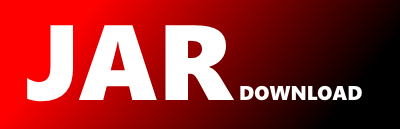
com.oracle.bmc.fleetappsmanagement.FleetAppsManagementAsync Maven / Gradle / Ivy
Show all versions of oci-java-sdk-fleetappsmanagement Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.fleetappsmanagement;
import com.oracle.bmc.fleetappsmanagement.requests.*;
import com.oracle.bmc.fleetappsmanagement.responses.*;
/**
* Fleet Application Management provides a centralized platform to help you automate resource
* management tasks, validate patch compliance, and enhance operational efficiency across an
* enterprise.
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20230831")
public interface FleetAppsManagementAsync extends AutoCloseable {
/** Rebuilds the client from scratch. Useful to refresh certificates. */
void refreshClient();
/**
* Sets the endpoint to call (ex, https://www.example.com).
*
* @param endpoint The endpoint of the serice.
*/
void setEndpoint(String endpoint);
/** Gets the set endpoint for REST call (ex, https://www.example.com) */
String getEndpoint();
/**
* Sets the region to call (ex, Region.US_PHOENIX_1).
*
* Note, this will call {@link #setEndpoint(String) setEndpoint} after resolving the
* endpoint. If the service is not available in this region, however, an
* IllegalArgumentException will be raised.
*
* @param region The region of the service.
*/
void setRegion(com.oracle.bmc.Region region);
/**
* Sets the region to call (ex, 'us-phoenix-1').
*
*
Note, this will first try to map the region ID to a known Region and call {@link
* #setRegion(Region) setRegion}.
*
*
If no known Region could be determined, it will create an endpoint based on the default
* endpoint format ({@link com.oracle.bmc.Region#formatDefaultRegionEndpoint(Service, String)}
* and then call {@link #setEndpoint(String) setEndpoint}.
*
* @param regionId The public region ID.
*/
void setRegion(String regionId);
/**
* Determines whether realm specific endpoint should be used or not. Set
* realmSpecificEndpointTemplateEnabled to "true" if the user wants to enable use of realm
* specific endpoint template, otherwise set it to "false"
*
* @param realmSpecificEndpointTemplateEnabled flag to enable the use of realm specific endpoint
* template
*/
void useRealmSpecificEndpointTemplate(boolean realmSpecificEndpointTemplateEnabled);
/**
* Check if Fleet Application Management tags can be added to the resources.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future checkResourceTagging(
CheckResourceTaggingRequest request,
com.oracle.bmc.responses.AsyncHandler<
CheckResourceTaggingRequest, CheckResourceTaggingResponse>
handler);
/**
* Confirm targets to be managed for a Fleet. Only targets that are confirmed will be managed by
* Fleet Application Management
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future confirmTargets(
ConfirmTargetsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Create a product, environment, group, or generic type of fleet in Fleet Application
* Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createFleet(
CreateFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Add credentials to a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createFleetCredential(
CreateFleetCredentialRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateFleetCredentialRequest, CreateFleetCredentialResponse>
handler);
/**
* Add an existing global property to a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createFleetProperty(
CreateFleetPropertyRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateFleetPropertyRequest, CreateFleetPropertyResponse>
handler);
/**
* Add resource to a fleet in\u00A0Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createFleetResource(
CreateFleetResourceRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateFleetResourceRequest, CreateFleetResourceResponse>
handler);
/**
* Delete a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteFleet(
DeleteFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Delete a credential associated with a fleet product or application in\u00A0Fleet Application
* Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteFleetCredential(
DeleteFleetCredentialRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteFleetCredentialRequest, DeleteFleetCredentialResponse>
handler);
/**
* Delete a property associated with a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteFleetProperty(
DeleteFleetPropertyRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteFleetPropertyRequest, DeleteFleetPropertyResponse>
handler);
/**
* Removes a resource from the fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteFleetResource(
DeleteFleetResourceRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteFleetResourceRequest, DeleteFleetResourceResponse>
handler);
/**
* Generate compliance reports for a Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future generateComplianceReport(
GenerateComplianceReportRequest request,
com.oracle.bmc.responses.AsyncHandler<
GenerateComplianceReportRequest, GenerateComplianceReportResponse>
handler);
/**
* Retrieve compliance report for a fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getComplianceReport(
GetComplianceReportRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetComplianceReportRequest, GetComplianceReportResponse>
handler);
/**
* Get the details of a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getFleet(
GetFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Gets a FleetCredential by identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getFleetCredential(
GetFleetCredentialRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetFleetCredentialRequest, GetFleetCredentialResponse>
handler);
/**
* Gets a Fleet Property by identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getFleetProperty(
GetFleetPropertyRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Gets a Fleet Resource by identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getFleetResource(
GetFleetResourceRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Gets details of the work request with the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getWorkRequest(
GetWorkRequestRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Return a list of AnnouncementSummary items.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listAnnouncements(
ListAnnouncementsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListAnnouncementsRequest, ListAnnouncementsResponse>
handler);
/**
* List credentials in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleetCredentials(
ListFleetCredentialsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListFleetCredentialsRequest, ListFleetCredentialsResponse>
handler);
/**
* Returns a list of products associated with the confirmed targets.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleetProducts(
ListFleetProductsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListFleetProductsRequest, ListFleetProductsResponse>
handler);
/**
* List fleet properties in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleetProperties(
ListFleetPropertiesRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListFleetPropertiesRequest, ListFleetPropertiesResponse>
handler);
/**
* List resources for a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleetResources(
ListFleetResourcesRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListFleetResourcesRequest, ListFleetResourcesResponse>
handler);
/**
* Returns the list of all confirmed targets within a fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleetTargets(
ListFleetTargetsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns a list of Fleets in the specified Tenancy.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleets(
ListFleetsRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Returns a list of InventoryResources.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listInventoryResources(
ListInventoryResourcesRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListInventoryResourcesRequest, ListInventoryResourcesResponse>
handler);
/**
* Return all targets belonging to the resources within a fleet. It will include both confirmed
* and unconfirmed targets.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listTargets(
ListTargetsRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Returns a (paginated) list of errors for the work request with the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequestErrors(
ListWorkRequestErrorsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListWorkRequestErrorsRequest, ListWorkRequestErrorsResponse>
handler);
/**
* Returns a (paginated) list of logs for the work request with the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequestLogs(
ListWorkRequestLogsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListWorkRequestLogsRequest, ListWorkRequestLogsResponse>
handler);
/**
* Lists the work requests in a compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequests(
ListWorkRequestsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Request validation for resources within a fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future requestResourceValidation(
RequestResourceValidationRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestResourceValidationRequest, RequestResourceValidationResponse>
handler);
/**
* Confirm targets to be managed for a Fleet. Only targets that are confirmed will be managed by
* Fleet Application Management
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future requestTargetDiscovery(
RequestTargetDiscoveryRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestTargetDiscoveryRequest, RequestTargetDiscoveryResponse>
handler);
/**
* Update fleet information in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateFleet(
UpdateFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Edit credentials associated with a product or application in\u00A0Fleet Application
* Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateFleetCredential(
UpdateFleetCredentialRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateFleetCredentialRequest, UpdateFleetCredentialResponse>
handler);
/**
* Edit a property associated with a fleet in Fleet Application Management.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateFleetProperty(
UpdateFleetPropertyRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateFleetPropertyRequest, UpdateFleetPropertyResponse>
handler);
/**
* Updates the FleetResource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateFleetResource(
UpdateFleetResourceRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateFleetResourceRequest, UpdateFleetResourceResponse>
handler);
}