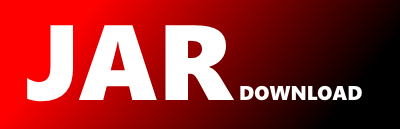
com.oracle.bmc.datascience.model.ModelDeployment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oci-java-sdk-shaded-full Show documentation
Show all versions of oci-java-sdk-shaded-full Show documentation
This project contains the SDK distribution used for Oracle Cloud Infrastructure, and all the dependencies that can be shaded. It also has Maven dependencies that cannot be shaded. Therefore, use this module to depend on the shaded distribution via Maven -- it will shade everything that can be shaded, and automatically pull in the other dependencies.
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.datascience.model;
/**
* Model deployments are used by data scientists to perform predictions from the model hosted on an
* HTTP server.
* Note: Objects should always be created or deserialized using the {@link Builder}. This model
* distinguishes fields that are {@code null} because they are unset from fields that are explicitly
* set to {@code null}. This is done in the setter methods of the {@link Builder}, which maintain a
* set of all explicitly set fields called {@link Builder#__explicitlySet__}. The {@link
* #hashCode()} and {@link #equals(Object)} methods are implemented to take the explicitly set
* fields into account. The constructor, on the other hand, does not take the explicitly set fields
* into account (since the constructor cannot distinguish explicit {@code null} from unset {@code
* null}).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20190101")
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = ModelDeployment.Builder.class)
@com.fasterxml.jackson.annotation.JsonFilter(
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public final class ModelDeployment
extends com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel {
@Deprecated
@java.beans.ConstructorProperties({
"id",
"timeCreated",
"displayName",
"description",
"projectId",
"createdBy",
"compartmentId",
"modelDeploymentConfigurationDetails",
"categoryLogDetails",
"modelDeploymentUrl",
"modelDeploymentSystemData",
"lifecycleState",
"lifecycleDetails",
"freeformTags",
"definedTags"
})
public ModelDeployment(
String id,
java.util.Date timeCreated,
String displayName,
String description,
String projectId,
String createdBy,
String compartmentId,
ModelDeploymentConfigurationDetails modelDeploymentConfigurationDetails,
CategoryLogDetails categoryLogDetails,
String modelDeploymentUrl,
ModelDeploymentSystemData modelDeploymentSystemData,
ModelDeploymentLifecycleState lifecycleState,
String lifecycleDetails,
java.util.Map freeformTags,
java.util.Map> definedTags) {
super();
this.id = id;
this.timeCreated = timeCreated;
this.displayName = displayName;
this.description = description;
this.projectId = projectId;
this.createdBy = createdBy;
this.compartmentId = compartmentId;
this.modelDeploymentConfigurationDetails = modelDeploymentConfigurationDetails;
this.categoryLogDetails = categoryLogDetails;
this.modelDeploymentUrl = modelDeploymentUrl;
this.modelDeploymentSystemData = modelDeploymentSystemData;
this.lifecycleState = lifecycleState;
this.lifecycleDetails = lifecycleDetails;
this.freeformTags = freeformTags;
this.definedTags = definedTags;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
public static class Builder {
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the model deployment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("id")
private String id;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the model deployment.
*
* @param id the value to set
* @return this builder
*/
public Builder id(String id) {
this.id = id;
this.__explicitlySet__.add("id");
return this;
}
/**
* The date and time the resource was created, in the timestamp format defined by
* [RFC3339](https://tools.ietf.org/html/rfc3339). Example: 2019-08-25T21:10:29.41Z
*/
@com.fasterxml.jackson.annotation.JsonProperty("timeCreated")
private java.util.Date timeCreated;
/**
* The date and time the resource was created, in the timestamp format defined by
* [RFC3339](https://tools.ietf.org/html/rfc3339). Example: 2019-08-25T21:10:29.41Z
*
* @param timeCreated the value to set
* @return this builder
*/
public Builder timeCreated(java.util.Date timeCreated) {
this.timeCreated = timeCreated;
this.__explicitlySet__.add("timeCreated");
return this;
}
/**
* A user-friendly display name for the resource. Does not have to be unique, and can be
* modified. Avoid entering confidential information. Example: {@code My ModelDeployment}
*/
@com.fasterxml.jackson.annotation.JsonProperty("displayName")
private String displayName;
/**
* A user-friendly display name for the resource. Does not have to be unique, and can be
* modified. Avoid entering confidential information. Example: {@code My ModelDeployment}
*
* @param displayName the value to set
* @return this builder
*/
public Builder displayName(String displayName) {
this.displayName = displayName;
this.__explicitlySet__.add("displayName");
return this;
}
/** A short description of the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private String description;
/**
* A short description of the model deployment.
*
* @param description the value to set
* @return this builder
*/
public Builder description(String description) {
this.description = description;
this.__explicitlySet__.add("description");
return this;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the project associated with the model deployment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("projectId")
private String projectId;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the project associated with the model deployment.
*
* @param projectId the value to set
* @return this builder
*/
public Builder projectId(String projectId) {
this.projectId = projectId;
this.__explicitlySet__.add("projectId");
return this;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the user who created the model deployment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("createdBy")
private String createdBy;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the user who created the model deployment.
*
* @param createdBy the value to set
* @return this builder
*/
public Builder createdBy(String createdBy) {
this.createdBy = createdBy;
this.__explicitlySet__.add("createdBy");
return this;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the model deployment's compartment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private String compartmentId;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm)
* of the model deployment's compartment.
*
* @param compartmentId the value to set
* @return this builder
*/
public Builder compartmentId(String compartmentId) {
this.compartmentId = compartmentId;
this.__explicitlySet__.add("compartmentId");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("modelDeploymentConfigurationDetails")
private ModelDeploymentConfigurationDetails modelDeploymentConfigurationDetails;
public Builder modelDeploymentConfigurationDetails(
ModelDeploymentConfigurationDetails modelDeploymentConfigurationDetails) {
this.modelDeploymentConfigurationDetails = modelDeploymentConfigurationDetails;
this.__explicitlySet__.add("modelDeploymentConfigurationDetails");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("categoryLogDetails")
private CategoryLogDetails categoryLogDetails;
public Builder categoryLogDetails(CategoryLogDetails categoryLogDetails) {
this.categoryLogDetails = categoryLogDetails;
this.__explicitlySet__.add("categoryLogDetails");
return this;
}
/** The URL to interact with the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("modelDeploymentUrl")
private String modelDeploymentUrl;
/**
* The URL to interact with the model deployment.
*
* @param modelDeploymentUrl the value to set
* @return this builder
*/
public Builder modelDeploymentUrl(String modelDeploymentUrl) {
this.modelDeploymentUrl = modelDeploymentUrl;
this.__explicitlySet__.add("modelDeploymentUrl");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("modelDeploymentSystemData")
private ModelDeploymentSystemData modelDeploymentSystemData;
public Builder modelDeploymentSystemData(
ModelDeploymentSystemData modelDeploymentSystemData) {
this.modelDeploymentSystemData = modelDeploymentSystemData;
this.__explicitlySet__.add("modelDeploymentSystemData");
return this;
}
/** The state of the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleState")
private ModelDeploymentLifecycleState lifecycleState;
/**
* The state of the model deployment.
*
* @param lifecycleState the value to set
* @return this builder
*/
public Builder lifecycleState(ModelDeploymentLifecycleState lifecycleState) {
this.lifecycleState = lifecycleState;
this.__explicitlySet__.add("lifecycleState");
return this;
}
/** Details about the state of the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleDetails")
private String lifecycleDetails;
/**
* Details about the state of the model deployment.
*
* @param lifecycleDetails the value to set
* @return this builder
*/
public Builder lifecycleDetails(String lifecycleDetails) {
this.lifecycleDetails = lifecycleDetails;
this.__explicitlySet__.add("lifecycleDetails");
return this;
}
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. See [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm). Example:
* {@code {"Department": "Finance"}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("freeformTags")
private java.util.Map freeformTags;
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. See [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm). Example:
* {@code {"Department": "Finance"}}
*
* @param freeformTags the value to set
* @return this builder
*/
public Builder freeformTags(java.util.Map freeformTags) {
this.freeformTags = freeformTags;
this.__explicitlySet__.add("freeformTags");
return this;
}
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. See
* [Resource Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("definedTags")
private java.util.Map> definedTags;
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. See
* [Resource Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*
* @param definedTags the value to set
* @return this builder
*/
public Builder definedTags(
java.util.Map> definedTags) {
this.definedTags = definedTags;
this.__explicitlySet__.add("definedTags");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public ModelDeployment build() {
ModelDeployment model =
new ModelDeployment(
this.id,
this.timeCreated,
this.displayName,
this.description,
this.projectId,
this.createdBy,
this.compartmentId,
this.modelDeploymentConfigurationDetails,
this.categoryLogDetails,
this.modelDeploymentUrl,
this.modelDeploymentSystemData,
this.lifecycleState,
this.lifecycleDetails,
this.freeformTags,
this.definedTags);
for (String explicitlySetProperty : this.__explicitlySet__) {
model.markPropertyAsExplicitlySet(explicitlySetProperty);
}
return model;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(ModelDeployment model) {
if (model.wasPropertyExplicitlySet("id")) {
this.id(model.getId());
}
if (model.wasPropertyExplicitlySet("timeCreated")) {
this.timeCreated(model.getTimeCreated());
}
if (model.wasPropertyExplicitlySet("displayName")) {
this.displayName(model.getDisplayName());
}
if (model.wasPropertyExplicitlySet("description")) {
this.description(model.getDescription());
}
if (model.wasPropertyExplicitlySet("projectId")) {
this.projectId(model.getProjectId());
}
if (model.wasPropertyExplicitlySet("createdBy")) {
this.createdBy(model.getCreatedBy());
}
if (model.wasPropertyExplicitlySet("compartmentId")) {
this.compartmentId(model.getCompartmentId());
}
if (model.wasPropertyExplicitlySet("modelDeploymentConfigurationDetails")) {
this.modelDeploymentConfigurationDetails(
model.getModelDeploymentConfigurationDetails());
}
if (model.wasPropertyExplicitlySet("categoryLogDetails")) {
this.categoryLogDetails(model.getCategoryLogDetails());
}
if (model.wasPropertyExplicitlySet("modelDeploymentUrl")) {
this.modelDeploymentUrl(model.getModelDeploymentUrl());
}
if (model.wasPropertyExplicitlySet("modelDeploymentSystemData")) {
this.modelDeploymentSystemData(model.getModelDeploymentSystemData());
}
if (model.wasPropertyExplicitlySet("lifecycleState")) {
this.lifecycleState(model.getLifecycleState());
}
if (model.wasPropertyExplicitlySet("lifecycleDetails")) {
this.lifecycleDetails(model.getLifecycleDetails());
}
if (model.wasPropertyExplicitlySet("freeformTags")) {
this.freeformTags(model.getFreeformTags());
}
if (model.wasPropertyExplicitlySet("definedTags")) {
this.definedTags(model.getDefinedTags());
}
return this;
}
}
/** Create a new builder. */
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return new Builder().copy(this);
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the model deployment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("id")
private final String id;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the model deployment.
*
* @return the value
*/
public String getId() {
return id;
}
/**
* The date and time the resource was created, in the timestamp format defined by
* [RFC3339](https://tools.ietf.org/html/rfc3339). Example: 2019-08-25T21:10:29.41Z
*/
@com.fasterxml.jackson.annotation.JsonProperty("timeCreated")
private final java.util.Date timeCreated;
/**
* The date and time the resource was created, in the timestamp format defined by
* [RFC3339](https://tools.ietf.org/html/rfc3339). Example: 2019-08-25T21:10:29.41Z
*
* @return the value
*/
public java.util.Date getTimeCreated() {
return timeCreated;
}
/**
* A user-friendly display name for the resource. Does not have to be unique, and can be
* modified. Avoid entering confidential information. Example: {@code My ModelDeployment}
*/
@com.fasterxml.jackson.annotation.JsonProperty("displayName")
private final String displayName;
/**
* A user-friendly display name for the resource. Does not have to be unique, and can be
* modified. Avoid entering confidential information. Example: {@code My ModelDeployment}
*
* @return the value
*/
public String getDisplayName() {
return displayName;
}
/** A short description of the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private final String description;
/**
* A short description of the model deployment.
*
* @return the value
*/
public String getDescription() {
return description;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the project associated with the model deployment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("projectId")
private final String projectId;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the project associated with the model deployment.
*
* @return the value
*/
public String getProjectId() {
return projectId;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the user who created the model deployment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("createdBy")
private final String createdBy;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the user who created the model deployment.
*
* @return the value
*/
public String getCreatedBy() {
return createdBy;
}
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the model deployment's compartment.
*/
@com.fasterxml.jackson.annotation.JsonProperty("compartmentId")
private final String compartmentId;
/**
* The [OCID](https://docs.cloud.oracle.com/iaas/Content/General/Concepts/identifiers.htm) of
* the model deployment's compartment.
*
* @return the value
*/
public String getCompartmentId() {
return compartmentId;
}
@com.fasterxml.jackson.annotation.JsonProperty("modelDeploymentConfigurationDetails")
private final ModelDeploymentConfigurationDetails modelDeploymentConfigurationDetails;
public ModelDeploymentConfigurationDetails getModelDeploymentConfigurationDetails() {
return modelDeploymentConfigurationDetails;
}
@com.fasterxml.jackson.annotation.JsonProperty("categoryLogDetails")
private final CategoryLogDetails categoryLogDetails;
public CategoryLogDetails getCategoryLogDetails() {
return categoryLogDetails;
}
/** The URL to interact with the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("modelDeploymentUrl")
private final String modelDeploymentUrl;
/**
* The URL to interact with the model deployment.
*
* @return the value
*/
public String getModelDeploymentUrl() {
return modelDeploymentUrl;
}
@com.fasterxml.jackson.annotation.JsonProperty("modelDeploymentSystemData")
private final ModelDeploymentSystemData modelDeploymentSystemData;
public ModelDeploymentSystemData getModelDeploymentSystemData() {
return modelDeploymentSystemData;
}
/** The state of the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleState")
private final ModelDeploymentLifecycleState lifecycleState;
/**
* The state of the model deployment.
*
* @return the value
*/
public ModelDeploymentLifecycleState getLifecycleState() {
return lifecycleState;
}
/** Details about the state of the model deployment. */
@com.fasterxml.jackson.annotation.JsonProperty("lifecycleDetails")
private final String lifecycleDetails;
/**
* Details about the state of the model deployment.
*
* @return the value
*/
public String getLifecycleDetails() {
return lifecycleDetails;
}
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. See [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm). Example:
* {@code {"Department": "Finance"}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("freeformTags")
private final java.util.Map freeformTags;
/**
* Free-form tags for this resource. Each tag is a simple key-value pair with no predefined
* name, type, or namespace. See [Resource
* Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm). Example:
* {@code {"Department": "Finance"}}
*
* @return the value
*/
public java.util.Map getFreeformTags() {
return freeformTags;
}
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. See
* [Resource Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*/
@com.fasterxml.jackson.annotation.JsonProperty("definedTags")
private final java.util.Map> definedTags;
/**
* Defined tags for this resource. Each key is predefined and scoped to a namespace. See
* [Resource Tags](https://docs.cloud.oracle.com/Content/General/Concepts/resourcetags.htm).
* Example: {@code {"Operations": {"CostCenter": "42"}}}
*
* @return the value
*/
public java.util.Map> getDefinedTags() {
return definedTags;
}
@Override
public String toString() {
return this.toString(true);
}
/**
* Return a string representation of the object.
*
* @param includeByteArrayContents true to include the full contents of byte arrays
* @return string representation
*/
public String toString(boolean includeByteArrayContents) {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("ModelDeployment(");
sb.append("super=").append(super.toString());
sb.append("id=").append(String.valueOf(this.id));
sb.append(", timeCreated=").append(String.valueOf(this.timeCreated));
sb.append(", displayName=").append(String.valueOf(this.displayName));
sb.append(", description=").append(String.valueOf(this.description));
sb.append(", projectId=").append(String.valueOf(this.projectId));
sb.append(", createdBy=").append(String.valueOf(this.createdBy));
sb.append(", compartmentId=").append(String.valueOf(this.compartmentId));
sb.append(", modelDeploymentConfigurationDetails=")
.append(String.valueOf(this.modelDeploymentConfigurationDetails));
sb.append(", categoryLogDetails=").append(String.valueOf(this.categoryLogDetails));
sb.append(", modelDeploymentUrl=").append(String.valueOf(this.modelDeploymentUrl));
sb.append(", modelDeploymentSystemData=")
.append(String.valueOf(this.modelDeploymentSystemData));
sb.append(", lifecycleState=").append(String.valueOf(this.lifecycleState));
sb.append(", lifecycleDetails=").append(String.valueOf(this.lifecycleDetails));
sb.append(", freeformTags=").append(String.valueOf(this.freeformTags));
sb.append(", definedTags=").append(String.valueOf(this.definedTags));
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ModelDeployment)) {
return false;
}
ModelDeployment other = (ModelDeployment) o;
return java.util.Objects.equals(this.id, other.id)
&& java.util.Objects.equals(this.timeCreated, other.timeCreated)
&& java.util.Objects.equals(this.displayName, other.displayName)
&& java.util.Objects.equals(this.description, other.description)
&& java.util.Objects.equals(this.projectId, other.projectId)
&& java.util.Objects.equals(this.createdBy, other.createdBy)
&& java.util.Objects.equals(this.compartmentId, other.compartmentId)
&& java.util.Objects.equals(
this.modelDeploymentConfigurationDetails,
other.modelDeploymentConfigurationDetails)
&& java.util.Objects.equals(this.categoryLogDetails, other.categoryLogDetails)
&& java.util.Objects.equals(this.modelDeploymentUrl, other.modelDeploymentUrl)
&& java.util.Objects.equals(
this.modelDeploymentSystemData, other.modelDeploymentSystemData)
&& java.util.Objects.equals(this.lifecycleState, other.lifecycleState)
&& java.util.Objects.equals(this.lifecycleDetails, other.lifecycleDetails)
&& java.util.Objects.equals(this.freeformTags, other.freeformTags)
&& java.util.Objects.equals(this.definedTags, other.definedTags)
&& super.equals(other);
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = (result * PRIME) + (this.id == null ? 43 : this.id.hashCode());
result = (result * PRIME) + (this.timeCreated == null ? 43 : this.timeCreated.hashCode());
result = (result * PRIME) + (this.displayName == null ? 43 : this.displayName.hashCode());
result = (result * PRIME) + (this.description == null ? 43 : this.description.hashCode());
result = (result * PRIME) + (this.projectId == null ? 43 : this.projectId.hashCode());
result = (result * PRIME) + (this.createdBy == null ? 43 : this.createdBy.hashCode());
result =
(result * PRIME)
+ (this.compartmentId == null ? 43 : this.compartmentId.hashCode());
result =
(result * PRIME)
+ (this.modelDeploymentConfigurationDetails == null
? 43
: this.modelDeploymentConfigurationDetails.hashCode());
result =
(result * PRIME)
+ (this.categoryLogDetails == null
? 43
: this.categoryLogDetails.hashCode());
result =
(result * PRIME)
+ (this.modelDeploymentUrl == null
? 43
: this.modelDeploymentUrl.hashCode());
result =
(result * PRIME)
+ (this.modelDeploymentSystemData == null
? 43
: this.modelDeploymentSystemData.hashCode());
result =
(result * PRIME)
+ (this.lifecycleState == null ? 43 : this.lifecycleState.hashCode());
result =
(result * PRIME)
+ (this.lifecycleDetails == null ? 43 : this.lifecycleDetails.hashCode());
result = (result * PRIME) + (this.freeformTags == null ? 43 : this.freeformTags.hashCode());
result = (result * PRIME) + (this.definedTags == null ? 43 : this.definedTags.hashCode());
result = (result * PRIME) + super.hashCode();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy