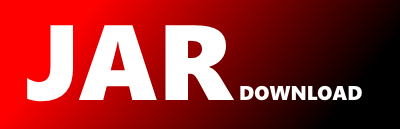
com.oracle.truffle.polyglot.EmptyGlobalBindingsResolutionForeignFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-api Show documentation
Show all versions of truffle-api Show documentation
Truffle is a multi-language framework for executing dynamic languages
that achieves high performance when combined with Graal.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.polyglot;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.polyglot.DefaultScope.EmptyGlobalBindings;
import com.oracle.truffle.polyglot.EmptyGlobalBindingsResolutionForeign.ReadSubNode;
import com.oracle.truffle.polyglot.EmptyGlobalBindingsResolutionForeign.SymbolsHasKeysSubNode;
import com.oracle.truffle.polyglot.EmptyGlobalBindingsResolutionForeign.SymbolsKeyInfoSubNode;
import com.oracle.truffle.polyglot.EmptyGlobalBindingsResolutionForeign.SymbolsKeysSubNode;
import java.util.concurrent.locks.Lock;
@GeneratedBy(EmptyGlobalBindingsResolutionForeign.class)
final class EmptyGlobalBindingsResolutionForeignFactory {
@GeneratedBy(SymbolsKeyInfoSubNode.class)
static final class SymbolsKeyInfoSubNodeGen extends SymbolsKeyInfoSubNode {
@CompilationFinal private int state_;
private SymbolsKeyInfoSubNodeGen() {
}
@Override
public Object executeWithTarget(VirtualFrame frameValue, Object arg0Value, Object arg1Value) {
int state = state_;
if (state != 0 /* is-active accessWithTarget(EmptyGlobalBindings, String) */ && arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
if (arg1Value instanceof String) {
String arg1Value_ = (String) arg1Value;
return accessWithTarget(arg0Value_, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
if (arg1Value instanceof String) {
String arg1Value_ = (String) arg1Value;
this.state_ = state = state | 0b1 /* add-active accessWithTarget(EmptyGlobalBindings, String) */;
lock.unlock();
hasLock = false;
return accessWithTarget(arg0Value_, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
public static SymbolsKeyInfoSubNode create() {
return new SymbolsKeyInfoSubNodeGen();
}
}
@GeneratedBy(SymbolsHasKeysSubNode.class)
static final class SymbolsHasKeysSubNodeGen extends SymbolsHasKeysSubNode {
@CompilationFinal private int state_;
private SymbolsHasKeysSubNodeGen() {
}
@Override
public Object executeWithTarget(VirtualFrame frameValue, Object arg0Value) {
int state = state_;
if (state != 0 /* is-active accessWithTarget(EmptyGlobalBindings) */ && arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
return accessWithTarget(arg0Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
this.state_ = state = state | 0b1 /* add-active accessWithTarget(EmptyGlobalBindings) */;
lock.unlock();
hasLock = false;
return accessWithTarget(arg0Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null}, arg0Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
public static SymbolsHasKeysSubNode create() {
return new SymbolsHasKeysSubNodeGen();
}
}
@GeneratedBy(ReadSubNode.class)
static final class ReadSubNodeGen extends ReadSubNode {
@CompilationFinal private int state_;
private ReadSubNodeGen() {
}
@Override
public Object executeWithTarget(VirtualFrame frameValue, Object arg0Value, Object arg1Value) {
int state = state_;
if (state != 0 /* is-active accessWithTarget(EmptyGlobalBindings, String) */ && arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
if (arg1Value instanceof String) {
String arg1Value_ = (String) arg1Value;
return accessWithTarget(arg0Value_, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
if (arg1Value instanceof String) {
String arg1Value_ = (String) arg1Value;
this.state_ = state = state | 0b1 /* add-active accessWithTarget(EmptyGlobalBindings, String) */;
lock.unlock();
hasLock = false;
return accessWithTarget(arg0Value_, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
public static ReadSubNode create() {
return new ReadSubNodeGen();
}
}
@GeneratedBy(SymbolsKeysSubNode.class)
static final class SymbolsKeysSubNodeGen extends SymbolsKeysSubNode {
@CompilationFinal private int state_;
private SymbolsKeysSubNodeGen() {
}
@Override
public Object executeWithTarget(VirtualFrame frameValue, Object arg0Value) {
int state = state_;
if (state != 0 /* is-active accessWithTarget(EmptyGlobalBindings) */ && arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
return accessWithTarget(arg0Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
if (arg0Value instanceof EmptyGlobalBindings) {
EmptyGlobalBindings arg0Value_ = (EmptyGlobalBindings) arg0Value;
this.state_ = state = state | 0b1 /* add-active accessWithTarget(EmptyGlobalBindings) */;
lock.unlock();
hasLock = false;
return accessWithTarget(arg0Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null}, arg0Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
public static SymbolsKeysSubNode create() {
return new SymbolsKeysSubNodeGen();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy