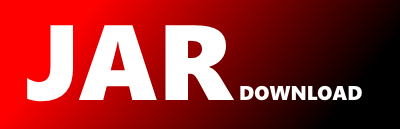
com.oracle.bmc.servicecatalog.ServiceCatalog Maven / Gradle / Ivy
Show all versions of oci-java-sdk-shaded-full Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.servicecatalog;
import com.oracle.bmc.servicecatalog.requests.*;
import com.oracle.bmc.servicecatalog.responses.*;
/**
* Manage solutions in Oracle Cloud Infrastructure Service Catalog. This service client uses
* CircuitBreakerUtils.DEFAULT_CIRCUIT_BREAKER for all the operations by default if no circuit
* breaker configuration is defined by the user.
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20210527")
public interface ServiceCatalog extends AutoCloseable {
/** Rebuilds the client from scratch. Useful to refresh certificates. */
void refreshClient();
/**
* Sets the endpoint to call (ex, https://www.example.com).
*
* @param endpoint The endpoint of the service.
*/
void setEndpoint(String endpoint);
/** Gets the set endpoint for REST call (ex, https://www.example.com) */
String getEndpoint();
/**
* Sets the region to call (ex, Region.US_PHOENIX_1).
*
* Note, this will call {@link #setEndpoint(String) setEndpoint} after resolving the
* endpoint. If the service is not available in this Region, however, an
* IllegalArgumentException will be raised.
*
* @param region The region of the service.
*/
void setRegion(com.oracle.bmc.Region region);
/**
* Sets the region to call (ex, 'us-phoenix-1').
*
*
Note, this will first try to map the region ID to a known Region and call {@link
* #setRegion(Region) setRegion}.
*
*
If no known Region could be determined, it will create an endpoint based on the default
* endpoint format ({@link com.oracle.bmc.Region#formatDefaultRegionEndpoint(Service, String)}
* and then call {@link #setEndpoint(String) setEndpoint}.
*
* @param regionId The public region ID.
*/
void setRegion(String regionId);
/**
* Determines whether realm specific endpoint should be used or not. Set
* realmSpecificEndpointTemplateEnabled to "true" if the user wants to enable use of realm
* specific endpoint template, otherwise set it to "false"
*
* @param realmSpecificEndpointTemplateEnabled flag to enable the use of realm specific endpoint
* template
*/
void useRealmSpecificEndpointTemplate(boolean realmSpecificEndpointTemplateEnabled);
/**
* Replace all associations of a given service catalog in one bulk transaction.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* BulkReplaceServiceCatalogAssociations API.
*/
BulkReplaceServiceCatalogAssociationsResponse bulkReplaceServiceCatalogAssociations(
BulkReplaceServiceCatalogAssociationsRequest request);
/**
* Moves the specified private application from one compartment to another.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ChangePrivateApplicationCompartment API.
*/
ChangePrivateApplicationCompartmentResponse changePrivateApplicationCompartment(
ChangePrivateApplicationCompartmentRequest request);
/**
* Moves the specified service catalog from one compartment to another.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ChangeServiceCatalogCompartment API.
*/
ChangeServiceCatalogCompartmentResponse changeServiceCatalogCompartment(
ChangeServiceCatalogCompartmentRequest request);
/**
* Creates a private application along with a single package to be hosted.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* CreatePrivateApplication API.
*/
CreatePrivateApplicationResponse createPrivateApplication(
CreatePrivateApplicationRequest request);
/**
* Creates a brand new service catalog in a given compartment.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* CreateServiceCatalog API.
*/
CreateServiceCatalogResponse createServiceCatalog(CreateServiceCatalogRequest request);
/**
* Creates an association between service catalog and a resource.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* CreateServiceCatalogAssociation API.
*/
CreateServiceCatalogAssociationResponse createServiceCatalogAssociation(
CreateServiceCatalogAssociationRequest request);
/**
* Deletes an existing private application.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* DeletePrivateApplication API.
*/
DeletePrivateApplicationResponse deletePrivateApplication(
DeletePrivateApplicationRequest request);
/**
* Deletes the specified service catalog from the compartment.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* DeleteServiceCatalog API.
*/
DeleteServiceCatalogResponse deleteServiceCatalog(DeleteServiceCatalogRequest request);
/**
* Removes an association between service catalog and a resource.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* DeleteServiceCatalogAssociation API.
*/
DeleteServiceCatalogAssociationResponse deleteServiceCatalogAssociation(
DeleteServiceCatalogAssociationRequest request);
/**
* Gets the details of the specified private application.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetPrivateApplication API.
*/
GetPrivateApplicationResponse getPrivateApplication(GetPrivateApplicationRequest request);
/**
* Downloads the binary payload of the logo image of the private application.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetPrivateApplicationActionDownloadLogo API.
*/
GetPrivateApplicationActionDownloadLogoResponse getPrivateApplicationActionDownloadLogo(
GetPrivateApplicationActionDownloadLogoRequest request);
/**
* Gets the details of a specific package within a given private application.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetPrivateApplicationPackage API.
*/
GetPrivateApplicationPackageResponse getPrivateApplicationPackage(
GetPrivateApplicationPackageRequest request);
/**
* Downloads the configuration that was used to create the private application package.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetPrivateApplicationPackageActionDownloadConfig API.
*/
GetPrivateApplicationPackageActionDownloadConfigResponse
getPrivateApplicationPackageActionDownloadConfig(
GetPrivateApplicationPackageActionDownloadConfigRequest request);
/**
* Gets detailed information about the service catalog including name, compartmentId
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use GetServiceCatalog
* API.
*/
GetServiceCatalogResponse getServiceCatalog(GetServiceCatalogRequest request);
/**
* Gets detailed information about specific service catalog association.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetServiceCatalogAssociation API.
*/
GetServiceCatalogAssociationResponse getServiceCatalogAssociation(
GetServiceCatalogAssociationRequest request);
/**
* Gets the status of the work request with the given ID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use GetWorkRequest API.
*/
GetWorkRequestResponse getWorkRequest(GetWorkRequestRequest request);
/**
* Lists all the applications in a service catalog or a tenancy. If no parameter is specified,
* all catalogs from all compartments in the tenancy will be scanned for any type of content.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListApplications
* API.
*/
ListApplicationsResponse listApplications(ListApplicationsRequest request);
/**
* Lists the packages in the specified private application.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ListPrivateApplicationPackages API.
*/
ListPrivateApplicationPackagesResponse listPrivateApplicationPackages(
ListPrivateApplicationPackagesRequest request);
/**
* Lists all the private applications in a given compartment.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ListPrivateApplications API.
*/
ListPrivateApplicationsResponse listPrivateApplications(ListPrivateApplicationsRequest request);
/**
* Lists all the resource associations for a specific service catalog.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ListServiceCatalogAssociations API.
*/
ListServiceCatalogAssociationsResponse listServiceCatalogAssociations(
ListServiceCatalogAssociationsRequest request);
/**
* Lists all the service catalogs in the given compartment.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListServiceCatalogs
* API.
*/
ListServiceCatalogsResponse listServiceCatalogs(ListServiceCatalogsRequest request);
/**
* Return a (paginated) list of errors for a given work request.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ListWorkRequestErrors API.
*/
ListWorkRequestErrorsResponse listWorkRequestErrors(ListWorkRequestErrorsRequest request);
/**
* Return a (paginated) list of logs for a given work request.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListWorkRequestLogs
* API.
*/
ListWorkRequestLogsResponse listWorkRequestLogs(ListWorkRequestLogsRequest request);
/**
* Lists the work requests in a compartment.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListWorkRequests
* API.
*/
ListWorkRequestsResponse listWorkRequests(ListWorkRequestsRequest request);
/**
* Updates the details of an existing private application.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* UpdatePrivateApplication API.
*/
UpdatePrivateApplicationResponse updatePrivateApplication(
UpdatePrivateApplicationRequest request);
/**
* Updates the details of a previously created service catalog.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* UpdateServiceCatalog API.
*/
UpdateServiceCatalogResponse updateServiceCatalog(UpdateServiceCatalogRequest request);
/**
* Gets the pre-configured waiters available for resources for this service.
*
* @return The service waiters.
*/
ServiceCatalogWaiters getWaiters();
/**
* Gets the pre-configured paginators available for list operations in this service which may
* return multiple pages of data. These paginators provide an {@link java.lang.Iterable}
* interface so that service responses, or resources/records, can be iterated through without
* having to manually deal with pagination and page tokens.
*
* @return The service paginators.
*/
ServiceCatalogPaginators getPaginators();
}