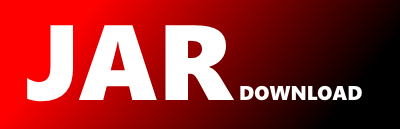
com.oracle.bmc.osmanagement.model.ModuleStreamProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oci-java-sdk-shaded-full Show documentation
Show all versions of oci-java-sdk-shaded-full Show documentation
This project contains the SDK distribution used for Oracle Cloud Infrastructure, and all the dependencies that can be shaded. It also has Maven dependencies that cannot be shaded. Therefore, use this module to depend on the shaded distribution via Maven -- it will shade everything that can be shaded, and automatically pull in the other dependencies.
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.osmanagement.model;
/**
* A module stream profile provided by a software source
* Note: Objects should always be created or deserialized using the {@link Builder}. This model
* distinguishes fields that are {@code null} because they are unset from fields that are explicitly
* set to {@code null}. This is done in the setter methods of the {@link Builder}, which maintain a
* set of all explicitly set fields called {@link Builder#__explicitlySet__}. The {@link
* #hashCode()} and {@link #equals(Object)} methods are implemented to take the explicitly set
* fields into account. The constructor, on the other hand, does not take the explicitly set fields
* into account (since the constructor cannot distinguish explicit {@code null} from unset {@code
* null}).
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20190801")
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(
builder = ModuleStreamProfile.Builder.class)
@com.fasterxml.jackson.annotation.JsonFilter(
com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel.EXPLICITLY_SET_FILTER_NAME)
public final class ModuleStreamProfile
extends com.oracle.bmc.http.client.internal.ExplicitlySetBmcModel {
@Deprecated
@java.beans.ConstructorProperties({
"moduleName",
"streamName",
"profileName",
"isDefault",
"description",
"packages"
})
public ModuleStreamProfile(
String moduleName,
String streamName,
String profileName,
Boolean isDefault,
String description,
java.util.List packages) {
super();
this.moduleName = moduleName;
this.streamName = streamName;
this.profileName = profileName;
this.isDefault = isDefault;
this.description = description;
this.packages = packages;
}
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "")
public static class Builder {
/** The name of the module that contains the stream profile */
@com.fasterxml.jackson.annotation.JsonProperty("moduleName")
private String moduleName;
/**
* The name of the module that contains the stream profile
*
* @param moduleName the value to set
* @return this builder
*/
public Builder moduleName(String moduleName) {
this.moduleName = moduleName;
this.__explicitlySet__.add("moduleName");
return this;
}
/** The name of the stream that contains the profile */
@com.fasterxml.jackson.annotation.JsonProperty("streamName")
private String streamName;
/**
* The name of the stream that contains the profile
*
* @param streamName the value to set
* @return this builder
*/
public Builder streamName(String streamName) {
this.streamName = streamName;
this.__explicitlySet__.add("streamName");
return this;
}
/** The name of the profile */
@com.fasterxml.jackson.annotation.JsonProperty("profileName")
private String profileName;
/**
* The name of the profile
*
* @param profileName the value to set
* @return this builder
*/
public Builder profileName(String profileName) {
this.profileName = profileName;
this.__explicitlySet__.add("profileName");
return this;
}
/** Indicates if this profile is the default for its module stream. */
@com.fasterxml.jackson.annotation.JsonProperty("isDefault")
private Boolean isDefault;
/**
* Indicates if this profile is the default for its module stream.
*
* @param isDefault the value to set
* @return this builder
*/
public Builder isDefault(Boolean isDefault) {
this.isDefault = isDefault;
this.__explicitlySet__.add("isDefault");
return this;
}
/** A description of the contents of the module stream profile */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private String description;
/**
* A description of the contents of the module stream profile
*
* @param description the value to set
* @return this builder
*/
public Builder description(String description) {
this.description = description;
this.__explicitlySet__.add("description");
return this;
}
/**
* A list of packages that constitute the profile. Each element in the list is the name of a
* package. The name is suitable to use as an argument to other OS Management APIs that
* interact directly with packages.
*/
@com.fasterxml.jackson.annotation.JsonProperty("packages")
private java.util.List packages;
/**
* A list of packages that constitute the profile. Each element in the list is the name of a
* package. The name is suitable to use as an argument to other OS Management APIs that
* interact directly with packages.
*
* @param packages the value to set
* @return this builder
*/
public Builder packages(java.util.List packages) {
this.packages = packages;
this.__explicitlySet__.add("packages");
return this;
}
@com.fasterxml.jackson.annotation.JsonIgnore
private final java.util.Set __explicitlySet__ = new java.util.HashSet();
public ModuleStreamProfile build() {
ModuleStreamProfile model =
new ModuleStreamProfile(
this.moduleName,
this.streamName,
this.profileName,
this.isDefault,
this.description,
this.packages);
for (String explicitlySetProperty : this.__explicitlySet__) {
model.markPropertyAsExplicitlySet(explicitlySetProperty);
}
return model;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public Builder copy(ModuleStreamProfile model) {
if (model.wasPropertyExplicitlySet("moduleName")) {
this.moduleName(model.getModuleName());
}
if (model.wasPropertyExplicitlySet("streamName")) {
this.streamName(model.getStreamName());
}
if (model.wasPropertyExplicitlySet("profileName")) {
this.profileName(model.getProfileName());
}
if (model.wasPropertyExplicitlySet("isDefault")) {
this.isDefault(model.getIsDefault());
}
if (model.wasPropertyExplicitlySet("description")) {
this.description(model.getDescription());
}
if (model.wasPropertyExplicitlySet("packages")) {
this.packages(model.getPackages());
}
return this;
}
}
/** Create a new builder. */
public static Builder builder() {
return new Builder();
}
public Builder toBuilder() {
return new Builder().copy(this);
}
/** The name of the module that contains the stream profile */
@com.fasterxml.jackson.annotation.JsonProperty("moduleName")
private final String moduleName;
/**
* The name of the module that contains the stream profile
*
* @return the value
*/
public String getModuleName() {
return moduleName;
}
/** The name of the stream that contains the profile */
@com.fasterxml.jackson.annotation.JsonProperty("streamName")
private final String streamName;
/**
* The name of the stream that contains the profile
*
* @return the value
*/
public String getStreamName() {
return streamName;
}
/** The name of the profile */
@com.fasterxml.jackson.annotation.JsonProperty("profileName")
private final String profileName;
/**
* The name of the profile
*
* @return the value
*/
public String getProfileName() {
return profileName;
}
/** Indicates if this profile is the default for its module stream. */
@com.fasterxml.jackson.annotation.JsonProperty("isDefault")
private final Boolean isDefault;
/**
* Indicates if this profile is the default for its module stream.
*
* @return the value
*/
public Boolean getIsDefault() {
return isDefault;
}
/** A description of the contents of the module stream profile */
@com.fasterxml.jackson.annotation.JsonProperty("description")
private final String description;
/**
* A description of the contents of the module stream profile
*
* @return the value
*/
public String getDescription() {
return description;
}
/**
* A list of packages that constitute the profile. Each element in the list is the name of a
* package. The name is suitable to use as an argument to other OS Management APIs that interact
* directly with packages.
*/
@com.fasterxml.jackson.annotation.JsonProperty("packages")
private final java.util.List packages;
/**
* A list of packages that constitute the profile. Each element in the list is the name of a
* package. The name is suitable to use as an argument to other OS Management APIs that interact
* directly with packages.
*
* @return the value
*/
public java.util.List getPackages() {
return packages;
}
@Override
public String toString() {
return this.toString(true);
}
/**
* Return a string representation of the object.
*
* @param includeByteArrayContents true to include the full contents of byte arrays
* @return string representation
*/
public String toString(boolean includeByteArrayContents) {
java.lang.StringBuilder sb = new java.lang.StringBuilder();
sb.append("ModuleStreamProfile(");
sb.append("super=").append(super.toString());
sb.append("moduleName=").append(String.valueOf(this.moduleName));
sb.append(", streamName=").append(String.valueOf(this.streamName));
sb.append(", profileName=").append(String.valueOf(this.profileName));
sb.append(", isDefault=").append(String.valueOf(this.isDefault));
sb.append(", description=").append(String.valueOf(this.description));
sb.append(", packages=").append(String.valueOf(this.packages));
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ModuleStreamProfile)) {
return false;
}
ModuleStreamProfile other = (ModuleStreamProfile) o;
return java.util.Objects.equals(this.moduleName, other.moduleName)
&& java.util.Objects.equals(this.streamName, other.streamName)
&& java.util.Objects.equals(this.profileName, other.profileName)
&& java.util.Objects.equals(this.isDefault, other.isDefault)
&& java.util.Objects.equals(this.description, other.description)
&& java.util.Objects.equals(this.packages, other.packages)
&& super.equals(other);
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = (result * PRIME) + (this.moduleName == null ? 43 : this.moduleName.hashCode());
result = (result * PRIME) + (this.streamName == null ? 43 : this.streamName.hashCode());
result = (result * PRIME) + (this.profileName == null ? 43 : this.profileName.hashCode());
result = (result * PRIME) + (this.isDefault == null ? 43 : this.isDefault.hashCode());
result = (result * PRIME) + (this.description == null ? 43 : this.description.hashCode());
result = (result * PRIME) + (this.packages == null ? 43 : this.packages.hashCode());
result = (result * PRIME) + super.hashCode();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy