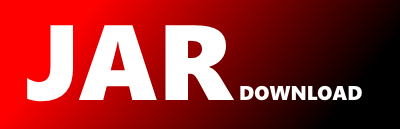
com.oracle.bmc.database.model.ExecutionWindow Maven / Gradle / Ivy
Show all versions of oci-java-sdk-database Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.database.model;
/**
* Details of an execution window.
* Note: Objects should always be created or deserialized using the {@link Builder}. This model
* distinguishes fields that are {@code null} because they are unset from fields that are explicitly
* set to {@code null}. This is done in the setter methods of the {@link Builder}, which maintain a
* set of all explicitly set fields called {@link Builder#__explicitlySet__}. The {@link
* #hashCode()} and {@link #equals(Object)} methods are implemented to take the explicitly set
* fields into account. The constructor, on the other hand, does not take the explicitly set fields
* into account (since the constructor cannot distinguish explicit {@code null} from unset {@code
* null}).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20160918")
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = ExecutionWindow.Builder.class)
@com.fasterxml.jackson.annotation.JsonFilter(
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public final class ExecutionWindow
extends com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel {
@Deprecated
@java.beans.ConstructorProperties({
"id",
"executionResourceId",
"compartmentId",
"displayName",
"description",
"lifecycleState",
"lifecycleSubstate",
"lifecycleDetails",
"timeCreated",
"timeUpdated",
"timeStarted",
"timeEnded",
"timeScheduled",
"windowDurationInMins",
"isEnforcedDuration",
"estimatedTimeInMins",
"totalTimeTakenInMins",
"windowType",
"freeformTags",
"definedTags"
})
public ExecutionWindow(
String id,
String executionResourceId,
String compartmentId,
String displayName,
String description,
LifecycleState lifecycleState,
LifecycleSubstate lifecycleSubstate,
String lifecycleDetails,
java.util.Date timeCreated,
java.util.Date timeUpdated,
java.util.Date timeStarted,
java.util.Date timeEnded,
java.util.Date timeScheduled,
Integer windowDurationInMins,
Boolean isEnforcedDuration,
Integer estimatedTimeInMins,
Integer totalTimeTakenInMins,
WindowType windowType,
java.util.Map freeformTags,
java.util.Map> definedTags) {
super();
this.id = id;
this.executionResourceId = executionResourceId;
this.compartmentId = compartmentId;
this.displayName = displayName;
this.description = description;
this.lifecycleState = lifecycleState;
this.lifecycleSubstate = lifecycleSubstate;
this.lifecycleDetails = lifecycleDetails;
this.timeCreated = timeCreated;
this.timeUpdated = timeUpdated;
this.timeStarted = timeStarted;
this.timeEnded = timeEnded;
this.timeScheduled = timeScheduled;
this.windowDurationInMins = windowDurationInMins;
this.isEnforcedDuration = isEnforcedDuration;
this.estimatedTimeInMins = estimatedTimeInMins;
this.totalTimeTakenInMins = totalTimeTakenInMins;
this.windowType = windowType;
this.freeformTags = freeformTags;
this.definedTags = definedTags;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
public static class Builder {
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution window.
*/
@com.fasterxml.jackson.annotation.JsonProperty("id")
private String id;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution window.
*
* @param id the value to set
* @return this builder
*/
public Builder id(String id) {
this.id = id;
this.__explicitlySet__.add("id");
return this;
}
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution resource the execution window belongs to.
*/
@com.fasterxml.jackson.annotation.JsonProperty("executionResourceId")
private String executionResourceId;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution resource the execution window belongs to.
*
* @param executionResourceId the value to set
* @return this builder
*/
public Builder executionResourceId(String executionResourceId) {
this.executionResourceId = executionResourceId;
this.__explicitlySet__.add("executionResourceId");
return this;
}
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* compartment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private String compartmentId;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* compartment.
*
* @param compartmentId the value to set
* @return this builder
*/
public Builder compartmentId(String compartmentId) {
this.compartmentId = compartmentId;
this.__explicitlySet__.add("compartmentId");
return this;
}
/** The user-friendly name for the execution window. The name does not need to be unique. */
@com.fasterxml.jackson.annotation.JsonProperty("displayName")
private String displayName;
/**
* The user-friendly name for the execution window. The name does not need to be unique.
*
* @param displayName the value to set
* @return this builder
*/
public Builder displayName(String displayName) {
this.displayName = displayName;
this.__explicitlySet__.add("displayName");
return this;
}
/** Description of the execution window. */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private String description;
/**
* Description of the execution window.
*
* @param description the value to set
* @return this builder
*/
public Builder description(String description) {
this.description = description;
this.__explicitlySet__.add("description");
return this;
}
/**
* The current state of the Schedule Policy. Valid states are CREATED, SCHEDULED,
* IN_PROGRESS, FAILED, CANCELED, UPDATING, DELETED, SUCCEEDED and PARTIAL_SUCCESS.
*/
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleState")
private LifecycleState lifecycleState;
/**
* The current state of the Schedule Policy. Valid states are CREATED, SCHEDULED,
* IN_PROGRESS, FAILED, CANCELED, UPDATING, DELETED, SUCCEEDED and PARTIAL_SUCCESS.
*
* @param lifecycleState the value to set
* @return this builder
*/
public Builder lifecycleState(LifecycleState lifecycleState) {
this.lifecycleState = lifecycleState;
this.__explicitlySet__.add("lifecycleState");
return this;
}
/**
* The current sub-state of the execution window. Valid states are DURATION_EXCEEDED,
* MAINTENANCE_IN_PROGRESS and WAITING.
*/
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleSubstate")
private LifecycleSubstate lifecycleSubstate;
/**
* The current sub-state of the execution window. Valid states are DURATION_EXCEEDED,
* MAINTENANCE_IN_PROGRESS and WAITING.
*
* @param lifecycleSubstate the value to set
* @return this builder
*/
public Builder lifecycleSubstate(LifecycleSubstate lifecycleSubstate) {
this.lifecycleSubstate = lifecycleSubstate;
this.__explicitlySet__.add("lifecycleSubstate");
return this;
}
/** Additional information about the current lifecycle state. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleDetails")
private String lifecycleDetails;
/**
* Additional information about the current lifecycle state.
*
* @param lifecycleDetails the value to set
* @return this builder
*/
public Builder lifecycleDetails(String lifecycleDetails) {
this.lifecycleDetails = lifecycleDetails;
this.__explicitlySet__.add("lifecycleDetails");
return this;
}
/** The date and time the execution window was created. */
@com.fasterxml.jackson.annotation.JsonProperty("timeCreated")
private java.util.Date timeCreated;
/**
* The date and time the execution window was created.
*
* @param timeCreated the value to set
* @return this builder
*/
public Builder timeCreated(java.util.Date timeCreated) {
this.timeCreated = timeCreated;
this.__explicitlySet__.add("timeCreated");
return this;
}
/** The last date and time that the execution window was updated. */
@com.fasterxml.jackson.annotation.JsonProperty("timeUpdated")
private java.util.Date timeUpdated;
/**
* The last date and time that the execution window was updated.
*
* @param timeUpdated the value to set
* @return this builder
*/
public Builder timeUpdated(java.util.Date timeUpdated) {
this.timeUpdated = timeUpdated;
this.__explicitlySet__.add("timeUpdated");
return this;
}
/** The date and time that the execution window was started. */
@com.fasterxml.jackson.annotation.JsonProperty("timeStarted")
private java.util.Date timeStarted;
/**
* The date and time that the execution window was started.
*
* @param timeStarted the value to set
* @return this builder
*/
public Builder timeStarted(java.util.Date timeStarted) {
this.timeStarted = timeStarted;
this.__explicitlySet__.add("timeStarted");
return this;
}
/** The date and time that the execution window ended. */
@com.fasterxml.jackson.annotation.JsonProperty("timeEnded")
private java.util.Date timeEnded;
/**
* The date and time that the execution window ended.
*
* @param timeEnded the value to set
* @return this builder
*/
public Builder timeEnded(java.util.Date timeEnded) {
this.timeEnded = timeEnded;
this.__explicitlySet__.add("timeEnded");
return this;
}
/** The scheduled start date and time of the execution window. */
@com.fasterxml.jackson.annotation.JsonProperty("timeScheduled")
private java.util.Date timeScheduled;
/**
* The scheduled start date and time of the execution window.
*
* @param timeScheduled the value to set
* @return this builder
*/
public Builder timeScheduled(java.util.Date timeScheduled) {
this.timeScheduled = timeScheduled;
this.__explicitlySet__.add("timeScheduled");
return this;
}
/**
* Duration window allows user to set a duration they plan to allocate for Scheduling
* window. The duration is in minutes.
*/
@com.fasterxml.jackson.annotation.JsonProperty("windowDurationInMins")
private Integer windowDurationInMins;
/**
* Duration window allows user to set a duration they plan to allocate for Scheduling
* window. The duration is in minutes.
*
* @param windowDurationInMins the value to set
* @return this builder
*/
public Builder windowDurationInMins(Integer windowDurationInMins) {
this.windowDurationInMins = windowDurationInMins;
this.__explicitlySet__.add("windowDurationInMins");
return this;
}
/**
* Indicates if duration the user plans to allocate for scheduling window is strictly
* enforced. The default value is {@code FALSE}.
*/
@com.fasterxml.jackson.annotation.JsonProperty("isEnforcedDuration")
private Boolean isEnforcedDuration;
/**
* Indicates if duration the user plans to allocate for scheduling window is strictly
* enforced. The default value is {@code FALSE}.
*
* @param isEnforcedDuration the value to set
* @return this builder
*/
public Builder isEnforcedDuration(Boolean isEnforcedDuration) {
this.isEnforcedDuration = isEnforcedDuration;
this.__explicitlySet__.add("isEnforcedDuration");
return this;
}
/** The estimated time of the execution window in minutes. */
@com.fasterxml.jackson.annotation.JsonProperty("estimatedTimeInMins")
private Integer estimatedTimeInMins;
/**
* The estimated time of the execution window in minutes.
*
* @param estimatedTimeInMins the value to set
* @return this builder
*/
public Builder estimatedTimeInMins(Integer estimatedTimeInMins) {
this.estimatedTimeInMins = estimatedTimeInMins;
this.__explicitlySet__.add("estimatedTimeInMins");
return this;
}
/** The total time taken by corresponding resource activity in minutes. */
@com.fasterxml.jackson.annotation.JsonProperty("totalTimeTakenInMins")
private Integer totalTimeTakenInMins;
/**
* The total time taken by corresponding resource activity in minutes.
*
* @param totalTimeTakenInMins the value to set
* @return this builder
*/
public Builder totalTimeTakenInMins(Integer totalTimeTakenInMins) {
this.totalTimeTakenInMins = totalTimeTakenInMins;
this.__explicitlySet__.add("totalTimeTakenInMins");
return this;
}
/** The execution window is of PLANNED or UNPLANNED type. */
@com.fasterxml.jackson.annotation.JsonProperty("windowType")
private WindowType windowType;
/**
* The execution window is of PLANNED or UNPLANNED type.
*
* @param windowType the value to set
* @return this builder
*/
public Builder windowType(WindowType windowType) {
this.windowType = windowType;
this.__explicitlySet__.add("windowType");
return this;
}
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Department": "Finance"}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("freeformTags")
private java.util.Map freeformTags;
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Department": "Finance"}}
*
* @param freeformTags the value to set
* @return this builder
*/
public Builder freeformTags(java.util.Map freeformTags) {
this.freeformTags = freeformTags;
this.__explicitlySet__.add("freeformTags");
return this;
}
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For
* more information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*/
@com.fasterxml.jackson.annotation.JsonProperty("definedTags")
private java.util.Map> definedTags;
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For
* more information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*
* @param definedTags the value to set
* @return this builder
*/
public Builder definedTags(
java.util.Map> definedTags) {
this.definedTags = definedTags;
this.__explicitlySet__.add("definedTags");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public ExecutionWindow build() {
ExecutionWindow model =
new ExecutionWindow(
this.id,
this.executionResourceId,
this.compartmentId,
this.displayName,
this.description,
this.lifecycleState,
this.lifecycleSubstate,
this.lifecycleDetails,
this.timeCreated,
this.timeUpdated,
this.timeStarted,
this.timeEnded,
this.timeScheduled,
this.windowDurationInMins,
this.isEnforcedDuration,
this.estimatedTimeInMins,
this.totalTimeTakenInMins,
this.windowType,
this.freeformTags,
this.definedTags);
for (String explicitlySetProperty : this.__explicitlySet__) {
model.markPropertyAsExplicitlySet(explicitlySetProperty);
}
return model;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(ExecutionWindow model) {
if (model.wasPropertyExplicitlySet("id")) {
this.id(model.getId());
}
if (model.wasPropertyExplicitlySet("executionResourceId")) {
this.executionResourceId(model.getExecutionResourceId());
}
if (model.wasPropertyExplicitlySet("compartmentId")) {
this.compartmentId(model.getCompartmentId());
}
if (model.wasPropertyExplicitlySet("displayName")) {
this.displayName(model.getDisplayName());
}
if (model.wasPropertyExplicitlySet("description")) {
this.description(model.getDescription());
}
if (model.wasPropertyExplicitlySet("lifecycleState")) {
this.lifecycleState(model.getLifecycleState());
}
if (model.wasPropertyExplicitlySet("lifecycleSubstate")) {
this.lifecycleSubstate(model.getLifecycleSubstate());
}
if (model.wasPropertyExplicitlySet("lifecycleDetails")) {
this.lifecycleDetails(model.getLifecycleDetails());
}
if (model.wasPropertyExplicitlySet("timeCreated")) {
this.timeCreated(model.getTimeCreated());
}
if (model.wasPropertyExplicitlySet("timeUpdated")) {
this.timeUpdated(model.getTimeUpdated());
}
if (model.wasPropertyExplicitlySet("timeStarted")) {
this.timeStarted(model.getTimeStarted());
}
if (model.wasPropertyExplicitlySet("timeEnded")) {
this.timeEnded(model.getTimeEnded());
}
if (model.wasPropertyExplicitlySet("timeScheduled")) {
this.timeScheduled(model.getTimeScheduled());
}
if (model.wasPropertyExplicitlySet("windowDurationInMins")) {
this.windowDurationInMins(model.getWindowDurationInMins());
}
if (model.wasPropertyExplicitlySet("isEnforcedDuration")) {
this.isEnforcedDuration(model.getIsEnforcedDuration());
}
if (model.wasPropertyExplicitlySet("estimatedTimeInMins")) {
this.estimatedTimeInMins(model.getEstimatedTimeInMins());
}
if (model.wasPropertyExplicitlySet("totalTimeTakenInMins")) {
this.totalTimeTakenInMins(model.getTotalTimeTakenInMins());
}
if (model.wasPropertyExplicitlySet("windowType")) {
this.windowType(model.getWindowType());
}
if (model.wasPropertyExplicitlySet("freeformTags")) {
this.freeformTags(model.getFreeformTags());
}
if (model.wasPropertyExplicitlySet("definedTags")) {
this.definedTags(model.getDefinedTags());
}
return this;
}
}
/** Create a new builder. */
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return new Builder().copy(this);
}
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution window.
*/
@com.fasterxml.jackson.annotation.JsonProperty("id")
private final String id;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution window.
*
* @return the value
*/
public String getId() {
return id;
}
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution resource the execution window belongs to.
*/
@com.fasterxml.jackson.annotation.JsonProperty("executionResourceId")
private final String executionResourceId;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* execution resource the execution window belongs to.
*
* @return the value
*/
public String getExecutionResourceId() {
return executionResourceId;
}
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* compartment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private final String compartmentId;
/**
* The [OCID](https://docs.cloud.oracle.com/Content/General/Concepts/identifiers.htm) of the
* compartment.
*
* @return the value
*/
public String getCompartmentId() {
return compartmentId;
}
/** The user-friendly name for the execution window. The name does not need to be unique. */
@com.fasterxml.jackson.annotation.JsonProperty("displayName")
private final String displayName;
/**
* The user-friendly name for the execution window. The name does not need to be unique.
*
* @return the value
*/
public String getDisplayName() {
return displayName;
}
/** Description of the execution window. */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private final String description;
/**
* Description of the execution window.
*
* @return the value
*/
public String getDescription() {
return description;
}
/**
* The current state of the Schedule Policy. Valid states are CREATED, SCHEDULED, IN_PROGRESS,
* FAILED, CANCELED, UPDATING, DELETED, SUCCEEDED and PARTIAL_SUCCESS.
*/
public enum LifecycleState implements com.oracle.bmc.http.internal.BmcEnum {
Created("CREATED"),
Scheduled("SCHEDULED"),
InProgress("IN_PROGRESS"),
Failed("FAILED"),
Canceled("CANCELED"),
Updating("UPDATING"),
Deleted("DELETED"),
Succeeded("SUCCEEDED"),
PartialSuccess("PARTIAL_SUCCESS"),
Creating("CREATING"),
Deleting("DELETING"),
/**
* This value is used if a service returns a value for this enum that is not recognized by
* this version of the SDK.
*/
UnknownEnumValue(null);
private static final org.slf4j.Logger LOG =
org.slf4j.LoggerFactory.getLogger(LifecycleState.class);
private final String value;
private static java.util.Map map;
static {
map = new java.util.HashMap<>();
for (LifecycleState v : LifecycleState.values()) {
if (v != UnknownEnumValue) {
map.put(v.getValue(), v);
}
}
}
LifecycleState(String value) {
this.value = value;
}
@com.fasterxml.jackson.annotation.JsonValue
public String getValue() {
return value;
}
@com.fasterxml.jackson.annotation.JsonCreator
public static LifecycleState create(String key) {
if (map.containsKey(key)) {
return map.get(key);
}
LOG.warn(
"Received unknown value '{}' for enum 'LifecycleState', returning UnknownEnumValue",
key);
return UnknownEnumValue;
}
};
/**
* The current state of the Schedule Policy. Valid states are CREATED, SCHEDULED, IN_PROGRESS,
* FAILED, CANCELED, UPDATING, DELETED, SUCCEEDED and PARTIAL_SUCCESS.
*/
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleState")
private final LifecycleState lifecycleState;
/**
* The current state of the Schedule Policy. Valid states are CREATED, SCHEDULED, IN_PROGRESS,
* FAILED, CANCELED, UPDATING, DELETED, SUCCEEDED and PARTIAL_SUCCESS.
*
* @return the value
*/
public LifecycleState getLifecycleState() {
return lifecycleState;
}
/**
* The current sub-state of the execution window. Valid states are DURATION_EXCEEDED,
* MAINTENANCE_IN_PROGRESS and WAITING.
*/
public enum LifecycleSubstate implements com.oracle.bmc.http.internal.BmcEnum {
DurationExceeded("DURATION_EXCEEDED"),
MaintenanceInProgress("MAINTENANCE_IN_PROGRESS"),
Waiting("WAITING"),
Rescheduled("RESCHEDULED"),
/**
* This value is used if a service returns a value for this enum that is not recognized by
* this version of the SDK.
*/
UnknownEnumValue(null);
private static final org.slf4j.Logger LOG =
org.slf4j.LoggerFactory.getLogger(LifecycleSubstate.class);
private final String value;
private static java.util.Map map;
static {
map = new java.util.HashMap<>();
for (LifecycleSubstate v : LifecycleSubstate.values()) {
if (v != UnknownEnumValue) {
map.put(v.getValue(), v);
}
}
}
LifecycleSubstate(String value) {
this.value = value;
}
@com.fasterxml.jackson.annotation.JsonValue
public String getValue() {
return value;
}
@com.fasterxml.jackson.annotation.JsonCreator
public static LifecycleSubstate create(String key) {
if (map.containsKey(key)) {
return map.get(key);
}
LOG.warn(
"Received unknown value '{}' for enum 'LifecycleSubstate', returning UnknownEnumValue",
key);
return UnknownEnumValue;
}
};
/**
* The current sub-state of the execution window. Valid states are DURATION_EXCEEDED,
* MAINTENANCE_IN_PROGRESS and WAITING.
*/
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleSubstate")
private final LifecycleSubstate lifecycleSubstate;
/**
* The current sub-state of the execution window. Valid states are DURATION_EXCEEDED,
* MAINTENANCE_IN_PROGRESS and WAITING.
*
* @return the value
*/
public LifecycleSubstate getLifecycleSubstate() {
return lifecycleSubstate;
}
/** Additional information about the current lifecycle state. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleDetails")
private final String lifecycleDetails;
/**
* Additional information about the current lifecycle state.
*
* @return the value
*/
public String getLifecycleDetails() {
return lifecycleDetails;
}
/** The date and time the execution window was created. */
@com.fasterxml.jackson.annotation.JsonProperty("timeCreated")
private final java.util.Date timeCreated;
/**
* The date and time the execution window was created.
*
* @return the value
*/
public java.util.Date getTimeCreated() {
return timeCreated;
}
/** The last date and time that the execution window was updated. */
@com.fasterxml.jackson.annotation.JsonProperty("timeUpdated")
private final java.util.Date timeUpdated;
/**
* The last date and time that the execution window was updated.
*
* @return the value
*/
public java.util.Date getTimeUpdated() {
return timeUpdated;
}
/** The date and time that the execution window was started. */
@com.fasterxml.jackson.annotation.JsonProperty("timeStarted")
private final java.util.Date timeStarted;
/**
* The date and time that the execution window was started.
*
* @return the value
*/
public java.util.Date getTimeStarted() {
return timeStarted;
}
/** The date and time that the execution window ended. */
@com.fasterxml.jackson.annotation.JsonProperty("timeEnded")
private final java.util.Date timeEnded;
/**
* The date and time that the execution window ended.
*
* @return the value
*/
public java.util.Date getTimeEnded() {
return timeEnded;
}
/** The scheduled start date and time of the execution window. */
@com.fasterxml.jackson.annotation.JsonProperty("timeScheduled")
private final java.util.Date timeScheduled;
/**
* The scheduled start date and time of the execution window.
*
* @return the value
*/
public java.util.Date getTimeScheduled() {
return timeScheduled;
}
/**
* Duration window allows user to set a duration they plan to allocate for Scheduling window.
* The duration is in minutes.
*/
@com.fasterxml.jackson.annotation.JsonProperty("windowDurationInMins")
private final Integer windowDurationInMins;
/**
* Duration window allows user to set a duration they plan to allocate for Scheduling window.
* The duration is in minutes.
*
* @return the value
*/
public Integer getWindowDurationInMins() {
return windowDurationInMins;
}
/**
* Indicates if duration the user plans to allocate for scheduling window is strictly enforced.
* The default value is {@code FALSE}.
*/
@com.fasterxml.jackson.annotation.JsonProperty("isEnforcedDuration")
private final Boolean isEnforcedDuration;
/**
* Indicates if duration the user plans to allocate for scheduling window is strictly enforced.
* The default value is {@code FALSE}.
*
* @return the value
*/
public Boolean getIsEnforcedDuration() {
return isEnforcedDuration;
}
/** The estimated time of the execution window in minutes. */
@com.fasterxml.jackson.annotation.JsonProperty("estimatedTimeInMins")
private final Integer estimatedTimeInMins;
/**
* The estimated time of the execution window in minutes.
*
* @return the value
*/
public Integer getEstimatedTimeInMins() {
return estimatedTimeInMins;
}
/** The total time taken by corresponding resource activity in minutes. */
@com.fasterxml.jackson.annotation.JsonProperty("totalTimeTakenInMins")
private final Integer totalTimeTakenInMins;
/**
* The total time taken by corresponding resource activity in minutes.
*
* @return the value
*/
public Integer getTotalTimeTakenInMins() {
return totalTimeTakenInMins;
}
/** The execution window is of PLANNED or UNPLANNED type. */
public enum WindowType implements com.oracle.bmc.http.internal.BmcEnum {
Planned("PLANNED"),
Unplanned("UNPLANNED"),
/**
* This value is used if a service returns a value for this enum that is not recognized by
* this version of the SDK.
*/
UnknownEnumValue(null);
private static final org.slf4j.Logger LOG =
org.slf4j.LoggerFactory.getLogger(WindowType.class);
private final String value;
private static java.util.Map map;
static {
map = new java.util.HashMap<>();
for (WindowType v : WindowType.values()) {
if (v != UnknownEnumValue) {
map.put(v.getValue(), v);
}
}
}
WindowType(String value) {
this.value = value;
}
@com.fasterxml.jackson.annotation.JsonValue
public String getValue() {
return value;
}
@com.fasterxml.jackson.annotation.JsonCreator
public static WindowType create(String key) {
if (map.containsKey(key)) {
return map.get(key);
}
LOG.warn(
"Received unknown value '{}' for enum 'WindowType', returning UnknownEnumValue",
key);
return UnknownEnumValue;
}
};
/** The execution window is of PLANNED or UNPLANNED type. */
@com.fasterxml.jackson.annotation.JsonProperty("windowType")
private final WindowType windowType;
/**
* The execution window is of PLANNED or UNPLANNED type.
*
* @return the value
*/
public WindowType getWindowType() {
return windowType;
}
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Department": "Finance"}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("freeformTags")
private final java.util.Map freeformTags;
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Department": "Finance"}}
*
* @return the value
*/
public java.util.Map getFreeformTags() {
return freeformTags;
}
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For more
* information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*/
@com.fasterxml.jackson.annotation.JsonProperty("definedTags")
private final java.util.Map> definedTags;
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For more
* information, see [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
*
* @return the value
*/
public java.util.Map> getDefinedTags() {
return definedTags;
}
@Override
public String toString() {
return this.toString(true);
}
/**
* Return a string representation of the object.
*
* @param includeByteArrayContents true to include the full contents of byte arrays
* @return string representation
*/
public String toString(boolean includeByteArrayContents) {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("ExecutionWindow(");
sb.append("super=").append(super.toString());
sb.append("id=").append(String.valueOf(this.id));
sb.append(", executionResourceId=").append(String.valueOf(this.executionResourceId));
sb.append(", compartmentId=").append(String.valueOf(this.compartmentId));
sb.append(", displayName=").append(String.valueOf(this.displayName));
sb.append(", description=").append(String.valueOf(this.description));
sb.append(", lifecycleState=").append(String.valueOf(this.lifecycleState));
sb.append(", lifecycleSubstate=").append(String.valueOf(this.lifecycleSubstate));
sb.append(", lifecycleDetails=").append(String.valueOf(this.lifecycleDetails));
sb.append(", timeCreated=").append(String.valueOf(this.timeCreated));
sb.append(", timeUpdated=").append(String.valueOf(this.timeUpdated));
sb.append(", timeStarted=").append(String.valueOf(this.timeStarted));
sb.append(", timeEnded=").append(String.valueOf(this.timeEnded));
sb.append(", timeScheduled=").append(String.valueOf(this.timeScheduled));
sb.append(", windowDurationInMins=").append(String.valueOf(this.windowDurationInMins));
sb.append(", isEnforcedDuration=").append(String.valueOf(this.isEnforcedDuration));
sb.append(", estimatedTimeInMins=").append(String.valueOf(this.estimatedTimeInMins));
sb.append(", totalTimeTakenInMins=").append(String.valueOf(this.totalTimeTakenInMins));
sb.append(", windowType=").append(String.valueOf(this.windowType));
sb.append(", freeformTags=").append(String.valueOf(this.freeformTags));
sb.append(", definedTags=").append(String.valueOf(this.definedTags));
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ExecutionWindow)) {
return false;
}
ExecutionWindow other = (ExecutionWindow) o;
return java.util.Objects.equals(this.id, other.id)
&& java.util.Objects.equals(this.executionResourceId, other.executionResourceId)
&& java.util.Objects.equals(this.compartmentId, other.compartmentId)
&& java.util.Objects.equals(this.displayName, other.displayName)
&& java.util.Objects.equals(this.description, other.description)
&& java.util.Objects.equals(this.lifecycleState, other.lifecycleState)
&& java.util.Objects.equals(this.lifecycleSubstate, other.lifecycleSubstate)
&& java.util.Objects.equals(this.lifecycleDetails, other.lifecycleDetails)
&& java.util.Objects.equals(this.timeCreated, other.timeCreated)
&& java.util.Objects.equals(this.timeUpdated, other.timeUpdated)
&& java.util.Objects.equals(this.timeStarted, other.timeStarted)
&& java.util.Objects.equals(this.timeEnded, other.timeEnded)
&& java.util.Objects.equals(this.timeScheduled, other.timeScheduled)
&& java.util.Objects.equals(this.windowDurationInMins, other.windowDurationInMins)
&& java.util.Objects.equals(this.isEnforcedDuration, other.isEnforcedDuration)
&& java.util.Objects.equals(this.estimatedTimeInMins, other.estimatedTimeInMins)
&& java.util.Objects.equals(this.totalTimeTakenInMins, other.totalTimeTakenInMins)
&& java.util.Objects.equals(this.windowType, other.windowType)
&& java.util.Objects.equals(this.freeformTags, other.freeformTags)
&& java.util.Objects.equals(this.definedTags, other.definedTags)
&& super.equals(other);
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = (result * PRIME) + (this.id == null ? 43 : this.id.hashCode());
result =
(result * PRIME)
+ (this.executionResourceId == null
? 43
: this.executionResourceId.hashCode());
result =
(result * PRIME)
+ (this.compartmentId == null ? 43 : this.compartmentId.hashCode());
result = (result * PRIME) + (this.displayName == null ? 43 : this.displayName.hashCode());
result = (result * PRIME) + (this.description == null ? 43 : this.description.hashCode());
result =
(result * PRIME)
+ (this.lifecycleState == null ? 43 : this.lifecycleState.hashCode());
result =
(result * PRIME)
+ (this.lifecycleSubstate == null ? 43 : this.lifecycleSubstate.hashCode());
result =
(result * PRIME)
+ (this.lifecycleDetails == null ? 43 : this.lifecycleDetails.hashCode());
result = (result * PRIME) + (this.timeCreated == null ? 43 : this.timeCreated.hashCode());
result = (result * PRIME) + (this.timeUpdated == null ? 43 : this.timeUpdated.hashCode());
result = (result * PRIME) + (this.timeStarted == null ? 43 : this.timeStarted.hashCode());
result = (result * PRIME) + (this.timeEnded == null ? 43 : this.timeEnded.hashCode());
result =
(result * PRIME)
+ (this.timeScheduled == null ? 43 : this.timeScheduled.hashCode());
result =
(result * PRIME)
+ (this.windowDurationInMins == null
? 43
: this.windowDurationInMins.hashCode());
result =
(result * PRIME)
+ (this.isEnforcedDuration == null
? 43
: this.isEnforcedDuration.hashCode());
result =
(result * PRIME)
+ (this.estimatedTimeInMins == null
? 43
: this.estimatedTimeInMins.hashCode());
result =
(result * PRIME)
+ (this.totalTimeTakenInMins == null
? 43
: this.totalTimeTakenInMins.hashCode());
result = (result * PRIME) + (this.windowType == null ? 43 : this.windowType.hashCode());
result = (result * PRIME) + (this.freeformTags == null ? 43 : this.freeformTags.hashCode());
result = (result * PRIME) + (this.definedTags == null ? 43 : this.definedTags.hashCode());
result = (result * PRIME) + super.hashCode();
return result;
}
}