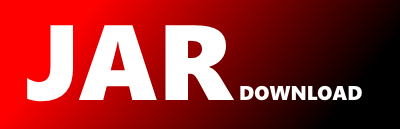
com.oracle.bmc.generativeaiagent.model.AgentEndpointSummary Maven / Gradle / Ivy
Show all versions of oci-java-sdk-generativeaiagent Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.generativeaiagent.model;
/**
* **AgentEndpointSummary**
*
* Summary information about an endpoint.
* Note: Objects should always be created or deserialized using the {@link Builder}. This model
* distinguishes fields that are {@code null} because they are unset from fields that are explicitly
* set to {@code null}. This is done in the setter methods of the {@link Builder}, which maintain a
* set of all explicitly set fields called {@link Builder#__explicitlySet__}. The {@link
* #hashCode()} and {@link #equals(Object)} methods are implemented to take the explicitly set
* fields into account. The constructor, on the other hand, does not take the explicitly set fields
* into account (since the constructor cannot distinguish explicit {@code null} from unset {@code
* null}).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20240531")
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(
builder = AgentEndpointSummary.Builder.class)
@com.fasterxml.jackson.annotation.JsonFilter(
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public final class AgentEndpointSummary
extends com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel {
@Deprecated
@java.beans.ConstructorProperties({
"id",
"displayName",
"description",
"compartmentId",
"agentId",
"contentModerationConfig",
"shouldEnableTrace",
"shouldEnableCitation",
"shouldEnableSession",
"sessionConfig",
"timeCreated",
"timeUpdated",
"lifecycleState",
"lifecycleDetails",
"freeformTags",
"definedTags",
"systemTags"
})
public AgentEndpointSummary(
String id,
String displayName,
String description,
String compartmentId,
String agentId,
ContentModerationConfig contentModerationConfig,
Boolean shouldEnableTrace,
Boolean shouldEnableCitation,
Boolean shouldEnableSession,
SessionConfig sessionConfig,
java.util.Date timeCreated,
java.util.Date timeUpdated,
AgentEndpoint.LifecycleState lifecycleState,
String lifecycleDetails,
java.util.Map freeformTags,
java.util.Map> definedTags,
java.util.Map> systemTags) {
super();
this.id = id;
this.displayName = displayName;
this.description = description;
this.compartmentId = compartmentId;
this.agentId = agentId;
this.contentModerationConfig = contentModerationConfig;
this.shouldEnableTrace = shouldEnableTrace;
this.shouldEnableCitation = shouldEnableCitation;
this.shouldEnableSession = shouldEnableSession;
this.sessionConfig = sessionConfig;
this.timeCreated = timeCreated;
this.timeUpdated = timeUpdated;
this.lifecycleState = lifecycleState;
this.lifecycleDetails = lifecycleDetails;
this.freeformTags = freeformTags;
this.definedTags = definedTags;
this.systemTags = systemTags;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
public static class Builder {
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the AgentEndpoint.
*/
@com.fasterxml.jackson.annotation.JsonProperty("id")
private String id;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the AgentEndpoint.
*
* @param id the value to set
* @return this builder
*/
public Builder id(String id) {
this.id = id;
this.__explicitlySet__.add("id");
return this;
}
/** A user-friendly name. Does not have to be unique, and it's changeable. */
@com.fasterxml.jackson.annotation.JsonProperty("displayName")
private String displayName;
/**
* A user-friendly name. Does not have to be unique, and it's changeable.
*
* @param displayName the value to set
* @return this builder
*/
public Builder displayName(String displayName) {
this.displayName = displayName;
this.__explicitlySet__.add("displayName");
return this;
}
/** An optional description of the endpoint. */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private String description;
/**
* An optional description of the endpoint.
*
* @param description the value to set
* @return this builder
*/
public Builder description(String description) {
this.description = description;
this.__explicitlySet__.add("description");
return this;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the compartment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private String compartmentId;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the compartment.
*
* @param compartmentId the value to set
* @return this builder
*/
public Builder compartmentId(String compartmentId) {
this.compartmentId = compartmentId;
this.__explicitlySet__.add("compartmentId");
return this;
}
/** The OCID of the agent that this AgentEndpoint is associated with. */
@com.fasterxml.jackson.annotation.JsonProperty("agentId")
private String agentId;
/**
* The OCID of the agent that this AgentEndpoint is associated with.
*
* @param agentId the value to set
* @return this builder
*/
public Builder agentId(String agentId) {
this.agentId = agentId;
this.__explicitlySet__.add("agentId");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("contentModerationConfig")
private ContentModerationConfig contentModerationConfig;
public Builder contentModerationConfig(ContentModerationConfig contentModerationConfig) {
this.contentModerationConfig = contentModerationConfig;
this.__explicitlySet__.add("contentModerationConfig");
return this;
}
/** Whether to show traces in the chat result. */
@com.fasterxml.jackson.annotation.JsonProperty("shouldEnableTrace")
private Boolean shouldEnableTrace;
/**
* Whether to show traces in the chat result.
*
* @param shouldEnableTrace the value to set
* @return this builder
*/
public Builder shouldEnableTrace(Boolean shouldEnableTrace) {
this.shouldEnableTrace = shouldEnableTrace;
this.__explicitlySet__.add("shouldEnableTrace");
return this;
}
/** Whether to show citations in the chat result. */
@com.fasterxml.jackson.annotation.JsonProperty("shouldEnableCitation")
private Boolean shouldEnableCitation;
/**
* Whether to show citations in the chat result.
*
* @param shouldEnableCitation the value to set
* @return this builder
*/
public Builder shouldEnableCitation(Boolean shouldEnableCitation) {
this.shouldEnableCitation = shouldEnableCitation;
this.__explicitlySet__.add("shouldEnableCitation");
return this;
}
/** Whether or not to enable Session-based chat. */
@com.fasterxml.jackson.annotation.JsonProperty("shouldEnableSession")
private Boolean shouldEnableSession;
/**
* Whether or not to enable Session-based chat.
*
* @param shouldEnableSession the value to set
* @return this builder
*/
public Builder shouldEnableSession(Boolean shouldEnableSession) {
this.shouldEnableSession = shouldEnableSession;
this.__explicitlySet__.add("shouldEnableSession");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("sessionConfig")
private SessionConfig sessionConfig;
public Builder sessionConfig(SessionConfig sessionConfig) {
this.sessionConfig = sessionConfig;
this.__explicitlySet__.add("sessionConfig");
return this;
}
/**
* The date and time the endpoint was created, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
* Example: {@code 2016-08-25T21:10:29.600Z}
*/
@com.fasterxml.jackson.annotation.JsonProperty("timeCreated")
private java.util.Date timeCreated;
/**
* The date and time the endpoint was created, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
*
Example: {@code 2016-08-25T21:10:29.600Z}
*
* @param timeCreated the value to set
* @return this builder
*/
public Builder timeCreated(java.util.Date timeCreated) {
this.timeCreated = timeCreated;
this.__explicitlySet__.add("timeCreated");
return this;
}
/**
* The date and time the AgentEndpoint was updated, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
*
Example: {@code 2016-08-25T21:10:29.600Z}
*/
@com.fasterxml.jackson.annotation.JsonProperty("timeUpdated")
private java.util.Date timeUpdated;
/**
* The date and time the AgentEndpoint was updated, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
*
Example: {@code 2016-08-25T21:10:29.600Z}
*
* @param timeUpdated the value to set
* @return this builder
*/
public Builder timeUpdated(java.util.Date timeUpdated) {
this.timeUpdated = timeUpdated;
this.__explicitlySet__.add("timeUpdated");
return this;
}
/** The current state of the endpoint. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleState")
private AgentEndpoint.LifecycleState lifecycleState;
/**
* The current state of the endpoint.
*
* @param lifecycleState the value to set
* @return this builder
*/
public Builder lifecycleState(AgentEndpoint.LifecycleState lifecycleState) {
this.lifecycleState = lifecycleState;
this.__explicitlySet__.add("lifecycleState");
return this;
}
/**
* A message that describes the current state of the endpoint in more detail. For example,
* can be used to provide actionable information for a resource in the Failed state.
*/
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleDetails")
private String lifecycleDetails;
/**
* A message that describes the current state of the endpoint in more detail. For example,
* can be used to provide actionable information for a resource in the Failed state.
*
* @param lifecycleDetails the value to set
* @return this builder
*/
public Builder lifecycleDetails(String lifecycleDetails) {
this.lifecycleDetails = lifecycleDetails;
this.__explicitlySet__.add("lifecycleDetails");
return this;
}
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
*
Example: {@code {"Department": "Finance"}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("freeformTags")
private java.util.Map freeformTags;
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Department": "Finance"}}
*
* @param freeformTags the value to set
* @return this builder
*/
public Builder freeformTags(java.util.Map freeformTags) {
this.freeformTags = freeformTags;
this.__explicitlySet__.add("freeformTags");
return this;
}
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For
* more information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("definedTags")
private java.util.Map> definedTags;
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For
* more information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*
* @param definedTags the value to set
* @return this builder
*/
public Builder definedTags(
java.util.Map> definedTags) {
this.definedTags = definedTags;
this.__explicitlySet__.add("definedTags");
return this;
}
/**
* System tags for this resource. Each key is predefined and scoped to a namespace.
*
* Example: {@code {"orcl-cloud": {"free-tier-retained": "true"}}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("systemTags")
private java.util.Map> systemTags;
/**
* System tags for this resource. Each key is predefined and scoped to a namespace.
*
* Example: {@code {"orcl-cloud": {"free-tier-retained": "true"}}}
*
* @param systemTags the value to set
* @return this builder
*/
public Builder systemTags(java.util.Map> systemTags) {
this.systemTags = systemTags;
this.__explicitlySet__.add("systemTags");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public AgentEndpointSummary build() {
AgentEndpointSummary model =
new AgentEndpointSummary(
this.id,
this.displayName,
this.description,
this.compartmentId,
this.agentId,
this.contentModerationConfig,
this.shouldEnableTrace,
this.shouldEnableCitation,
this.shouldEnableSession,
this.sessionConfig,
this.timeCreated,
this.timeUpdated,
this.lifecycleState,
this.lifecycleDetails,
this.freeformTags,
this.definedTags,
this.systemTags);
for (String explicitlySetProperty : this.__explicitlySet__) {
model.markPropertyAsExplicitlySet(explicitlySetProperty);
}
return model;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(AgentEndpointSummary model) {
if (model.wasPropertyExplicitlySet("id")) {
this.id(model.getId());
}
if (model.wasPropertyExplicitlySet("displayName")) {
this.displayName(model.getDisplayName());
}
if (model.wasPropertyExplicitlySet("description")) {
this.description(model.getDescription());
}
if (model.wasPropertyExplicitlySet("compartmentId")) {
this.compartmentId(model.getCompartmentId());
}
if (model.wasPropertyExplicitlySet("agentId")) {
this.agentId(model.getAgentId());
}
if (model.wasPropertyExplicitlySet("contentModerationConfig")) {
this.contentModerationConfig(model.getContentModerationConfig());
}
if (model.wasPropertyExplicitlySet("shouldEnableTrace")) {
this.shouldEnableTrace(model.getShouldEnableTrace());
}
if (model.wasPropertyExplicitlySet("shouldEnableCitation")) {
this.shouldEnableCitation(model.getShouldEnableCitation());
}
if (model.wasPropertyExplicitlySet("shouldEnableSession")) {
this.shouldEnableSession(model.getShouldEnableSession());
}
if (model.wasPropertyExplicitlySet("sessionConfig")) {
this.sessionConfig(model.getSessionConfig());
}
if (model.wasPropertyExplicitlySet("timeCreated")) {
this.timeCreated(model.getTimeCreated());
}
if (model.wasPropertyExplicitlySet("timeUpdated")) {
this.timeUpdated(model.getTimeUpdated());
}
if (model.wasPropertyExplicitlySet("lifecycleState")) {
this.lifecycleState(model.getLifecycleState());
}
if (model.wasPropertyExplicitlySet("lifecycleDetails")) {
this.lifecycleDetails(model.getLifecycleDetails());
}
if (model.wasPropertyExplicitlySet("freeformTags")) {
this.freeformTags(model.getFreeformTags());
}
if (model.wasPropertyExplicitlySet("definedTags")) {
this.definedTags(model.getDefinedTags());
}
if (model.wasPropertyExplicitlySet("systemTags")) {
this.systemTags(model.getSystemTags());
}
return this;
}
}
/** Create a new builder. */
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return new Builder().copy(this);
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the AgentEndpoint.
*/
@com.fasterxml.jackson.annotation.JsonProperty("id")
private final String id;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the AgentEndpoint.
*
* @return the value
*/
public String getId() {
return id;
}
/** A user-friendly name. Does not have to be unique, and it's changeable. */
@com.fasterxml.jackson.annotation.JsonProperty("displayName")
private final String displayName;
/**
* A user-friendly name. Does not have to be unique, and it's changeable.
*
* @return the value
*/
public String getDisplayName() {
return displayName;
}
/** An optional description of the endpoint. */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private final String description;
/**
* An optional description of the endpoint.
*
* @return the value
*/
public String getDescription() {
return description;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the compartment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private final String compartmentId;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the compartment.
*
* @return the value
*/
public String getCompartmentId() {
return compartmentId;
}
/** The OCID of the agent that this AgentEndpoint is associated with. */
@com.fasterxml.jackson.annotation.JsonProperty("agentId")
private final String agentId;
/**
* The OCID of the agent that this AgentEndpoint is associated with.
