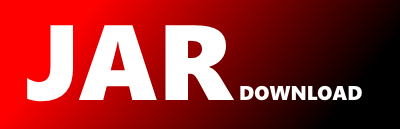
com.oracle.bmc.adm.model.Vulnerability Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oci-java-sdk-shaded-full Show documentation
Show all versions of oci-java-sdk-shaded-full Show documentation
This project contains the SDK distribution used for Oracle Cloud Infrastructure, and all the dependencies that can be shaded. It also has Maven dependencies that cannot be shaded. Therefore, use this module to depend on the shaded distribution via Maven -- it will shade everything that can be shaded, and automatically pull in the other dependencies.
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.adm.model;
/**
* A vulnerability is a weakness or error in an artifact. A vulnerability is a generalization of a
* CVE (every CVE is a vulnerability, but not every vulnerability has a CVE).
* Note: Objects should always be created or deserialized using the {@link Builder}. This model
* distinguishes fields that are {@code null} because they are unset from fields that are explicitly
* set to {@code null}. This is done in the setter methods of the {@link Builder}, which maintain a
* set of all explicitly set fields called {@link Builder#__explicitlySet__}. The {@link
* #hashCode()} and {@link #equals(Object)} methods are implemented to take the explicitly set
* fields into account. The constructor, on the other hand, does not take the explicitly set fields
* into account (since the constructor cannot distinguish explicit {@code null} from unset {@code
* null}).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20220421")
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = Vulnerability.Builder.class)
@com.fasterxml.jackson.annotation.JsonFilter(
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public final class Vulnerability extends com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel {
@Deprecated
@java.beans.ConstructorProperties({
"id",
"source",
"cvssV2Score",
"cvssV3Score",
"severity",
"isIgnored",
"isFalsePositive"
})
public Vulnerability(
String id,
String source,
Float cvssV2Score,
Float cvssV3Score,
VulnerabilitySeverity severity,
Boolean isIgnored,
Boolean isFalsePositive) {
super();
this.id = id;
this.source = source;
this.cvssV2Score = cvssV2Score;
this.cvssV3Score = cvssV3Score;
this.severity = severity;
this.isIgnored = isIgnored;
this.isFalsePositive = isFalsePositive;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
public static class Builder {
/** Unique vulnerability identifier, e.g. CVE-1999-0067. */
@com.fasterxml.jackson.annotation.JsonProperty("id")
private String id;
/**
* Unique vulnerability identifier, e.g. CVE-1999-0067.
*
* @param id the value to set
* @return this builder
*/
public Builder id(String id) {
this.id = id;
this.__explicitlySet__.add("id");
return this;
}
/** Source that published the vulnerability */
@com.fasterxml.jackson.annotation.JsonProperty("source")
private String source;
/**
* Source that published the vulnerability
*
* @param source the value to set
* @return this builder
*/
public Builder source(String source) {
this.source = source;
this.__explicitlySet__.add("source");
return this;
}
/** Common Vulnerability Scoring System (CVSS) Version 2. */
@com.fasterxml.jackson.annotation.JsonProperty("cvssV2Score")
private Float cvssV2Score;
/**
* Common Vulnerability Scoring System (CVSS) Version 2.
*
* @param cvssV2Score the value to set
* @return this builder
*/
public Builder cvssV2Score(Float cvssV2Score) {
this.cvssV2Score = cvssV2Score;
this.__explicitlySet__.add("cvssV2Score");
return this;
}
/** Common Vulnerability Scoring System (CVSS) Version 3. */
@com.fasterxml.jackson.annotation.JsonProperty("cvssV3Score")
private Float cvssV3Score;
/**
* Common Vulnerability Scoring System (CVSS) Version 3.
*
* @param cvssV3Score the value to set
* @return this builder
*/
public Builder cvssV3Score(Float cvssV3Score) {
this.cvssV3Score = cvssV3Score;
this.__explicitlySet__.add("cvssV3Score");
return this;
}
/** ADM qualitative severity score. Can be either NONE, LOW, MEDIUM, HIGH or CRITICAL. */
@com.fasterxml.jackson.annotation.JsonProperty("severity")
private VulnerabilitySeverity severity;
/**
* ADM qualitative severity score. Can be either NONE, LOW, MEDIUM, HIGH or CRITICAL.
*
* @param severity the value to set
* @return this builder
*/
public Builder severity(VulnerabilitySeverity severity) {
this.severity = severity;
this.__explicitlySet__.add("severity");
return this;
}
/** Indicates if the vulnerability was ignored according to the audit configuration. */
@com.fasterxml.jackson.annotation.JsonProperty("isIgnored")
private Boolean isIgnored;
/**
* Indicates if the vulnerability was ignored according to the audit configuration.
*
* @param isIgnored the value to set
* @return this builder
*/
public Builder isIgnored(Boolean isIgnored) {
this.isIgnored = isIgnored;
this.__explicitlySet__.add("isIgnored");
return this;
}
/**
* Indicates if the vulnerability is a false positive according to the usage data. If no
* usage data was provided or the service cannot infer usage of the vulnerable code then
* this property is {@code null}.
*/
@com.fasterxml.jackson.annotation.JsonProperty("isFalsePositive")
private Boolean isFalsePositive;
/**
* Indicates if the vulnerability is a false positive according to the usage data. If no
* usage data was provided or the service cannot infer usage of the vulnerable code then
* this property is {@code null}.
*
* @param isFalsePositive the value to set
* @return this builder
*/
public Builder isFalsePositive(Boolean isFalsePositive) {
this.isFalsePositive = isFalsePositive;
this.__explicitlySet__.add("isFalsePositive");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public Vulnerability build() {
Vulnerability model =
new Vulnerability(
this.id,
this.source,
this.cvssV2Score,
this.cvssV3Score,
this.severity,
this.isIgnored,
this.isFalsePositive);
for (String explicitlySetProperty : this.__explicitlySet__) {
model.markPropertyAsExplicitlySet(explicitlySetProperty);
}
return model;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(Vulnerability model) {
if (model.wasPropertyExplicitlySet("id")) {
this.id(model.getId());
}
if (model.wasPropertyExplicitlySet("source")) {
this.source(model.getSource());
}
if (model.wasPropertyExplicitlySet("cvssV2Score")) {
this.cvssV2Score(model.getCvssV2Score());
}
if (model.wasPropertyExplicitlySet("cvssV3Score")) {
this.cvssV3Score(model.getCvssV3Score());
}
if (model.wasPropertyExplicitlySet("severity")) {
this.severity(model.getSeverity());
}
if (model.wasPropertyExplicitlySet("isIgnored")) {
this.isIgnored(model.getIsIgnored());
}
if (model.wasPropertyExplicitlySet("isFalsePositive")) {
this.isFalsePositive(model.getIsFalsePositive());
}
return this;
}
}
/** Create a new builder. */
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return new Builder().copy(this);
}
/** Unique vulnerability identifier, e.g. CVE-1999-0067. */
@com.fasterxml.jackson.annotation.JsonProperty("id")
private final String id;
/**
* Unique vulnerability identifier, e.g. CVE-1999-0067.
*
* @return the value
*/
public String getId() {
return id;
}
/** Source that published the vulnerability */
@com.fasterxml.jackson.annotation.JsonProperty("source")
private final String source;
/**
* Source that published the vulnerability
*
* @return the value
*/
public String getSource() {
return source;
}
/** Common Vulnerability Scoring System (CVSS) Version 2. */
@com.fasterxml.jackson.annotation.JsonProperty("cvssV2Score")
private final Float cvssV2Score;
/**
* Common Vulnerability Scoring System (CVSS) Version 2.
*
* @return the value
*/
public Float getCvssV2Score() {
return cvssV2Score;
}
/** Common Vulnerability Scoring System (CVSS) Version 3. */
@com.fasterxml.jackson.annotation.JsonProperty("cvssV3Score")
private final Float cvssV3Score;
/**
* Common Vulnerability Scoring System (CVSS) Version 3.
*
* @return the value
*/
public Float getCvssV3Score() {
return cvssV3Score;
}
/** ADM qualitative severity score. Can be either NONE, LOW, MEDIUM, HIGH or CRITICAL. */
@com.fasterxml.jackson.annotation.JsonProperty("severity")
private final VulnerabilitySeverity severity;
/**
* ADM qualitative severity score. Can be either NONE, LOW, MEDIUM, HIGH or CRITICAL.
*
* @return the value
*/
public VulnerabilitySeverity getSeverity() {
return severity;
}
/** Indicates if the vulnerability was ignored according to the audit configuration. */
@com.fasterxml.jackson.annotation.JsonProperty("isIgnored")
private final Boolean isIgnored;
/**
* Indicates if the vulnerability was ignored according to the audit configuration.
*
* @return the value
*/
public Boolean getIsIgnored() {
return isIgnored;
}
/**
* Indicates if the vulnerability is a false positive according to the usage data. If no usage
* data was provided or the service cannot infer usage of the vulnerable code then this property
* is {@code null}.
*/
@com.fasterxml.jackson.annotation.JsonProperty("isFalsePositive")
private final Boolean isFalsePositive;
/**
* Indicates if the vulnerability is a false positive according to the usage data. If no usage
* data was provided or the service cannot infer usage of the vulnerable code then this property
* is {@code null}.
*
* @return the value
*/
public Boolean getIsFalsePositive() {
return isFalsePositive;
}
@Override
public String toString() {
return this.toString(true);
}
/**
* Return a string representation of the object.
*
* @param includeByteArrayContents true to include the full contents of byte arrays
* @return string representation
*/
public String toString(boolean includeByteArrayContents) {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("Vulnerability(");
sb.append("super=").append(super.toString());
sb.append("id=").append(String.valueOf(this.id));
sb.append(", source=").append(String.valueOf(this.source));
sb.append(", cvssV2Score=").append(String.valueOf(this.cvssV2Score));
sb.append(", cvssV3Score=").append(String.valueOf(this.cvssV3Score));
sb.append(", severity=").append(String.valueOf(this.severity));
sb.append(", isIgnored=").append(String.valueOf(this.isIgnored));
sb.append(", isFalsePositive=").append(String.valueOf(this.isFalsePositive));
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Vulnerability)) {
return false;
}
Vulnerability other = (Vulnerability) o;
return java.util.Objects.equals(this.id, other.id)
&& java.util.Objects.equals(this.source, other.source)
&& java.util.Objects.equals(this.cvssV2Score, other.cvssV2Score)
&& java.util.Objects.equals(this.cvssV3Score, other.cvssV3Score)
&& java.util.Objects.equals(this.severity, other.severity)
&& java.util.Objects.equals(this.isIgnored, other.isIgnored)
&& java.util.Objects.equals(this.isFalsePositive, other.isFalsePositive)
&& super.equals(other);
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = (result * PRIME) + (this.id == null ? 43 : this.id.hashCode());
result = (result * PRIME) + (this.source == null ? 43 : this.source.hashCode());
result = (result * PRIME) + (this.cvssV2Score == null ? 43 : this.cvssV2Score.hashCode());
result = (result * PRIME) + (this.cvssV3Score == null ? 43 : this.cvssV3Score.hashCode());
result = (result * PRIME) + (this.severity == null ? 43 : this.severity.hashCode());
result = (result * PRIME) + (this.isIgnored == null ? 43 : this.isIgnored.hashCode());
result =
(result * PRIME)
+ (this.isFalsePositive == null ? 43 : this.isFalsePositive.hashCode());
result = (result * PRIME) + super.hashCode();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy