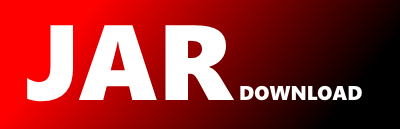
com.oracle.bmc.usageapi.Usageapi Maven / Gradle / Ivy
Show all versions of oci-java-sdk-shaded-full Show documentation
/**
* Copyright (c) 2016, 2024, Oracle and/or its affiliates. All rights reserved.
* This software is dual-licensed to you under the Universal Permissive License (UPL) 1.0 as shown at https://oss.oracle.com/licenses/upl or Apache License 2.0 as shown at http://www.apache.org/licenses/LICENSE-2.0. You may choose either license.
*/
package com.oracle.bmc.usageapi;
import com.oracle.bmc.usageapi.requests.*;
import com.oracle.bmc.usageapi.responses.*;
/**
* Use the Usage API to view your Oracle Cloud usage and costs. The API allows you to request data
* that meets the specified filter criteria, and to group that data by the chosen dimension. The
* Usage API is used by the Cost Analysis and Carbon Emissions Analysis tools in the Console. See
* [Cost Analysis
* Overview](https://docs.cloud.oracle.com/Content/Billing/Concepts/costanalysisoverview.htm) and
* [Using the Usage
* API](https://docs.cloud.oracle.com/Content/Billing/Concepts/costanalysisoverview.htm#cost_analysis_using_the_api)
* for more information. This service client uses CircuitBreakerUtils.DEFAULT_CIRCUIT_BREAKER for
* all the operations by default if no circuit breaker configuration is defined by the user.
*/
@jakarta.annotation.Generated(value = "OracleSDKGenerator", comments = "API Version: 20200107")
public interface Usageapi extends AutoCloseable {
/** Rebuilds the client from scratch. Useful to refresh certificates. */
void refreshClient();
/**
* Sets the endpoint to call (ex, https://www.example.com).
*
* @param endpoint The endpoint of the service.
*/
void setEndpoint(String endpoint);
/** Gets the set endpoint for REST call (ex, https://www.example.com) */
String getEndpoint();
/**
* Sets the region to call (ex, Region.US_PHOENIX_1).
*
* Note, this will call {@link #setEndpoint(String) setEndpoint} after resolving the
* endpoint. If the service is not available in this Region, however, an
* IllegalArgumentException will be raised.
*
* @param region The region of the service.
*/
void setRegion(com.oracle.bmc.Region region);
/**
* Sets the region to call (ex, 'us-phoenix-1').
*
*
Note, this will first try to map the region ID to a known Region and call {@link
* #setRegion(Region) setRegion}.
*
*
If no known Region could be determined, it will create an endpoint based on the default
* endpoint format ({@link com.oracle.bmc.Region#formatDefaultRegionEndpoint(Service, String)}
* and then call {@link #setEndpoint(String) setEndpoint}.
*
* @param regionId The public region ID.
*/
void setRegion(String regionId);
/**
* Determines whether realm specific endpoint should be used or not. Set
* realmSpecificEndpointTemplateEnabled to "true" if the user wants to enable use of realm
* specific endpoint template, otherwise set it to "false"
*
* @param realmSpecificEndpointTemplateEnabled flag to enable the use of realm specific endpoint
* template
*/
void useRealmSpecificEndpointTemplate(boolean realmSpecificEndpointTemplateEnabled);
/**
* Returns the created custom table.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use CreateCustomTable
* API.
*/
CreateCustomTableResponse createCustomTable(CreateCustomTableRequest request);
/**
* Add a list of email recipients that can receive usage statements for the subscription.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* CreateEmailRecipientsGroup API.
*/
CreateEmailRecipientsGroupResponse createEmailRecipientsGroup(
CreateEmailRecipientsGroupRequest request);
/**
* Returns the created query.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use CreateQuery API.
*/
CreateQueryResponse createQuery(CreateQueryRequest request);
/**
* Returns the created schedule.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use CreateSchedule API.
*/
CreateScheduleResponse createSchedule(CreateScheduleRequest request);
/**
* Returns the created usage carbon emissions query.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* CreateUsageCarbonEmissionsQuery API.
*/
CreateUsageCarbonEmissionsQueryResponse createUsageCarbonEmissionsQuery(
CreateUsageCarbonEmissionsQueryRequest request);
/**
* Delete a saved custom table by the OCID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use DeleteCustomTable
* API.
*/
DeleteCustomTableResponse deleteCustomTable(DeleteCustomTableRequest request);
/**
* Delete the email recipients group for the usage statement subscription.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* DeleteEmailRecipientsGroup API.
*/
DeleteEmailRecipientsGroupResponse deleteEmailRecipientsGroup(
DeleteEmailRecipientsGroupRequest request);
/**
* Delete a saved query by the OCID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use DeleteQuery API.
*/
DeleteQueryResponse deleteQuery(DeleteQueryRequest request);
/**
* Delete a saved scheduled report by the OCID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use DeleteSchedule API.
*/
DeleteScheduleResponse deleteSchedule(DeleteScheduleRequest request);
/**
* Delete a usage carbon emissions saved query by the OCID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* DeleteUsageCarbonEmissionsQuery API.
*/
DeleteUsageCarbonEmissionsQueryResponse deleteUsageCarbonEmissionsQuery(
DeleteUsageCarbonEmissionsQueryRequest request);
/**
* Returns the saved custom table.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use GetCustomTable API.
*/
GetCustomTableResponse getCustomTable(GetCustomTableRequest request);
/**
* Return the saved usage statement email recipient group.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetEmailRecipientsGroup API.
*/
GetEmailRecipientsGroupResponse getEmailRecipientsGroup(GetEmailRecipientsGroupRequest request);
/**
* Returns the saved query.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use GetQuery API.
*/
GetQueryResponse getQuery(GetQueryRequest request);
/**
* Returns the saved schedule.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use GetSchedule API.
*/
GetScheduleResponse getSchedule(GetScheduleRequest request);
/**
* Returns the saved schedule run.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use GetScheduledRun
* API.
*/
GetScheduledRunResponse getScheduledRun(GetScheduledRunRequest request);
/**
* Returns the usage carbon emissions saved query.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* GetUsageCarbonEmissionsQuery API.
*/
GetUsageCarbonEmissionsQueryResponse getUsageCarbonEmissionsQuery(
GetUsageCarbonEmissionsQueryRequest request);
/**
* Returns the saved custom table list.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListCustomTables
* API.
*/
ListCustomTablesResponse listCustomTables(ListCustomTablesRequest request);
/**
* Return the saved usage statement email recipient group.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ListEmailRecipientsGroups API.
*/
ListEmailRecipientsGroupsResponse listEmailRecipientsGroups(
ListEmailRecipientsGroupsRequest request);
/**
* Returns the saved query list.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListQueries API.
*/
ListQueriesResponse listQueries(ListQueriesRequest request);
/**
* Returns schedule history list.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListScheduledRuns
* API.
*/
ListScheduledRunsResponse listScheduledRuns(ListScheduledRunsRequest request);
/**
* Returns the saved schedule list.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use ListSchedules API.
*/
ListSchedulesResponse listSchedules(ListSchedulesRequest request);
/**
* Returns the usage carbon emissions saved query list.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* ListUsageCarbonEmissionsQueries API.
*/
ListUsageCarbonEmissionsQueriesResponse listUsageCarbonEmissionsQueries(
ListUsageCarbonEmissionsQueriesRequest request);
/**
* Returns the average carbon emissions summary by SKU.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* RequestAverageCarbonEmission API.
*/
RequestAverageCarbonEmissionResponse requestAverageCarbonEmission(
RequestAverageCarbonEmissionRequest request);
/**
* Returns the clean energy usage summary by region.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* RequestCleanEnergyUsage API.
*/
RequestCleanEnergyUsageResponse requestCleanEnergyUsage(RequestCleanEnergyUsageRequest request);
/**
* Returns the configurations list for the UI drop-down list.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* RequestSummarizedConfigurations API.
*/
RequestSummarizedConfigurationsResponse requestSummarizedConfigurations(
RequestSummarizedConfigurationsRequest request);
/**
* Returns usage for the given account.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* RequestSummarizedUsages API.
*/
RequestSummarizedUsagesResponse requestSummarizedUsages(RequestSummarizedUsagesRequest request);
/**
* Returns the configuration list for the UI drop-down list of carbon emission console.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* RequestUsageCarbonEmissionConfig API.
*/
RequestUsageCarbonEmissionConfigResponse requestUsageCarbonEmissionConfig(
RequestUsageCarbonEmissionConfigRequest request);
/**
* Returns usage carbon emission for the given account.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* RequestUsageCarbonEmissions API.
*/
RequestUsageCarbonEmissionsResponse requestUsageCarbonEmissions(
RequestUsageCarbonEmissionsRequest request);
/**
* Update a saved custom table by table id.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use UpdateCustomTable
* API.
*/
UpdateCustomTableResponse updateCustomTable(UpdateCustomTableRequest request);
/**
* Update a saved email recipients group.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* UpdateEmailRecipientsGroup API.
*/
UpdateEmailRecipientsGroupResponse updateEmailRecipientsGroup(
UpdateEmailRecipientsGroupRequest request);
/**
* Update a saved query by the OCID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use UpdateQuery API.
*/
UpdateQueryResponse updateQuery(UpdateQueryRequest request);
/**
* Update a saved schedule
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use UpdateSchedule API.
*/
UpdateScheduleResponse updateSchedule(UpdateScheduleRequest request);
/**
* Update a usage carbon emissions saved query by the OCID.
*
* @param request The request object containing the details to send
* @return A response object containing details about the completed operation
* @throws BmcException when an error occurs. This operation will not retry by default, users
* can also use RetryConfiguration.SDK_DEFAULT_RETRY_CONFIGURATION provided by the SDK to
* enable retries for it. The specifics of the default retry strategy are described here
* https://docs.oracle.com/en-us/iaas/Content/API/SDKDocs/javasdkconcepts.htm#javasdkconcepts_topic_Retries
*
Example: Click here to see how to use
* UpdateUsageCarbonEmissionsQuery API.
*/
UpdateUsageCarbonEmissionsQueryResponse updateUsageCarbonEmissionsQuery(
UpdateUsageCarbonEmissionsQueryRequest request);
/**
* Gets the pre-configured waiters available for resources for this service.
*
* @return The service waiters.
*/
UsageapiWaiters getWaiters();
/**
* Gets the pre-configured paginators available for list operations in this service which may
* return multiple pages of data. These paginators provide an {@link java.lang.Iterable}
* interface so that service responses, or resources/records, can be iterated through without
* having to manually deal with pagination and page tokens.
*
* @return The service paginators.
*/
UsageapiPaginators getPaginators();
}