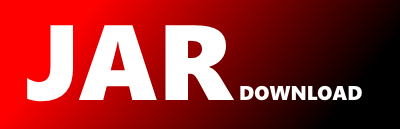
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel Maven / Gradle / Ivy
Show all versions of oci-java-sdk-common-httpclient Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.http.client.internal;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import java.util.HashSet;
import java.util.Set;
/**
* Base class for models implementing the explicitly set behavior. Subclasses should be annotated
* with {@code
* com.fasterxml.jackson.annotation.JsonFilter(com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)}.
*
* All the properties that were explicitly set by user will be serialized, even if set to {@code
* null}.
*/
@JsonFilter(ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public abstract class ExplicitlySetBmcModel {
/**
* The name of the explicitly set filter for usage in serialization / deserialization
* implementations
*/
public static final String EXPLICITLY_SET_FILTER_NAME = "explicitlySetFilter";
/**
* The name of the explicitly set map property for usage in serialization / deserialization
* implementations
*/
public static final String EXPLICITLY_SET_PROPERTY_NAME = "__explicitlySet__";
/**
* A hash set that stores the names of the properties that were explicitly set by user. These
* properties will be included in the json request even if they were set to {@code null}.
*/
@JsonIgnore private final Set __explicitlySet__ = new HashSet<>();
/**
* Specify that a model property was explicitly set by user. The method should be used inside
* property setters.
*
* @param propertyName the name of the model property to mark as set by user. Should match the
* property name exactly.
*/
protected void markPropertyAsExplicitlySet(String propertyName) {
__explicitlySet__.add(propertyName);
}
/**
* Get whether a property was explicitly specified by user. It is highly unlikely that this is
* the method you want to use as a user.
*
* @param propertyName the name of the property.
* @return whether the property was explicitly specified by user.
*/
public boolean wasPropertyExplicitlySet(String propertyName) {
return this.__explicitlySet__.contains(propertyName);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ExplicitlySetBmcModel)) {
return false;
}
return java.util.Objects.equals(
this.__explicitlySet__, ((ExplicitlySetBmcModel) o).__explicitlySet__);
}
@Override
public int hashCode() {
return this.__explicitlySet__.hashCode();
}
@Override
public String toString() {
return new StringBuilder()
.append("BmcModel(__explicitlySet__=")
.append(this.__explicitlySet__)
.append(")")
.toString();
}
}