*
* @return the value
*/
public String getAgentId() {
return agentId;
}
@com.fasterxml.jackson.annotation.JsonProperty("contentModerationConfig")
private final ContentModerationConfig contentModerationConfig;
public ContentModerationConfig getContentModerationConfig() {
return contentModerationConfig;
}
/** Whether to show traces in the chat result. */
@com.fasterxml.jackson.annotation.JsonProperty("shouldEnableTrace")
private final Boolean shouldEnableTrace;
/**
* Whether to show traces in the chat result.
*
* @return the value
*/
public Boolean getShouldEnableTrace() {
return shouldEnableTrace;
}
/** Whether to show citations in the chat result. */
@com.fasterxml.jackson.annotation.JsonProperty("shouldEnableCitation")
private final Boolean shouldEnableCitation;
/**
* Whether to show citations in the chat result.
*
* @return the value
*/
public Boolean getShouldEnableCitation() {
return shouldEnableCitation;
}
/** Whether or not to enable Session-based chat. */
@com.fasterxml.jackson.annotation.JsonProperty("shouldEnableSession")
private final Boolean shouldEnableSession;
/**
* Whether or not to enable Session-based chat.
*
* @return the value
*/
public Boolean getShouldEnableSession() {
return shouldEnableSession;
}
@com.fasterxml.jackson.annotation.JsonProperty("sessionConfig")
private final SessionConfig sessionConfig;
public SessionConfig getSessionConfig() {
return sessionConfig;
}
/**
* The date and time the endpoint was created, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
* Example: {@code 2016-08-25T21:10:29.600Z}
*/
@com.fasterxml.jackson.annotation.JsonProperty("timeCreated")
private final java.util.Date timeCreated;
/**
* The date and time the endpoint was created, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
*
Example: {@code 2016-08-25T21:10:29.600Z}
*
* @return the value
*/
public java.util.Date getTimeCreated() {
return timeCreated;
}
/**
* The date and time the AgentEndpoint was updated, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
*
Example: {@code 2016-08-25T21:10:29.600Z}
*/
@com.fasterxml.jackson.annotation.JsonProperty("timeUpdated")
private final java.util.Date timeUpdated;
/**
* The date and time the AgentEndpoint was updated, in the format defined by [RFC
* 3339](https://tools.ietf.org/html/rfc3339).
*
*
Example: {@code 2016-08-25T21:10:29.600Z}
*
* @return the value
*/
public java.util.Date getTimeUpdated() {
return timeUpdated;
}
/** The current state of the endpoint. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleState")
private final AgentEndpoint.LifecycleState lifecycleState;
/**
* The current state of the endpoint.
*
* @return the value
*/
public AgentEndpoint.LifecycleState getLifecycleState() {
return lifecycleState;
}
/**
* A message that describes the current state of the endpoint in more detail. For example, can
* be used to provide actionable information for a resource in the Failed state.
*/
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleDetails")
private final String lifecycleDetails;
/**
* A message that describes the current state of the endpoint in more detail. For example, can
* be used to provide actionable information for a resource in the Failed state.
*
* @return the value
*/
public String getLifecycleDetails() {
return lifecycleDetails;
}
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
*
Example: {@code {"Department": "Finance"}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("freeformTags")
private final java.util.Map freeformTags;
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. For more information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Department": "Finance"}}
*
* @return the value
*/
public java.util.Map getFreeformTags() {
return freeformTags;
}
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For more
* information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("definedTags")
private final java.util.Map> definedTags;
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. For more
* information, see [Resource
* Tags](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/resourcetags.htm).
*
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*
* @return the value
*/
public java.util.Map> getDefinedTags() {
return definedTags;
}
/**
* System tags for this resource. Each key is predefined and scoped to a namespace.
*
* Example: {@code {"orcl-cloud": {"free-tier-retained": "true"}}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("systemTags")
private final java.util.Map> systemTags;
/**
* System tags for this resource. Each key is predefined and scoped to a namespace.
*
* Example: {@code {"orcl-cloud": {"free-tier-retained": "true"}}}
*
* @return the value
*/
public java.util.Map> getSystemTags() {
return systemTags;
}
@Override
public String toString() {
return this.toString(true);
}
/**
* Return a string representation of the object.
*
* @param includeByteArrayContents true to include the full contents of byte arrays
* @return string representation
*/
public String toString(boolean includeByteArrayContents) {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("AgentEndpointSummary(");
sb.append("super=").append(super.toString());
sb.append("id=").append(String.valueOf(this.id));
sb.append(", displayName=").append(String.valueOf(this.displayName));
sb.append(", description=").append(String.valueOf(this.description));
sb.append(", compartmentId=").append(String.valueOf(this.compartmentId));
sb.append(", agentId=").append(String.valueOf(this.agentId));
sb.append(", contentModerationConfig=")
.append(String.valueOf(this.contentModerationConfig));
sb.append(", shouldEnableTrace=").append(String.valueOf(this.shouldEnableTrace));
sb.append(", shouldEnableCitation=").append(String.valueOf(this.shouldEnableCitation));
sb.append(", shouldEnableSession=").append(String.valueOf(this.shouldEnableSession));
sb.append(", sessionConfig=").append(String.valueOf(this.sessionConfig));
sb.append(", timeCreated=").append(String.valueOf(this.timeCreated));
sb.append(", timeUpdated=").append(String.valueOf(this.timeUpdated));
sb.append(", lifecycleState=").append(String.valueOf(this.lifecycleState));
sb.append(", lifecycleDetails=").append(String.valueOf(this.lifecycleDetails));
sb.append(", freeformTags=").append(String.valueOf(this.freeformTags));
sb.append(", definedTags=").append(String.valueOf(this.definedTags));
sb.append(", systemTags=").append(String.valueOf(this.systemTags));
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof AgentEndpointSummary)) {
return false;
}
AgentEndpointSummary other = (AgentEndpointSummary) o;
return java.util.Objects.equals(this.id, other.id)
&& java.util.Objects.equals(this.displayName, other.displayName)
&& java.util.Objects.equals(this.description, other.description)
&& java.util.Objects.equals(this.compartmentId, other.compartmentId)
&& java.util.Objects.equals(this.agentId, other.agentId)
&& java.util.Objects.equals(
this.contentModerationConfig, other.contentModerationConfig)
&& java.util.Objects.equals(this.shouldEnableTrace, other.shouldEnableTrace)
&& java.util.Objects.equals(this.shouldEnableCitation, other.shouldEnableCitation)
&& java.util.Objects.equals(this.shouldEnableSession, other.shouldEnableSession)
&& java.util.Objects.equals(this.sessionConfig, other.sessionConfig)
&& java.util.Objects.equals(this.timeCreated, other.timeCreated)
&& java.util.Objects.equals(this.timeUpdated, other.timeUpdated)
&& java.util.Objects.equals(this.lifecycleState, other.lifecycleState)
&& java.util.Objects.equals(this.lifecycleDetails, other.lifecycleDetails)
&& java.util.Objects.equals(this.freeformTags, other.freeformTags)
&& java.util.Objects.equals(this.definedTags, other.definedTags)
&& java.util.Objects.equals(this.systemTags, other.systemTags)
&& super.equals(other);
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = (result * PRIME) + (this.id == null ? 43 : this.id.hashCode());
result = (result * PRIME) + (this.displayName == null ? 43 : this.displayName.hashCode());
result = (result * PRIME) + (this.description == null ? 43 : this.description.hashCode());
result =
(result * PRIME)
+ (this.compartmentId == null ? 43 : this.compartmentId.hashCode());
result = (result * PRIME) + (this.agentId == null ? 43 : this.agentId.hashCode());
result =
(result * PRIME)
+ (this.contentModerationConfig == null
? 43
: this.contentModerationConfig.hashCode());
result =
(result * PRIME)
+ (this.shouldEnableTrace == null ? 43 : this.shouldEnableTrace.hashCode());
result =
(result * PRIME)
+ (this.shouldEnableCitation == null
? 43
: this.shouldEnableCitation.hashCode());
result =
(result * PRIME)
+ (this.shouldEnableSession == null
? 43
: this.shouldEnableSession.hashCode());
result =
(result * PRIME)
+ (this.sessionConfig == null ? 43 : this.sessionConfig.hashCode());
result = (result * PRIME) + (this.timeCreated == null ? 43 : this.timeCreated.hashCode());
result = (result * PRIME) + (this.timeUpdated == null ? 43 : this.timeUpdated.hashCode());
result =
(result * PRIME)
+ (this.lifecycleState == null ? 43 : this.lifecycleState.hashCode());
result =
(result * PRIME)
+ (this.lifecycleDetails == null ? 43 : this.lifecycleDetails.hashCode());
result = (result * PRIME) + (this.freeformTags == null ? 43 : this.freeformTags.hashCode());
result = (result * PRIME) + (this.definedTags == null ? 43 : this.definedTags.hashCode());
result = (result * PRIME) + (this.systemTags == null ? 43 : this.systemTags.hashCode());
result = (result * PRIME) + super.hashCode();
return result;
}
}