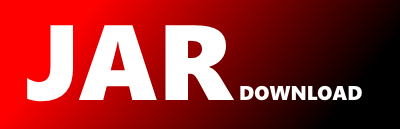
com.oracle.bmc.apigateway.model.WorkRequestSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oci-java-sdk-apigateway Show documentation
Show all versions of oci-java-sdk-apigateway Show documentation
This project contains the SDK used for Oracle Cloud Infrastructure Apigateway
/**
* Copyright (c) 2016, 2020, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.apigateway.model;
/**
* A summary of the work request.
*
* Note: Objects should always be created or deserialized using the {@link Builder}. This model distinguishes fields
* that are {@code null} because they are unset from fields that are explicitly set to {@code null}. This is done in
* the setter methods of the {@link Builder}, which maintain a set of all explicitly set fields called
* {@link #__explicitlySet__}. The {@link #hashCode()} and {@link #equals(Object)} methods are implemented to take
* {@link #__explicitlySet__} into account. The constructor, on the other hand, does not set {@link #__explicitlySet__}
* (since the constructor cannot distinguish explicit {@code null} from unset {@code null}).
**/
@javax.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20190501")
@lombok.AllArgsConstructor(onConstructor = @__({@Deprecated}))
@lombok.Value
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(
builder = WorkRequestSummary.Builder.class
)
@com.fasterxml.jackson.annotation.JsonFilter(com.oracle.bmc.http.internal.ExplicitlySetFilter.NAME)
@lombok.Builder(builderClassName = "Builder", toBuilder = true)
public class WorkRequestSummary {
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
@lombok.experimental.Accessors(fluent = true)
public static class Builder {
@com.fasterxml.jackson.annotation.JsonProperty("operationType")
private WorkRequest.OperationType operationType;
public Builder operationType(WorkRequest.OperationType operationType) {
this.operationType = operationType;
this.__explicitlySet__.add("operationType");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("status")
private WorkRequest.Status status;
public Builder status(WorkRequest.Status status) {
this.status = status;
this.__explicitlySet__.add("status");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("workRequestId")
private String workRequestId;
public Builder workRequestId(String workRequestId) {
this.workRequestId = workRequestId;
this.__explicitlySet__.add("workRequestId");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private String compartmentId;
public Builder compartmentId(String compartmentId) {
this.compartmentId = compartmentId;
this.__explicitlySet__.add("compartmentId");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("percentComplete")
private Float percentComplete;
public Builder percentComplete(Float percentComplete) {
this.percentComplete = percentComplete;
this.__explicitlySet__.add("percentComplete");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("timeAccepted")
private java.util.Date timeAccepted;
public Builder timeAccepted(java.util.Date timeAccepted) {
this.timeAccepted = timeAccepted;
this.__explicitlySet__.add("timeAccepted");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("timeStarted")
private java.util.Date timeStarted;
public Builder timeStarted(java.util.Date timeStarted) {
this.timeStarted = timeStarted;
this.__explicitlySet__.add("timeStarted");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("timeFinished")
private java.util.Date timeFinished;
public Builder timeFinished(java.util.Date timeFinished) {
this.timeFinished = timeFinished;
this.__explicitlySet__.add("timeFinished");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public WorkRequestSummary build() {
WorkRequestSummary __instance__ =
new WorkRequestSummary(
operationType,
status,
workRequestId,
compartmentId,
percentComplete,
timeAccepted,
timeStarted,
timeFinished);
__instance__.__explicitlySet__.addAll(__explicitlySet__);
return __instance__;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(WorkRequestSummary o) {
Builder copiedBuilder =
operationType(o.getOperationType())
.status(o.getStatus())
.workRequestId(o.getWorkRequestId())
.compartmentId(o.getCompartmentId())
.percentComplete(o.getPercentComplete())
.timeAccepted(o.getTimeAccepted())
.timeStarted(o.getTimeStarted())
.timeFinished(o.getTimeFinished());
copiedBuilder.__explicitlySet__.retainAll(o.__explicitlySet__);
return copiedBuilder;
}
}
/**
* Create a new builder.
*/
public static Builder builder() {
return new Builder();
}
/**
* The type of the work request.
**/
@com.fasterxml.jackson.annotation.JsonProperty("operationType")
WorkRequest.OperationType operationType;
/**
* The status of the work request.
**/
@com.fasterxml.jackson.annotation.JsonProperty("status")
WorkRequest.Status status;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the resource.
*
**/
@com.fasterxml.jackson.annotation.JsonProperty("workRequestId")
String workRequestId;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the compartment in which the
* resource is created.
*
**/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
String compartmentId;
/**
* Percentage of the request completed.
**/
@com.fasterxml.jackson.annotation.JsonProperty("percentComplete")
Float percentComplete;
/**
* The date and time the request was created, as described in
* [RFC 3339](https://tools.ietf.org/rfc/rfc3339), section 14.29.
*
**/
@com.fasterxml.jackson.annotation.JsonProperty("timeAccepted")
java.util.Date timeAccepted;
/**
* The date and time the request was started, as described in [RFC 3339](https://tools.ietf.org/rfc/rfc3339),
* section 14.29.
*
**/
@com.fasterxml.jackson.annotation.JsonProperty("timeStarted")
java.util.Date timeStarted;
/**
* The date and time the request was finished, as described in [RFC 3339](https://tools.ietf.org/rfc/rfc3339).
*
**/
@com.fasterxml.jackson.annotation.JsonProperty("timeFinished")
java.util.Date timeFinished;
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy