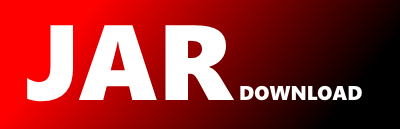
com.oracle.bmc.database.DatabaseAsync Maven / Gradle / Ivy
Show all versions of oci-java-sdk-database Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.database;
import com.oracle.bmc.database.requests.*;
import com.oracle.bmc.database.responses.*;
/**
* The API for the Database Service. Use this API to manage resources such as databases and DB
* Systems. For more information, see [Overview of the Database
* Service](https://docs.cloud.oracle.com/iaas/Content/Database/Concepts/databaseoverview.htm).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20160918")
public interface DatabaseAsync extends AutoCloseable {
/** Rebuilds the client from scratch. Useful to refresh certificates. */
void refreshClient();
/**
* Sets the endpoint to call (ex, https://www.example.com).
*
* @param endpoint The endpoint of the serice.
*/
void setEndpoint(String endpoint);
/** Gets the set endpoint for REST call (ex, https://www.example.com) */
String getEndpoint();
/**
* Sets the region to call (ex, Region.US_PHOENIX_1).
*
* Note, this will call {@link #setEndpoint(String) setEndpoint} after resolving the
* endpoint. If the service is not available in this region, however, an
* IllegalArgumentException will be raised.
*
* @param region The region of the service.
*/
void setRegion(com.oracle.bmc.Region region);
/**
* Sets the region to call (ex, 'us-phoenix-1').
*
*
Note, this will first try to map the region ID to a known Region and call {@link
* #setRegion(Region) setRegion}.
*
*
If no known Region could be determined, it will create an endpoint based on the default
* endpoint format ({@link com.oracle.bmc.Region#formatDefaultRegionEndpoint(Service, String)}
* and then call {@link #setEndpoint(String) setEndpoint}.
*
* @param regionId The public region ID.
*/
void setRegion(String regionId);
/**
* Determines whether realm specific endpoint should be used or not. Set
* realmSpecificEndpointTemplateEnabled to "true" if the user wants to enable use of realm
* specific endpoint template, otherwise set it to "false"
*
* @param realmSpecificEndpointTemplateEnabled flag to enable the use of realm specific endpoint
* template
*/
void useRealmSpecificEndpointTemplate(boolean realmSpecificEndpointTemplateEnabled);
/**
* Activates the specified Exadata infrastructure resource. Applies to Exadata Cloud@Customer
* instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
activateExadataInfrastructure(
ActivateExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
ActivateExadataInfrastructureRequest,
ActivateExadataInfrastructureResponse>
handler);
/**
* Makes the storage capacity from additional storage servers available for Cloud VM Cluster
* consumption. Applies to Exadata Cloud Service instances and Autonomous Database on dedicated
* Exadata infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
addStorageCapacityCloudExadataInfrastructure(
AddStorageCapacityCloudExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
AddStorageCapacityCloudExadataInfrastructureRequest,
AddStorageCapacityCloudExadataInfrastructureResponse>
handler);
/**
* Makes the storage capacity from additional storage servers available for VM Cluster
* consumption. Applies to Exadata Cloud@Customer instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
addStorageCapacityExadataInfrastructure(
AddStorageCapacityExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
AddStorageCapacityExadataInfrastructureRequest,
AddStorageCapacityExadataInfrastructureResponse>
handler);
/**
* Add Virtual Machines to the Cloud VM cluster. Applies to Exadata Cloud instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
addVirtualMachineToCloudVmCluster(
AddVirtualMachineToCloudVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
AddVirtualMachineToCloudVmClusterRequest,
AddVirtualMachineToCloudVmClusterResponse>
handler);
/**
* Add Virtual Machines to the VM cluster. Applies to Exadata Cloud@Customer instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addVirtualMachineToVmCluster(
AddVirtualMachineToVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
AddVirtualMachineToVmClusterRequest,
AddVirtualMachineToVmClusterResponse>
handler);
/**
* Initiates a data refresh for an Autonomous Database refreshable clone. Data is refreshed from
* the source database to the point of a specified timestamp.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
autonomousDatabaseManualRefresh(
AutonomousDatabaseManualRefreshRequest request,
com.oracle.bmc.responses.AsyncHandler<
AutonomousDatabaseManualRefreshRequest,
AutonomousDatabaseManualRefreshResponse>
handler);
/**
* Cancel automatic/standalone full/incremental create backup workrequests specified by the
* backup Id.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future cancelBackup(
CancelBackupRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Cancels the in progress maintenance activity under this execution window.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future cancelExecutionWindow(
CancelExecutionWindowRequest request,
com.oracle.bmc.responses.AsyncHandler<
CancelExecutionWindowRequest, CancelExecutionWindowResponse>
handler);
/**
* Delete the scheduling plan resource along with all the scheduled actions associated with this
* resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
cascadingDeleteSchedulingPlan(
CascadingDeleteSchedulingPlanRequest request,
com.oracle.bmc.responses.AsyncHandler<
CascadingDeleteSchedulingPlanRequest,
CascadingDeleteSchedulingPlanResponse>
handler);
/**
* Move the Autonomous Container Database and its dependent resources to the specified
* compartment. For more information about moving Autonomous Container Databases, see [Moving
* Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeAutonomousContainerDatabaseCompartment(
ChangeAutonomousContainerDatabaseCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeAutonomousContainerDatabaseCompartmentRequest,
ChangeAutonomousContainerDatabaseCompartmentResponse>
handler);
/**
* Move the Autonomous Database and its dependent resources to the specified compartment. For
* more information about moving Autonomous Databases, see [Moving Database Resources to a
* Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeAutonomousDatabaseCompartment(
ChangeAutonomousDatabaseCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeAutonomousDatabaseCompartmentRequest,
ChangeAutonomousDatabaseCompartmentResponse>
handler);
/**
* Move the Autonomous Database Software Image and its dependent resources to the specified
* compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeAutonomousDatabaseSoftwareImageCompartment(
ChangeAutonomousDatabaseSoftwareImageCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeAutonomousDatabaseSoftwareImageCompartmentRequest,
ChangeAutonomousDatabaseSoftwareImageCompartmentResponse>
handler);
/**
* Associate an Autonomous Database with a different subscription.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeAutonomousDatabaseSubscription(
ChangeAutonomousDatabaseSubscriptionRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeAutonomousDatabaseSubscriptionRequest,
ChangeAutonomousDatabaseSubscriptionResponse>
handler);
/**
* **Deprecated.** Use the {@link
* #changeCloudExadataInfrastructureCompartment(ChangeCloudExadataInfrastructureCompartmentRequest,
* Consumer, Consumer) changeCloudExadataInfrastructureCompartment} operation to move an Exadata
* infrastructure resource to a different compartment and {@link
* #changeCloudAutonomousVmClusterCompartment(ChangeCloudAutonomousVmClusterCompartmentRequest,
* Consumer, Consumer) changeCloudAutonomousVmClusterCompartment} operation to move an
* Autonomous Exadata VM cluster to a different compartment. For more information, see [Moving
* Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeAutonomousExadataInfrastructureCompartment(
ChangeAutonomousExadataInfrastructureCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeAutonomousExadataInfrastructureCompartmentRequest,
ChangeAutonomousExadataInfrastructureCompartmentResponse>
handler);
/**
* Moves an Autonomous VM cluster and its dependent resources to another compartment. Applies to
* Exadata Cloud@Customer only. For systems in the Oracle cloud, see {@link
* #changeAutonomousVmClusterCompartment(ChangeAutonomousVmClusterCompartmentRequest, Consumer,
* Consumer) changeAutonomousVmClusterCompartment}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeAutonomousVmClusterCompartment(
ChangeAutonomousVmClusterCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeAutonomousVmClusterCompartmentRequest,
ChangeAutonomousVmClusterCompartmentResponse>
handler);
/**
* Move the backup destination and its dependent resources to the specified compartment. For
* more information, see [Moving Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeBackupDestinationCompartment(
ChangeBackupDestinationCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeBackupDestinationCompartmentRequest,
ChangeBackupDestinationCompartmentResponse>
handler);
/**
* Moves an Autonomous Exadata VM cluster in the Oracle cloud and its dependent resources to
* another compartment. For Exadata Cloud@Customer systems, see {@link
* #changeAutonomousVmClusterCompartment(ChangeAutonomousVmClusterCompartmentRequest, Consumer,
* Consumer) changeAutonomousVmClusterCompartment}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeCloudAutonomousVmClusterCompartment(
ChangeCloudAutonomousVmClusterCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeCloudAutonomousVmClusterCompartmentRequest,
ChangeCloudAutonomousVmClusterCompartmentResponse>
handler);
/**
* Moves a cloud Exadata infrastructure resource and its dependent resources to another
* compartment. Applies to Exadata Cloud Service instances and Autonomous Database on dedicated
* Exadata infrastructure only.For more information about moving resources to a different
* compartment, see [Moving Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeCloudExadataInfrastructureCompartment(
ChangeCloudExadataInfrastructureCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeCloudExadataInfrastructureCompartmentRequest,
ChangeCloudExadataInfrastructureCompartmentResponse>
handler);
/**
* Associate a cloud Exadata infrastructure with a different subscription.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeCloudExadataInfrastructureSubscription(
ChangeCloudExadataInfrastructureSubscriptionRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeCloudExadataInfrastructureSubscriptionRequest,
ChangeCloudExadataInfrastructureSubscriptionResponse>
handler);
/**
* Moves a cloud VM cluster and its dependent resources to another compartment. Applies to
* Exadata Cloud Service instances and Autonomous Database on dedicated Exadata infrastructure
* only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeCloudVmClusterCompartment(
ChangeCloudVmClusterCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeCloudVmClusterCompartmentRequest,
ChangeCloudVmClusterCompartmentResponse>
handler);
/**
* Associate a cloud VM cluster with a different subscription.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeCloudVmClusterSubscription(
ChangeCloudVmClusterSubscriptionRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeCloudVmClusterSubscriptionRequest,
ChangeCloudVmClusterSubscriptionResponse>
handler);
/**
* Move the Database Software Image and its dependent resources to the specified compartment.
* For more information about moving Databse Software Images, see [Moving Database Resources to
* a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeDatabaseSoftwareImageCompartment(
ChangeDatabaseSoftwareImageCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeDatabaseSoftwareImageCompartmentRequest,
ChangeDatabaseSoftwareImageCompartmentResponse>
handler);
/**
* Switch the Autonomous Container Database role between Standby and Snapshot Standby. For more
* information about changing Autonomous Container Databases Dataguard Role, see [Convert
* Physical Standby to Snapshot
* Standby](https://docs.oracle.com/en/cloud/paas/autonomous-database/dedicated/adbcl/index.html#ADBCL-GUID-D3B503F1-0032-4B0D-9F00-ACAE8151AB80)
* and [Convert Snapshot Standby to Physical
* Standby](https://docs.oracle.com/en/cloud/paas/autonomous-database/dedicated/adbcl/index.html#ADBCL-GUID-E8D7E0EE-8244-467D-B33A-1BC6F969A0A4).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeDataguardRole(
ChangeDataguardRoleRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeDataguardRoleRequest, ChangeDataguardRoleResponse>
handler);
/**
* Moves the DB system and its dependent resources to the specified compartment. For more
* information about moving DB systems, see [Moving Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeDbSystemCompartment(
ChangeDbSystemCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeDbSystemCompartmentRequest, ChangeDbSystemCompartmentResponse>
handler);
/**
* This operation updates the cross-region disaster recovery (DR) details of the standby
* Autonomous Database Serverless database, and must be run on the standby side.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeDisasterRecoveryConfiguration(
ChangeDisasterRecoveryConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeDisasterRecoveryConfigurationRequest,
ChangeDisasterRecoveryConfigurationResponse>
handler);
/**
* Moves an Exadata infrastructure resource and its dependent resources to another compartment.
* Applies to Exadata Cloud@Customer instances only. To move an Exadata Cloud Service
* infrastructure resource to another compartment, use the {@link
* #changeCloudExadataInfrastructureCompartment(ChangeCloudExadataInfrastructureCompartmentRequest,
* Consumer, Consumer) changeCloudExadataInfrastructureCompartment} operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeExadataInfrastructureCompartment(
ChangeExadataInfrastructureCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeExadataInfrastructureCompartmentRequest,
ChangeExadataInfrastructureCompartmentResponse>
handler);
/**
* Moves a Exadata VM cluster on Exascale Infrastructure and its dependent resources to another
* compartment. Applies to Exadata Database Service on Exascale Infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeExadbVmClusterCompartment(
ChangeExadbVmClusterCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeExadbVmClusterCompartmentRequest,
ChangeExadbVmClusterCompartmentResponse>
handler);
/**
* Moves a Exadata Database Storage Vault to another compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeExascaleDbStorageVaultCompartment(
ChangeExascaleDbStorageVaultCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeExascaleDbStorageVaultCompartmentRequest,
ChangeExascaleDbStorageVaultCompartmentResponse>
handler);
/**
* Move the {@link
* #createExternalContainerDatabaseDetails(CreateExternalContainerDatabaseDetailsRequest,
* Consumer, Consumer) createExternalContainerDatabaseDetails} and its dependent resources to
* the specified compartment. For more information about moving external container databases,
* see [Moving Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeExternalContainerDatabaseCompartment(
ChangeExternalContainerDatabaseCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeExternalContainerDatabaseCompartmentRequest,
ChangeExternalContainerDatabaseCompartmentResponse>
handler);
/**
* Move the external non-container database and its dependent resources to the specified
* compartment. For more information about moving external non-container databases, see [Moving
* Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeExternalNonContainerDatabaseCompartment(
ChangeExternalNonContainerDatabaseCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeExternalNonContainerDatabaseCompartmentRequest,
ChangeExternalNonContainerDatabaseCompartmentResponse>
handler);
/**
* Move the {@link
* #createExternalPluggableDatabaseDetails(CreateExternalPluggableDatabaseDetailsRequest,
* Consumer, Consumer) createExternalPluggableDatabaseDetails} and its dependent resources to
* the specified compartment. For more information about moving external pluggable databases,
* see [Moving Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeExternalPluggableDatabaseCompartment(
ChangeExternalPluggableDatabaseCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeExternalPluggableDatabaseCompartmentRequest,
ChangeExternalPluggableDatabaseCompartmentResponse>
handler);
/**
* Move the key store resource to the specified compartment. For more information about moving
* key stores, see [Moving Database Resources to a Different
* Compartment](https://docs.cloud.oracle.com/Content/Database/Concepts/databaseoverview.htm#moveRes).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeKeyStoreCompartment(
ChangeKeyStoreCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeKeyStoreCompartmentRequest, ChangeKeyStoreCompartmentResponse>
handler);
/**
* Changes encryption key management type
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeKeyStoreType(
ChangeKeyStoreTypeRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeKeyStoreTypeRequest, ChangeKeyStoreTypeResponse>
handler);
/**
* Move the one-off patch to the specified compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeOneoffPatchCompartment(
ChangeOneoffPatchCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeOneoffPatchCompartmentRequest,
ChangeOneoffPatchCompartmentResponse>
handler);
/**
* Moves an scheduling plan resource to another compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeSchedulingPlanCompartment(
ChangeSchedulingPlanCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeSchedulingPlanCompartmentRequest,
ChangeSchedulingPlanCompartmentResponse>
handler);
/**
* Moves an scheduling policy resource to another compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
changeSchedulingPolicyCompartment(
ChangeSchedulingPolicyCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeSchedulingPolicyCompartmentRequest,
ChangeSchedulingPolicyCompartmentResponse>
handler);
/**
* Moves a VM cluster and its dependent resources to another compartment. Applies to Exadata
* Cloud@Customer instances only. To move a cloud VM cluster in an Exadata Cloud Service
* instance to another compartment, use the {@link
* #changeCloudVmClusterCompartment(ChangeCloudVmClusterCompartmentRequest, Consumer, Consumer)
* changeCloudVmClusterCompartment} operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeVmClusterCompartment(
ChangeVmClusterCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeVmClusterCompartmentRequest, ChangeVmClusterCompartmentResponse>
handler);
/**
* Check the status of the external database connection specified in this connector. This
* operation will refresh the connectionStatus and timeConnectionStatusLastUpdated fields.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
checkExternalDatabaseConnectorConnectionStatus(
CheckExternalDatabaseConnectorConnectionStatusRequest request,
com.oracle.bmc.responses.AsyncHandler<
CheckExternalDatabaseConnectorConnectionStatusRequest,
CheckExternalDatabaseConnectorConnectionStatusResponse>
handler);
/**
* Changes the status of the standalone backup resource to `ACTIVE` after the backup is created
* from the on-premises database and placed in Oracle Cloud Infrastructure Object Storage.
*
* *Note:** This API is used by an Oracle Cloud Infrastructure Python script that is packaged
* with the Oracle Cloud Infrastructure CLI. Oracle recommends that you use the script instead
* using the API directly. See [Migrating an On-Premises Database to Oracle Cloud Infrastructure
* by Creating a Backup in the
* Cloud](https://docs.cloud.oracle.com/Content/Database/Tasks/mig-onprembackup.htm) for more
* information.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future completeExternalBackupJob(
CompleteExternalBackupJobRequest request,
com.oracle.bmc.responses.AsyncHandler<
CompleteExternalBackupJobRequest, CompleteExternalBackupJobResponse>
handler);
/**
* Configures the Autonomous Database Vault service
* [key](https://docs.cloud.oracle.com/Content/KeyManagement/Concepts/keyoverview.htm#concepts).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
configureAutonomousDatabaseVaultKey(
ConfigureAutonomousDatabaseVaultKeyRequest request,
com.oracle.bmc.responses.AsyncHandler<
ConfigureAutonomousDatabaseVaultKeyRequest,
ConfigureAutonomousDatabaseVaultKeyResponse>
handler);
/**
* This operation updates SaaS administrative user configuration of the Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future configureSaasAdminUser(
ConfigureSaasAdminUserRequest request,
com.oracle.bmc.responses.AsyncHandler<
ConfigureSaasAdminUserRequest, ConfigureSaasAdminUserResponse>
handler);
/**
* This is for user to confirm to DBaaS that the Oracle Key Valut (OKV) connection IPs, username
* and password are all correct. This operation will put the Key Store back into Active state.
* If details are incorrect, your OKV account may get locked after some unsuccessful attempts to
* connect.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
confirmKeyStoreDetailsAreCorrect(
ConfirmKeyStoreDetailsAreCorrectRequest request,
com.oracle.bmc.responses.AsyncHandler<
ConfirmKeyStoreDetailsAreCorrectRequest,
ConfirmKeyStoreDetailsAreCorrectResponse>
handler);
/**
* Converts a non-container database to a pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future convertToPdb(
ConvertToPdbRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Converts a Refreshable clone to Regular pluggable database (PDB). Pluggable Database will be
* in `READ_WRITE` openmode after conversion.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
convertToRegularPluggableDatabase(
ConvertToRegularPluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
ConvertToRegularPluggableDatabaseRequest,
ConvertToRegularPluggableDatabaseResponse>
handler);
/**
* Creates a new application virtual IP (VIP) address in the specified cloud VM cluster based on
* the request parameters you provide.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createApplicationVip(
CreateApplicationVipRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateApplicationVipRequest, CreateApplicationVipResponse>
handler);
/**
* Creates an Autonomous Container Database in the specified Autonomous Exadata Infrastructure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createAutonomousContainerDatabase(
CreateAutonomousContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateAutonomousContainerDatabaseRequest,
CreateAutonomousContainerDatabaseResponse>
handler);
/**
* Create a new Autonomous Data Guard association. An Autonomous Data Guard association
* represents the replication relationship between the specified Autonomous Container database
* and a peer Autonomous Container database. For more information, see [Using Oracle Data
* Guard](https://docs.cloud.oracle.com/Content/Database/Tasks/usingdataguard.htm).
*
* All Oracle Cloud Infrastructure resources, including Data Guard associations, get an
* Oracle-assigned, unique ID called an Oracle Cloud Identifier (OCID). When you create a
* resource, you can find its OCID in the response. You can also retrieve a resource's OCID by
* using a List API operation on that resource type, or by viewing the resource in the Console.
* For more information, see [Resource
* Identifiers](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createAutonomousContainerDatabaseDataguardAssociation(
CreateAutonomousContainerDatabaseDataguardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateAutonomousContainerDatabaseDataguardAssociationRequest,
CreateAutonomousContainerDatabaseDataguardAssociationResponse>
handler);
/**
* Creates a new Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createAutonomousDatabase(
CreateAutonomousDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateAutonomousDatabaseRequest, CreateAutonomousDatabaseResponse>
handler);
/**
* Creates a new Autonomous Database backup for the specified database based on the provided
* request parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createAutonomousDatabaseBackup(
CreateAutonomousDatabaseBackupRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateAutonomousDatabaseBackupRequest,
CreateAutonomousDatabaseBackupResponse>
handler);
/**
* create Autonomous Database Software Image in the specified compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createAutonomousDatabaseSoftwareImage(
CreateAutonomousDatabaseSoftwareImageRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateAutonomousDatabaseSoftwareImageRequest,
CreateAutonomousDatabaseSoftwareImageResponse>
handler);
/**
* Creates an Autonomous VM cluster for Exadata Cloud@Customer. To create an Autonomous VM
* Cluster in the Oracle cloud, see {@link
* #createCloudAutonomousVmCluster(CreateCloudAutonomousVmClusterRequest, Consumer, Consumer)
* createCloudAutonomousVmCluster}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createAutonomousVmCluster(
CreateAutonomousVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateAutonomousVmClusterRequest, CreateAutonomousVmClusterResponse>
handler);
/**
* Creates a new backup in the specified database based on the request parameters you provide.
* If you previously used RMAN or dbcli to configure backups and then you switch to using the
* Console or the API for backups, a new backup configuration is created and associated with
* your database. This means that you can no longer rely on your previously configured unmanaged
* backups to work.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createBackup(
CreateBackupRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Creates a backup destination in an Exadata Cloud@Customer system.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createBackupDestination(
CreateBackupDestinationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateBackupDestinationRequest, CreateBackupDestinationResponse>
handler);
/**
* Creates an Autonomous Exadata VM cluster in the Oracle cloud. For Exadata Cloud@Customer
* systems, see {@link #createAutonomousVmCluster(CreateAutonomousVmClusterRequest, Consumer,
* Consumer) createAutonomousVmCluster}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createCloudAutonomousVmCluster(
CreateCloudAutonomousVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateCloudAutonomousVmClusterRequest,
CreateCloudAutonomousVmClusterResponse>
handler);
/**
* Creates a cloud Exadata infrastructure resource. This resource is used to create either an
* [Exadata Cloud
* Service](https://docs.cloud.oracle.com/Content/Database/Concepts/exaoverview.htm) instance or
* an Autonomous Database on dedicated Exadata infrastructure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createCloudExadataInfrastructure(
CreateCloudExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateCloudExadataInfrastructureRequest,
CreateCloudExadataInfrastructureResponse>
handler);
/**
* Creates a cloud VM cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createCloudVmCluster(
CreateCloudVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateCloudVmClusterRequest, CreateCloudVmClusterResponse>
handler);
/**
* Creates a new console connection to the specified database node. After the console connection
* has been created and is available, you connect to the console using SSH.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createConsoleConnection(
CreateConsoleConnectionRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateConsoleConnectionRequest, CreateConsoleConnectionResponse>
handler);
/**
* Captures the most recent serial console data (up to a megabyte) for the specified database
* node.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createConsoleHistory(
CreateConsoleHistoryRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateConsoleHistoryRequest, CreateConsoleHistoryResponse>
handler);
/**
* Creates a new Data Guard association. A Data Guard association represents the replication
* relationship between the specified database and a peer database. For more information, see
* [Using Oracle Data
* Guard](https://docs.cloud.oracle.com/Content/Database/Tasks/usingdataguard.htm).
*
* All Oracle Cloud Infrastructure resources, including Data Guard associations, get an
* Oracle-assigned, unique ID called an Oracle Cloud Identifier (OCID). When you create a
* resource, you can find its OCID in the response. You can also retrieve a resource's OCID by
* using a List API operation on that resource type, or by viewing the resource in the Console.
* For more information, see [Resource
* Identifiers](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createDataGuardAssociation(
CreateDataGuardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateDataGuardAssociationRequest, CreateDataGuardAssociationResponse>
handler);
/**
* Creates a new database in the specified Database Home. If the database version is provided,
* it must match the version of the Database Home. Applies to Exadata and Exadata Cloud@Customer
* systems.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createDatabase(
CreateDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* create database software image in the specified compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createDatabaseSoftwareImage(
CreateDatabaseSoftwareImageRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateDatabaseSoftwareImageRequest, CreateDatabaseSoftwareImageResponse>
handler);
/**
* Creates a new Database Home in the specified database system based on the request parameters
* you provide. Applies to bare metal DB systems, Exadata systems, and Exadata Cloud@Customer
* systems.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createDbHome(
CreateDbHomeRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Creates an Exadata infrastructure resource. Applies to Exadata Cloud@Customer instances only.
* To create an Exadata Cloud Service infrastructure resource, use the {@link
* #createCloudExadataInfrastructure(CreateCloudExadataInfrastructureRequest, Consumer,
* Consumer) createCloudExadataInfrastructure} operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createExadataInfrastructure(
CreateExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExadataInfrastructureRequest, CreateExadataInfrastructureResponse>
handler);
/**
* Creates an Exadata VM cluster on Exascale Infrastructure
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createExadbVmCluster(
CreateExadbVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExadbVmClusterRequest, CreateExadbVmClusterResponse>
handler);
/**
* Creates an Exadata Database Storage Vault
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createExascaleDbStorageVault(
CreateExascaleDbStorageVaultRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExascaleDbStorageVaultRequest,
CreateExascaleDbStorageVaultResponse>
handler);
/**
* Creates an execution action resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createExecutionAction(
CreateExecutionActionRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExecutionActionRequest, CreateExecutionActionResponse>
handler);
/**
* Creates an execution window resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createExecutionWindow(
CreateExecutionWindowRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExecutionWindowRequest, CreateExecutionWindowResponse>
handler);
/**
* Creates a new backup resource and returns the information the caller needs to back up an
* on-premises Oracle Database to Oracle Cloud Infrastructure.
*
* *Note:** This API is used by an Oracle Cloud Infrastructure Python script that is packaged
* with the Oracle Cloud Infrastructure CLI. Oracle recommends that you use the script instead
* using the API directly. See [Migrating an On-Premises Database to Oracle Cloud Infrastructure
* by Creating a Backup in the
* Cloud](https://docs.cloud.oracle.com/Content/Database/Tasks/mig-onprembackup.htm) for more
* information.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createExternalBackupJob(
CreateExternalBackupJobRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExternalBackupJobRequest, CreateExternalBackupJobResponse>
handler);
/**
* Creates a new external container database resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createExternalContainerDatabase(
CreateExternalContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExternalContainerDatabaseRequest,
CreateExternalContainerDatabaseResponse>
handler);
/**
* Creates a new external database connector.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createExternalDatabaseConnector(
CreateExternalDatabaseConnectorRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExternalDatabaseConnectorRequest,
CreateExternalDatabaseConnectorResponse>
handler);
/**
* Creates a new ExternalNonContainerDatabase resource
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createExternalNonContainerDatabase(
CreateExternalNonContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExternalNonContainerDatabaseRequest,
CreateExternalNonContainerDatabaseResponse>
handler);
/**
* Registers a new {@link
* #createExternalPluggableDatabaseDetails(CreateExternalPluggableDatabaseDetailsRequest,
* Consumer, Consumer) createExternalPluggableDatabaseDetails} resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createExternalPluggableDatabase(
CreateExternalPluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateExternalPluggableDatabaseRequest,
CreateExternalPluggableDatabaseResponse>
handler);
/**
* Creates a Key Store.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createKeyStore(
CreateKeyStoreRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Creates a maintenance run with one of the following: 1. The latest available release update
* patch (RUP) for the Autonomous Container Database. 2. The latest available RUP and DST
* time-zone (TZ) file updates for the Autonomous Container Database. 3. The DST TZ file updates
* for the Autonomous Container Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createMaintenanceRun(
CreateMaintenanceRunRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateMaintenanceRunRequest, CreateMaintenanceRunResponse>
handler);
/**
* Creates one-off patch for specified database version to download.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createOneoffPatch(
CreateOneoffPatchRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateOneoffPatchRequest, CreateOneoffPatchResponse>
handler);
/**
* Creates and starts a pluggable database in the specified container database. Pluggable
* Database can be created using different operations (e.g. LocalClone, RemoteClone, Relocate )
* with this API. Use the {@link #startPluggableDatabase(StartPluggableDatabaseRequest,
* Consumer, Consumer) startPluggableDatabase} and {@link
* #stopPluggableDatabase(StopPluggableDatabaseRequest, Consumer, Consumer)
* stopPluggableDatabase} APIs to start and stop the pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createPluggableDatabase(
CreatePluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreatePluggableDatabaseRequest, CreatePluggableDatabaseResponse>
handler);
/**
* Creates a Scheduled Action resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createScheduledAction(
CreateScheduledActionRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateScheduledActionRequest, CreateScheduledActionResponse>
handler);
/**
* Creates a Scheduling Plan resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createSchedulingPlan(
CreateSchedulingPlanRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateSchedulingPlanRequest, CreateSchedulingPlanResponse>
handler);
/**
* Creates a Scheduling Policy resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createSchedulingPolicy(
CreateSchedulingPolicyRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateSchedulingPolicyRequest, CreateSchedulingPolicyResponse>
handler);
/**
* Creates a Scheduling Window resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createSchedulingWindow(
CreateSchedulingWindowRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateSchedulingWindowRequest, CreateSchedulingWindowResponse>
handler);
/**
* Creates an Exadata Cloud@Customer VM cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createVmCluster(
CreateVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Creates the VM cluster network. Applies to Exadata Cloud@Customer instances only. To create a
* cloud VM cluster in an Exadata Cloud Service instance, use the {@link
* #createCloudVmCluster(CreateCloudVmClusterRequest, Consumer, Consumer) createCloudVmCluster}
* operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createVmClusterNetwork(
CreateVmClusterNetworkRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateVmClusterNetworkRequest, CreateVmClusterNetworkResponse>
handler);
/**
* Performs one of the following power actions on the specified DB node: - start - power on -
* stop - power off - softreset - ACPI shutdown and power on - reset - power off and power on
*
* *Note:** Stopping a node affects billing differently, depending on the type of DB system:
* *Bare metal and Exadata systems* - The _stop_ state has no effect on the resources you
* consume. Billing continues for DB nodes that you stop, and related resources continue to
* apply against any relevant quotas. You must terminate the DB system ({@link
* #terminateDbSystem(TerminateDbSystemRequest, Consumer, Consumer) terminateDbSystem}) to
* remove its resources from billing and quotas. *Virtual machine DB systems* - Stopping a node
* stops billing for all OCPUs associated with that node, and billing resumes when you restart
* the node.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future dbNodeAction(
DbNodeActionRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes and deregisters the specified application virtual IP (VIP) address.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteApplicationVip(
DeleteApplicationVipRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteApplicationVipRequest, DeleteApplicationVipResponse>
handler);
/**
* Deletes the specified Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteAutonomousDatabase(
DeleteAutonomousDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteAutonomousDatabaseRequest, DeleteAutonomousDatabaseResponse>
handler);
/**
* Deletes a long-term backup. You cannot delete other backups using this API.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteAutonomousDatabaseBackup(
DeleteAutonomousDatabaseBackupRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteAutonomousDatabaseBackupRequest,
DeleteAutonomousDatabaseBackupResponse>
handler);
/**
* Delete an Autonomous Database Software Image
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteAutonomousDatabaseSoftwareImage(
DeleteAutonomousDatabaseSoftwareImageRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteAutonomousDatabaseSoftwareImageRequest,
DeleteAutonomousDatabaseSoftwareImageResponse>
handler);
/**
* Deletes the specified Autonomous VM cluster in an Exadata Cloud@Customer system. To delete an
* Autonomous VM Cluster in the Oracle cloud, see {@link
* #deleteCloudAutonomousVmCluster(DeleteCloudAutonomousVmClusterRequest, Consumer, Consumer)
* deleteCloudAutonomousVmCluster}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteAutonomousVmCluster(
DeleteAutonomousVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteAutonomousVmClusterRequest, DeleteAutonomousVmClusterResponse>
handler);
/**
* Deletes a full backup. You cannot delete automatic backups using this API.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteBackup(
DeleteBackupRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes a backup destination in an Exadata Cloud@Customer system.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteBackupDestination(
DeleteBackupDestinationRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteBackupDestinationRequest, DeleteBackupDestinationResponse>
handler);
/**
* Deletes the specified Autonomous Exadata VM cluster in the Oracle cloud. For Exadata
* Cloud@Customer systems, see {@link
* #deleteAutonomousVmCluster(DeleteAutonomousVmClusterRequest, Consumer, Consumer)
* deleteAutonomousVmCluster}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteCloudAutonomousVmCluster(
DeleteCloudAutonomousVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteCloudAutonomousVmClusterRequest,
DeleteCloudAutonomousVmClusterResponse>
handler);
/**
* Deletes the cloud Exadata infrastructure resource. Applies to Exadata Cloud Service instances
* and Autonomous Database on dedicated Exadata infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteCloudExadataInfrastructure(
DeleteCloudExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteCloudExadataInfrastructureRequest,
DeleteCloudExadataInfrastructureResponse>
handler);
/**
* Deletes the specified cloud VM cluster. Applies to Exadata Cloud Service instances and
* Autonomous Database on dedicated Exadata infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteCloudVmCluster(
DeleteCloudVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteCloudVmClusterRequest, DeleteCloudVmClusterResponse>
handler);
/**
* Deletes the specified database node console connection.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteConsoleConnection(
DeleteConsoleConnectionRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteConsoleConnectionRequest, DeleteConsoleConnectionResponse>
handler);
/**
* Deletes the specified database node console history.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteConsoleHistory(
DeleteConsoleHistoryRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteConsoleHistoryRequest, DeleteConsoleHistoryResponse>
handler);
/**
* Deletes the specified database. Applies only to Exadata systems.
*
* The data in this database is local to the Exadata system and will be lost when the
* database is deleted. Oracle recommends that you back up any data in the Exadata system prior
* to deleting it. You can use the `performFinalBackup` parameter to have the Exadata system
* database backed up before it is deleted.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteDatabase(
DeleteDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Delete a database software image
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteDatabaseSoftwareImage(
DeleteDatabaseSoftwareImageRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteDatabaseSoftwareImageRequest, DeleteDatabaseSoftwareImageResponse>
handler);
/**
* Deletes a Database Home. Applies to bare metal DB systems, Exadata Cloud Service, and Exadata
* Cloud@Customer systems.
*
* Oracle recommends that you use the `performFinalBackup` parameter to back up any data on a
* bare metal DB system before you delete a Database Home. On an Exadata Cloud@Customer system
* or an Exadata Cloud Service system, you can delete a Database Home only when there are no
* databases in it and therefore you cannot use the `performFinalBackup` parameter to back up
* data.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteDbHome(
DeleteDbHomeRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes the Exadata Cloud@Customer infrastructure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteExadataInfrastructure(
DeleteExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExadataInfrastructureRequest, DeleteExadataInfrastructureResponse>
handler);
/**
* Deletes the specified Exadata VM cluster on Exascale Infrastructure. Applies to Exadata
* Database Service on Exascale Infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteExadbVmCluster(
DeleteExadbVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExadbVmClusterRequest, DeleteExadbVmClusterResponse>
handler);
/**
* Deletes the specified Exadata Database Storage Vault.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteExascaleDbStorageVault(
DeleteExascaleDbStorageVaultRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExascaleDbStorageVaultRequest,
DeleteExascaleDbStorageVaultResponse>
handler);
/**
* Deletes the execution action.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteExecutionAction(
DeleteExecutionActionRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExecutionActionRequest, DeleteExecutionActionResponse>
handler);
/**
* Deletes the execution window.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteExecutionWindow(
DeleteExecutionWindowRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExecutionWindowRequest, DeleteExecutionWindowResponse>
handler);
/**
* Deletes the {@link
* #createExternalContainerDatabaseDetails(CreateExternalContainerDatabaseDetailsRequest,
* Consumer, Consumer) createExternalContainerDatabaseDetails} resource. Any external pluggable
* databases registered under this container database must be deleted in your Oracle Cloud
* Infrastructure tenancy prior to this operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteExternalContainerDatabase(
DeleteExternalContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExternalContainerDatabaseRequest,
DeleteExternalContainerDatabaseResponse>
handler);
/**
* Deletes an external database connector. Any services enabled using the external database
* connector must be deleted prior to this operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteExternalDatabaseConnector(
DeleteExternalDatabaseConnectorRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExternalDatabaseConnectorRequest,
DeleteExternalDatabaseConnectorResponse>
handler);
/**
* Deletes the Oracle Cloud Infrastructure resource representing an external non-container
* database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteExternalNonContainerDatabase(
DeleteExternalNonContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExternalNonContainerDatabaseRequest,
DeleteExternalNonContainerDatabaseResponse>
handler);
/**
* Deletes the {@link
* #createExternalPluggableDatabaseDetails(CreateExternalPluggableDatabaseDetailsRequest,
* Consumer, Consumer) createExternalPluggableDatabaseDetails}. resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteExternalPluggableDatabase(
DeleteExternalPluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteExternalPluggableDatabaseRequest,
DeleteExternalPluggableDatabaseResponse>
handler);
/**
* Deletes a key store.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteKeyStore(
DeleteKeyStoreRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes a one-off patch.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteOneoffPatch(
DeleteOneoffPatchRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteOneoffPatchRequest, DeleteOneoffPatchResponse>
handler);
/**
* Deletes the specified pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deletePluggableDatabase(
DeletePluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeletePluggableDatabaseRequest, DeletePluggableDatabaseResponse>
handler);
/**
* Deletes the scheduled action.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteScheduledAction(
DeleteScheduledActionRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteScheduledActionRequest, DeleteScheduledActionResponse>
handler);
/**
* Deletes the scheduling plan.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteSchedulingPlan(
DeleteSchedulingPlanRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteSchedulingPlanRequest, DeleteSchedulingPlanResponse>
handler);
/**
* Deletes the scheduling policy.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteSchedulingPolicy(
DeleteSchedulingPolicyRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteSchedulingPolicyRequest, DeleteSchedulingPolicyResponse>
handler);
/**
* Deletes the scheduling window.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteSchedulingWindow(
DeleteSchedulingWindowRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteSchedulingWindowRequest, DeleteSchedulingWindowResponse>
handler);
/**
* Deletes the specified VM cluster. Applies to Exadata Cloud@Customer instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteVmCluster(
DeleteVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes the specified VM cluster network. Applies to Exadata Cloud@Customer instances only.
* To delete a cloud VM cluster in an Exadata Cloud Service instance, use the {@link
* #deleteCloudVmCluster(DeleteCloudVmClusterRequest, Consumer, Consumer) deleteCloudVmCluster}
* operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteVmClusterNetwork(
DeleteVmClusterNetworkRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteVmClusterNetworkRequest, DeleteVmClusterNetworkResponse>
handler);
/**
* Asynchronously deregisters this Autonomous Database with Data Safe.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deregisterAutonomousDatabaseDataSafe(
DeregisterAutonomousDatabaseDataSafeRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeregisterAutonomousDatabaseDataSafeRequest,
DeregisterAutonomousDatabaseDataSafeResponse>
handler);
/**
* Disables Database Management for the Autonomous Database resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableAutonomousDatabaseManagement(
DisableAutonomousDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableAutonomousDatabaseManagementRequest,
DisableAutonomousDatabaseManagementResponse>
handler);
/**
* Disables Operations Insights for the Autonomous Database resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableAutonomousDatabaseOperationsInsights(
DisableAutonomousDatabaseOperationsInsightsRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableAutonomousDatabaseOperationsInsightsRequest,
DisableAutonomousDatabaseOperationsInsightsResponse>
handler);
/**
* Disables the Database Management service for the database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future disableDatabaseManagement(
DisableDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableDatabaseManagementRequest, DisableDatabaseManagementResponse>
handler);
/**
* Disable Database Management service for the external container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalContainerDatabaseDatabaseManagement(
DisableExternalContainerDatabaseDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalContainerDatabaseDatabaseManagementRequest,
DisableExternalContainerDatabaseDatabaseManagementResponse>
handler);
/**
* Disable Stack Monitoring for the external container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalContainerDatabaseStackMonitoring(
DisableExternalContainerDatabaseStackMonitoringRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalContainerDatabaseStackMonitoringRequest,
DisableExternalContainerDatabaseStackMonitoringResponse>
handler);
/**
* Disable Database Management Service for the external non-container database. For more
* information about the Database Management Service, see [Database Management
* Service](https://docs.cloud.oracle.com/Content/ExternalDatabase/Concepts/databasemanagementservice.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalNonContainerDatabaseDatabaseManagement(
DisableExternalNonContainerDatabaseDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalNonContainerDatabaseDatabaseManagementRequest,
DisableExternalNonContainerDatabaseDatabaseManagementResponse>
handler);
/**
* Disable Operations Insights for the external non-container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalNonContainerDatabaseOperationsInsights(
DisableExternalNonContainerDatabaseOperationsInsightsRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalNonContainerDatabaseOperationsInsightsRequest,
DisableExternalNonContainerDatabaseOperationsInsightsResponse>
handler);
/**
* Disable Stack Monitoring for the external non-container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalNonContainerDatabaseStackMonitoring(
DisableExternalNonContainerDatabaseStackMonitoringRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalNonContainerDatabaseStackMonitoringRequest,
DisableExternalNonContainerDatabaseStackMonitoringResponse>
handler);
/**
* Disable Database Management Service for the external pluggable database. For more information
* about the Database Management Service, see [Database Management
* Service](https://docs.cloud.oracle.com/Content/ExternalDatabase/Concepts/databasemanagementservice.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalPluggableDatabaseDatabaseManagement(
DisableExternalPluggableDatabaseDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalPluggableDatabaseDatabaseManagementRequest,
DisableExternalPluggableDatabaseDatabaseManagementResponse>
handler);
/**
* Disable Operations Insights for the external pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalPluggableDatabaseOperationsInsights(
DisableExternalPluggableDatabaseOperationsInsightsRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalPluggableDatabaseOperationsInsightsRequest,
DisableExternalPluggableDatabaseOperationsInsightsResponse>
handler);
/**
* Disable Stack Monitoring for the external pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disableExternalPluggableDatabaseStackMonitoring(
DisableExternalPluggableDatabaseStackMonitoringRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableExternalPluggableDatabaseStackMonitoringRequest,
DisableExternalPluggableDatabaseStackMonitoringResponse>
handler);
/**
* Disables the Database Management service for the pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
disablePluggableDatabaseManagement(
DisablePluggableDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisablePluggableDatabaseManagementRequest,
DisablePluggableDatabaseManagementResponse>
handler);
/**
* Downloads the configuration file for the specified Exadata Cloud@Customer infrastructure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
downloadExadataInfrastructureConfigFile(
DownloadExadataInfrastructureConfigFileRequest request,
com.oracle.bmc.responses.AsyncHandler<
DownloadExadataInfrastructureConfigFileRequest,
DownloadExadataInfrastructureConfigFileResponse>
handler);
/**
* Download one-off patch.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future downloadOneoffPatch(
DownloadOneoffPatchRequest request,
com.oracle.bmc.responses.AsyncHandler<
DownloadOneoffPatchRequest, DownloadOneoffPatchResponse>
handler);
/**
* Downloads the network validation report file for the specified VM cluster network. Applies to
* Exadata Cloud@Customer instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future downloadValidationReport(
DownloadValidationReportRequest request,
com.oracle.bmc.responses.AsyncHandler<
DownloadValidationReportRequest, DownloadValidationReportResponse>
handler);
/**
* Downloads the configuration file for the specified VM cluster network. Applies to Exadata
* Cloud@Customer instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
downloadVmClusterNetworkConfigFile(
DownloadVmClusterNetworkConfigFileRequest request,
com.oracle.bmc.responses.AsyncHandler<
DownloadVmClusterNetworkConfigFileRequest,
DownloadVmClusterNetworkConfigFileResponse>
handler);
/**
* Enables Database Management for Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableAutonomousDatabaseManagement(
EnableAutonomousDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableAutonomousDatabaseManagementRequest,
EnableAutonomousDatabaseManagementResponse>
handler);
/**
* Enables the specified Autonomous Database with Operations Insights.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableAutonomousDatabaseOperationsInsights(
EnableAutonomousDatabaseOperationsInsightsRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableAutonomousDatabaseOperationsInsightsRequest,
EnableAutonomousDatabaseOperationsInsightsResponse>
handler);
/**
* Enables the Database Management service for an Oracle Database located in Oracle Cloud
* Infrastructure. This service allows the database to access tools including Metrics and
* Performance hub. Database Management is enabled at the container database (CDB) level.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future enableDatabaseManagement(
EnableDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableDatabaseManagementRequest, EnableDatabaseManagementResponse>
handler);
/**
* Enables Database Management Service for the external container database. For more information
* about the Database Management Service, see [Database Management
* Service](https://docs.cloud.oracle.com/Content/ExternalDatabase/Concepts/databasemanagementservice.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalContainerDatabaseDatabaseManagement(
EnableExternalContainerDatabaseDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalContainerDatabaseDatabaseManagementRequest,
EnableExternalContainerDatabaseDatabaseManagementResponse>
handler);
/**
* Enable Stack Monitoring for the external container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalContainerDatabaseStackMonitoring(
EnableExternalContainerDatabaseStackMonitoringRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalContainerDatabaseStackMonitoringRequest,
EnableExternalContainerDatabaseStackMonitoringResponse>
handler);
/**
* Enable Database Management Service for the external non-container database. For more
* information about the Database Management Service, see [Database Management
* Service](https://docs.cloud.oracle.com/Content/ExternalDatabase/Concepts/databasemanagementservice.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalNonContainerDatabaseDatabaseManagement(
EnableExternalNonContainerDatabaseDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalNonContainerDatabaseDatabaseManagementRequest,
EnableExternalNonContainerDatabaseDatabaseManagementResponse>
handler);
/**
* Enable Operations Insights for the external non-container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalNonContainerDatabaseOperationsInsights(
EnableExternalNonContainerDatabaseOperationsInsightsRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalNonContainerDatabaseOperationsInsightsRequest,
EnableExternalNonContainerDatabaseOperationsInsightsResponse>
handler);
/**
* Enable Stack Monitoring for the external non-container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalNonContainerDatabaseStackMonitoring(
EnableExternalNonContainerDatabaseStackMonitoringRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalNonContainerDatabaseStackMonitoringRequest,
EnableExternalNonContainerDatabaseStackMonitoringResponse>
handler);
/**
* Enable Database Management Service for the external pluggable database. For more information
* about the Database Management Service, see [Database Management
* Service](https://docs.cloud.oracle.com/Content/ExternalDatabase/Concepts/databasemanagementservice.htm).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalPluggableDatabaseDatabaseManagement(
EnableExternalPluggableDatabaseDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalPluggableDatabaseDatabaseManagementRequest,
EnableExternalPluggableDatabaseDatabaseManagementResponse>
handler);
/**
* Enable Operations Insights for the external pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalPluggableDatabaseOperationsInsights(
EnableExternalPluggableDatabaseOperationsInsightsRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalPluggableDatabaseOperationsInsightsRequest,
EnableExternalPluggableDatabaseOperationsInsightsResponse>
handler);
/**
* Enable Stack Monitoring for the external pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enableExternalPluggableDatabaseStackMonitoring(
EnableExternalPluggableDatabaseStackMonitoringRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableExternalPluggableDatabaseStackMonitoringRequest,
EnableExternalPluggableDatabaseStackMonitoringResponse>
handler);
/**
* Enables the Database Management service for an Oracle Pluggable Database located in Oracle
* Cloud Infrastructure. This service allows the pluggable database to access tools including
* Metrics and Performance hub. Database Management is enabled at the pluggable database (PDB)
* level.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
enablePluggableDatabaseManagement(
EnablePluggableDatabaseManagementRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnablePluggableDatabaseManagementRequest,
EnablePluggableDatabaseManagementResponse>
handler);
/**
* Initiates a failover of the specified Autonomous Database to the associated peer database.
* Applicable only to databases with Disaster Recovery enabled. This API should be called in the
* remote region where the peer database resides. Below parameter is optional: - `peerDbId` Use
* this parameter to specify the database OCID of the Disaster Recovery peer, which is located
* in a different (remote) region from the current peer database. If this parameter is not
* provided, the failover will happen in the same region.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future failOverAutonomousDatabase(
FailOverAutonomousDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
FailOverAutonomousDatabaseRequest, FailOverAutonomousDatabaseResponse>
handler);
/**
* Fails over the standby Autonomous Container Database identified by the
* autonomousContainerDatabaseId parameter to the primary Autonomous Container Database after
* the existing primary Autonomous Container Database fails or becomes unreachable.
*
* A failover can result in data loss, depending on the protection mode in effect at the time
* the primary Autonomous Container Database fails.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
failoverAutonomousContainerDatabaseDataguardAssociation(
FailoverAutonomousContainerDatabaseDataguardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
FailoverAutonomousContainerDatabaseDataguardAssociationRequest,
FailoverAutonomousContainerDatabaseDataguardAssociationResponse>
handler);
/**
* Performs a failover to transition the standby database identified by the `databaseId`
* parameter into the specified Data Guard association's primary role after the existing primary
* database fails or becomes unreachable.
*
* A failover might result in data loss depending on the protection mode in effect at the
* time of the primary database failure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future failoverDataGuardAssociation(
FailoverDataGuardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
FailoverDataGuardAssociationRequest,
FailoverDataGuardAssociationResponse>
handler);
/**
* Creates and downloads a wallet for the specified Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
generateAutonomousDatabaseWallet(
GenerateAutonomousDatabaseWalletRequest request,
com.oracle.bmc.responses.AsyncHandler<
GenerateAutonomousDatabaseWalletRequest,
GenerateAutonomousDatabaseWalletResponse>
handler);
/**
* Generates a recommended Cloud@Customer VM cluster network configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
generateRecommendedVmClusterNetwork(
GenerateRecommendedVmClusterNetworkRequest request,
com.oracle.bmc.responses.AsyncHandler<
GenerateRecommendedVmClusterNetworkRequest,
GenerateRecommendedVmClusterNetworkResponse>
handler);
/**
* Gets information about a specified application virtual IP (VIP) address.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getApplicationVip(
GetApplicationVipRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetApplicationVipRequest, GetApplicationVipResponse>
handler);
/**
* Gets information about the specified Autonomous Container Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousContainerDatabase(
GetAutonomousContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousContainerDatabaseRequest,
GetAutonomousContainerDatabaseResponse>
handler);
/**
* Gets an Autonomous Container Database enabled with Autonomous Data Guard associated with the
* specified Autonomous Container Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousContainerDatabaseDataguardAssociation(
GetAutonomousContainerDatabaseDataguardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousContainerDatabaseDataguardAssociationRequest,
GetAutonomousContainerDatabaseDataguardAssociationResponse>
handler);
/**
* Get resource usage details for the specified Autonomous Container Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousContainerDatabaseResourceUsage(
GetAutonomousContainerDatabaseResourceUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousContainerDatabaseResourceUsageRequest,
GetAutonomousContainerDatabaseResourceUsageResponse>
handler);
/**
* Gets the details of the specified Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutonomousDatabase(
GetAutonomousDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousDatabaseRequest, GetAutonomousDatabaseResponse>
handler);
/**
* Gets information about the specified Autonomous Database backup.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutonomousDatabaseBackup(
GetAutonomousDatabaseBackupRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousDatabaseBackupRequest, GetAutonomousDatabaseBackupResponse>
handler);
/**
* Gets an Autonomous Data Guard-enabled database associated with the specified Autonomous
* Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousDatabaseDataguardAssociation(
GetAutonomousDatabaseDataguardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousDatabaseDataguardAssociationRequest,
GetAutonomousDatabaseDataguardAssociationResponse>
handler);
/**
* Gets the Autonomous Database regional wallet details.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousDatabaseRegionalWallet(
GetAutonomousDatabaseRegionalWalletRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousDatabaseRegionalWalletRequest,
GetAutonomousDatabaseRegionalWalletResponse>
handler);
/**
* Gets information about the specified Autonomous Database Software Image.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousDatabaseSoftwareImage(
GetAutonomousDatabaseSoftwareImageRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousDatabaseSoftwareImageRequest,
GetAutonomousDatabaseSoftwareImageResponse>
handler);
/**
* Gets the wallet details for the specified Autonomous Database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutonomousDatabaseWallet(
GetAutonomousDatabaseWalletRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousDatabaseWalletRequest, GetAutonomousDatabaseWalletResponse>
handler);
/**
* **Deprecated.** Use the {@link
* #getCloudExadataInfrastructure(GetCloudExadataInfrastructureRequest, Consumer, Consumer)
* getCloudExadataInfrastructure} operation to get details of an Exadata Infrastructure resource
* and the {@link #getCloudAutonomousVmCluster(GetCloudAutonomousVmClusterRequest, Consumer,
* Consumer) getCloudAutonomousVmCluster} operation to get details of an Autonomous Exadata VM
* cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousExadataInfrastructure(
GetAutonomousExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousExadataInfrastructureRequest,
GetAutonomousExadataInfrastructureResponse>
handler);
/**
* Gets information about a specific autonomous patch.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutonomousPatch(
GetAutonomousPatchRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousPatchRequest, GetAutonomousPatchResponse>
handler);
/**
* Gets the details of specific Autonomous Virtual Machine.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutonomousVirtualMachine(
GetAutonomousVirtualMachineRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousVirtualMachineRequest, GetAutonomousVirtualMachineResponse>
handler);
/**
* Gets information about the specified Autonomous VM cluster for an Exadata Cloud@Customer
* system. To get information about an Autonomous VM Cluster in the Oracle cloud, see {@link
* #getCloudAutonomousVmCluster(GetCloudAutonomousVmClusterRequest, Consumer, Consumer)
* getCloudAutonomousVmCluster}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutonomousVmCluster(
GetAutonomousVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousVmClusterRequest, GetAutonomousVmClusterResponse>
handler);
/**
* Get the resource usage details for the specified Autonomous Exadata VM cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getAutonomousVmClusterResourceUsage(
GetAutonomousVmClusterResourceUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutonomousVmClusterResourceUsageRequest,
GetAutonomousVmClusterResourceUsageResponse>
handler);
/**
* Gets information about the specified backup.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getBackup(
GetBackupRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information about the specified backup destination in an Exadata Cloud@Customer system.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getBackupDestination(
GetBackupDestinationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetBackupDestinationRequest, GetBackupDestinationResponse>
handler);
/**
* Gets information about the specified Autonomous Exadata VM cluster in the Oracle cloud. For
* Exadata Cloud@Custustomer systems, see {@link
* #getAutonomousVmCluster(GetAutonomousVmClusterRequest, Consumer, Consumer)
* getAutonomousVmCluster}.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getCloudAutonomousVmCluster(
GetCloudAutonomousVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudAutonomousVmClusterRequest, GetCloudAutonomousVmClusterResponse>
handler);
/**
* Get the resource usage details for the specified Cloud Autonomous Exadata VM cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getCloudAutonomousVmClusterResourceUsage(
GetCloudAutonomousVmClusterResourceUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudAutonomousVmClusterResourceUsageRequest,
GetCloudAutonomousVmClusterResourceUsageResponse>
handler);
/**
* Gets information about the specified cloud Exadata infrastructure resource. Applies to
* Exadata Cloud Service instances and Autonomous Database on dedicated Exadata infrastructure
* only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getCloudExadataInfrastructure(
GetCloudExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudExadataInfrastructureRequest,
GetCloudExadataInfrastructureResponse>
handler);
/**
* Gets unallocated resources information for the specified Cloud Exadata infrastructure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getCloudExadataInfrastructureUnallocatedResources(
GetCloudExadataInfrastructureUnallocatedResourcesRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudExadataInfrastructureUnallocatedResourcesRequest,
GetCloudExadataInfrastructureUnallocatedResourcesResponse>
handler);
/**
* Gets information about the specified cloud VM cluster. Applies to Exadata Cloud Service
* instances and Autonomous Database on dedicated Exadata infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getCloudVmCluster(
GetCloudVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudVmClusterRequest, GetCloudVmClusterResponse>
handler);
/**
* Gets the IORM configuration for the specified cloud VM cluster in an Exadata Cloud Service
* instance. If you have not specified an IORM configuration, the default configuration is
* returned.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getCloudVmClusterIormConfig(
GetCloudVmClusterIormConfigRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudVmClusterIormConfigRequest, GetCloudVmClusterIormConfigResponse>
handler);
/**
* Gets information about a specified maintenance update package for a cloud VM cluster. Applies
* to Exadata Cloud Service instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getCloudVmClusterUpdate(
GetCloudVmClusterUpdateRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudVmClusterUpdateRequest, GetCloudVmClusterUpdateResponse>
handler);
/**
* Gets the maintenance update history details for the specified update history entry. Applies
* to Exadata Cloud Service instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getCloudVmClusterUpdateHistoryEntry(
GetCloudVmClusterUpdateHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCloudVmClusterUpdateHistoryEntryRequest,
GetCloudVmClusterUpdateHistoryEntryResponse>
handler);
/**
* Gets the specified database node console connection's information.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getConsoleConnection(
GetConsoleConnectionRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetConsoleConnectionRequest, GetConsoleConnectionResponse>
handler);
/**
* Gets information about the specified database node console history.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getConsoleHistory(
GetConsoleHistoryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetConsoleHistoryRequest, GetConsoleHistoryResponse>
handler);
/**
* Retrieves the specified database node console history contents upto a megabyte.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getConsoleHistoryContent(
GetConsoleHistoryContentRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetConsoleHistoryContentRequest, GetConsoleHistoryContentResponse>
handler);
/**
* Gets the specified Data Guard association's configuration information.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDataGuardAssociation(
GetDataGuardAssociationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetDataGuardAssociationRequest, GetDataGuardAssociationResponse>
handler);
/**
* Gets information about the specified database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDatabase(
GetDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information about the specified database software image.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDatabaseSoftwareImage(
GetDatabaseSoftwareImageRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetDatabaseSoftwareImageRequest, GetDatabaseSoftwareImageResponse>
handler);
/**
* gets the upgrade history for a specified database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getDatabaseUpgradeHistoryEntry(
GetDatabaseUpgradeHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetDatabaseUpgradeHistoryEntryRequest,
GetDatabaseUpgradeHistoryEntryResponse>
handler);
/**
* Gets information about the specified Database Home.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbHome(
GetDbHomeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information about a specified patch package.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbHomePatch(
GetDbHomePatchRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Gets the patch history details for the specified patchHistoryEntryId
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbHomePatchHistoryEntry(
GetDbHomePatchHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetDbHomePatchHistoryEntryRequest, GetDbHomePatchHistoryEntryResponse>
handler);
/**
* Gets information about the specified database node.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbNode(
GetDbNodeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information about the Exadata Db server.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbServer(
GetDbServerRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information about the specified DB system.
*
* *Note:** Deprecated for Exadata Cloud Service systems. Use the [new resource model
* APIs](https://docs.cloud.oracle.com/iaas/Content/Database/Concepts/exaflexsystem.htm#exaflexsystem_topic-resource_model)
* instead.
*
*
For Exadata Cloud Service instances, support for this API will end on May 15th, 2021. See
* [Switching an Exadata DB System to the New Resource Model and
* APIs](https://docs.cloud.oracle.com/iaas/Content/Database/Concepts/exaflexsystem_topic-resource_model_conversion.htm)
* for details on converting existing Exadata DB systems to the new resource model.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbSystem(
GetDbSystemRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information the specified patch.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbSystemPatch(
GetDbSystemPatchRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Gets the details of the specified patch operation on the specified DB system.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDbSystemPatchHistoryEntry(
GetDbSystemPatchHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetDbSystemPatchHistoryEntryRequest,
GetDbSystemPatchHistoryEntryResponse>
handler);
/**
* Gets the details of the specified operating system upgrade operation for the specified DB
* system.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getDbSystemUpgradeHistoryEntry(
GetDbSystemUpgradeHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetDbSystemUpgradeHistoryEntryRequest,
GetDbSystemUpgradeHistoryEntryResponse>
handler);
/**
* Gets information about the specified Exadata infrastructure. Applies to Exadata
* Cloud@Customer instances only. To get information on an Exadata Cloud Service infrastructure
* resource, use the {@link #getCloudExadataInfrastructure(GetCloudExadataInfrastructureRequest,
* Consumer, Consumer) getCloudExadataInfrastructure} operation.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExadataInfrastructure(
GetExadataInfrastructureRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadataInfrastructureRequest, GetExadataInfrastructureResponse>
handler);
/**
* Gets details of the available and consumed OCPUs for the specified Autonomous Exadata
* Infrastructure resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getExadataInfrastructureOcpus(
GetExadataInfrastructureOcpusRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadataInfrastructureOcpusRequest,
GetExadataInfrastructureOcpusResponse>
handler);
/**
* Gets un allocated resources information for the specified Exadata infrastructure. Applies to
* Exadata Cloud@Customer instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getExadataInfrastructureUnAllocatedResources(
GetExadataInfrastructureUnAllocatedResourcesRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadataInfrastructureUnAllocatedResourcesRequest,
GetExadataInfrastructureUnAllocatedResourcesResponse>
handler);
/**
* Gets the IORM configuration settings for the specified cloud Exadata DB system. All Exadata
* service instances have default IORM settings.
*
* *Note:** Deprecated for Exadata Cloud Service systems. Use the [new resource model
* APIs](https://docs.cloud.oracle.com/iaas/Content/Database/Concepts/exaflexsystem.htm#exaflexsystem_topic-resource_model)
* instead.
*
*
For Exadata Cloud Service instances, support for this API will end on May 15th, 2021. See
* [Switching an Exadata DB System to the New Resource Model and
* APIs](https://docs.cloud.oracle.com/iaas/Content/Database/Concepts/exaflexsystem_topic-resource_model_conversion.htm)
* for details on converting existing Exadata DB systems to the new resource model.
*
*
The {@link #getCloudVmClusterIormConfig(GetCloudVmClusterIormConfigRequest, Consumer,
* Consumer) getCloudVmClusterIormConfig} API is used for this operation with Exadata systems
* using the new resource model.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExadataIormConfig(
GetExadataIormConfigRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadataIormConfigRequest, GetExadataIormConfigResponse>
handler);
/**
* Gets information about the specified Exadata VM cluster on Exascale Infrastructure. Applies
* to Exadata Database Service on Exascale Infrastructure only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExadbVmCluster(
GetExadbVmClusterRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadbVmClusterRequest, GetExadbVmClusterResponse>
handler);
/**
* Gets information about a specified maintenance update package for a Exadata VM cluster on
* Exascale Infrastructure.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExadbVmClusterUpdate(
GetExadbVmClusterUpdateRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadbVmClusterUpdateRequest, GetExadbVmClusterUpdateResponse>
handler);
/**
* Gets the maintenance update history details for the specified update history entry.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getExadbVmClusterUpdateHistoryEntry(
GetExadbVmClusterUpdateHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExadbVmClusterUpdateHistoryEntryRequest,
GetExadbVmClusterUpdateHistoryEntryResponse>
handler);
/**
* Gets information about the specified Exadata Database Storage Vaults in the specified
* compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExascaleDbStorageVault(
GetExascaleDbStorageVaultRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExascaleDbStorageVaultRequest, GetExascaleDbStorageVaultResponse>
handler);
/**
* Gets information about the specified execution action.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExecutionAction(
GetExecutionActionRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExecutionActionRequest, GetExecutionActionResponse>
handler);
/**
* Gets information about the specified execution window.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExecutionWindow(
GetExecutionWindowRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExecutionWindowRequest, GetExecutionWindowResponse>
handler);
/**
* Gets information about the specified external backup job.
*
* *Note:** This API is used by an Oracle Cloud Infrastructure Python script that is packaged
* with the Oracle Cloud Infrastructure CLI. Oracle recommends that you use the script instead
* using the API directly. See [Migrating an On-Premises Database to Oracle Cloud Infrastructure
* by Creating a Backup in the
* Cloud](https://docs.cloud.oracle.com/Content/Database/Tasks/mig-onprembackup.htm) for more
* information.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExternalBackupJob(
GetExternalBackupJobRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExternalBackupJobRequest, GetExternalBackupJobResponse>
handler);
/**
* Gets information about the specified external container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExternalContainerDatabase(
GetExternalContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExternalContainerDatabaseRequest,
GetExternalContainerDatabaseResponse>
handler);
/**
* Gets information about the specified external database connector.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExternalDatabaseConnector(
GetExternalDatabaseConnectorRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExternalDatabaseConnectorRequest,
GetExternalDatabaseConnectorResponse>
handler);
/**
* Gets information about a specific external non-container database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getExternalNonContainerDatabase(
GetExternalNonContainerDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExternalNonContainerDatabaseRequest,
GetExternalNonContainerDatabaseResponse>
handler);
/**
* Gets information about a specific {@link
* #createExternalPluggableDatabaseDetails(CreateExternalPluggableDatabaseDetailsRequest,
* Consumer, Consumer) createExternalPluggableDatabaseDetails} resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExternalPluggableDatabase(
GetExternalPluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetExternalPluggableDatabaseRequest,
GetExternalPluggableDatabaseResponse>
handler);
/**
* Gets details of the Exadata Infrastructure target system software versions that can be
* applied to the specified infrastructure resource for maintenance updates. Applies to Exadata
* Cloud@Customer and Exadata Cloud instances only.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getInfrastructureTargetVersions(
GetInfrastructureTargetVersionsRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetInfrastructureTargetVersionsRequest,
GetInfrastructureTargetVersionsResponse>
handler);
/**
* Gets information about the specified key store.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getKeyStore(
GetKeyStoreRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets information about the specified maintenance run.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getMaintenanceRun(
GetMaintenanceRunRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetMaintenanceRunRequest, GetMaintenanceRunResponse>
handler);
/**
* Gets information about the specified maintenance run history.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getMaintenanceRunHistory(
GetMaintenanceRunHistoryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetMaintenanceRunHistoryRequest, GetMaintenanceRunHistoryResponse>
handler);
/**
* Gets information about the specified one-off patch.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getOneoffPatch(
GetOneoffPatchRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Gets the details of operations performed to convert the specified database from non-container
* (non-CDB) to pluggable (PDB).
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getPdbConversionHistoryEntry(
GetPdbConversionHistoryEntryRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetPdbConversionHistoryEntryRequest,
GetPdbConversionHistoryEntryResponse>
handler);
/**
* Gets information about the specified pluggable database.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getPluggableDatabase(
GetPluggableDatabaseRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetPluggableDatabaseRequest, GetPluggableDatabaseResponse>
handler);
/**
* Gets information about the specified Scheduled Action.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getScheduledAction(
GetScheduledActionRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetScheduledActionRequest, GetScheduledActionResponse>
handler);
/**
* Gets information about the specified Scheduling Plan.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future