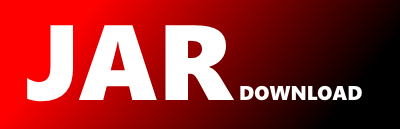
com.oracle.truffle.api.TruffleLanguage Maven / Gradle / Ivy
Show all versions of truffle-api Show documentation
/*
* Copyright (c) 2014, 2016, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package com.oracle.truffle.api;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.TruffleLanguage.Env;
import com.oracle.truffle.api.frame.FrameDescriptor;
import com.oracle.truffle.api.frame.FrameSlot;
import com.oracle.truffle.api.frame.FrameSlotTypeException;
import com.oracle.truffle.api.frame.MaterializedFrame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.impl.Accessor;
import com.oracle.truffle.api.impl.ReadOnlyArrayList;
import com.oracle.truffle.api.nodes.LanguageInfo;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.RootNode;
import com.oracle.truffle.api.source.Source;
import com.oracle.truffle.api.source.SourceSection;
/**
* A Truffle language implementation for executing guest language code in a
* {@linkplain com.oracle.truffle.api.vm.PolyglotEngine PolyglotEngine}. Subclasses of
* {@link TruffleLanguage} must provide a public default constructor.
*
* Lifecycle
*
* A language implementation becomes available for use by an engine when metadata is added using the
* {@link Registration} annotation and the implementation's JAR file placed on the host Java Virtual
* Machine's class path.
*
* A newly created engine locates all available language implementations and creates a
* {@linkplain com.oracle.truffle.api.vm.PolyglotEngine.Language descriptor} for each. The
* descriptor holds the language's registered metadata, but its execution environment is not
* initialized until the language is needed for code execution. That execution environment remains
* initialized for the lifetime of the engine and is isolated from the environment in any other
* engine instance.
*
* A new {@link TruffleLanguage language implementation} instance is instantiated for each runtime
* that is created using the {@linkplain com.oracle.truffle.api.vm.PolyglotRuntime.Builder#build()
* runtime builder}. If an engine is created without a runtime then the language implementation
* instance is created for each engine.
*
* Global state can be shared between multiple context instances by saving them as in a field of the
* {@link TruffleLanguage} subclass. The implementation needs to ensure data isolation between the
* contexts. However ASTs or assumptions can be shared across multiple contexts if modifying them
* does not affect language semantics. Languages are strongly discouraged from using static mutable
* state in their languages. Instead language implementation instances should be used instead.
*
* The methods in {@link TruffleLanguage} might be invoked from multiple threads. However, one
* context instance is guaranteed to be invoked only from one single thread. Therefore context
* objects don't need to be thread-safe. Code that is shared using the {@link TruffleLanguage}
* instance needs to be prepared to be accessed from multiple threads.
*
* Whenever an engine is disposed then each initialized context will be disposed
* {@link #disposeContext(Object) disposed}.
*
*
Cardinalities
*
* One host virtual machine depends on other system instances using the following
* cardinalities:
*
*
* K = number of installed languages
* I = number of installed instruments
* N = unbounded
*
* - 1:Host VM Processs
* - N:PolyglotRuntime
* - K:TruffleLanguage
* - I:PolyglotRuntime.Instrument
* - 1:TruffleInstrument
* - N:PolyglotEngine
* - 1:Thread
* - K:PolyglotEngine.Language
* - 1:Language Context
*
*
* Language Configuration
*
* Each engine instance can, during its creation, register
* {@linkplain com.oracle.truffle.api.vm.PolyglotEngine.Builder#config(String, String, Object)
* language-specific configuration data} (for example originating from command line arguments) in
* the form of {@code MIME-type/key/object} triples. A Language implementation retrieves the data
* via {@link Env#getConfig()} for configuring {@link #createContext(Env) initial execution state}.
*
* Global Symbols
*
* Language implementations communicate with one another (and with instrumentation-based tools such
* as debuggers) by exporting/importing named values known as global symbols. These
* typically implement guest language export/import statements used for language
* interoperation.
*
* A language manages its namespace of exported global symbols dynamically, by its response to the
* query {@link #findExportedSymbol(Object, String, boolean)}. No attempt is made to avoid
* cross-language name conflicts.
*
* A language implementation can also {@linkplain Env#importSymbol(String) import} a global symbol
* by name, according to the following rules:
*
* - A global symbol
* {@linkplain com.oracle.truffle.api.vm.PolyglotEngine.Builder#globalSymbol(String, Object)
* registered by the engine} will be returned, if any, ignoring global symbols exported by other
* languages.
* - Otherwise all languages are queried in unspecified order, and the first global symbol found,
* if any, is returned.
*
*
* Configuration vs. Initialization
*
* To ensure that a Truffle language can be used in a language-agnostic way, the implementation
* should be designed to decouple its configuration and initialization from language specifics as
* much as possible. One aspect of this is the initialization and start of execution via the
* {@link com.oracle.truffle.api.vm.PolyglotEngine}, which should be designed in a generic way.
* Language-specific entry points, for instance to emulate the command-line interface of an existing
* implementation, should be handled externally.
*
* @param internal state of the language associated with every thread that is executing program
* {@link #parse(com.oracle.truffle.api.TruffleLanguage.ParsingRequest) parsed} by the
* language
* @since 0.8 or earlier
*/
@SuppressWarnings({"javadoc"})
public abstract class TruffleLanguage {
// get and isFinal are frequent operations -> cache the engine access call
@CompilationFinal private LanguageInfo languageInfo;
@CompilationFinal private ContextReference reference;
@CompilationFinal private boolean singletonLanguage;
/**
* Constructor to be called by subclasses.
*
* @since 0.8 or earlier
*/
protected TruffleLanguage() {
}
/**
* The annotation to use to register your language to the
* {@link com.oracle.truffle.api.vm.PolyglotEngine Truffle} system. By annotating your
* implementation of {@link TruffleLanguage} by this annotation you are just a one JAR drop
* to the class path away from your users. Once they include your JAR in their application,
* your language will be available to the {@link com.oracle.truffle.api.vm.PolyglotEngine
* Truffle virtual machine}.
*
* @since 0.8 or earlier
*/
@Retention(RetentionPolicy.SOURCE)
@Target(ElementType.TYPE)
public @interface Registration {
/**
* Unique name of your language. This name will be exposed to users via the
* {@link com.oracle.truffle.api.vm.PolyglotEngine.Language#getName()} getter.
*
* @return identifier of your language
*/
String name();
/**
* Unique string identifying the language version. This name will be exposed to users via
* the {@link com.oracle.truffle.api.vm.PolyglotEngine.Language#getVersion()} getter.
*
* @return version of your language
*/
String version();
/**
* List of MIME types associated with your language. Users will use them (directly or
* indirectly) when
* {@link com.oracle.truffle.api.vm.PolyglotEngine#eval(com.oracle.truffle.api.source.Source)
* executing} their code snippets or their {@link Source files}.
*
* @return array of MIME types assigned to your language files
*/
String[] mimeType();
/**
* Specifies if the language is suitable for interactive evaluation of {@link Source
* sources}. {@link #interactive() Interactive} languages should be displayed in interactive
* environments and presented to the user. The default value of this attribute is
* true
assuming majority of the languages is interactive. Change the value to
* false
to opt-out and turn your language into non-interactive one.
*
* @return true
if the language should be presented to end-user in an
* interactive environment
* @since 0.22
*/
boolean interactive() default true;
}
/**
* Creates internal representation of the executing context suitable for given environment. Each
* time the {@link TruffleLanguage language} is used by a new
* {@link com.oracle.truffle.api.vm.PolyglotEngine} or in a new thread, the system calls this
* method to let the {@link TruffleLanguage language} prepare for execution. The
* returned execution context is completely language specific; it is however expected it will
* contain reference to here-in provided env
and adjust itself according to
* parameters provided by the env
object.
*
* The context created by this method is accessible using {@link #getContextReference()}. An
* {@link IllegalStateException} is thrown if the context is tried to be accessed while the
* createContext method is executed.
*
* This method shouldn't perform any complex operations. The runtime system is just being
* initialized and for example making
* {@link Env#parse(com.oracle.truffle.api.source.Source, java.lang.String...) calls into other
* languages} and assuming your language is already initialized and others can see it would be
* wrong - until you return from this method, the initialization isn't over. Should there be a
* need to perform complex initializaton, do it by overriding the
* {@link #initializeContext(java.lang.Object)} method.
*
* @param env the environment the language is supposed to operate in
* @return internal data of the language in given environment
* @since 0.8 or earlier
*/
protected abstract C createContext(Env env);
/**
* Perform any complex initialization. The
* {@link #createContext(com.oracle.truffle.api.TruffleLanguage.Env) } factory method shouldn't
* do any complex operations. Just create the instance of the context, let the runtime system
* register it properly. Should there be a need to perform complex initializaton, override this
* method and let the runtime call it later to finish any post initialization
* actions. Example:
*
* {@link TruffleLanguageSnippets.PostInitLanguage#createContext}
*
* @param context the context created by
* {@link #createContext(com.oracle.truffle.api.TruffleLanguage.Env)}
* @throws java.lang.Exception if something goes wrong
* @since 0.17
*/
protected void initializeContext(C context) throws Exception {
}
/**
* Disposes the context created by
* {@link #createContext(com.oracle.truffle.api.TruffleLanguage.Env)}. A language can be asked
* by its user to clean-up. In such case the language is supposed to dispose any
* resources acquired before and dispose the context
- e.g. render it
* useless for any future calls.
*
* @param context the context {@link #createContext(com.oracle.truffle.api.TruffleLanguage.Env)
* created by the language}
* @since 0.8 or earlier
*/
protected void disposeContext(C context) {
}
/**
* Parses the provided source and generates appropriate AST. The parsing should execute no user
* code, it should only create the {@link Node} tree to represent the source. If the provided
* source does not correspond naturally to a call target, the returned call target should create
* and if necessary initialize the corresponding language entity and return it. The parsing may
* be performed in a context (specified as another {@link Node}) or without context. The
* {@code argumentNames} may contain symbolic names for actual parameters of the call to the
* returned value. The result should be a call target with method
* {@link CallTarget#call(java.lang.Object...)} that accepts as many arguments as were provided
* via the {@code argumentNames} array.
*
* @param code source code to parse
* @param context a {@link Node} defining context for the parsing
* @param argumentNames symbolic names for parameters of
* {@link CallTarget#call(java.lang.Object...)}
* @return a call target to invoke which also keeps in memory the {@link Node} tree representing
* just parsed code
* @throws Exception if parsing goes wrong. Here-in thrown exception is propagated to the user
* who called one of eval
methods of
* {@link com.oracle.truffle.api.vm.PolyglotEngine}
* @since 0.8 or earlier
* @deprecated override {@link #parse(com.oracle.truffle.api.TruffleLanguage.ParsingRequest)}
*/
@Deprecated
protected CallTarget parse(Source code, Node context, String... argumentNames) throws Exception {
throw new UnsupportedOperationException("Call parse with ParsingRequest parameter!");
}
/**
* Parses the {@link ParsingRequest#getSource() provided source} and generates its appropriate
* AST representation. The parsing should execute no user code, it should only create the
* {@link Node} tree to represent the source. If the {@link ParsingRequest#getSource() provided
* source} does not correspond naturally to a {@link CallTarget call target}, the returned call
* target should create and if necessary initialize the corresponding language entity and return
* it.
*
* The parsing may be performed in a context (specified by {@link ParsingRequest#getLocation()})
* or without context. The {@code argumentNames} may contain symbolic names for actual
* parameters of the call to the returned value. The result should be a call target with method
* {@link CallTarget#call(java.lang.Object...)} that accepts as many arguments as were provided
* via the {@link ParsingRequest#getArgumentNames()} method.
*
* @param request request for parsing
* @return a call target to invoke which also keeps in memory the {@link Node} tree representing
* just parsed code
* @throws Exception exception can be thrown parsing goes wrong. Here-in thrown exception is
* propagated to the user who called one of eval
methods of
* {@link com.oracle.truffle.api.vm.PolyglotEngine}
* @since 0.22
*/
protected CallTarget parse(ParsingRequest request) throws Exception {
throw new UnsupportedOperationException(
String.format("Override parse method of %s, it will be made abstract in future version of Truffle API!", getClass().getName()));
}
/**
* Request for parsing. Contains information of what to parse and in which context.
*
* @since 0.22
*/
public static final class ParsingRequest {
private final Node node;
private final MaterializedFrame frame;
private final Source source;
private final String[] argumentNames;
private boolean disposed;
ParsingRequest(Source source, Node node, MaterializedFrame frame, String... argumentNames) {
Objects.nonNull(source);
this.node = node;
this.frame = frame;
this.source = source;
this.argumentNames = argumentNames;
}
/**
* The source code to parse.
*
* @return the source code, never null
* @since 0.22
*/
public Source getSource() {
if (disposed) {
throw new IllegalStateException();
}
return source;
}
/**
* Specifies the code location for parsing. The location is specified as an instance of a
* {@link Node} in the AST. There doesn't have to be any specific location and in such case
* this method returns null
. If the node is provided, it can be for example
* {@link com.oracle.truffle.api.instrumentation.EventContext#getInstrumentedNode()} when
* {@link com.oracle.truffle.api.instrumentation.EventContext#parseInContext} is called.
*
*
* @return a {@link Node} defining AST context for the parsing or null
* @since 0.22
*/
public Node getLocation() {
if (disposed) {
throw new IllegalStateException();
}
return node;
}
/**
* Specifies the execution context for parsing. If the parsing request is used for
* evaluation during halted execution, for example as in
* {@link com.oracle.truffle.api.debug.DebugStackFrame#eval(String)} method, this method
* provides access to current {@link MaterializedFrame frame} with local variables, etc.
*
* @return a {@link MaterializedFrame} exposing the current execution state or
* null
if there is none
* @since 0.22
*/
public MaterializedFrame getFrame() {
if (disposed) {
throw new IllegalStateException();
}
return frame;
}
/**
* Argument names. The result of
* {@link #parse(com.oracle.truffle.api.TruffleLanguage.ParsingRequest) parsing} is an
* instance of {@link CallTarget} that {@link CallTarget#call(java.lang.Object...) can be
* invoked} without or with some parameters. If the invocation requires some arguments, and
* the {@link #getSource()} references them, it is essential to name them. Example that uses
* the argument names:
*
* {@link TruffleLanguageSnippets#parseWithParams}
*
* @return symbolic names for parameters of {@link CallTarget#call(java.lang.Object...)}
* @since 0.22
*/
public List getArgumentNames() {
if (disposed) {
throw new IllegalStateException();
}
return argumentNames == null ? Collections. emptyList() : ReadOnlyArrayList.asList(argumentNames, 0, argumentNames.length);
}
void dispose() {
disposed = true;
}
CallTarget parse(TruffleLanguage> truffleLanguage) throws Exception {
try {
return truffleLanguage.parse(this);
} catch (UnsupportedOperationException ex) {
return truffleLanguage.parse(source, node, argumentNames);
}
}
}
/**
* Called when some other language is seeking for a global symbol. This method is supposed to do
* lazy binding, e.g. there is no need to export symbols in advance, it is fine to wait until
* somebody asks for it (by calling this method).
*
* The exported object can either be TruffleObject
(e.g. a native object from the
* other language) to support interoperability between languages, {@link String} or one of the
* Java primitive wrappers ( {@link Integer}, {@link Double}, {@link Short}, {@link Boolean},
* etc.).
*
* The way a symbol becomes exported is language dependent. In general it is preferred
* to make the export explicit - e.g. call some function or method to register an object under
* specific name. Some languages may however decide to support implicit export of symbols (for
* example from global scope, if they have one). However explicit exports should always be
* preferred. Implicitly exported object of some name should only be used when there is no
* explicit export under such globalName
. To ensure so the infrastructure first
* asks all known languages for onlyExplicit
symbols and only when none is found,
* it does one more round with onlyExplicit
set to false
.
*
* @param context context to locate the global symbol in
* @param globalName the name of the global symbol to find
* @param onlyExplicit should the language seek for implicitly exported object or only consider
* the explicitly exported ones?
* @return an exported object or null
, if the symbol does not represent anything
* meaningful in this language
* @since 0.8 or earlier
*/
protected abstract Object findExportedSymbol(C context, String globalName, boolean onlyExplicit);
/**
* Returns global object for the language.
*
* The object is expected to be TruffleObject
(e.g. a native object from the other
* language) but technically it can be one of the Java primitive wrappers ({@link Integer},
* {@link Double}, {@link Short}, etc.).
*
* @param context context to find the language global in
* @return the global object or null
if the language does not support such concept
* @since 0.8 or earlier
*/
protected abstract Object getLanguageGlobal(C context);
/**
* Checks whether the object is provided by this language.
*
* @param object the object to check
* @return true
if this language can deal with such object in native way
* @since 0.8 or earlier
*/
protected abstract boolean isObjectOfLanguage(Object object);
/**
* Runs source code in a halted execution context, or at top level.
*
* @param source the code to run
* @param node node where execution halted, {@code null} if no execution context
* @param mFrame frame where execution halted, {@code null} if no execution context
* @return result of running the code in the context, or at top level if no execution context.
* @throws Exception if the evaluation cannot be performed
* @since 0.8 or earlier
* @deprecated override {@link #parse(com.oracle.truffle.api.TruffleLanguage.ParsingRequest)}
* and use {@link ParsingRequest#getFrame()} to obtain the current frame information
*/
@Deprecated
protected Object evalInContext(Source source, Node node, MaterializedFrame mFrame) throws IOException {
ParsingRequest request = new ParsingRequest(source, node, mFrame);
CallTarget target;
try {
target = parse(request);
} catch (Exception ex) {
if (ex instanceof IOException) {
throw (IOException) ex;
}
if (ex instanceof RuntimeException) {
throw (RuntimeException) ex;
}
throw new RuntimeException(ex);
}
return target.call();
}
/**
* Generates language specific textual representation of a value. Each language may have special
* formating conventions - even primitive values may not follow the traditional Java formating
* rules. As such when {@link com.oracle.truffle.api.vm.PolyglotEngine.Value#as(java.lang.Class)
* value.as(String.class)} is requested, it consults the language that produced the value by
* calling this method. By default this method calls {@link Objects#toString(java.lang.Object)}.
*
* @param context the execution context for doing the conversion
* @param value the value to convert. Either primitive type or
* {@link com.oracle.truffle.api.interop.TruffleObject}
* @return textual representation of the value in this language
* @since 0.8 or earlier
*/
protected String toString(C context, Object value) {
return Objects.toString(value);
}
/**
* Decides whether the result of evaluating an interactive source should be printed to stdout.
* By default this methods returns true
claiming all values are visible.
*
* This method affects behavior of {@link com.oracle.truffle.api.vm.PolyglotEngine#eval} - when
* evaluating an {@link Source#isInteractive() interactive source} the result of the
* {@link com.oracle.truffle.api.vm.PolyglotEngine#eval evaluation} is tested for
* {@link #isVisible(java.lang.Object, java.lang.Object) visibility} and if the value is found
* visible, it gets {@link TruffleLanguage#toString(java.lang.Object, java.lang.Object)
* converted to string} and printed to
* {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setOut standard output}.
*
* A language can control whether a value is or isn't printed by overriding this method and
* returning false
for some or all values. In such case it is up to the language
* itself to use {@link com.oracle.truffle.api.vm.PolyglotEngine polyglot engine}
* {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setOut output},
* {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setErr error} and
* {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setIn input} streams. When
* {@link com.oracle.truffle.api.vm.PolyglotEngine#eval} is called over an
* {@link Source#isInteractive() interactive source} of a language that controls its interactive
* behavior, it is the reponsibility of the language itself to print the result to
* {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setOut(OutputStream) standard output}
* or {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setErr(OutputStream) error output}
* and/or access {@link com.oracle.truffle.api.vm.PolyglotEngine.Builder#setIn(InputStream)
* standard input} in an appropriate way.
*
* @param context the execution context for doing the conversion
* @param value the value to check. Either primitive type or
* {@link com.oracle.truffle.api.interop.TruffleObject}
* @return true
if the language implements an interactive response to evaluation of
* interactive sources.
* @since 0.22
*/
protected boolean isVisible(C context, Object value) {
return true;
}
/**
* Looks an additional language service up. By default it checks if the language itself is
* implementing the requested class and if so, it returns this
.
*
* In future this method can be made protected and overridable by language implementors to
* create more dynamic service system.
*
* @param the type to request
* @param clazz
* @return
*/
final /* protected */ T lookup(Class clazz) {
if (clazz.isInterface()) {
if (clazz.isInstance(this)) {
return clazz.cast(this);
}
}
return null;
}
/**
* Find a meta-object of a value, if any. The meta-object represents a description of the
* object, reveals it's kind and it's features. Some information that a meta-object might define
* includes the base object's type, interface, class, methods, attributes, etc.
*
* A programmatic {@link #toString(java.lang.Object, java.lang.Object) textual representation}
* should be provided for meta-objects, when possible. The meta-object may have properties
* describing their structure.
*
* When no meta-object is known, return null
. The default implementation returns
* null
.
*
* @param context the execution context
* @param value a value to find the meta-object of
* @return the meta-object, or null
* @since 0.22
*/
protected Object findMetaObject(C context, Object value) {
return null;
}
/**
* Find a source location where a value is declared, if any. This is often useful especially for
* retrieval of source locations of {@link #findMetaObject meta-objects}. The default
* implementation returns null
.
*
* @param context the execution context
* @param value a value to get the source location for
* @return a source location of the object, or null
* @since 0.22
*/
protected SourceSection findSourceLocation(C context, Object value) {
return null;
}
/**
* @since 0.8 or earlier
* @deprecated in 0.25 use {@link #getContextReference()} instead
*/
@Deprecated
protected final Node createFindContextNode() {
return AccessAPI.engineAccess().createFindContextNode(this);
}
/**
* @since 0.8 or earlier
* @deprecated in 0.25 use {@linkplain #getContextReference()}.
* {@linkplain ContextReference#get() get()} instead
*/
@SuppressWarnings({"rawtypes", "unchecked", "deprecation"})
@Deprecated
protected final C findContext(Node n) {
com.oracle.truffle.api.impl.FindContextNode fcn = (com.oracle.truffle.api.impl.FindContextNode) n;
if (fcn.getTruffleLanguage() != this) {
throw new ClassCastException();
}
return (C) fcn.executeFindContext();
}
/**
* Creates a reference to the current context to be stored in an AST. The current context can be
* accessed using the {@link ContextReference#get()} method of the returned reference. If a
* context reference is created in the language class constructor an
* {@link IllegalStateException} is thrown. The exception is also thrown if the reference is
* tried to be created or accessed outside of the execution of an engine.
*
* The returned reference identity is undefined. It might either return always the same instance
* or a new reference for each invocation of the method. Please note that the current context
* might vary between {@link RootNode#execute(VirtualFrame) executions} if resources or code is
* shared between multiple contexts.
*
* @since 0.25
*/
public final ContextReference getContextReference() {
if (reference == null) {
throw new IllegalStateException("TruffleLanguage instance is not initialized. Cannot get the current context reference.");
}
return reference;
}
void initialize(LanguageInfo language, boolean singleton) {
this.singletonLanguage = singleton;
if (!singleton) {
this.languageInfo = language;
this.reference = new ContextReference<>(API.nodes().getEngineObject(languageInfo));
}
}
CallTarget parse(Source source, Node context, MaterializedFrame frame, String... argumentNames) {
ParsingRequest request = new ParsingRequest(source, context, frame, argumentNames);
CallTarget target;
try {
target = request.parse(this);
} catch (RuntimeException ex) {
throw ex;
} catch (Exception ex) {
throw new RuntimeException(ex);
} finally {
request.dispose();
}
return target;
}
/**
* Represents execution environment of the {@link TruffleLanguage}. Each active
* {@link TruffleLanguage} receives instance of the environment before any code is executed upon
* it. The environment has knowledge of all active languages and can exchange symbols between
* them.
*
* @since 0.8 or earlier
*/
public static final class Env {
private final Object vmObject; // PolyglotEngine.Language
private final LanguageInfo language;
private final TruffleLanguage