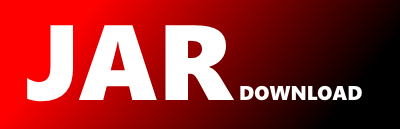
com.oracle.bmc.bds.BdsAsync Maven / Gradle / Ivy
Show all versions of oci-java-sdk-shaded-full Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.bds;
import com.oracle.bmc.bds.requests.*;
import com.oracle.bmc.bds.responses.*;
/**
* REST API for Oracle Big Data Service. Use this API to build, deploy, and manage fully elastic Big
* Data Service clusters. Build on Hadoop, Spark and Data Science distributions, which can be fully
* integrated with existing enterprise data in Oracle Database and Oracle applications.
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20190531")
public interface BdsAsync extends AutoCloseable {
/** Rebuilds the client from scratch. Useful to refresh certificates. */
void refreshClient();
/**
* Sets the endpoint to call (ex, https://www.example.com).
*
* @param endpoint The endpoint of the serice.
*/
void setEndpoint(String endpoint);
/** Gets the set endpoint for REST call (ex, https://www.example.com) */
String getEndpoint();
/**
* Sets the region to call (ex, Region.US_PHOENIX_1).
*
* Note, this will call {@link #setEndpoint(String) setEndpoint} after resolving the
* endpoint. If the service is not available in this region, however, an
* IllegalArgumentException will be raised.
*
* @param region The region of the service.
*/
void setRegion(com.oracle.bmc.Region region);
/**
* Sets the region to call (ex, 'us-phoenix-1').
*
*
Note, this will first try to map the region ID to a known Region and call {@link
* #setRegion(Region) setRegion}.
*
*
If no known Region could be determined, it will create an endpoint based on the default
* endpoint format ({@link com.oracle.bmc.Region#formatDefaultRegionEndpoint(Service, String)}
* and then call {@link #setEndpoint(String) setEndpoint}.
*
* @param regionId The public region ID.
*/
void setRegion(String regionId);
/**
* Determines whether realm specific endpoint should be used or not. Set
* realmSpecificEndpointTemplateEnabled to "true" if the user wants to enable use of realm
* specific endpoint template, otherwise set it to "false"
*
* @param realmSpecificEndpointTemplateEnabled flag to enable the use of realm specific endpoint
* template
*/
void useRealmSpecificEndpointTemplate(boolean realmSpecificEndpointTemplateEnabled);
/**
* Activate specified metastore configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
activateBdsMetastoreConfiguration(
ActivateBdsMetastoreConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
ActivateBdsMetastoreConfigurationRequest,
ActivateBdsMetastoreConfigurationResponse>
handler);
/**
* Add an autoscale configuration to the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addAutoScalingConfiguration(
AddAutoScalingConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
AddAutoScalingConfigurationRequest, AddAutoScalingConfigurationResponse>
handler);
/**
* Adds block storage to existing worker/compute only worker nodes. The same amount of storage
* will be added to all worker/compute only worker nodes. No change will be made to storage that
* is already attached. Block storage cannot be removed.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addBlockStorage(
AddBlockStorageRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Adds Cloud SQL to your cluster. You can use Cloud SQL to query against non-relational data
* stored in multiple big data sources, including Apache Hive, HDFS, Oracle NoSQL Database, and
* Apache HBase. Adding Cloud SQL adds a query server node to the cluster and creates cell
* servers on all the worker nodes in the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addCloudSql(
AddCloudSqlRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Adds Kafka to a cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addKafka(
AddKafkaRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Increases the size (scales out) of a cluster by adding master nodes. The added master nodes
* will have the same shape and will have the same amount of attached block storage as other
* master nodes in the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addMasterNodes(
AddMasterNodesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Increases the size (scales out) of a cluster by adding utility nodes. The added utility nodes
* will have the same shape and will have the same amount of attached block storage as other
* utility nodes in the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addUtilityNodes(
AddUtilityNodesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Increases the size (scales out) a cluster by adding worker nodes(data/compute). The added
* worker nodes will have the same shape and will have the same amount of attached block storage
* as other worker nodes in the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addWorkerNodes(
AddWorkerNodesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Takes a backup of of given nodes.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future backupNode(
BackupNodeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* A list of services and their certificate details.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future certificateServiceInfo(
CertificateServiceInfoRequest request,
com.oracle.bmc.responses.AsyncHandler<
CertificateServiceInfoRequest, CertificateServiceInfoResponse>
handler);
/**
* Moves a Big Data Service cluster into a different compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeBdsInstanceCompartment(
ChangeBdsInstanceCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeBdsInstanceCompartmentRequest,
ChangeBdsInstanceCompartmentResponse>
handler);
/**
* Changes the size of a cluster by scaling up or scaling down the nodes. Nodes are scaled up or
* down by changing the shapes of all the nodes of the same type to the next larger or smaller
* shape. The node types are master, utility, worker, and Cloud SQL. Only nodes with VM-STANDARD
* shapes can be scaled.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeShape(
ChangeShapeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Create an API key on behalf of the specified user.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createBdsApiKey(
CreateBdsApiKeyRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Creates a Big Data Service cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createBdsInstance(
CreateBdsInstanceRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateBdsInstanceRequest, CreateBdsInstanceResponse>
handler);
/**
* Create and activate external metastore configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createBdsMetastoreConfiguration(
CreateBdsMetastoreConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateBdsMetastoreConfigurationRequest,
CreateBdsMetastoreConfigurationResponse>
handler);
/**
* Add a node volume backup configuration to the cluster for an indicated node type or node.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createNodeBackupConfiguration(
CreateNodeBackupConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateNodeBackupConfigurationRequest,
CreateNodeBackupConfigurationResponse>
handler);
/**
* Add a nodeReplaceConfigurations to the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createNodeReplaceConfiguration(
CreateNodeReplaceConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateNodeReplaceConfigurationRequest,
CreateNodeReplaceConfigurationResponse>
handler);
/**
* Create a resource principal session token configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
createResourcePrincipalConfiguration(
CreateResourcePrincipalConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
CreateResourcePrincipalConfigurationRequest,
CreateResourcePrincipalConfigurationResponse>
handler);
/**
* Deletes the user's API key represented by the provided ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteBdsApiKey(
DeleteBdsApiKeyRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes the cluster identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteBdsInstance(
DeleteBdsInstanceRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteBdsInstanceRequest, DeleteBdsInstanceResponse>
handler);
/**
* Delete the BDS metastore configuration represented by the provided ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteBdsMetastoreConfiguration(
DeleteBdsMetastoreConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteBdsMetastoreConfigurationRequest,
DeleteBdsMetastoreConfigurationResponse>
handler);
/**
* Delete the NodeBackup represented by the provided ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteNodeBackup(
DeleteNodeBackupRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Delete the NodeBackupConfiguration represented by the provided ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteNodeBackupConfiguration(
DeleteNodeBackupConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteNodeBackupConfigurationRequest,
DeleteNodeBackupConfigurationResponse>
handler);
/**
* Disabling TLS/SSL for various ODH services running on the BDS cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future disableCertificate(
DisableCertificateRequest request,
com.oracle.bmc.responses.AsyncHandler<
DisableCertificateRequest, DisableCertificateResponse>
handler);
/**
* Configuring TLS/SSL for various ODH services running on the BDS cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future enableCertificate(
EnableCertificateRequest request,
com.oracle.bmc.responses.AsyncHandler<
EnableCertificateRequest, EnableCertificateResponse>
handler);
/**
* Execute bootstrap script.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future executeBootstrapScript(
ExecuteBootstrapScriptRequest request,
com.oracle.bmc.responses.AsyncHandler<
ExecuteBootstrapScriptRequest, ExecuteBootstrapScriptResponse>
handler);
/**
* Force Refresh Resource Principal for the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
forceRefreshResourcePrincipal(
ForceRefreshResourcePrincipalRequest request,
com.oracle.bmc.responses.AsyncHandler<
ForceRefreshResourcePrincipalRequest,
ForceRefreshResourcePrincipalResponse>
handler);
/**
* Returns details of the autoscale configuration identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getAutoScalingConfiguration(
GetAutoScalingConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetAutoScalingConfigurationRequest, GetAutoScalingConfigurationResponse>
handler);
/**
* Returns the user's API key information for the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getBdsApiKey(
GetBdsApiKeyRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns information about the Big Data Service cluster identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getBdsInstance(
GetBdsInstanceRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns the BDS Metastore configuration information for the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getBdsMetastoreConfiguration(
GetBdsMetastoreConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetBdsMetastoreConfigurationRequest,
GetBdsMetastoreConfigurationResponse>
handler);
/**
* Returns details of NodeBackup identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getNodeBackup(
GetNodeBackupRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns details of the NodeBackupConfiguration identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getNodeBackupConfiguration(
GetNodeBackupConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetNodeBackupConfigurationRequest, GetNodeBackupConfigurationResponse>
handler);
/**
* Returns details of the nodeReplaceConfiguration identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getNodeReplaceConfiguration(
GetNodeReplaceConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetNodeReplaceConfigurationRequest, GetNodeReplaceConfigurationResponse>
handler);
/**
* Get the details of an os patch
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getOsPatchDetails(
GetOsPatchDetailsRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetOsPatchDetailsRequest, GetOsPatchDetailsResponse>
handler);
/**
* Returns details of the resourcePrincipalConfiguration identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getResourcePrincipalConfiguration(
GetResourcePrincipalConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetResourcePrincipalConfigurationRequest,
GetResourcePrincipalConfigurationResponse>
handler);
/**
* Returns the status of the work request identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getWorkRequest(
GetWorkRequestRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Install an os patch on a cluster
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future installOsPatch(
InstallOsPatchRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Install the specified patch to this cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future installPatch(
InstallPatchRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns information about the autoscaling configurations for a cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
listAutoScalingConfigurations(
ListAutoScalingConfigurationsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListAutoScalingConfigurationsRequest,
ListAutoScalingConfigurationsResponse>
handler);
/**
* Returns a list of all API keys associated with this Big Data Service cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listBdsApiKeys(
ListBdsApiKeysRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns a list of all Big Data Service clusters in a compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listBdsInstances(
ListBdsInstancesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns a list of metastore configurations ssociated with this Big Data Service cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
listBdsMetastoreConfigurations(
ListBdsMetastoreConfigurationsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListBdsMetastoreConfigurationsRequest,
ListBdsMetastoreConfigurationsResponse>
handler);
/**
* Returns information about the NodeBackupConfigurations.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listNodeBackupConfigurations(
ListNodeBackupConfigurationsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListNodeBackupConfigurationsRequest,
ListNodeBackupConfigurationsResponse>
handler);
/**
* Returns information about the node Backups.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listNodeBackups(
ListNodeBackupsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns information about the NodeReplaceConfiguration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
listNodeReplaceConfigurations(
ListNodeReplaceConfigurationsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListNodeReplaceConfigurationsRequest,
ListNodeReplaceConfigurationsResponse>
handler);
/**
* List all available os patches for a given cluster
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listOsPatches(
ListOsPatchesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* List the patch history of this cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listPatchHistories(
ListPatchHistoriesRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListPatchHistoriesRequest, ListPatchHistoriesResponse>
handler);
/**
* List all the available patches for this cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listPatches(
ListPatchesRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Returns information about the ResourcePrincipalConfiguration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
listResourcePrincipalConfigurations(
ListResourcePrincipalConfigurationsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListResourcePrincipalConfigurationsRequest,
ListResourcePrincipalConfigurationsResponse>
handler);
/**
* Returns a paginated list of errors for a work request identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequestErrors(
ListWorkRequestErrorsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListWorkRequestErrorsRequest, ListWorkRequestErrorsResponse>
handler);
/**
* Returns a paginated list of logs for a given work request.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequestLogs(
ListWorkRequestLogsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListWorkRequestLogsRequest, ListWorkRequestLogsResponse>
handler);
/**
* Lists the work requests in a compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequests(
ListWorkRequestsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes an autoscale configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
removeAutoScalingConfiguration(
RemoveAutoScalingConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
RemoveAutoScalingConfigurationRequest,
RemoveAutoScalingConfigurationResponse>
handler);
/**
* Removes Cloud SQL from the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future removeCloudSql(
RemoveCloudSqlRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Remove Kafka from the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future removeKafka(
RemoveKafkaRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Remove a single node of a Big Data Service cluster
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future removeNode(
RemoveNodeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Deletes a nodeReplaceConfiguration
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
removeNodeReplaceConfiguration(
RemoveNodeReplaceConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
RemoveNodeReplaceConfigurationRequest,
RemoveNodeReplaceConfigurationResponse>
handler);
/**
* Delete the resource principal configuration for the cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
removeResourcePrincipalConfiguration(
RemoveResourcePrincipalConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
RemoveResourcePrincipalConfigurationRequest,
RemoveResourcePrincipalConfigurationResponse>
handler);
/**
* Renewing TLS/SSL for various ODH services running on the BDS cluster.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future renewCertificate(
RenewCertificateRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Replaces a node of a Big Data Service cluster from backup.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future replaceNode(
ReplaceNodeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Restarts a single node of a Big Data Service cluster
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future restartNode(
RestartNodeRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Starts the BDS cluster that was stopped earlier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future startBdsInstance(
StartBdsInstanceRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Stops the BDS cluster that can be started at later point of time.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future stopBdsInstance(
StopBdsInstanceRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Test specified metastore configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
testBdsMetastoreConfiguration(
TestBdsMetastoreConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
TestBdsMetastoreConfigurationRequest,
TestBdsMetastoreConfigurationResponse>
handler);
/**
* Test access to specified Object Storage bucket using the API key.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
testBdsObjectStorageConnection(
TestBdsObjectStorageConnectionRequest request,
com.oracle.bmc.responses.AsyncHandler<
TestBdsObjectStorageConnectionRequest,
TestBdsObjectStorageConnectionResponse>
handler);
/**
* Updates fields on an autoscale configuration, including the name, the threshold value, and
* whether the autoscale configuration is enabled.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateAutoScalingConfiguration(
UpdateAutoScalingConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateAutoScalingConfigurationRequest,
UpdateAutoScalingConfigurationResponse>
handler);
/**
* Updates the Big Data Service cluster identified by the given ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateBdsInstance(
UpdateBdsInstanceRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateBdsInstanceRequest, UpdateBdsInstanceResponse>
handler);
/**
* Update the BDS metastore configuration represented by the provided ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateBdsMetastoreConfiguration(
UpdateBdsMetastoreConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateBdsMetastoreConfigurationRequest,
UpdateBdsMetastoreConfigurationResponse>
handler);
/**
* Updates fields on NodeBackupConfiguration, including the name, the schedule.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateNodeBackupConfiguration(
UpdateNodeBackupConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateNodeBackupConfigurationRequest,
UpdateNodeBackupConfigurationResponse>
handler);
/**
* Updates fields on nodeReplaceConfigurations, including the name, the schedule
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateNodeReplaceConfiguration(
UpdateNodeReplaceConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateNodeReplaceConfigurationRequest,
UpdateNodeReplaceConfigurationResponse>
handler);
/**
* Updates fields on resourcePrincipalConfiguration, including the name, the lifeSpanInHours of
* the token.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateResourcePrincipalConfiguration(
UpdateResourcePrincipalConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateResourcePrincipalConfigurationRequest,
UpdateResourcePrincipalConfigurationResponse>
handler);
}