonNull(logger,
"NoSQLHandleConfig.setLogger: logger must be non-null");
this.logger = logger;
return this;
}
/**
* Returns the Logger, or null if not configured by user.
*
* @return the Logger
*/
public Logger getLogger() {
return logger;
}
/**
* Cloud service only.
*
* Sets the default compartment to use for requests sent using the
* handle. Setting the default is optional and if set it is overridden
* by any compartment specified in a request or table name. If no
* compartment is set for a request, either using this default or
* by specification in a request, the behavior varies with how the
* application is authenticated.
*
* - If authenticated with a user identity the default is the root
* compartment of the tenancy
* - If authenticated as an instance principal (see
* {@link SignatureProvider#createWithInstancePrincipal}) the
* compartment id (OCID )must be specified by either using this method or
* in each Request object. If not an exception is thrown
*
*
* @param compartment may be either the name of a compartment or the
* id (OCID) of a compartment.
*
* @return this
*/
public NoSQLHandleConfig setDefaultCompartment(String compartment) {
this.compartment = compartment;
return this;
}
/**
* Cloud service only.
*
* Returns the default compartment to use for requests or null if not set.
* The value may be a compartment name or id, as set by
* {@link #setDefaultCompartment}.
*
* @return the compartment
*/
public String getDefaultCompartment() {
return compartment;
}
/**
* Returns the SSL cipher suites to enable.
* @return cipher suites.
*/
public List getSSLCipherSuites() {
return ciphers;
}
/**
* Returns the SSL protocols to enable.
* @return cipher suites.
*/
public String[] getSSLProtocols() {
if (protocols == null) {
return null;
}
return protocols.stream().toArray(String[] :: new);
}
/**
* Returns the configured SSLSession objects timeout, in seconds.
*
* @return the timeout, in seconds, or 0 if it has not been set
*/
public int getSSLSessionTimeout() {
return sslSessionTimeout;
}
/**
* Returns the configured size of cache used to store SSLSession objects.
*
* @return the cache size
*/
public int getSSLSessionCacheSize() {
return sslSessionCacheSize;
}
/**
* Set SSL cipher suites to enable, in the order of preference. null to
* use default cipher suites.
*
* @param cipherSuites cipher suites list
* @return this
*/
public NoSQLHandleConfig setSSLCipherSuites(String... cipherSuites) {
if (ciphers == null) {
ciphers = new ArrayList<>(Arrays.asList(cipherSuites));
} else {
ciphers.addAll(Arrays.asList(cipherSuites));
}
return this;
}
/**
* Set SSL protocols to enable, in the order of preference. null to
* use default protocols.
*
* @param sslProtocols SSL protocols list
* @return this
*/
public NoSQLHandleConfig setSSLProtocols(String... sslProtocols) {
if (protocols == null) {
protocols = new ArrayList<>(Arrays.asList(sslProtocols));
} else {
protocols.addAll(Arrays.asList(sslProtocols));
}
for (String protocol : protocols) {
if (!VALID_SSL_PROTOCOLS.contains(protocol)) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setSSLProtocols: " + protocol +
" is not a valid SSL protocol name. Must be one of " +
VALID_SSL_PROTOCOLS);
}
}
return this;
}
/**
* Sets the size of the cache used for storing SSL session objects, 0
* to use the default value, no size limit.
*
* @param cacheSize the size of SSLSession objects.
*
* @return this
*/
public NoSQLHandleConfig setSSLSessionCacheSize(int cacheSize) {
if (cacheSize < 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setSSLSessionCacheSize: cacheSize must " +
"be a positive value or zero");
}
this.sslSessionCacheSize = cacheSize;
return this;
}
/**
* Set the timeout for the cached SSL session objects, in seconds. 0 to
* use the default value, no limit. When the timeout limit is exceeded for
* a session, the SSLSession object is invalidated and future connections
* cannot resume or rejoin the session.
*
* @param timeout the session timeout
*
* @return this
*/
public NoSQLHandleConfig setSSLSessionTimeout(int timeout) {
if (timeout < 0) {
throw new IllegalArgumentException(
"NoSQLHandleConfig.setSSLSessionTimeout: timeout must " +
"be a positive value or zero");
}
this.sslSessionTimeout = timeout;
return this;
}
/**
* @hidden
* Sets an HTTP proxy host to be used for the session. If a proxy host
* is specified a proxy port must also be specified, using
* {@link #setProxyPort}.
*
* @param proxyHost the proxy host
*
* @return this
*/
public NoSQLHandleConfig setProxyHost(String proxyHost) {
this.proxyHost = proxyHost;
return this;
}
/**
* @hidden
* Sets an HTTP proxy user name if the configured proxy host requires
* authentication. If a proxy host is not configured this configuration
* is ignored.
*
* @param proxyUsername the user name
*
* @return this
*/
public NoSQLHandleConfig setProxyUsername(String proxyUsername) {
this.proxyUsername = proxyUsername;
return this;
}
/**
* @hidden
* Sets an HTTP proxy password if the configured proxy host requires
* authentication. If a proxy user name is not configured this configuration
* is ignored.
*
* @param proxyPassword the password
*
* @return this
*/
public NoSQLHandleConfig setProxyPassword(String proxyPassword) {
this.proxyPassword = proxyPassword;
return this;
}
/**
* @hidden
* Sets an HTTP proxy port to be used for the session. If a proxy port
* is specified a proxy host must also be specified, using
* {@link #setProxyHost}.
*
* @param proxyPort the proxy port
*
* @return this
*/
public NoSQLHandleConfig setProxyPort(int proxyPort) {
this.proxyPort = proxyPort;
return this;
}
/**
* @hidden
* Returns a proxy host, or null if not configured
*
* @return the host, or null
*/
public String getProxyHost() {
return proxyHost;
}
/**
* @hidden
* Returns a proxy user name, or null if not configured
*
* @return the user name, or null
*/
public String getProxyUsername() {
return proxyUsername;
}
/**
* @hidden
* Returns a proxy password, or null if not configured
*
* @return the password, or null
*/
public String getProxyPassword() {
return proxyPassword;
}
/**
* @hidden
* Returns a proxy port, or 0 if not configured
*
* @return the proxy port
*/
public int getProxyPort() {
return proxyPort;
}
/**
* @hidden
* @param sslCtx the SSL context to set
* @return this
*/
public NoSQLHandleConfig setSslContext(SslContext sslCtx) {
this.sslCtx = sslCtx;
return this;
}
/**
* @hidden
* @return the SSL context
*/
public SslContext getSslContext() {
return sslCtx;
}
@Override
public NoSQLHandleConfig clone() {
try {
NoSQLHandleConfig clone = (NoSQLHandleConfig) super.clone();
return clone;
} catch (CloneNotSupportedException neverHappens) {
return null;
}
}
static private String checkRegionId(String endpoint,
AuthorizationProvider provider) {
try {
Region region = Region.fromRegionId(endpoint);
if (region != null) {
checkRegion(region, provider);
return region.endpoint();
}
} catch (IllegalArgumentException iae) {
/* ignore */
}
return endpoint;
}
static private void checkRegion(Region region,
AuthorizationProvider provider) {
if (provider instanceof RegionProvider) {
Region regionInProvider = ((RegionProvider) provider).getRegion();
if (regionInProvider != null && regionInProvider != region) {
throw new IllegalArgumentException(
"Specified region " + region + " doesn't match the region " +
" in AuthorizationProvider");
}
}
}
}