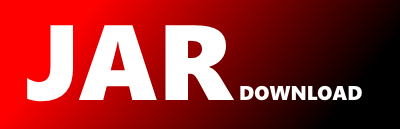
com.oracle.truffle.polyglot.HostObjectMRFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-api Show documentation
Show all versions of truffle-api Show documentation
Truffle is a multi-language framework for executing dynamic languages
that achieves high performance when combined with Graal.
// CheckStyle: start generated
package com.oracle.truffle.polyglot;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.ExplodeLoop.LoopExplosionKind;
import com.oracle.truffle.polyglot.HostObjectMR.ArrayReadNode;
import com.oracle.truffle.polyglot.HostObjectMR.ArrayRemoveNode;
import com.oracle.truffle.polyglot.HostObjectMR.ArrayWriteNode;
import com.oracle.truffle.polyglot.HostObjectMR.KeyInfoCacheNode;
import com.oracle.truffle.polyglot.HostObjectMR.LookupConstructorNode;
import com.oracle.truffle.polyglot.HostObjectMR.LookupFieldNode;
import com.oracle.truffle.polyglot.HostObjectMR.LookupFunctionalMethodNode;
import com.oracle.truffle.polyglot.HostObjectMR.LookupInnerClassNode;
import com.oracle.truffle.polyglot.HostObjectMR.LookupMethodNode;
import com.oracle.truffle.polyglot.HostObjectMR.MapRemoveNode;
import com.oracle.truffle.polyglot.HostObjectMR.ReadFieldNode;
import com.oracle.truffle.polyglot.HostObjectMR.WriteFieldNode;
import com.oracle.truffle.polyglot.PolyglotLanguageContext.ToGuestValueNode;
import java.util.concurrent.locks.Lock;
@GeneratedBy(HostObjectMR.class)
final class HostObjectMRFactory {
@GeneratedBy(ArrayReadNode.class)
static final class ArrayReadNodeGen extends ArrayReadNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private ArrayCachedData arrayCached_cache;
private ArrayReadNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
protected Object executeWithTarget(HostObject arg0Value, Object arg1Value) {
int state = state_;
if (state != 0 /* is-active doArrayIntIndex(HostObject, int) || doArrayCached(HostObject, Number, Class extends Number>) || doArrayGeneric(HostObject, Number) || doListIntIndex(HostObject, int) || doListGeneric(HostObject, Number) || notArray(HostObject, Number) */) {
if ((state & 0b1) != 0 /* is-active doArrayIntIndex(HostObject, int) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((arg0Value.isArray())) {
return doArrayIntIndex(arg0Value, arg1Value_);
}
}
if ((state & 0b110) != 0 /* is-active doArrayCached(HostObject, Number, Class extends Number>) || doArrayGeneric(HostObject, Number) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10) != 0 /* is-active doArrayCached(HostObject, Number, Class extends Number>) */ && (arg0Value.isArray())) {
ArrayCachedData s2_ = this.arrayCached_cache;
while (s2_ != null) {
if ((arg1Value_.getClass() == s2_.clazz_)) {
return doArrayCached(arg0Value, arg1Value_, s2_.clazz_);
}
s2_ = s2_.next_;
}
}
if ((state & 0b100) != 0 /* is-active doArrayGeneric(HostObject, Number) */) {
if ((arg0Value.isArray())) {
return doArrayGeneric(arg0Value, arg1Value_);
}
}
}
if ((state & 0b1000) != 0 /* is-active doListIntIndex(HostObject, int) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayReadNode.isList(arg0Value))) {
return doListIntIndex(arg0Value, arg1Value_);
}
}
if ((state & 0b110000) != 0 /* is-active doListGeneric(HostObject, Number) || notArray(HostObject, Number) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10000) != 0 /* is-active doListGeneric(HostObject, Number) */) {
if ((ArrayReadNode.isList(arg0Value))) {
return doListGeneric(arg0Value, arg1Value_);
}
}
if ((state & 0b100000) != 0 /* is-active notArray(HostObject, Number) */) {
if ((!(arg0Value.isArray())) && (!(ArrayReadNode.isList(arg0Value)))) {
return ArrayReadNode.notArray(arg0Value, arg1Value_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(HostObject arg0Value, Object arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if (((exclude & 0b1)) == 0 /* is-not-excluded doArrayIntIndex(HostObject, int) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((arg0Value.isArray())) {
this.state_ = state = state | 0b1 /* add-active doArrayIntIndex(HostObject, int) */;
lock.unlock();
hasLock = false;
return doArrayIntIndex(arg0Value, arg1Value_);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if (((exclude & 0b10)) == 0 /* is-not-excluded doArrayCached(HostObject, Number, Class extends Number>) */ && (arg0Value.isArray())) {
int count2_ = 0;
ArrayCachedData s2_ = this.arrayCached_cache;
if ((state & 0b10) != 0 /* is-active doArrayCached(HostObject, Number, Class extends Number>) */) {
while (s2_ != null) {
if ((arg1Value_.getClass() == s2_.clazz_)) {
break;
}
s2_ = s2_.next_;
count2_++;
}
}
if (s2_ == null) {
{
Class extends Number> clazz__ = (arg1Value_.getClass());
if ((arg1Value_.getClass() == clazz__) && count2_ < (3)) {
s2_ = new ArrayCachedData(arrayCached_cache);
s2_.clazz_ = clazz__;
this.arrayCached_cache = s2_;
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doArrayIntIndex(HostObject, int) */;
state = state & 0xfffffffe /* remove-active doArrayIntIndex(HostObject, int) */;
this.state_ = state = state | 0b10 /* add-active doArrayCached(HostObject, Number, Class extends Number>) */;
}
}
}
if (s2_ != null) {
lock.unlock();
hasLock = false;
return doArrayCached(arg0Value, arg1Value_, s2_.clazz_);
}
}
if ((arg0Value.isArray())) {
this.exclude_ = exclude = exclude | 0b11 /* add-excluded doArrayIntIndex(HostObject, int), doArrayCached(HostObject, Number, Class extends Number>) */;
this.arrayCached_cache = null;
state = state & 0xfffffffc /* remove-active doArrayIntIndex(HostObject, int), doArrayCached(HostObject, Number, Class extends Number>) */;
this.state_ = state = state | 0b100 /* add-active doArrayGeneric(HostObject, Number) */;
lock.unlock();
hasLock = false;
return doArrayGeneric(arg0Value, arg1Value_);
}
}
if (((exclude & 0b100)) == 0 /* is-not-excluded doListIntIndex(HostObject, int) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayReadNode.isList(arg0Value))) {
this.state_ = state = state | 0b1000 /* add-active doListIntIndex(HostObject, int) */;
lock.unlock();
hasLock = false;
return doListIntIndex(arg0Value, arg1Value_);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((ArrayReadNode.isList(arg0Value))) {
this.exclude_ = exclude = exclude | 0b100 /* add-excluded doListIntIndex(HostObject, int) */;
state = state & 0xfffffff7 /* remove-active doListIntIndex(HostObject, int) */;
this.state_ = state = state | 0b10000 /* add-active doListGeneric(HostObject, Number) */;
lock.unlock();
hasLock = false;
return doListGeneric(arg0Value, arg1Value_);
}
if ((!(arg0Value.isArray())) && (!(ArrayReadNode.isList(arg0Value)))) {
this.state_ = state = state | 0b100000 /* add-active notArray(HostObject, Number) */;
lock.unlock();
hasLock = false;
return ArrayReadNode.notArray(arg0Value, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
ArrayCachedData s2_ = this.arrayCached_cache;
if ((s2_ == null || s2_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static ArrayReadNode create() {
return new ArrayReadNodeGen();
}
@GeneratedBy(ArrayReadNode.class)
private static final class ArrayCachedData {
@CompilationFinal ArrayCachedData next_;
@CompilationFinal Class extends Number> clazz_;
ArrayCachedData(ArrayCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(ArrayGet.class)
static final class ArrayGetNodeGen extends ArrayGet {
@CompilationFinal private int state_;
private ArrayGetNodeGen() {
}
@Override
protected Object execute(Object arg0Value, int arg1Value) {
int state = state_;
if (state != 0 /* is-active doBoolean(boolean[], int) || doByte(byte[], int) || doShort(short[], int) || doChar(char[], int) || doInt(int[], int) || doLong(long[], int) || doFloat(float[], int) || doDouble(double[], int) || doObject(Object[], int) */) {
if ((state & 0b1) != 0 /* is-active doBoolean(boolean[], int) */ && arg0Value instanceof boolean[]) {
boolean[] arg0Value_ = (boolean[]) arg0Value;
return doBoolean(arg0Value_, arg1Value);
}
if ((state & 0b10) != 0 /* is-active doByte(byte[], int) */ && arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
return doByte(arg0Value_, arg1Value);
}
if ((state & 0b100) != 0 /* is-active doShort(short[], int) */ && arg0Value instanceof short[]) {
short[] arg0Value_ = (short[]) arg0Value;
return doShort(arg0Value_, arg1Value);
}
if ((state & 0b1000) != 0 /* is-active doChar(char[], int) */ && arg0Value instanceof char[]) {
char[] arg0Value_ = (char[]) arg0Value;
return doChar(arg0Value_, arg1Value);
}
if ((state & 0b10000) != 0 /* is-active doInt(int[], int) */ && arg0Value instanceof int[]) {
int[] arg0Value_ = (int[]) arg0Value;
return doInt(arg0Value_, arg1Value);
}
if ((state & 0b100000) != 0 /* is-active doLong(long[], int) */ && arg0Value instanceof long[]) {
long[] arg0Value_ = (long[]) arg0Value;
return doLong(arg0Value_, arg1Value);
}
if ((state & 0b1000000) != 0 /* is-active doFloat(float[], int) */ && arg0Value instanceof float[]) {
float[] arg0Value_ = (float[]) arg0Value;
return doFloat(arg0Value_, arg1Value);
}
if ((state & 0b10000000) != 0 /* is-active doDouble(double[], int) */ && arg0Value instanceof double[]) {
double[] arg0Value_ = (double[]) arg0Value;
return doDouble(arg0Value_, arg1Value);
}
if ((state & 0b100000000) != 0 /* is-active doObject(Object[], int) */ && arg0Value instanceof Object[]) {
Object[] arg0Value_ = (Object[]) arg0Value;
return doObject(arg0Value_, arg1Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, int arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof boolean[]) {
boolean[] arg0Value_ = (boolean[]) arg0Value;
this.state_ = state = state | 0b1 /* add-active doBoolean(boolean[], int) */;
lock.unlock();
hasLock = false;
return doBoolean(arg0Value_, arg1Value);
}
if (arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
this.state_ = state = state | 0b10 /* add-active doByte(byte[], int) */;
lock.unlock();
hasLock = false;
return doByte(arg0Value_, arg1Value);
}
if (arg0Value instanceof short[]) {
short[] arg0Value_ = (short[]) arg0Value;
this.state_ = state = state | 0b100 /* add-active doShort(short[], int) */;
lock.unlock();
hasLock = false;
return doShort(arg0Value_, arg1Value);
}
if (arg0Value instanceof char[]) {
char[] arg0Value_ = (char[]) arg0Value;
this.state_ = state = state | 0b1000 /* add-active doChar(char[], int) */;
lock.unlock();
hasLock = false;
return doChar(arg0Value_, arg1Value);
}
if (arg0Value instanceof int[]) {
int[] arg0Value_ = (int[]) arg0Value;
this.state_ = state = state | 0b10000 /* add-active doInt(int[], int) */;
lock.unlock();
hasLock = false;
return doInt(arg0Value_, arg1Value);
}
if (arg0Value instanceof long[]) {
long[] arg0Value_ = (long[]) arg0Value;
this.state_ = state = state | 0b100000 /* add-active doLong(long[], int) */;
lock.unlock();
hasLock = false;
return doLong(arg0Value_, arg1Value);
}
if (arg0Value instanceof float[]) {
float[] arg0Value_ = (float[]) arg0Value;
this.state_ = state = state | 0b1000000 /* add-active doFloat(float[], int) */;
lock.unlock();
hasLock = false;
return doFloat(arg0Value_, arg1Value);
}
if (arg0Value instanceof double[]) {
double[] arg0Value_ = (double[]) arg0Value;
this.state_ = state = state | 0b10000000 /* add-active doDouble(double[], int) */;
lock.unlock();
hasLock = false;
return doDouble(arg0Value_, arg1Value);
}
if (arg0Value instanceof Object[]) {
Object[] arg0Value_ = (Object[]) arg0Value;
this.state_ = state = state | 0b100000000 /* add-active doObject(Object[], int) */;
lock.unlock();
hasLock = false;
return doObject(arg0Value_, arg1Value);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
public static ArrayGet create() {
return new ArrayGetNodeGen();
}
}
}
@GeneratedBy(ArrayWriteNode.class)
static final class ArrayWriteNodeGen extends ArrayWriteNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private ArrayCachedData arrayCached_cache;
private ArrayWriteNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
protected Object executeWithTarget(HostObject arg0Value, Object arg1Value, Object arg2Value) {
int state = state_;
if (state != 0 /* is-active doArrayIntIndex(HostObject, int, Object) || doArrayCached(HostObject, Number, Object, Class extends Number>) || doArrayGeneric(HostObject, Number, Object) || doListIntIndex(HostObject, int, Object) || doListGeneric(HostObject, Number, Object) || notArray(HostObject, Number, Object) */) {
if ((state & 0b1) != 0 /* is-active doArrayIntIndex(HostObject, int, Object) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((arg0Value.isArray())) {
return doArrayIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if ((state & 0b110) != 0 /* is-active doArrayCached(HostObject, Number, Object, Class extends Number>) || doArrayGeneric(HostObject, Number, Object) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10) != 0 /* is-active doArrayCached(HostObject, Number, Object, Class extends Number>) */ && (arg0Value.isArray())) {
ArrayCachedData s2_ = this.arrayCached_cache;
while (s2_ != null) {
if ((arg1Value_.getClass() == s2_.clazz_)) {
return doArrayCached(arg0Value, arg1Value_, arg2Value, s2_.clazz_);
}
s2_ = s2_.next_;
}
}
if ((state & 0b100) != 0 /* is-active doArrayGeneric(HostObject, Number, Object) */) {
if ((arg0Value.isArray())) {
return doArrayGeneric(arg0Value, arg1Value_, arg2Value);
}
}
}
if ((state & 0b1000) != 0 /* is-active doListIntIndex(HostObject, int, Object) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayWriteNode.isList(arg0Value))) {
return doListIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if ((state & 0b110000) != 0 /* is-active doListGeneric(HostObject, Number, Object) || notArray(HostObject, Number, Object) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10000) != 0 /* is-active doListGeneric(HostObject, Number, Object) */) {
if ((ArrayWriteNode.isList(arg0Value))) {
return doListGeneric(arg0Value, arg1Value_, arg2Value);
}
}
if ((state & 0b100000) != 0 /* is-active notArray(HostObject, Number, Object) */) {
if ((!(arg0Value.isArray())) && (!(ArrayWriteNode.isList(arg0Value)))) {
return ArrayWriteNode.notArray(arg0Value, arg1Value_, arg2Value);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(HostObject arg0Value, Object arg1Value, Object arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((arg0Value.isArray())) {
this.state_ = state = state | 0b1 /* add-active doArrayIntIndex(HostObject, int, Object) */;
lock.unlock();
hasLock = false;
return doArrayIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if (((exclude & 0b1)) == 0 /* is-not-excluded doArrayCached(HostObject, Number, Object, Class extends Number>) */ && (arg0Value.isArray())) {
int count2_ = 0;
ArrayCachedData s2_ = this.arrayCached_cache;
if ((state & 0b10) != 0 /* is-active doArrayCached(HostObject, Number, Object, Class extends Number>) */) {
while (s2_ != null) {
if ((arg1Value_.getClass() == s2_.clazz_)) {
break;
}
s2_ = s2_.next_;
count2_++;
}
}
if (s2_ == null) {
{
Class extends Number> clazz__ = (arg1Value_.getClass());
if ((arg1Value_.getClass() == clazz__) && count2_ < (3)) {
s2_ = new ArrayCachedData(arrayCached_cache);
s2_.clazz_ = clazz__;
this.arrayCached_cache = s2_;
this.state_ = state = state | 0b10 /* add-active doArrayCached(HostObject, Number, Object, Class extends Number>) */;
}
}
}
if (s2_ != null) {
lock.unlock();
hasLock = false;
return doArrayCached(arg0Value, arg1Value_, arg2Value, s2_.clazz_);
}
}
if ((arg0Value.isArray())) {
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doArrayCached(HostObject, Number, Object, Class extends Number>) */;
this.arrayCached_cache = null;
state = state & 0xfffffffd /* remove-active doArrayCached(HostObject, Number, Object, Class extends Number>) */;
this.state_ = state = state | 0b100 /* add-active doArrayGeneric(HostObject, Number, Object) */;
lock.unlock();
hasLock = false;
return doArrayGeneric(arg0Value, arg1Value_, arg2Value);
}
}
if (((exclude & 0b10)) == 0 /* is-not-excluded doListIntIndex(HostObject, int, Object) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayWriteNode.isList(arg0Value))) {
this.state_ = state = state | 0b1000 /* add-active doListIntIndex(HostObject, int, Object) */;
lock.unlock();
hasLock = false;
return doListIntIndex(arg0Value, arg1Value_, arg2Value);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((ArrayWriteNode.isList(arg0Value))) {
this.exclude_ = exclude = exclude | 0b10 /* add-excluded doListIntIndex(HostObject, int, Object) */;
state = state & 0xfffffff7 /* remove-active doListIntIndex(HostObject, int, Object) */;
this.state_ = state = state | 0b10000 /* add-active doListGeneric(HostObject, Number, Object) */;
lock.unlock();
hasLock = false;
return doListGeneric(arg0Value, arg1Value_, arg2Value);
}
if ((!(arg0Value.isArray())) && (!(ArrayWriteNode.isList(arg0Value)))) {
this.state_ = state = state | 0b100000 /* add-active notArray(HostObject, Number, Object) */;
lock.unlock();
hasLock = false;
return ArrayWriteNode.notArray(arg0Value, arg1Value_, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
ArrayCachedData s2_ = this.arrayCached_cache;
if ((s2_ == null || s2_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static ArrayWriteNode create() {
return new ArrayWriteNodeGen();
}
@GeneratedBy(ArrayWriteNode.class)
private static final class ArrayCachedData {
@CompilationFinal ArrayCachedData next_;
@CompilationFinal Class extends Number> clazz_;
ArrayCachedData(ArrayCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(ArraySet.class)
static final class ArraySetNodeGen extends ArraySet {
@CompilationFinal private int state_;
private ArraySetNodeGen() {
}
@Override
protected void execute(Object arg0Value, int arg1Value, Object arg2Value) {
int state = state_;
if (state != 0 /* is-active doBoolean(boolean[], int, boolean) || doByte(byte[], int, byte) || doShort(short[], int, short) || doChar(char[], int, char) || doInt(int[], int, int) || doLong(long[], int, long) || doFloat(float[], int, float) || doDouble(double[], int, double) || doObject(Object[], int, Object) */) {
if ((state & 0b1) != 0 /* is-active doBoolean(boolean[], int, boolean) */ && arg0Value instanceof boolean[]) {
boolean[] arg0Value_ = (boolean[]) arg0Value;
if (arg2Value instanceof Boolean) {
boolean arg2Value_ = (boolean) arg2Value;
doBoolean(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b10) != 0 /* is-active doByte(byte[], int, byte) */ && arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
if (arg2Value instanceof Byte) {
byte arg2Value_ = (byte) arg2Value;
doByte(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b100) != 0 /* is-active doShort(short[], int, short) */ && arg0Value instanceof short[]) {
short[] arg0Value_ = (short[]) arg0Value;
if (arg2Value instanceof Short) {
short arg2Value_ = (short) arg2Value;
doShort(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b1000) != 0 /* is-active doChar(char[], int, char) */ && arg0Value instanceof char[]) {
char[] arg0Value_ = (char[]) arg0Value;
if (arg2Value instanceof Character) {
char arg2Value_ = (char) arg2Value;
doChar(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b10000) != 0 /* is-active doInt(int[], int, int) */ && arg0Value instanceof int[]) {
int[] arg0Value_ = (int[]) arg0Value;
if (arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
doInt(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b100000) != 0 /* is-active doLong(long[], int, long) */ && arg0Value instanceof long[]) {
long[] arg0Value_ = (long[]) arg0Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
doLong(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b1000000) != 0 /* is-active doFloat(float[], int, float) */ && arg0Value instanceof float[]) {
float[] arg0Value_ = (float[]) arg0Value;
if (arg2Value instanceof Float) {
float arg2Value_ = (float) arg2Value;
doFloat(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b10000000) != 0 /* is-active doDouble(double[], int, double) */ && arg0Value instanceof double[]) {
double[] arg0Value_ = (double[]) arg0Value;
if (arg2Value instanceof Double) {
double arg2Value_ = (double) arg2Value;
doDouble(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if ((state & 0b100000000) != 0 /* is-active doObject(Object[], int, Object) */ && arg0Value instanceof Object[]) {
Object[] arg0Value_ = (Object[]) arg0Value;
doObject(arg0Value_, arg1Value, arg2Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void executeAndSpecialize(Object arg0Value, int arg1Value, Object arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof boolean[]) {
boolean[] arg0Value_ = (boolean[]) arg0Value;
if (arg2Value instanceof Boolean) {
boolean arg2Value_ = (boolean) arg2Value;
this.state_ = state = state | 0b1 /* add-active doBoolean(boolean[], int, boolean) */;
lock.unlock();
hasLock = false;
doBoolean(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
if (arg2Value instanceof Byte) {
byte arg2Value_ = (byte) arg2Value;
this.state_ = state = state | 0b10 /* add-active doByte(byte[], int, byte) */;
lock.unlock();
hasLock = false;
doByte(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof short[]) {
short[] arg0Value_ = (short[]) arg0Value;
if (arg2Value instanceof Short) {
short arg2Value_ = (short) arg2Value;
this.state_ = state = state | 0b100 /* add-active doShort(short[], int, short) */;
lock.unlock();
hasLock = false;
doShort(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof char[]) {
char[] arg0Value_ = (char[]) arg0Value;
if (arg2Value instanceof Character) {
char arg2Value_ = (char) arg2Value;
this.state_ = state = state | 0b1000 /* add-active doChar(char[], int, char) */;
lock.unlock();
hasLock = false;
doChar(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof int[]) {
int[] arg0Value_ = (int[]) arg0Value;
if (arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
this.state_ = state = state | 0b10000 /* add-active doInt(int[], int, int) */;
lock.unlock();
hasLock = false;
doInt(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof long[]) {
long[] arg0Value_ = (long[]) arg0Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
this.state_ = state = state | 0b100000 /* add-active doLong(long[], int, long) */;
lock.unlock();
hasLock = false;
doLong(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof float[]) {
float[] arg0Value_ = (float[]) arg0Value;
if (arg2Value instanceof Float) {
float arg2Value_ = (float) arg2Value;
this.state_ = state = state | 0b1000000 /* add-active doFloat(float[], int, float) */;
lock.unlock();
hasLock = false;
doFloat(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof double[]) {
double[] arg0Value_ = (double[]) arg0Value;
if (arg2Value instanceof Double) {
double arg2Value_ = (double) arg2Value;
this.state_ = state = state | 0b10000000 /* add-active doDouble(double[], int, double) */;
lock.unlock();
hasLock = false;
doDouble(arg0Value_, arg1Value, arg2Value_);
return;
}
}
if (arg0Value instanceof Object[]) {
Object[] arg0Value_ = (Object[]) arg0Value;
this.state_ = state = state | 0b100000000 /* add-active doObject(Object[], int, Object) */;
lock.unlock();
hasLock = false;
doObject(arg0Value_, arg1Value, arg2Value);
return;
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
public static ArraySet create() {
return new ArraySetNodeGen();
}
}
}
@GeneratedBy(MapRemoveNode.class)
static final class MapRemoveNodeGen extends MapRemoveNode {
@CompilationFinal private int state_;
private MapRemoveNodeGen() {
}
@Override
protected Object executeWithTarget(HostObject arg0Value, String arg1Value) {
int state = state_;
if (state != 0 /* is-active doMapGeneric(HostObject, String) || notMap(HostObject, String) */) {
if ((state & 0b1) != 0 /* is-active doMapGeneric(HostObject, String) */) {
if ((MapRemoveNode.isMap(arg0Value))) {
return doMapGeneric(arg0Value, arg1Value);
}
}
if ((state & 0b10) != 0 /* is-active notMap(HostObject, String) */) {
if ((!(MapRemoveNode.isMap(arg0Value)))) {
return MapRemoveNode.notMap(arg0Value, arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(HostObject arg0Value, String arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if ((MapRemoveNode.isMap(arg0Value))) {
this.state_ = state = state | 0b1 /* add-active doMapGeneric(HostObject, String) */;
lock.unlock();
hasLock = false;
return doMapGeneric(arg0Value, arg1Value);
}
if ((!(MapRemoveNode.isMap(arg0Value)))) {
this.state_ = state = state | 0b10 /* add-active notMap(HostObject, String) */;
lock.unlock();
hasLock = false;
return MapRemoveNode.notMap(arg0Value, arg1Value);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
public static MapRemoveNode create() {
return new MapRemoveNodeGen();
}
}
@GeneratedBy(ArrayRemoveNode.class)
static final class ArrayRemoveNodeGen extends ArrayRemoveNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
private ArrayRemoveNodeGen() {
}
@Override
protected boolean executeWithTarget(HostObject arg0Value, Object arg1Value) {
int state = state_;
if (state != 0 /* is-active doListIntIndex(HostObject, int) || doListGeneric(HostObject, Number) || notArray(HostObject, Number) */) {
if ((state & 0b1) != 0 /* is-active doListIntIndex(HostObject, int) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayRemoveNode.isList(arg0Value))) {
return doListIntIndex(arg0Value, arg1Value_);
}
}
if ((state & 0b110) != 0 /* is-active doListGeneric(HostObject, Number) || notArray(HostObject, Number) */ && arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((state & 0b10) != 0 /* is-active doListGeneric(HostObject, Number) */) {
if ((ArrayRemoveNode.isList(arg0Value))) {
return doListGeneric(arg0Value, arg1Value_);
}
}
if ((state & 0b100) != 0 /* is-active notArray(HostObject, Number) */) {
if ((!(ArrayRemoveNode.isList(arg0Value)))) {
return ArrayRemoveNode.notArray(arg0Value, arg1Value_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(HostObject arg0Value, Object arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doListIntIndex(HostObject, int) */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((ArrayRemoveNode.isList(arg0Value))) {
this.state_ = state = state | 0b1 /* add-active doListIntIndex(HostObject, int) */;
lock.unlock();
hasLock = false;
return doListIntIndex(arg0Value, arg1Value_);
}
}
if (arg1Value instanceof Number) {
Number arg1Value_ = (Number) arg1Value;
if ((ArrayRemoveNode.isList(arg0Value))) {
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doListIntIndex(HostObject, int) */;
state = state & 0xfffffffe /* remove-active doListIntIndex(HostObject, int) */;
this.state_ = state = state | 0b10 /* add-active doListGeneric(HostObject, Number) */;
lock.unlock();
hasLock = false;
return doListGeneric(arg0Value, arg1Value_);
}
if ((!(ArrayRemoveNode.isList(arg0Value)))) {
this.state_ = state = state | 0b100 /* add-active notArray(HostObject, Number) */;
lock.unlock();
hasLock = false;
return ArrayRemoveNode.notArray(arg0Value, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
public static ArrayRemoveNode create() {
return new ArrayRemoveNodeGen();
}
}
@GeneratedBy(KeyInfoCacheNode.class)
static final class KeyInfoCacheNodeGen extends KeyInfoCacheNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private KeyInfoCacheNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public int execute(Class> arg0Value, String arg1Value, boolean arg2Value) {
int state = state_;
if (state != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, int) || doUncached(Class<>, String, boolean) */) {
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, int) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg2Value == s1_.cachedStatic_) && (arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
return KeyInfoCacheNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedStatic_, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedKeyInfo_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(Class<>, String, boolean) */) {
return KeyInfoCacheNode.doUncached(arg0Value, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private int executeAndSpecialize(Class> arg0Value, String arg1Value, boolean arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(Class<>, String, boolean, boolean, Class<>, String, int) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, int) */) {
while (s1_ != null) {
if ((arg2Value == s1_.cachedStatic_) && (arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg2Value == s1_.cachedStatic_);
// assert (arg0Value == s1_.cachedClazz_);
// assert (s1_.cachedName_.equals(arg1Value));
if (count1_ < (KeyInfoCacheNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedStatic_ = (arg2Value);
s1_.cachedClazz_ = (arg0Value);
s1_.cachedName_ = (arg1Value);
s1_.cachedKeyInfo_ = (KeyInfoCacheNode.doUncached(arg0Value, arg1Value, arg2Value));
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(Class<>, String, boolean, boolean, Class<>, String, int) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return KeyInfoCacheNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedStatic_, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedKeyInfo_);
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Class<>, String, boolean, boolean, Class<>, String, int) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(Class<>, String, boolean, boolean, Class<>, String, int) */;
this.state_ = state = state | 0b10 /* add-active doUncached(Class<>, String, boolean) */;
lock.unlock();
hasLock = false;
return KeyInfoCacheNode.doUncached(arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static KeyInfoCacheNode create() {
return new KeyInfoCacheNodeGen();
}
@GeneratedBy(KeyInfoCacheNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal boolean cachedStatic_;
@CompilationFinal Class> cachedClazz_;
@CompilationFinal String cachedName_;
@CompilationFinal int cachedKeyInfo_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(LookupConstructorNode.class)
static final class LookupConstructorNodeGen extends LookupConstructorNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private LookupConstructorNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public HostMethodDesc execute(Class> arg0Value) {
int state = state_;
if (state != 0 /* is-active doCached(Class<>, Class<>, HostMethodDesc) || doUncached(Class<>) */) {
if ((state & 0b1) != 0 /* is-active doCached(Class<>, Class<>, HostMethodDesc) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg0Value == s1_.cachedClazz_)) {
return LookupConstructorNode.doCached(arg0Value, s1_.cachedClazz_, s1_.cachedMethod_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(Class<>) */) {
return LookupConstructorNode.doUncached(arg0Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private HostMethodDesc executeAndSpecialize(Class> arg0Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(Class<>, Class<>, HostMethodDesc) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(Class<>, Class<>, HostMethodDesc) */) {
while (s1_ != null) {
if ((arg0Value == s1_.cachedClazz_)) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg0Value == s1_.cachedClazz_);
if (count1_ < (LookupConstructorNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedClazz_ = (arg0Value);
s1_.cachedMethod_ = (LookupConstructorNode.doUncached(arg0Value));
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(Class<>, Class<>, HostMethodDesc) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return LookupConstructorNode.doCached(arg0Value, s1_.cachedClazz_, s1_.cachedMethod_);
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Class<>, Class<>, HostMethodDesc) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(Class<>, Class<>, HostMethodDesc) */;
this.state_ = state = state | 0b10 /* add-active doUncached(Class<>) */;
lock.unlock();
hasLock = false;
return LookupConstructorNode.doUncached(arg0Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static LookupConstructorNode create() {
return new LookupConstructorNodeGen();
}
@GeneratedBy(LookupConstructorNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal Class> cachedClazz_;
@CompilationFinal HostMethodDesc cachedMethod_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(LookupFieldNode.class)
static final class LookupFieldNodeGen extends LookupFieldNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private LookupFieldNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public HostFieldDesc execute(Class> arg0Value, String arg1Value, boolean arg2Value) {
int state = state_;
if (state != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) || doUncached(Class<>, String, boolean) */) {
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg2Value == s1_.cachedStatic_) && (arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
return LookupFieldNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedStatic_, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedField_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(Class<>, String, boolean) */) {
return LookupFieldNode.doUncached(arg0Value, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private HostFieldDesc executeAndSpecialize(Class> arg0Value, String arg1Value, boolean arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) */) {
while (s1_ != null) {
if ((arg2Value == s1_.cachedStatic_) && (arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg2Value == s1_.cachedStatic_);
// assert (arg0Value == s1_.cachedClazz_);
// assert (s1_.cachedName_.equals(arg1Value));
if (count1_ < (LookupFieldNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedStatic_ = (arg2Value);
s1_.cachedClazz_ = (arg0Value);
s1_.cachedName_ = (arg1Value);
s1_.cachedField_ = (LookupFieldNode.doUncached(arg0Value, arg1Value, arg2Value));
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return LookupFieldNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedStatic_, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedField_);
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc) */;
this.state_ = state = state | 0b10 /* add-active doUncached(Class<>, String, boolean) */;
lock.unlock();
hasLock = false;
return LookupFieldNode.doUncached(arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static LookupFieldNode create() {
return new LookupFieldNodeGen();
}
@GeneratedBy(LookupFieldNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal boolean cachedStatic_;
@CompilationFinal Class> cachedClazz_;
@CompilationFinal String cachedName_;
@CompilationFinal HostFieldDesc cachedField_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(LookupFunctionalMethodNode.class)
static final class LookupFunctionalMethodNodeGen extends LookupFunctionalMethodNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private LookupFunctionalMethodNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public HostMethodDesc execute(Class> arg0Value) {
int state = state_;
if (state != 0 /* is-active doCached(Class<>, Class<>, HostMethodDesc) || doUncached(Class<>) */) {
if ((state & 0b1) != 0 /* is-active doCached(Class<>, Class<>, HostMethodDesc) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg0Value == s1_.cachedClazz_)) {
return LookupFunctionalMethodNode.doCached(arg0Value, s1_.cachedClazz_, s1_.cachedMethod_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(Class<>) */) {
return LookupFunctionalMethodNode.doUncached(arg0Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private HostMethodDesc executeAndSpecialize(Class> arg0Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(Class<>, Class<>, HostMethodDesc) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(Class<>, Class<>, HostMethodDesc) */) {
while (s1_ != null) {
if ((arg0Value == s1_.cachedClazz_)) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg0Value == s1_.cachedClazz_);
if (count1_ < (LookupFunctionalMethodNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedClazz_ = (arg0Value);
s1_.cachedMethod_ = (LookupFunctionalMethodNode.doUncached(arg0Value));
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(Class<>, Class<>, HostMethodDesc) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return LookupFunctionalMethodNode.doCached(arg0Value, s1_.cachedClazz_, s1_.cachedMethod_);
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Class<>, Class<>, HostMethodDesc) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(Class<>, Class<>, HostMethodDesc) */;
this.state_ = state = state | 0b10 /* add-active doUncached(Class<>) */;
lock.unlock();
hasLock = false;
return LookupFunctionalMethodNode.doUncached(arg0Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static LookupFunctionalMethodNode create() {
return new LookupFunctionalMethodNodeGen();
}
@GeneratedBy(LookupFunctionalMethodNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal Class> cachedClazz_;
@CompilationFinal HostMethodDesc cachedMethod_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(LookupInnerClassNode.class)
static final class LookupInnerClassNodeGen extends LookupInnerClassNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private LookupInnerClassNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public Class> execute(Class> arg0Value, String arg1Value) {
int state = state_;
if (state != 0 /* is-active doCached(Class<>, String, Class<>, String, Class<>) || doUncached(Class<>, String) */) {
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, Class<>, String, Class<>) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
return LookupInnerClassNode.doCached(arg0Value, arg1Value, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedInnerClass_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(Class<>, String) */) {
return LookupInnerClassNode.doUncached(arg0Value, arg1Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Class> executeAndSpecialize(Class> arg0Value, String arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(Class<>, String, Class<>, String, Class<>) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, Class<>, String, Class<>) */) {
while (s1_ != null) {
if ((arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg0Value == s1_.cachedClazz_);
// assert (s1_.cachedName_.equals(arg1Value));
if (count1_ < (LookupInnerClassNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedClazz_ = (arg0Value);
s1_.cachedName_ = (arg1Value);
s1_.cachedInnerClass_ = (LookupInnerClassNode.doUncached(arg0Value, arg1Value));
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(Class<>, String, Class<>, String, Class<>) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return LookupInnerClassNode.doCached(arg0Value, arg1Value, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedInnerClass_);
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Class<>, String, Class<>, String, Class<>) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(Class<>, String, Class<>, String, Class<>) */;
this.state_ = state = state | 0b10 /* add-active doUncached(Class<>, String) */;
lock.unlock();
hasLock = false;
return LookupInnerClassNode.doUncached(arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static LookupInnerClassNode create() {
return new LookupInnerClassNodeGen();
}
@GeneratedBy(LookupInnerClassNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal Class> cachedClazz_;
@CompilationFinal String cachedName_;
@CompilationFinal Class> cachedInnerClass_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(LookupMethodNode.class)
static final class LookupMethodNodeGen extends LookupMethodNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private LookupMethodNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public HostMethodDesc execute(Class> arg0Value, String arg1Value, boolean arg2Value) {
int state = state_;
if (state != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) || doUncached(Class<>, String, boolean) */) {
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg2Value == s1_.cachedStatic_) && (arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
return LookupMethodNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedStatic_, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedMethod_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(Class<>, String, boolean) */) {
return LookupMethodNode.doUncached(arg0Value, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private HostMethodDesc executeAndSpecialize(Class> arg0Value, String arg1Value, boolean arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) */) {
while (s1_ != null) {
if ((arg2Value == s1_.cachedStatic_) && (arg0Value == s1_.cachedClazz_) && (s1_.cachedName_.equals(arg1Value))) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg2Value == s1_.cachedStatic_);
// assert (arg0Value == s1_.cachedClazz_);
// assert (s1_.cachedName_.equals(arg1Value));
if (count1_ < (LookupMethodNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedStatic_ = (arg2Value);
s1_.cachedClazz_ = (arg0Value);
s1_.cachedName_ = (arg1Value);
s1_.cachedMethod_ = (LookupMethodNode.doUncached(arg0Value, arg1Value, arg2Value));
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return LookupMethodNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedStatic_, s1_.cachedClazz_, s1_.cachedName_, s1_.cachedMethod_);
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc) */;
this.state_ = state = state | 0b10 /* add-active doUncached(Class<>, String, boolean) */;
lock.unlock();
hasLock = false;
return LookupMethodNode.doUncached(arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static LookupMethodNode create() {
return new LookupMethodNodeGen();
}
@GeneratedBy(LookupMethodNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal boolean cachedStatic_;
@CompilationFinal Class> cachedClazz_;
@CompilationFinal String cachedName_;
@CompilationFinal HostMethodDesc cachedMethod_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(ReadFieldNode.class)
static final class ReadFieldNodeGen extends ReadFieldNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
@CompilationFinal private ToGuestValueNode uncached_toGuest_;
private ReadFieldNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public Object execute(HostFieldDesc arg0Value, HostObject arg1Value) {
int state = state_;
if (state != 0 /* is-active doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) || doUncached(HostFieldDesc, HostObject, ToGuestValueNode) */) {
if ((state & 0b1) != 0 /* is-active doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg0Value == s1_.cachedField_)) {
return ReadFieldNode.doCached(arg0Value, arg1Value, s1_.cachedField_, s1_.toGuest_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(HostFieldDesc, HostObject, ToGuestValueNode) */) {
return ReadFieldNode.doUncached(arg0Value, arg1Value, this.uncached_toGuest_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(HostFieldDesc arg0Value, HostObject arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) */) {
while (s1_ != null) {
if ((arg0Value == s1_.cachedField_)) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg0Value == s1_.cachedField_);
if (count1_ < (ReadFieldNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedField_ = (arg0Value);
s1_.toGuest_ = (ToGuestValueNode.create());
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return ReadFieldNode.doCached(arg0Value, arg1Value, s1_.cachedField_, s1_.toGuest_);
}
}
this.uncached_toGuest_ = (ToGuestValueNode.create());
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode) */;
this.state_ = state = state | 0b10 /* add-active doUncached(HostFieldDesc, HostObject, ToGuestValueNode) */;
lock.unlock();
hasLock = false;
return ReadFieldNode.doUncached(arg0Value, arg1Value, this.uncached_toGuest_);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static ReadFieldNode create() {
return new ReadFieldNodeGen();
}
@GeneratedBy(ReadFieldNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal HostFieldDesc cachedField_;
@CompilationFinal ToGuestValueNode toGuest_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
@GeneratedBy(WriteFieldNode.class)
static final class WriteFieldNodeGen extends WriteFieldNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private WriteFieldNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public void execute(HostFieldDesc arg0Value, HostObject arg1Value, Object arg2Value) {
int state = state_;
if (state != 0 /* is-active doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) || doUncached(HostFieldDesc, HostObject, Object) */) {
if ((state & 0b1) != 0 /* is-active doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) */) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg0Value == s1_.cachedField_)) {
doCached(arg0Value, arg1Value, arg2Value, s1_.cachedField_);
return;
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doUncached(HostFieldDesc, HostObject, Object) */) {
doUncached(arg0Value, arg1Value, arg2Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void executeAndSpecialize(HostFieldDesc arg0Value, HostObject arg1Value, Object arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((exclude) == 0 /* is-not-excluded doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) */) {
int count1_ = 0;
CachedData s1_ = this.cached_cache;
if ((state & 0b1) != 0 /* is-active doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) */) {
while (s1_ != null) {
if ((arg0Value == s1_.cachedField_)) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg0Value == s1_.cachedField_);
if (count1_ < (WriteFieldNode.LIMIT)) {
s1_ = new CachedData(cached_cache);
s1_.cachedField_ = (arg0Value);
this.cached_cache = s1_;
this.state_ = state = state | 0b1 /* add-active doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
doCached(arg0Value, arg1Value, arg2Value, s1_.cachedField_);
return;
}
}
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) */;
this.cached_cache = null;
state = state & 0xfffffffe /* remove-active doCached(HostFieldDesc, HostObject, Object, HostFieldDesc) */;
this.state_ = state = state | 0b10 /* add-active doUncached(HostFieldDesc, HostObject, Object) */;
lock.unlock();
hasLock = false;
doUncached(arg0Value, arg1Value, arg2Value);
return;
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static WriteFieldNode create() {
return new WriteFieldNodeGen();
}
@GeneratedBy(WriteFieldNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal HostFieldDesc cachedField_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy