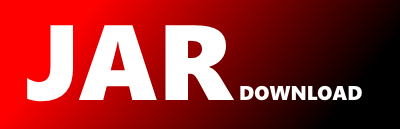
com.oracle.bmc.http.client.jersey3.Jersey3HttpResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oci-java-sdk-common-httpclient-jersey3 Show documentation
Show all versions of oci-java-sdk-common-httpclient-jersey3 Show documentation
This project contains the HTTP client jersey 3 implementation of the SDK used for Oracle Cloud Infrastructure
/**
* Copyright (c) 2016, 2023, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.http.client.jersey3;
import com.oracle.bmc.http.client.HttpResponse;
import com.oracle.bmc.http.client.Options;
import com.oracle.bmc.http.client.jersey3.io.internal.AutoCloseableContentLengthVerifyingInputStream;
import com.oracle.bmc.http.client.jersey3.io.internal.ContentLengthVerifyingInputStream;
import jakarta.ws.rs.core.GenericType;
import jakarta.ws.rs.core.HttpHeaders;
import jakarta.ws.rs.core.Response;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
class Jersey3HttpResponse implements HttpResponse {
private static final Logger log = LoggerFactory.getLogger(Jersey3HttpResponse.class);
private final Response response;
private boolean bodyConsumed = false;
Jersey3HttpResponse(Response response) {
this.response = response;
}
@Override
public int status() {
return response.getStatus();
}
@Override
public Map> headers() {
return response.getStringHeaders();
}
@Override
public String header(String name) {
return response.getHeaderString(name);
}
@Override
public CompletionStage streamBody() {
bodyConsumed = true;
// If we want an InputStream, then we don't care about the content type.
// This will also allow us to process invalid content types like "text" (instead of
// "text/plain").
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy