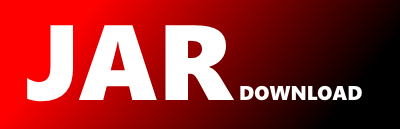
com.oracle.bmc.jms.JavaManagementServiceAsync Maven / Gradle / Ivy
Show all versions of oci-java-sdk-shaded-full Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.jms;
import com.oracle.bmc.jms.requests.*;
import com.oracle.bmc.jms.responses.*;
/**
* The APIs for the [Fleet
* Management](https://docs.oracle.com/en-us/iaas/jms/doc/fleet-management.html) feature of Java
* Management Service to monitor and manage the usage of Java in your enterprise. Use these APIs to
* manage fleets, configure managed instances to report to fleets, and gain insights into the Java
* workloads running on these instances by carrying out basic and advanced features.
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20210610")
public interface JavaManagementServiceAsync extends AutoCloseable {
/** Rebuilds the client from scratch. Useful to refresh certificates. */
void refreshClient();
/**
* Sets the endpoint to call (ex, https://www.example.com).
*
* @param endpoint The endpoint of the serice.
*/
void setEndpoint(String endpoint);
/** Gets the set endpoint for REST call (ex, https://www.example.com) */
String getEndpoint();
/**
* Sets the region to call (ex, Region.US_PHOENIX_1).
*
* Note, this will call {@link #setEndpoint(String) setEndpoint} after resolving the
* endpoint. If the service is not available in this region, however, an
* IllegalArgumentException will be raised.
*
* @param region The region of the service.
*/
void setRegion(com.oracle.bmc.Region region);
/**
* Sets the region to call (ex, 'us-phoenix-1').
*
*
Note, this will first try to map the region ID to a known Region and call {@link
* #setRegion(Region) setRegion}.
*
*
If no known Region could be determined, it will create an endpoint based on the default
* endpoint format ({@link com.oracle.bmc.Region#formatDefaultRegionEndpoint(Service, String)}
* and then call {@link #setEndpoint(String) setEndpoint}.
*
* @param regionId The public region ID.
*/
void setRegion(String regionId);
/**
* Determines whether realm specific endpoint should be used or not. Set
* realmSpecificEndpointTemplateEnabled to "true" if the user wants to enable use of realm
* specific endpoint template, otherwise set it to "false"
*
* @param realmSpecificEndpointTemplateEnabled flag to enable the use of realm specific endpoint
* template
*/
void useRealmSpecificEndpointTemplate(boolean realmSpecificEndpointTemplateEnabled);
/**
* Add Java installation sites in a Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future addFleetInstallationSites(
AddFleetInstallationSitesRequest request,
com.oracle.bmc.responses.AsyncHandler<
AddFleetInstallationSitesRequest, AddFleetInstallationSitesResponse>
handler);
/**
* Deletes the work request specified by an identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future cancelWorkRequest(
CancelWorkRequestRequest request,
com.oracle.bmc.responses.AsyncHandler<
CancelWorkRequestRequest, CancelWorkRequestResponse>
handler);
/**
* Move a specified Fleet into the compartment identified in the POST form. When provided,
* If-Match is checked against ETag values of the resource.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future changeFleetCompartment(
ChangeFleetCompartmentRequest request,
com.oracle.bmc.responses.AsyncHandler<
ChangeFleetCompartmentRequest, ChangeFleetCompartmentResponse>
handler);
/**
* Add a new record to the fleet blocklist.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createBlocklist(
CreateBlocklistRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Request to perform validation of the DRS file and create the file to the Object Storage.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createDrsFile(
CreateDrsFileRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Create a new Fleet using the information provided.
*
* `inventoryLog` is now a required parameter for CreateFleet API. Update existing
* applications using this API before July 15, 2022 to ensure the applications continue to work.
* See the [Service Change
* Notice](https://docs.oracle.com/en-us/iaas/Content/servicechanges.htm#JMS) for more details.
* Migrate existing fleets using the `UpdateFleet` API to set the `inventoryLog` parameter.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createFleet(
CreateFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Registers an agent's JmsPlugin, optionally attaching to an existing fleet of the tenancy.
* JmsPlugins registered fleet-less are created with lifecycle state INACTIVE. For the operation
* to be authorized, the agent must exist, and the authorized user requires JMS_PLUGIN_CREATE
* permission for the agent's compartment.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future createJmsPlugin(
CreateJmsPluginRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes the blocklist record specified by an identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteBlocklist(
DeleteBlocklistRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes the metadata for the result of a Crypto event analysis. The actual report shall
* remain in the object storage.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteCryptoAnalysisResult(
DeleteCryptoAnalysisResultRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteCryptoAnalysisResultRequest, DeleteCryptoAnalysisResultResponse>
handler);
/**
* Request to delete the DRS file from the Object Storage.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteDrsFile(
DeleteDrsFileRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes the Fleet specified by an identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteFleet(
DeleteFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Delete the Java migration analysis result. The actual report will remain in the Object
* Storage bucket.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deleteJavaMigrationAnalysisResult(
DeleteJavaMigrationAnalysisResultRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeleteJavaMigrationAnalysisResultRequest,
DeleteJavaMigrationAnalysisResultResponse>
handler);
/**
* Deletes a JmsPlugin. The JmsPlugin may be visible for some time with state DELETED. Deleted
* plugins will not be able to communicate with the JMS service.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future deleteJmsPlugin(
DeleteJmsPluginRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Deletes only the metadata of the Performance Tuning Analysis result, but the file remains in
* the object storage.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
deletePerformanceTuningAnalysisResult(
DeletePerformanceTuningAnalysisResultRequest request,
com.oracle.bmc.responses.AsyncHandler<
DeletePerformanceTuningAnalysisResultRequest,
DeletePerformanceTuningAnalysisResultResponse>
handler);
/**
* Request to disable the DRS in the selected target in the Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future disableDrs(
DisableDrsRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Request to enable the DRS in the selected target in the Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future enableDrs(
EnableDrsRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Generates Agent Deploy Script for Fleet using the information provided.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future generateAgentDeployScript(
GenerateAgentDeployScriptRequest request,
com.oracle.bmc.responses.AsyncHandler<
GenerateAgentDeployScriptRequest, GenerateAgentDeployScriptResponse>
handler);
/**
* Generates the agent installer configuration using the information provided.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
generateAgentInstallerConfiguration(
GenerateAgentInstallerConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GenerateAgentInstallerConfigurationRequest,
GenerateAgentInstallerConfigurationResponse>
handler);
/**
* Generates Load Pipeline Script
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future generateLoadPipelineScript(
GenerateLoadPipelineScriptRequest request,
com.oracle.bmc.responses.AsyncHandler<
GenerateLoadPipelineScriptRequest, GenerateLoadPipelineScriptResponse>
handler);
/**
* Retrieve the metadata for the result of a Crypto event analysis.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getCryptoAnalysisResult(
GetCryptoAnalysisResultRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetCryptoAnalysisResultRequest, GetCryptoAnalysisResultResponse>
handler);
/**
* Get the detail about the created DRS file in the Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getDrsFile(
GetDrsFileRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Returns export setting for the specified fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExportSetting(
GetExportSettingRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns last export status for the specified fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getExportStatus(
GetExportStatusRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Retrieve a Fleet with the specified identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getFleet(
GetFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Returns Fleet level advanced feature configuration.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getFleetAdvancedFeatureConfiguration(
GetFleetAdvancedFeatureConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetFleetAdvancedFeatureConfigurationRequest,
GetFleetAdvancedFeatureConfigurationResponse>
handler);
/**
* Retrieve a Fleet Agent Configuration for the specified Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getFleetAgentConfiguration(
GetFleetAgentConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetFleetAgentConfigurationRequest, GetFleetAgentConfigurationResponse>
handler);
/**
* Returns metadata associated with a specific Java release family.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getJavaFamily(
GetJavaFamilyRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Retrieve Java Migration Analysis result.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getJavaMigrationAnalysisResult(
GetJavaMigrationAnalysisResultRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetJavaMigrationAnalysisResultRequest,
GetJavaMigrationAnalysisResultResponse>
handler);
/**
* Returns detail of a Java release.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getJavaRelease(
GetJavaReleaseRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns the JmsPlugin.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getJmsPlugin(
GetJmsPluginRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Retrieve metadata of the Performance Tuning Analysis result.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
getPerformanceTuningAnalysisResult(
GetPerformanceTuningAnalysisResultRequest request,
com.oracle.bmc.responses.AsyncHandler<
GetPerformanceTuningAnalysisResultRequest,
GetPerformanceTuningAnalysisResultResponse>
handler);
/**
* Retrieve the details of a work request with the specified ID.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future getWorkRequest(
GetWorkRequestRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Returns a list of the agent installer information.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listAgentInstallers(
ListAgentInstallersRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListAgentInstallersRequest, ListAgentInstallersResponse>
handler);
/**
* Return a list of AnnouncementSummary items
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listAnnouncements(
ListAnnouncementsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListAnnouncementsRequest, ListAnnouncementsResponse>
handler);
/**
* Returns a list of blocklist entities contained by a fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listBlocklists(
ListBlocklistsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Lists the results of a Crypto event analysis.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listCryptoAnalysisResults(
ListCryptoAnalysisResultsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListCryptoAnalysisResultsRequest, ListCryptoAnalysisResultsResponse>
handler);
/**
* List the details about the created DRS files in the Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listDrsFiles(
ListDrsFilesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* List potential diagnoses that would put a fleet into FAILED or NEEDS_ATTENTION lifecycle
* state.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleetDiagnoses(
ListFleetDiagnosesRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListFleetDiagnosesRequest, ListFleetDiagnosesResponse>
handler);
/**
* Returns a list of all the Fleets contained by a compartment. The query parameter
* `compartmentId` is required unless the query parameter `id` is specified.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listFleets(
ListFleetsRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* List Java installation sites in a Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listInstallationSites(
ListInstallationSitesRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListInstallationSitesRequest, ListInstallationSitesResponse>
handler);
/**
* Returns a list of the Java release family information. A Java release family is typically a
* major version in the Java version identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listJavaFamilies(
ListJavaFamiliesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Lists the results of a Java migration analysis.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
listJavaMigrationAnalysisResults(
ListJavaMigrationAnalysisResultsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListJavaMigrationAnalysisResultsRequest,
ListJavaMigrationAnalysisResultsResponse>
handler);
/**
* Returns a list of Java releases.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listJavaReleases(
ListJavaReleasesRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Lists the JmsPlugins.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listJmsPlugins(
ListJmsPluginsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* List Java Runtime usage in a specified host filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listJreUsage(
ListJreUsageRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* List Performance Tuning Analysis results.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
listPerformanceTuningAnalysisResults(
ListPerformanceTuningAnalysisResultsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListPerformanceTuningAnalysisResultsRequest,
ListPerformanceTuningAnalysisResultsResponse>
handler);
/**
* Retrieve a paginated list of work items for a specified work request.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkItems(
ListWorkItemsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Retrieve a (paginated) list of errors for a specified work request.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequestErrors(
ListWorkRequestErrorsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListWorkRequestErrorsRequest, ListWorkRequestErrorsResponse>
handler);
/**
* Retrieve a paginated list of logs for a specified work request.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequestLogs(
ListWorkRequestLogsRequest request,
com.oracle.bmc.responses.AsyncHandler<
ListWorkRequestLogsRequest, ListWorkRequestLogsResponse>
handler);
/**
* List the work requests in a compartment. The query parameter `compartmentId` is required
* unless the query parameter `id` or `fleetId` is specified.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future listWorkRequests(
ListWorkRequestsRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Remove Java installation sites in a Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future removeFleetInstallationSites(
RemoveFleetInstallationSitesRequest request,
com.oracle.bmc.responses.AsyncHandler<
RemoveFleetInstallationSitesRequest,
RemoveFleetInstallationSitesResponse>
handler);
/**
* Request to perform crypto analysis on one or more selected targets in the Fleet. The result
* of the crypto analysis will be uploaded to the object storage bucket created by JMS on
* enabling the Crypto Event Analysis feature in the Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future requestCryptoAnalyses(
RequestCryptoAnalysesRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestCryptoAnalysesRequest, RequestCryptoAnalysesResponse>
handler);
/**
* Request to perform a deployed Java migration analyses. The results of the deployed Java
* migration analyses will be uploaded to the Object Storage bucket that you designate when you
* enable the Java Migration Analyses feature.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
requestDeployedApplicationMigrationAnalyses(
RequestDeployedApplicationMigrationAnalysesRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestDeployedApplicationMigrationAnalysesRequest,
RequestDeployedApplicationMigrationAnalysesResponse>
handler);
/**
* Request to perform a Java migration analysis. The results of the Java migration analysis will
* be uploaded to the Object Storage bucket that you designate when you enable the Java
* Migration Analysis feature.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future requestJavaMigrationAnalyses(
RequestJavaMigrationAnalysesRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestJavaMigrationAnalysesRequest,
RequestJavaMigrationAnalysesResponse>
handler);
/**
* Request to collect the JFR recordings on the selected target in the Fleet. The JFR files are
* uploaded to the object storage bucket created by JMS on enabling Generic JFR feature in the
* Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future requestJfrRecordings(
RequestJfrRecordingsRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestJfrRecordingsRequest, RequestJfrRecordingsResponse>
handler);
/**
* Request to perform performance tuning analyses. The result of performance tuning analysis
* will be uploaded to the object storage bucket that you designated when you enabled the
* recording feature.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
requestPerformanceTuningAnalyses(
RequestPerformanceTuningAnalysesRequest request,
com.oracle.bmc.responses.AsyncHandler<
RequestPerformanceTuningAnalysesRequest,
RequestPerformanceTuningAnalysesResponse>
handler);
/**
* Scan Java Server usage in a fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future scanJavaServerUsage(
ScanJavaServerUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
ScanJavaServerUsageRequest, ScanJavaServerUsageResponse>
handler);
/**
* Scan library usage in a fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future scanLibraryUsage(
ScanLibraryUsageRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Summarizes the application installation usage in a Fleet filtered by query parameters. In
* contrast to SummarizeApplicationUsage, which provides only information aggregated by
* application name, this operation provides installation details. This allows for better
* focusing of actions.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
summarizeApplicationInstallationUsage(
SummarizeApplicationInstallationUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeApplicationInstallationUsageRequest,
SummarizeApplicationInstallationUsageResponse>
handler);
/**
* List application usage in a Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future summarizeApplicationUsage(
SummarizeApplicationUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeApplicationUsageRequest, SummarizeApplicationUsageResponse>
handler);
/**
* Summarize installation usage of an application deployed on Java servers in a fleet filtered
* by query parameters. In contrast to SummarizeDeployedApplicationUsage, which provides only
* information aggregated by the deployment information, this operation provides installation
* details and allows for better focusing of actions.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
summarizeDeployedApplicationInstallationUsage(
SummarizeDeployedApplicationInstallationUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeDeployedApplicationInstallationUsageRequest,
SummarizeDeployedApplicationInstallationUsageResponse>
handler);
/**
* List of deployed applications in a Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
summarizeDeployedApplicationUsage(
SummarizeDeployedApplicationUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeDeployedApplicationUsageRequest,
SummarizeDeployedApplicationUsageResponse>
handler);
/**
* List Java installation usage in a Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future summarizeInstallationUsage(
SummarizeInstallationUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeInstallationUsageRequest, SummarizeInstallationUsageResponse>
handler);
/**
* List Java Server instances in a fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
summarizeJavaServerInstanceUsage(
SummarizeJavaServerInstanceUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeJavaServerInstanceUsageRequest,
SummarizeJavaServerInstanceUsageResponse>
handler);
/**
* List of Java servers in a Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future summarizeJavaServerUsage(
SummarizeJavaServerUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeJavaServerUsageRequest, SummarizeJavaServerUsageResponse>
handler);
/**
* List Java Runtime usage in a specified Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future summarizeJreUsage(
SummarizeJreUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeJreUsageRequest, SummarizeJreUsageResponse>
handler);
/**
* List libraries in a fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future summarizeLibraryUsage(
SummarizeLibraryUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeLibraryUsageRequest, SummarizeLibraryUsageResponse>
handler);
/**
* List managed instance usage in a Fleet filtered by query parameters.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
summarizeManagedInstanceUsage(
SummarizeManagedInstanceUsageRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeManagedInstanceUsageRequest,
SummarizeManagedInstanceUsageResponse>
handler);
/**
* Retrieve the inventory of JMS resources in the specified compartment: a list of the number of
* _active_ fleets, managed instances, Java Runtimes, Java installations, and applications.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future summarizeResourceInventory(
SummarizeResourceInventoryRequest request,
com.oracle.bmc.responses.AsyncHandler<
SummarizeResourceInventoryRequest, SummarizeResourceInventoryResponse>
handler);
/**
* Request to perform validation of the DRS file and update the existing file in the Object
* Storage.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateDrsFile(
UpdateDrsFileRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
/**
* Updates existing export setting for the specified fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateExportSetting(
UpdateExportSettingRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateExportSettingRequest, UpdateExportSettingResponse>
handler);
/**
* Update the Fleet specified by an identifier.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateFleet(
UpdateFleetRequest request,
com.oracle.bmc.responses.AsyncHandler handler);
/**
* Update advanced feature configurations for the Fleet. Ensure that the namespace and bucket
* storage are created prior to turning on the JfrRecording or CryptoEventAnalysis feature.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateFleetAdvancedFeatureConfiguration(
UpdateFleetAdvancedFeatureConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateFleetAdvancedFeatureConfigurationRequest,
UpdateFleetAdvancedFeatureConfigurationResponse>
handler);
/**
* Update the Fleet Agent Configuration for the specified Fleet.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future
updateFleetAgentConfiguration(
UpdateFleetAgentConfigurationRequest request,
com.oracle.bmc.responses.AsyncHandler<
UpdateFleetAgentConfigurationRequest,
UpdateFleetAgentConfigurationResponse>
handler);
/**
* Updates the Fleet of a JmsPlugin.
*
* @param request The request object containing the details to send
* @param handler The request handler to invoke upon completion, may be null.
* @return A Future that can be used to get the response if no AsyncHandler was provided. Note,
* if you provide an AsyncHandler and use the Future, some types of responses (like
* java.io.InputStream) may not be able to be read in both places as the underlying stream
* may only be consumed once.
*/
java.util.concurrent.Future updateJmsPlugin(
UpdateJmsPluginRequest request,
com.oracle.bmc.responses.AsyncHandler
handler);
}