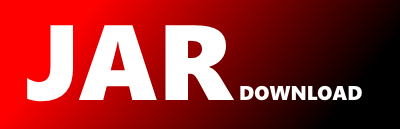
com.oracle.truffle.api.interop.java.ArrayWriteNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-api Show documentation
Show all versions of truffle-api Show documentation
Truffle is a multi-language framework for executing dynamic languages
that achieves high performance when combined with Graal.
// CheckStyle: start generated
package com.oracle.truffle.api.interop.java;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.ExplodeLoop.LoopExplosionKind;
import java.util.concurrent.locks.Lock;
@GeneratedBy(ArrayWriteNode.class)
final class ArrayWriteNodeGen extends ArrayWriteNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private ArrayCachedData arrayCached_cache;
private ArrayWriteNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
protected Object executeWithTarget(JavaObject arg0Value, Object arg1Value, Object arg2Value) {
int state = state_;
if (state != 0 /* is-active doArrayIntIndex(JavaObject, int, Object) || doArrayCached(JavaObject, Number, Object, Class extends Number>) || doArrayGeneric(JavaObject, Number, Object) || doListIntIndex(JavaObject, int, Object) || doListGeneric(JavaObject, Number, Object) || notArray(JavaObject, Number, Object) */) {
if ((state & 0b1) != 0 /* is-active doArrayIntIndex(JavaObject, int, Object) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((arg0Value.isArray())) {
return doArrayIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if ((state & 0b110) != 0 /* is-active doArrayCached(JavaObject, Number, Object, Class extends Number>) || doArrayGeneric(JavaObject, Number, Object) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10) != 0 /* is-active doArrayCached(JavaObject, Number, Object, Class extends Number>) */ && (arg0Value.isArray())) {
ArrayCachedData s2_ = this.arrayCached_cache;
while (s2_ != null) {
if ((arg1Value_.getClass() == s2_.clazz_)) {
return doArrayCached(arg0Value, arg1Value_, arg2Value, s2_.clazz_);
}
s2_ = s2_.next_;
}
}
if ((state & 0b100) != 0 /* is-active doArrayGeneric(JavaObject, Number, Object) */) {
if ((arg0Value.isArray())) {
return doArrayGeneric(arg0Value, arg1Value_, arg2Value);
}
}
}
if ((state & 0b1000) != 0 /* is-active doListIntIndex(JavaObject, int, Object) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayWriteNode.isList(arg0Value))) {
return doListIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if ((state & 0b110000) != 0 /* is-active doListGeneric(JavaObject, Number, Object) || notArray(JavaObject, Number, Object) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10000) != 0 /* is-active doListGeneric(JavaObject, Number, Object) */) {
if ((ArrayWriteNode.isList(arg0Value))) {
return doListGeneric(arg0Value, arg1Value_, arg2Value);
}
}
if ((state & 0b100000) != 0 /* is-active notArray(JavaObject, Number, Object) */) {
if ((!(arg0Value.isArray())) && (!(ArrayWriteNode.isList(arg0Value)))) {
return ArrayWriteNode.notArray(arg0Value, arg1Value_, arg2Value);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(JavaObject arg0Value, Object arg1Value, Object arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((arg0Value.isArray())) {
this.state_ = state = state | 0b1 /* add-active doArrayIntIndex(JavaObject, int, Object) */;
lock.unlock();
hasLock = false;
return doArrayIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if (((exclude & 0b1)) == 0 /* is-not-excluded doArrayCached(JavaObject, Number, Object, Class extends Number>) */ && (arg0Value.isArray())) {
int count2_ = 0;
ArrayCachedData s2_ = this.arrayCached_cache;
if ((state & 0b10) != 0 /* is-active doArrayCached(JavaObject, Number, Object, Class extends Number>) */) {
while (s2_ != null) {
if ((arg1Value_.getClass() == s2_.clazz_)) {
break;
}
s2_ = s2_.next_;
count2_++;
}
}
if (s2_ == null) {
{
Class extends Number> clazz__ = (arg1Value_.getClass());
if ((arg1Value_.getClass() == clazz__) && count2_ < (3)) {
s2_ = new ArrayCachedData(arrayCached_cache);
s2_.clazz_ = clazz__;
this.arrayCached_cache = s2_;
this.state_ = state = state | 0b10 /* add-active doArrayCached(JavaObject, Number, Object, Class extends Number>) */;
}
}
}
if (s2_ != null) {
lock.unlock();
hasLock = false;
return doArrayCached(arg0Value, arg1Value_, arg2Value, s2_.clazz_);
}
}
if ((arg0Value.isArray())) {
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doArrayCached(JavaObject, Number, Object, Class extends Number>) */;
this.arrayCached_cache = null;
state = state & 0xfffffffd /* remove-active doArrayCached(JavaObject, Number, Object, Class extends Number>) */;
this.state_ = state = state | 0b100 /* add-active doArrayGeneric(JavaObject, Number, Object) */;
lock.unlock();
hasLock = false;
return doArrayGeneric(arg0Value, arg1Value_, arg2Value);
}
}
if (((exclude & 0b10)) == 0 /* is-not-excluded doListIntIndex(JavaObject, int, Object) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayWriteNode.isList(arg0Value))) {
this.state_ = state = state | 0b1000 /* add-active doListIntIndex(JavaObject, int, Object) */;
lock.unlock();
hasLock = false;
return doListIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((ArrayWriteNode.isList(arg0Value))) {
this.exclude_ = exclude = exclude | 0b10 /* add-excluded doListIntIndex(JavaObject, int, Object) */;
state = state & 0xfffffff7 /* remove-active doListIntIndex(JavaObject, int, Object) */;
this.state_ = state = state | 0b10000 /* add-active doListGeneric(JavaObject, Number, Object) */;
lock.unlock();
hasLock = false;
return doListGeneric(arg0Value, arg1Value_, arg2Value);
}
if ((!(arg0Value.isArray())) && (!(ArrayWriteNode.isList(arg0Value)))) {
this.state_ = state = state | 0b100000 /* add-active notArray(JavaObject, Number, Object) */;
lock.unlock();
hasLock = false;
return ArrayWriteNode.notArray(arg0Value, arg1Value_, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
ArrayCachedData s2_ = this.arrayCached_cache;
if ((s2_ == null || s2_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static ArrayWriteNode create() {
return new ArrayWriteNodeGen();
}
@GeneratedBy(ArrayWriteNode.class)
private static final class ArrayCachedData {
@CompilationFinal ArrayCachedData next_;
@CompilationFinal Class extends Number> clazz_;
ArrayCachedData(ArrayCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(ArraySet.class)
static final class ArraySetNodeGen extends ArraySet {
@CompilationFinal private int state_;
private ArraySetNodeGen() {
}
@Override
protected void execute(Object arg0Value, int arg1Value, Object arg2Value) {
int state = state_;
if (state != 0 /* is-active doBoolean(boolean[], int, boolean) || doByte(byte[], int, byte) || doShort(short[], int, short) || doChar(char[], int, char) || doInt(int[], int, int) || doLong(long[], int, long) || doFloat(float[], int, float) || doDouble(double[], int, double) || doObject(Object[], int, Object) */) {
if ((state & 0b1) != 0 /* is-active doBoolean(boolean[], int, boolean) */ && arg0Value instanceof boolean[]) {
boolean[] arg0Value_ = (boolean[]) arg0Value;
if (arg2Value instanceof Boolean) {
boolean arg2Value_ = (boolean) arg2Value;
doBoolean(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b10) != 0 /* is-active doByte(byte[], int, byte) */ && arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
if (arg2Value instanceof Byte) {
byte arg2Value_ = (byte) arg2Value;
doByte(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b100) != 0 /* is-active doShort(short[], int, short) */ && arg0Value instanceof short[]) {
short[] arg0Value_ = (short[]) arg0Value;
if (arg2Value instanceof Short) {
short arg2Value_ = (short) arg2Value;
doShort(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b1000) != 0 /* is-active doChar(char[], int, char) */ && arg0Value instanceof char[]) {
char[] arg0Value_ = (char[]) arg0Value;
if (arg2Value instanceof Character) {
char arg2Value_ = (char) arg2Value;
doChar(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b10000) != 0 /* is-active doInt(int[], int, int) */ && arg0Value instanceof int[]) {
int[] arg0Value_ = (int[]) arg0Value;
if (arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
doInt(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b100000) != 0 /* is-active doLong(long[], int, long) */ && arg0Value instanceof long[]) {
long[] arg0Value_ = (long[]) arg0Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
doLong(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b1000000) != 0 /* is-active doFloat(float[], int, float) */ && arg0Value instanceof float[]) {
float[] arg0Value_ = (float[]) arg0Value;
if (arg2Value instanceof Float) {
float arg2Value_ = (float) arg2Value;
doFloat(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b10000000) != 0 /* is-active doDouble(double[], int, double) */ && arg0Value instanceof double[]) {
double[] arg0Value_ = (double[]) arg0Value;
if (arg2Value instanceof Double) {
double arg2Value_ = (double) arg2Value;
doDouble(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b100000000) != 0 /* is-active doObject(Object[], int, Object) */ && arg0Value instanceof Object[]) {
Object[] arg0Value_ = (Object[]) arg0Value;
doObject(arg0Value_, arg1Value, arg2Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void executeAndSpecialize(Object arg0Value, int arg1Value, Object arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof boolean[]) {
boolean[] arg0Value_ = (boolean[]) arg0Value;
if (arg2Value instanceof Boolean) {
boolean arg2Value_ = (boolean) arg2Value;
this.state_ = state = state | 0b1 /* add-active doBoolean(boolean[], int, boolean) */;
lock.unlock();
hasLock = false;
doBoolean(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
if (arg2Value instanceof Byte) {
byte arg2Value_ = (byte) arg2Value;
this.state_ = state = state | 0b10 /* add-active doByte(byte[], int, byte) */;
lock.unlock();
hasLock = false;
doByte(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof short[]) {
short[] arg0Value_ = (short[]) arg0Value;
if (arg2Value instanceof Short) {
short arg2Value_ = (short) arg2Value;
this.state_ = state = state | 0b100 /* add-active doShort(short[], int, short) */;
lock.unlock();
hasLock = false;
doShort(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof char[]) {
char[] arg0Value_ = (char[]) arg0Value;
if (arg2Value instanceof Character) {
char arg2Value_ = (char) arg2Value;
this.state_ = state = state | 0b1000 /* add-active doChar(char[], int, char) */;
lock.unlock();
hasLock = false;
doChar(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof int[]) {
int[] arg0Value_ = (int[]) arg0Value;
if (arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
this.state_ = state = state | 0b10000 /* add-active doInt(int[], int, int) */;
lock.unlock();
hasLock = false;
doInt(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof long[]) {
long[] arg0Value_ = (long[]) arg0Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
this.state_ = state = state | 0b100000 /* add-active doLong(long[], int, long) */;
lock.unlock();
hasLock = false;
doLong(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof float[]) {
float[] arg0Value_ = (float[]) arg0Value;
if (arg2Value instanceof Float) {
float arg2Value_ = (float) arg2Value;
this.state_ = state = state | 0b1000000 /* add-active doFloat(float[], int, float) */;
lock.unlock();
hasLock = false;
doFloat(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof double[]) {
double[] arg0Value_ = (double[]) arg0Value;
if (arg2Value instanceof Double) {
double arg2Value_ = (double) arg2Value;
this.state_ = state = state | 0b10000000 /* add-active doDouble(double[], int, double) */;
lock.unlock();
hasLock = false;
doDouble(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof Object[]) {
Object[] arg0Value_ = (Object[]) arg0Value;
this.state_ = state = state | 0b100000000 /* add-active doObject(Object[], int, Object) */;
lock.unlock();
hasLock = false;
doObject(arg0Value_, arg1Value, arg2Value);
return;
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
public static ArraySet create() {
return new ArraySetNodeGen();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy