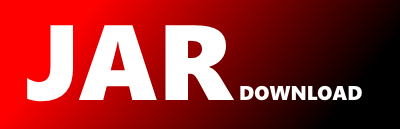
com.oracle.bmc.loganalytics.model.LabelSourceSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oci-java-sdk-shaded-full Show documentation
Show all versions of oci-java-sdk-shaded-full Show documentation
This project contains the SDK distribution used for Oracle Cloud Infrastructure, and all the dependencies that can be shaded. It also has Maven dependencies that cannot be shaded. Therefore, use this module to depend on the shaded distribution via Maven -- it will shade everything that can be shaded, and automatically pull in the other dependencies.
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.loganalytics.model;
/**
* source summary
* Note: Objects should always be created or deserialized using the {@link Builder}. This model
* distinguishes fields that are {@code null} because they are unset from fields that are explicitly
* set to {@code null}. This is done in the setter methods of the {@link Builder}, which maintain a
* set of all explicitly set fields called {@link Builder#__explicitlySet__}. The {@link
* #hashCode()} and {@link #equals(Object)} methods are implemented to take the explicitly set
* fields into account. The constructor, on the other hand, does not take the explicitly set fields
* into account (since the constructor cannot distinguish explicit {@code null} from unset {@code
* null}).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20200601")
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(
builder = LabelSourceSummary.Builder.class)
@com.fasterxml.jackson.annotation.JsonFilter(
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public final class LabelSourceSummary
extends com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel {
@Deprecated
@java.beans.ConstructorProperties({
"sourceDisplayName",
"sourceName",
"sourceId",
"labelOperatorName",
"labelCondition",
"conditionString",
"conditionBlock",
"labelFieldDisplayname",
"labelFieldName"
})
public LabelSourceSummary(
String sourceDisplayName,
String sourceName,
Long sourceId,
String labelOperatorName,
String labelCondition,
String conditionString,
ConditionBlock conditionBlock,
String labelFieldDisplayname,
String labelFieldName) {
super();
this.sourceDisplayName = sourceDisplayName;
this.sourceName = sourceName;
this.sourceId = sourceId;
this.labelOperatorName = labelOperatorName;
this.labelCondition = labelCondition;
this.conditionString = conditionString;
this.conditionBlock = conditionBlock;
this.labelFieldDisplayname = labelFieldDisplayname;
this.labelFieldName = labelFieldName;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
public static class Builder {
/** The source display name. */
@com.fasterxml.jackson.annotation.JsonProperty("sourceDisplayName")
private String sourceDisplayName;
/**
* The source display name.
*
* @param sourceDisplayName the value to set
* @return this builder
*/
public Builder sourceDisplayName(String sourceDisplayName) {
this.sourceDisplayName = sourceDisplayName;
this.__explicitlySet__.add("sourceDisplayName");
return this;
}
/** The source internal name. */
@com.fasterxml.jackson.annotation.JsonProperty("sourceName")
private String sourceName;
/**
* The source internal name.
*
* @param sourceName the value to set
* @return this builder
*/
public Builder sourceName(String sourceName) {
this.sourceName = sourceName;
this.__explicitlySet__.add("sourceName");
return this;
}
/** The source unique identifier. */
@com.fasterxml.jackson.annotation.JsonProperty("sourceId")
private Long sourceId;
/**
* The source unique identifier.
*
* @param sourceId the value to set
* @return this builder
*/
public Builder sourceId(Long sourceId) {
this.sourceId = sourceId;
this.__explicitlySet__.add("sourceId");
return this;
}
/** The label operator. */
@com.fasterxml.jackson.annotation.JsonProperty("labelOperatorName")
private String labelOperatorName;
/**
* The label operator.
*
* @param labelOperatorName the value to set
* @return this builder
*/
public Builder labelOperatorName(String labelOperatorName) {
this.labelOperatorName = labelOperatorName;
this.__explicitlySet__.add("labelOperatorName");
return this;
}
/** The label condition. */
@com.fasterxml.jackson.annotation.JsonProperty("labelCondition")
private String labelCondition;
/**
* The label condition.
*
* @param labelCondition the value to set
* @return this builder
*/
public Builder labelCondition(String labelCondition) {
this.labelCondition = labelCondition;
this.__explicitlySet__.add("labelCondition");
return this;
}
/** String representation of the label condition. */
@com.fasterxml.jackson.annotation.JsonProperty("conditionString")
private String conditionString;
/**
* String representation of the label condition.
*
* @param conditionString the value to set
* @return this builder
*/
public Builder conditionString(String conditionString) {
this.conditionString = conditionString;
this.__explicitlySet__.add("conditionString");
return this;
}
@com.fasterxml.jackson.annotation.JsonProperty("conditionBlock")
private ConditionBlock conditionBlock;
public Builder conditionBlock(ConditionBlock conditionBlock) {
this.conditionBlock = conditionBlock;
this.__explicitlySet__.add("conditionBlock");
return this;
}
/** The label field display name. */
@com.fasterxml.jackson.annotation.JsonProperty("labelFieldDisplayname")
private String labelFieldDisplayname;
/**
* The label field display name.
*
* @param labelFieldDisplayname the value to set
* @return this builder
*/
public Builder labelFieldDisplayname(String labelFieldDisplayname) {
this.labelFieldDisplayname = labelFieldDisplayname;
this.__explicitlySet__.add("labelFieldDisplayname");
return this;
}
/** The label field name. */
@com.fasterxml.jackson.annotation.JsonProperty("labelFieldName")
private String labelFieldName;
/**
* The label field name.
*
* @param labelFieldName the value to set
* @return this builder
*/
public Builder labelFieldName(String labelFieldName) {
this.labelFieldName = labelFieldName;
this.__explicitlySet__.add("labelFieldName");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public LabelSourceSummary build() {
LabelSourceSummary model =
new LabelSourceSummary(
this.sourceDisplayName,
this.sourceName,
this.sourceId,
this.labelOperatorName,
this.labelCondition,
this.conditionString,
this.conditionBlock,
this.labelFieldDisplayname,
this.labelFieldName);
for (String explicitlySetProperty : this.__explicitlySet__) {
model.markPropertyAsExplicitlySet(explicitlySetProperty);
}
return model;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(LabelSourceSummary model) {
if (model.wasPropertyExplicitlySet("sourceDisplayName")) {
this.sourceDisplayName(model.getSourceDisplayName());
}
if (model.wasPropertyExplicitlySet("sourceName")) {
this.sourceName(model.getSourceName());
}
if (model.wasPropertyExplicitlySet("sourceId")) {
this.sourceId(model.getSourceId());
}
if (model.wasPropertyExplicitlySet("labelOperatorName")) {
this.labelOperatorName(model.getLabelOperatorName());
}
if (model.wasPropertyExplicitlySet("labelCondition")) {
this.labelCondition(model.getLabelCondition());
}
if (model.wasPropertyExplicitlySet("conditionString")) {
this.conditionString(model.getConditionString());
}
if (model.wasPropertyExplicitlySet("conditionBlock")) {
this.conditionBlock(model.getConditionBlock());
}
if (model.wasPropertyExplicitlySet("labelFieldDisplayname")) {
this.labelFieldDisplayname(model.getLabelFieldDisplayname());
}
if (model.wasPropertyExplicitlySet("labelFieldName")) {
this.labelFieldName(model.getLabelFieldName());
}
return this;
}
}
/** Create a new builder. */
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return new Builder().copy(this);
}
/** The source display name. */
@com.fasterxml.jackson.annotation.JsonProperty("sourceDisplayName")
private final String sourceDisplayName;
/**
* The source display name.
*
* @return the value
*/
public String getSourceDisplayName() {
return sourceDisplayName;
}
/** The source internal name. */
@com.fasterxml.jackson.annotation.JsonProperty("sourceName")
private final String sourceName;
/**
* The source internal name.
*
* @return the value
*/
public String getSourceName() {
return sourceName;
}
/** The source unique identifier. */
@com.fasterxml.jackson.annotation.JsonProperty("sourceId")
private final Long sourceId;
/**
* The source unique identifier.
*
* @return the value
*/
public Long getSourceId() {
return sourceId;
}
/** The label operator. */
@com.fasterxml.jackson.annotation.JsonProperty("labelOperatorName")
private final String labelOperatorName;
/**
* The label operator.
*
* @return the value
*/
public String getLabelOperatorName() {
return labelOperatorName;
}
/** The label condition. */
@com.fasterxml.jackson.annotation.JsonProperty("labelCondition")
private final String labelCondition;
/**
* The label condition.
*
* @return the value
*/
public String getLabelCondition() {
return labelCondition;
}
/** String representation of the label condition. */
@com.fasterxml.jackson.annotation.JsonProperty("conditionString")
private final String conditionString;
/**
* String representation of the label condition.
*
* @return the value
*/
public String getConditionString() {
return conditionString;
}
@com.fasterxml.jackson.annotation.JsonProperty("conditionBlock")
private final ConditionBlock conditionBlock;
public ConditionBlock getConditionBlock() {
return conditionBlock;
}
/** The label field display name. */
@com.fasterxml.jackson.annotation.JsonProperty("labelFieldDisplayname")
private final String labelFieldDisplayname;
/**
* The label field display name.
*
* @return the value
*/
public String getLabelFieldDisplayname() {
return labelFieldDisplayname;
}
/** The label field name. */
@com.fasterxml.jackson.annotation.JsonProperty("labelFieldName")
private final String labelFieldName;
/**
* The label field name.
*
* @return the value
*/
public String getLabelFieldName() {
return labelFieldName;
}
@Override
public String toString() {
return this.toString(true);
}
/**
* Return a string representation of the object.
*
* @param includeByteArrayContents true to include the full contents of byte arrays
* @return string representation
*/
public String toString(boolean includeByteArrayContents) {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("LabelSourceSummary(");
sb.append("super=").append(super.toString());
sb.append("sourceDisplayName=").append(String.valueOf(this.sourceDisplayName));
sb.append(", sourceName=").append(String.valueOf(this.sourceName));
sb.append(", sourceId=").append(String.valueOf(this.sourceId));
sb.append(", labelOperatorName=").append(String.valueOf(this.labelOperatorName));
sb.append(", labelCondition=").append(String.valueOf(this.labelCondition));
sb.append(", conditionString=").append(String.valueOf(this.conditionString));
sb.append(", conditionBlock=").append(String.valueOf(this.conditionBlock));
sb.append(", labelFieldDisplayname=").append(String.valueOf(this.labelFieldDisplayname));
sb.append(", labelFieldName=").append(String.valueOf(this.labelFieldName));
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof LabelSourceSummary)) {
return false;
}
LabelSourceSummary other = (LabelSourceSummary) o;
return java.util.Objects.equals(this.sourceDisplayName, other.sourceDisplayName)
&& java.util.Objects.equals(this.sourceName, other.sourceName)
&& java.util.Objects.equals(this.sourceId, other.sourceId)
&& java.util.Objects.equals(this.labelOperatorName, other.labelOperatorName)
&& java.util.Objects.equals(this.labelCondition, other.labelCondition)
&& java.util.Objects.equals(this.conditionString, other.conditionString)
&& java.util.Objects.equals(this.conditionBlock, other.conditionBlock)
&& java.util.Objects.equals(this.labelFieldDisplayname, other.labelFieldDisplayname)
&& java.util.Objects.equals(this.labelFieldName, other.labelFieldName)
&& super.equals(other);
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result =
(result * PRIME)
+ (this.sourceDisplayName == null ? 43 : this.sourceDisplayName.hashCode());
result = (result * PRIME) + (this.sourceName == null ? 43 : this.sourceName.hashCode());
result = (result * PRIME) + (this.sourceId == null ? 43 : this.sourceId.hashCode());
result =
(result * PRIME)
+ (this.labelOperatorName == null ? 43 : this.labelOperatorName.hashCode());
result =
(result * PRIME)
+ (this.labelCondition == null ? 43 : this.labelCondition.hashCode());
result =
(result * PRIME)
+ (this.conditionString == null ? 43 : this.conditionString.hashCode());
result =
(result * PRIME)
+ (this.conditionBlock == null ? 43 : this.conditionBlock.hashCode());
result =
(result * PRIME)
+ (this.labelFieldDisplayname == null
? 43
: this.labelFieldDisplayname.hashCode());
result =
(result * PRIME)
+ (this.labelFieldName == null ? 43 : this.labelFieldName.hashCode());
result = (result * PRIME) + super.hashCode();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy