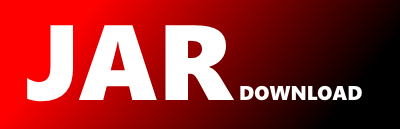
com.oracle.bmc.analytics.AnalyticsWaiters Maven / Gradle / Ivy
Show all versions of oci-java-sdk-shaded-full Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.analytics;
import com.oracle.bmc.analytics.requests.*;
import com.oracle.bmc.analytics.responses.*;
/**
* Collection of helper methods to produce {@link com.oracle.bmc.waiter.Waiter}s for different
* resources of Analytics.
*
* The default configuration used is defined by {@link
* com.oracle.bmc.waiter.Waiters.Waiters#DEFAULT_POLLING_WAITER}.
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20190331")
public class AnalyticsWaiters {
private final java.util.concurrent.ExecutorService executorService;
private final Analytics client;
public AnalyticsWaiters(
java.util.concurrent.ExecutorService executorService, Analytics client) {
this.executorService = executorService;
this.client = client;
}
/**
* Creates a new {@link com.oracle.bmc.waiter.Waiter} using the default configuration.
*
* @param request the request to send
* @param targetState the desired states to wait for. If multiple states are provided then the
* waiter will return once the resource reaches any of the provided states
* @return a new {@code com.oracle.bmc.waiter.Waiter} instance
*/
public com.oracle.bmc.waiter.Waiter
forAnalyticsInstance(
GetAnalyticsInstanceRequest request,
com.oracle.bmc.analytics.model.AnalyticsInstanceLifecycleState...
targetStates) {
com.oracle.bmc.util.internal.Validate.notEmpty(
targetStates, "At least one targetState must be provided");
com.oracle.bmc.util.internal.Validate.noNullElements(
targetStates, "Null targetState values are not permitted");
return forAnalyticsInstance(
com.oracle.bmc.waiter.Waiters.DEFAULT_POLLING_WAITER, request, targetStates);
}
/**
* Creates a new {@link com.oracle.bmc.waiter.Waiter} using the provided configuration.
*
* @param request the request to send
* @param targetState the desired state to wait for
* @param terminationStrategy the {@link com.oracle.bmc.waiter.TerminationStrategy} to use
* @param delayStrategy the {@link com.oracle.bmc.waiter.DelayStrategy} to use
* @return a new {@code com.oracle.bmc.waiter.Waiter} instance
*/
public com.oracle.bmc.waiter.Waiter
forAnalyticsInstance(
GetAnalyticsInstanceRequest request,
com.oracle.bmc.analytics.model.AnalyticsInstanceLifecycleState targetState,
com.oracle.bmc.waiter.TerminationStrategy terminationStrategy,
com.oracle.bmc.waiter.DelayStrategy delayStrategy) {
com.oracle.bmc.util.internal.Validate.notNull(
targetState, "The targetState cannot be null");
return forAnalyticsInstance(
com.oracle.bmc.waiter.Waiters.newWaiter(terminationStrategy, delayStrategy),
request,
targetState);
}
/**
* Creates a new {@link com.oracle.bmc.waiter.Waiter} using the provided configuration.
*
* @param request the request to send
* @param terminationStrategy the {@link com.oracle.bmc.waiter.TerminationStrategy} to use
* @param delayStrategy the {@link com.oracle.bmc.waiter.DelayStrategy} to use
* @param targetStates the desired states to wait for. The waiter will return once the resource
* reaches any of the provided states
* @return a new {@code Waiter} instance
*/
public com.oracle.bmc.waiter.Waiter
forAnalyticsInstance(
GetAnalyticsInstanceRequest request,
com.oracle.bmc.waiter.TerminationStrategy terminationStrategy,
com.oracle.bmc.waiter.DelayStrategy delayStrategy,
com.oracle.bmc.analytics.model.AnalyticsInstanceLifecycleState...
targetStates) {
com.oracle.bmc.util.internal.Validate.notEmpty(
targetStates, "At least one targetState must be provided");
com.oracle.bmc.util.internal.Validate.noNullElements(
targetStates, "Null targetState values are not permitted");
return forAnalyticsInstance(
com.oracle.bmc.waiter.Waiters.newWaiter(terminationStrategy, delayStrategy),
request,
targetStates);
}
// Helper method to create a new Waiter for AnalyticsInstance.
private com.oracle.bmc.waiter.Waiter
forAnalyticsInstance(
com.oracle.bmc.waiter.BmcGenericWaiter waiter,
final GetAnalyticsInstanceRequest request,
final com.oracle.bmc.analytics.model.AnalyticsInstanceLifecycleState...
targetStates) {
final java.util.Set
targetStatesSet = new java.util.HashSet<>(java.util.Arrays.asList(targetStates));
return new com.oracle.bmc.waiter.internal.SimpleWaiterImpl<>(
executorService,
waiter.toCallable(
() -> request,
new java.util.function.Function<
GetAnalyticsInstanceRequest, GetAnalyticsInstanceResponse>() {
@Override
public GetAnalyticsInstanceResponse apply(
GetAnalyticsInstanceRequest request) {
return client.getAnalyticsInstance(request);
}
},
new java.util.function.Predicate() {
@Override
public boolean test(GetAnalyticsInstanceResponse response) {
return targetStatesSet.contains(
response.getAnalyticsInstance().getLifecycleState());
}
},
targetStatesSet.contains(
com.oracle.bmc.analytics.model.AnalyticsInstanceLifecycleState
.Deleted)),
request);
}
/**
* Creates a new {@link com.oracle.bmc.waiter.Waiter} using default configuration.
*
* @param request the request to send
* @return a new {@code Waiter} instance
*/
public com.oracle.bmc.waiter.Waiter
forWorkRequest(GetWorkRequestRequest request) {
return forWorkRequest(com.oracle.bmc.waiter.Waiters.DEFAULT_POLLING_WAITER, request);
}
/**
* Creates a new {@link com.oracle.bmc.waiter.Waiter} using the provided configuration.
*
* @param request the request to send
* @param terminationStrategy the {@link com.oracle.bmc.waiter.TerminationStrategy} to use
* @param delayStrategy the {@linkcom.oracle.bmc.waiter. DelayStrategy} to use
* @return a new {@code com.oracle.bmc.waiter.Waiter} instance
*/
public com.oracle.bmc.waiter.Waiter
forWorkRequest(
GetWorkRequestRequest request,
com.oracle.bmc.waiter.TerminationStrategy terminationStrategy,
com.oracle.bmc.waiter.DelayStrategy delayStrategy) {
return forWorkRequest(
com.oracle.bmc.waiter.Waiters.newWaiter(terminationStrategy, delayStrategy),
request);
}
// Helper method to create a new Waiter for WorkRequest.
private com.oracle.bmc.waiter.Waiter
forWorkRequest(
com.oracle.bmc.waiter.BmcGenericWaiter waiter,
final GetWorkRequestRequest request) {
return new com.oracle.bmc.waiter.internal.SimpleWaiterImpl<>(
executorService,
waiter.toCallable(
() -> request,
new java.util.function.Function<
GetWorkRequestRequest, GetWorkRequestResponse>() {
@Override
public GetWorkRequestResponse apply(GetWorkRequestRequest request) {
return client.getWorkRequest(request);
}
},
new java.util.function.Predicate() {
@Override
public boolean test(GetWorkRequestResponse response) {
// work requests are complete once the time finished is available
return response.getWorkRequest().getTimeFinished() != null;
}
},
false),
request);
}
}