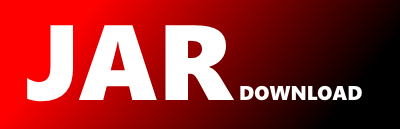
com.oracle.truffle.polyglot.ToHostNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-api Show documentation
Show all versions of truffle-api Show documentation
Truffle is a multi-language framework for executing dynamic languages
that achieves high performance when combined with Graal.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.polyglot;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.ExplodeLoop.LoopExplosionKind;
import java.lang.reflect.Type;
import java.util.concurrent.locks.Lock;
@GeneratedBy(ToHostNode.class)
final class ToHostNodeGen extends ToHostNode {
@CompilationFinal private int state_;
@CompilationFinal private int exclude_;
@CompilationFinal private CachedData cached_cache;
private ToHostNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public Object execute(Object arg0Value, Class> arg1Value, Type arg2Value, PolyglotLanguageContext arg3Value) {
int state = state_;
if (state != 0 /* is-active doNull(Object, Class<>, Type, PolyglotLanguageContext) || doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) || doGeneric(Object, Class<>, Type, PolyglotLanguageContext) */) {
if ((state & 0b1) != 0 /* is-active doNull(Object, Class<>, Type, PolyglotLanguageContext) */) {
if ((arg0Value == null)) {
return doNull(arg0Value, arg1Value, arg2Value, arg3Value);
}
}
if ((state & 0b10) != 0 /* is-active doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) */ && (arg0Value != null)) {
CachedData s2_ = this.cached_cache;
while (s2_ != null) {
if ((arg0Value.getClass() == s2_.cachedOperandType_) && (arg1Value == s2_.cachedTargetType_)) {
return doCached(arg0Value, arg1Value, arg2Value, arg3Value, s2_.cachedOperandType_, s2_.cachedTargetType_);
}
s2_ = s2_.next_;
}
}
if ((state & 0b100) != 0 /* is-active doGeneric(Object, Class<>, Type, PolyglotLanguageContext) */) {
if ((arg0Value != null)) {
return doGeneric(arg0Value, arg1Value, arg2Value, arg3Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(Object arg0Value, Class> arg1Value, Type arg2Value, PolyglotLanguageContext arg3Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if ((arg0Value == null)) {
this.state_ = state = state | 0b1 /* add-active doNull(Object, Class<>, Type, PolyglotLanguageContext) */;
lock.unlock();
hasLock = false;
return doNull(arg0Value, arg1Value, arg2Value, arg3Value);
}
if ((exclude) == 0 /* is-not-excluded doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) */ && (arg0Value != null)) {
int count2_ = 0;
CachedData s2_ = this.cached_cache;
if ((state & 0b10) != 0 /* is-active doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) */) {
while (s2_ != null) {
if ((arg0Value.getClass() == s2_.cachedOperandType_) && (arg1Value == s2_.cachedTargetType_)) {
break;
}
s2_ = s2_.next_;
count2_++;
}
}
if (s2_ == null) {
{
Class> cachedOperandType__ = (arg0Value.getClass());
if ((arg0Value.getClass() == cachedOperandType__)) {
// assert (arg1Value == s2_.cachedTargetType_);
if (count2_ < (ToHostNode.LIMIT)) {
s2_ = new CachedData(cached_cache);
s2_.cachedOperandType_ = cachedOperandType__;
s2_.cachedTargetType_ = (arg1Value);
this.cached_cache = s2_;
this.state_ = state = state | 0b10 /* add-active doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) */;
}
}
}
}
if (s2_ != null) {
lock.unlock();
hasLock = false;
return doCached(arg0Value, arg1Value, arg2Value, arg3Value, s2_.cachedOperandType_, s2_.cachedTargetType_);
}
}
if ((arg0Value != null)) {
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) */;
this.cached_cache = null;
state = state & 0xfffffffd /* remove-active doCached(Object, Class<>, Type, PolyglotLanguageContext, Class<>, Class<>) */;
this.state_ = state = state | 0b100 /* add-active doGeneric(Object, Class<>, Type, PolyglotLanguageContext) */;
lock.unlock();
hasLock = false;
return doGeneric(arg0Value, arg1Value, arg2Value, arg3Value);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
CachedData s2_ = this.cached_cache;
if ((s2_ == null || s2_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static ToHostNode create() {
return new ToHostNodeGen();
}
@GeneratedBy(ToHostNode.class)
private static final class CachedData {
@CompilationFinal CachedData next_;
@CompilationFinal Class> cachedOperandType_;
@CompilationFinal Class> cachedTargetType_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy