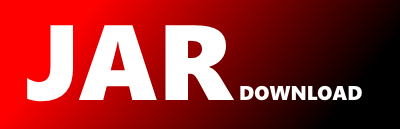
com.oracle.truffle.polyglot.HostExecuteNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-api Show documentation
Show all versions of truffle-api Show documentation
Truffle is a multi-language framework for executing dynamic languages
that achieves high performance when combined with Graal.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.polyglot;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.ExplodeLoop.LoopExplosionKind;
import com.oracle.truffle.api.profiles.ConditionProfile;
import com.oracle.truffle.api.profiles.ValueProfile;
import com.oracle.truffle.polyglot.HostMethodDesc.OverloadedMethod;
import com.oracle.truffle.polyglot.HostMethodDesc.SingleMethod;
import java.lang.reflect.Type;
import java.util.concurrent.locks.Lock;
@GeneratedBy(HostExecuteNode.class)
final class HostExecuteNodeGen extends HostExecuteNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@Child private FixedData fixed_cache;
@Child private VarArgsData varArgs_cache;
@Child private ToHostNode singleUncached_toJavaNode_;
@CompilationFinal private ConditionProfile singleUncached_isVarArgsProfile_;
@Child private OverloadedCachedData overloadedCached_cache;
@Child private ToHostNode overloadedUncached_toJavaNode_;
@CompilationFinal private ConditionProfile overloadedUncached_isVarArgsProfile_;
private HostExecuteNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
protected Object executeImpl(HostMethodDesc arg0Value, Object arg1Value, Object[] arg2Value, PolyglotLanguageContext arg3Value) {
int state = state_;
if (state != 0 /* is-active doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile) || doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) || doSingleUncached(SingleMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) || doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) || doOverloadedUncached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */) {
if ((state & 0b111) != 0 /* is-active doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile) || doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) || doSingleUncached(SingleMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */ && arg0Value instanceof SingleMethod) {
SingleMethod arg0Value_ = (SingleMethod) arg0Value;
if ((state & 0b1) != 0 /* is-active doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile) */ && (!(arg0Value_.isVarArgs()))) {
FixedData s1_ = this.fixed_cache;
while (s1_ != null) {
if ((arg0Value_ == s1_.cachedMethod_)) {
return doFixed(arg0Value_, arg1Value, arg2Value, arg3Value, s1_.cachedMethod_, s1_.toJavaNodes_, s1_.receiverProfile_);
}
s1_ = s1_.next_;
}
}
if ((state & 0b10) != 0 /* is-active doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) */ && (arg0Value_.isVarArgs())) {
VarArgsData s2_ = this.varArgs_cache;
while (s2_ != null) {
if ((arg0Value_ == s2_.cachedMethod_)) {
return doVarArgs(arg0Value_, arg1Value, arg2Value, arg3Value, s2_.cachedMethod_, s2_.toJavaNode_, s2_.receiverProfile_);
}
s2_ = s2_.next_;
}
}
if ((state & 0b100) != 0 /* is-active doSingleUncached(SingleMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */) {
return doSingleUncached(arg0Value_, arg1Value, arg2Value, arg3Value, this.singleUncached_toJavaNode_, this.singleUncached_isVarArgsProfile_);
}
}
if ((state & 0b11000) != 0 /* is-active doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) || doOverloadedUncached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */ && arg0Value instanceof OverloadedMethod) {
OverloadedMethod arg0Value_ = (OverloadedMethod) arg0Value;
if ((state & 0b1000) != 0 /* is-active doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) */) {
OverloadedCachedData s4_ = this.overloadedCached_cache;
while (s4_ != null) {
if ((arg0Value_ == s4_.cachedMethod_) && (HostExecuteNode.checkArgTypes(arg2Value, s4_.cachedArgTypes_, s4_.toJavaNode_, s4_.asVarArgs_))) {
return doOverloadedCached(arg0Value_, arg1Value, arg2Value, arg3Value, s4_.cachedMethod_, s4_.toJavaNode_, s4_.cachedArgTypes_, s4_.overload_, s4_.asVarArgs_, s4_.receiverProfile_);
}
s4_ = s4_.next_;
}
}
if ((state & 0b10000) != 0 /* is-active doOverloadedUncached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */) {
return doOverloadedUncached(arg0Value_, arg1Value, arg2Value, arg3Value, this.overloadedUncached_toJavaNode_, this.overloadedUncached_isVarArgsProfile_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(HostMethodDesc arg0Value, Object arg1Value, Object[] arg2Value, PolyglotLanguageContext arg3Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if (arg0Value instanceof SingleMethod) {
SingleMethod arg0Value_ = (SingleMethod) arg0Value;
if (((exclude & 0b1)) == 0 /* is-not-excluded doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile) */ && (!(arg0Value_.isVarArgs()))) {
int count1_ = 0;
FixedData s1_ = this.fixed_cache;
if ((state & 0b1) != 0 /* is-active doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile) */) {
while (s1_ != null) {
if ((arg0Value_ == s1_.cachedMethod_)) {
break;
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
// assert (arg0Value_ == s1_.cachedMethod_);
if (count1_ < (HostExecuteNode.LIMIT)) {
s1_ = new FixedData(fixed_cache);
s1_.cachedMethod_ = (arg0Value_);
s1_.toJavaNodes_ = (HostExecuteNode.createToHost(arg0Value_.getParameterCount()));
s1_.receiverProfile_ = (ValueProfile.createClassProfile());
this.fixed_cache = super.insert(s1_);
this.state_ = state = state | 0b1 /* add-active doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile) */;
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
return doFixed(arg0Value_, arg1Value, arg2Value, arg3Value, s1_.cachedMethod_, s1_.toJavaNodes_, s1_.receiverProfile_);
}
}
if (((exclude & 0b10)) == 0 /* is-not-excluded doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) */ && (arg0Value_.isVarArgs())) {
int count2_ = 0;
VarArgsData s2_ = this.varArgs_cache;
if ((state & 0b10) != 0 /* is-active doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) */) {
while (s2_ != null) {
if ((arg0Value_ == s2_.cachedMethod_)) {
break;
}
s2_ = s2_.next_;
count2_++;
}
}
if (s2_ == null) {
// assert (arg0Value_ == s2_.cachedMethod_);
if (count2_ < (HostExecuteNode.LIMIT)) {
s2_ = new VarArgsData(varArgs_cache);
s2_.cachedMethod_ = (arg0Value_);
s2_.toJavaNode_ = (ToHostNode.create());
s2_.receiverProfile_ = (ValueProfile.createClassProfile());
this.varArgs_cache = super.insert(s2_);
this.state_ = state = state | 0b10 /* add-active doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) */;
}
}
if (s2_ != null) {
lock.unlock();
hasLock = false;
return doVarArgs(arg0Value_, arg1Value, arg2Value, arg3Value, s2_.cachedMethod_, s2_.toJavaNode_, s2_.receiverProfile_);
}
}
this.singleUncached_toJavaNode_ = super.insert((ToHostNode.create()));
this.singleUncached_isVarArgsProfile_ = (ConditionProfile.createBinaryProfile());
this.exclude_ = exclude = exclude | 0b11 /* add-excluded doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile), doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) */;
this.fixed_cache = null;
this.varArgs_cache = null;
state = state & 0xfffffffc /* remove-active doFixed(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode[], ValueProfile), doVarArgs(SingleMethod, Object, Object[], PolyglotLanguageContext, SingleMethod, ToHostNode, ValueProfile) */;
this.state_ = state = state | 0b100 /* add-active doSingleUncached(SingleMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */;
lock.unlock();
hasLock = false;
return doSingleUncached(arg0Value_, arg1Value, arg2Value, arg3Value, this.singleUncached_toJavaNode_, this.singleUncached_isVarArgsProfile_);
}
if (arg0Value instanceof OverloadedMethod) {
OverloadedMethod arg0Value_ = (OverloadedMethod) arg0Value;
if (((exclude & 0b100)) == 0 /* is-not-excluded doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) */) {
int count4_ = 0;
OverloadedCachedData s4_ = this.overloadedCached_cache;
if ((state & 0b1000) != 0 /* is-active doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) */) {
while (s4_ != null) {
if ((arg0Value_ == s4_.cachedMethod_) && (HostExecuteNode.checkArgTypes(arg2Value, s4_.cachedArgTypes_, s4_.toJavaNode_, s4_.asVarArgs_))) {
break;
}
s4_ = s4_.next_;
count4_++;
}
}
if (s4_ == null) {
{
ToHostNode toJavaNode__ = (ToHostNode.create());
Type[] cachedArgTypes__ = (HostExecuteNode.createArgTypesArray(arg2Value));
SingleMethod overload__ = (HostExecuteNode.selectOverload(arg0Value_, arg2Value, arg3Value, cachedArgTypes__));
boolean asVarArgs__ = (HostExecuteNode.asVarArgs(arg2Value, overload__));
// assert (arg0Value_ == s4_.cachedMethod_);
if ((HostExecuteNode.checkArgTypes(arg2Value, cachedArgTypes__, toJavaNode__, asVarArgs__)) && count4_ < (HostExecuteNode.LIMIT)) {
s4_ = new OverloadedCachedData(overloadedCached_cache);
s4_.cachedMethod_ = (arg0Value_);
s4_.toJavaNode_ = toJavaNode__;
s4_.cachedArgTypes_ = cachedArgTypes__;
s4_.overload_ = overload__;
s4_.asVarArgs_ = asVarArgs__;
s4_.receiverProfile_ = (ValueProfile.createClassProfile());
this.overloadedCached_cache = super.insert(s4_);
this.state_ = state = state | 0b1000 /* add-active doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) */;
}
}
}
if (s4_ != null) {
lock.unlock();
hasLock = false;
return doOverloadedCached(arg0Value_, arg1Value, arg2Value, arg3Value, s4_.cachedMethod_, s4_.toJavaNode_, s4_.cachedArgTypes_, s4_.overload_, s4_.asVarArgs_, s4_.receiverProfile_);
}
}
this.overloadedUncached_toJavaNode_ = super.insert((ToHostNode.create()));
this.overloadedUncached_isVarArgsProfile_ = (ConditionProfile.createBinaryProfile());
this.exclude_ = exclude = exclude | 0b100 /* add-excluded doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) */;
this.overloadedCached_cache = null;
state = state & 0xfffffff7 /* remove-active doOverloadedCached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, OverloadedMethod, ToHostNode, Type[], SingleMethod, boolean, ValueProfile) */;
this.state_ = state = state | 0b10000 /* add-active doOverloadedUncached(OverloadedMethod, Object, Object[], PolyglotLanguageContext, ToHostNode, ConditionProfile) */;
lock.unlock();
hasLock = false;
return doOverloadedUncached(arg0Value_, arg1Value, arg2Value, arg3Value, this.overloadedUncached_toJavaNode_, this.overloadedUncached_isVarArgsProfile_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
FixedData s1_ = this.fixed_cache;
VarArgsData s2_ = this.varArgs_cache;
OverloadedCachedData s4_ = this.overloadedCached_cache;
if ((s1_ == null || s1_.next_ == null) && (s2_ == null || s2_.next_ == null) && (s4_ == null || s4_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static HostExecuteNode create() {
return new HostExecuteNodeGen();
}
@GeneratedBy(HostExecuteNode.class)
private static final class FixedData extends Node {
@Child FixedData next_;
@CompilationFinal SingleMethod cachedMethod_;
@Children ToHostNode[] toJavaNodes_;
@CompilationFinal ValueProfile receiverProfile_;
FixedData(FixedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(HostExecuteNode.class)
private static final class VarArgsData extends Node {
@Child VarArgsData next_;
@CompilationFinal SingleMethod cachedMethod_;
@Child ToHostNode toJavaNode_;
@CompilationFinal ValueProfile receiverProfile_;
VarArgsData(VarArgsData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(HostExecuteNode.class)
private static final class OverloadedCachedData extends Node {
@Child OverloadedCachedData next_;
@CompilationFinal OverloadedMethod cachedMethod_;
@Child ToHostNode toJavaNode_;
@CompilationFinal(dimensions = 1) Type[] cachedArgTypes_;
@CompilationFinal SingleMethod overload_;
@CompilationFinal boolean asVarArgs_;
@CompilationFinal ValueProfile receiverProfile_;
OverloadedCachedData(OverloadedCachedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy